SkyComponent
#include <skycomponent.h>
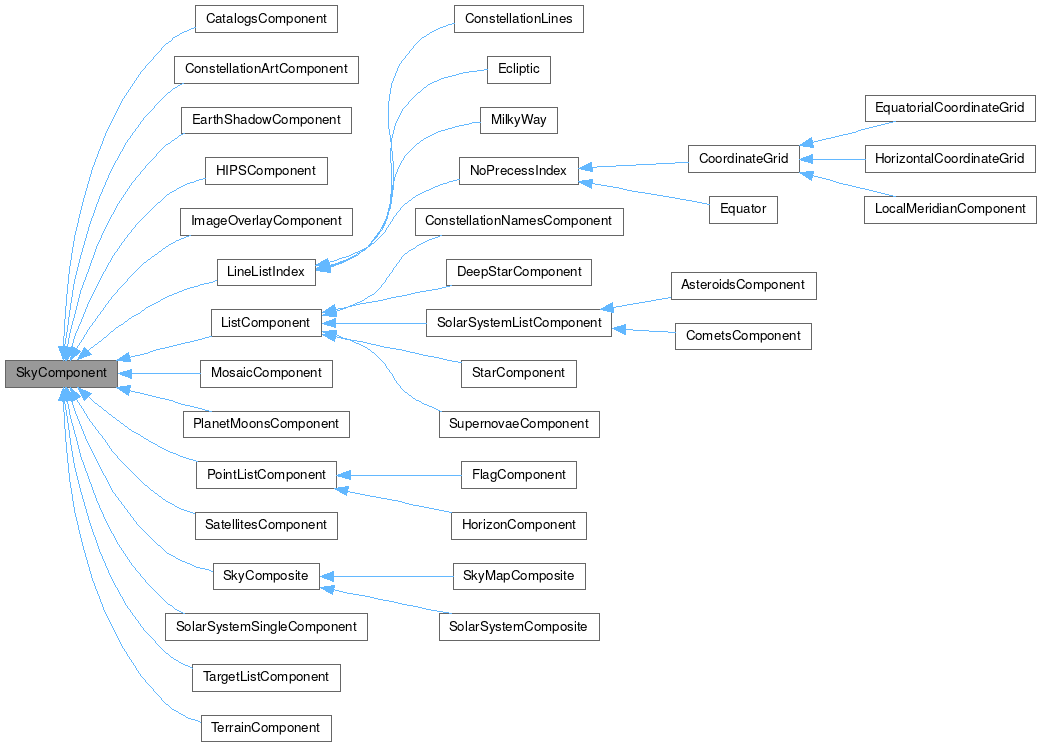
Public Member Functions | |
SkyComponent (SkyComposite *parent=nullptr) | |
virtual void | draw (SkyPainter *skyp)=0 |
virtual void | drawTrails (SkyPainter *skyp) |
virtual void | emitProgressText (const QString &message) |
virtual SkyObject * | findByName (const QString &name, bool exact=true) |
QHash< int, QVector< QPair< QString, const SkyObject * > > > & | objectLists () |
QVector< QPair< QString, const SkyObject * > > & | objectLists (int type) |
QHash< int, QStringList > & | objectNames () |
QStringList & | objectNames (int type) |
virtual SkyObject * | objectNearest (SkyPoint *p, double &maxrad) |
virtual void | objectsInArea (QList< SkyObject * > &list, const SkyRegion ®ion) |
SkyComposite * | parent () |
void | removeFromLists (const SkyObject *obj) |
void | removeFromNames (const SkyObject *obj) |
virtual bool | selected () |
virtual void | update (KSNumbers *) |
virtual void | updateMoons (KSNumbers *) |
virtual void | updateSolarSystemBodies (KSNumbers *) |
Detailed Description
SkyComponent represents an object on the sky map.
This may be a star, a planet or an imaginary line like the equator. The SkyComponent uses the composite design pattern. So if a new object type is needed, it has to be derived from this class and must be added into the hierarchy of components.
Overview
KStars utilizes the Composite/Component design pattern to store all elements that must be drawn on the SkyMap. In this design pattern, elements to be drawn in the map are called "Components", and they are hierarchically organized into groups called "Composites". A Composite can contain other Composites, as well as Components. From an organizational point of view, you can think of Composites and Components as being analogous to "Directories" and "Files" in a computer filesystem.
The advantage of this design pattern is that it minimizes code duplication and maximizes modularity. There is a set of operations which all Components must perform periodically (such as drawing on screen, or updating position), but each Component may perform its task slightly differently. Thus, each Component can implement its own version of the functions, and each Composite calls on its child Components to execute the desired function. For example, if we wanted to draw all Components in the SkyMap, we would simply need to call "skyComposite()->draw()". SkyComposite is the "top-level" Composite; its "draw()" function simply calls draw() on each of its children. Its child Components will draw themselves, while its child Composites will call "draw()" for each of their children. Note that we don't need to know any implementation details; when we need to draw the elements, we just tell the top-level Composite to spread the word.
Class Inheritance
The base class in this directory is SkyComponent; all other classes are derived from it (directly or indirectly). Its most important derivative is SkyComposite, the base classes for all Composites.
FIXME: There is no SingleComponent From SkyComponent, we derive three important subclasses: SingleComponent, ListComponent, and PointListComponent. SingleComponent represents a sky element consisting of a single SkyObject, such as the Sun. ListComponent represents a list of SkyObjects, such as the Asteroids. PointListComponent represents a list of SkyPoints (not SkyObjects), such as the Ecliptic. Almost all other Components are derived from one of these three classes (two Components are derived directly from SkyComponent).
The Solar System bodies require some special treatment. For example, only solar system bodies may have attached trails, and they move across the sky, so their positions must be updated frequently. To handle these features, we derive SolarSystemComposite from SkyComposite to be the container for all solar system Components. In addition, we derive SolarSystemSingleComponent from SingleComponent, and SolarSystemListComponent from ListComponent. These classes simply add functions to handle Trails, and to update the positions of the bodies. Also, they contain KSPlanetBase objects, instead of SkyObjects.
The Sun, Moon and Pluto each get a unique Component class, derived from SolarSystemSingleComponent (this is needed because each of these bodies uses a unique class derived from KSPlanetBase: KSSun, KSMoon, and KSPluto). The remaining major planets are each represented with a PlanetComponent object, which is also derived from SolarSystemSingleComponent. Finally, we have AsteroidsComponent and CometsComponent, each of which is derived from SolarSystemListComponent.
Next, we have the Components derived from PointListComponent. These Components represent imaginary guide lines in the sky, such as constellation boundaries or the coordinate grid. They are listed above, and most of them don't require further comment. The GuidesComposite contains two sub-Composites: CoordinateGridComposite, and ConstellationLinesComposite. Both of these contain a number of PointListComponents: CoordinateGridComposite contains one PointsListComponent for each circle in the grid, and ConstellationLineComposite contains one PointsListComponent for each constellation (representing the "stick figure" of the constellation).
Finally, we have the Components which don't inherit from either SingleComponent, ListComponent, or PointListComponent: PlanetMoonsComponent inherits from SkyComponent directly, because the planet moons have their own class (PlanetMoons) not derived directly from a SkyPoint.
DeepStarComponent and StarComponent
StarComponent and DeepStarComponent manage stars in KStars. StarComponent maintains a QVector of instances of DeepStarComponents and takes care of calling their draw routines etc. The machinery for handling stars is documented in great detail in Stars
Definition at line 95 of file skycomponent.h.
Constructor & Destructor Documentation
◆ SkyComponent()
|
explicit |
Constructor parent
pointer to the parent SkyComposite.
Definition at line 13 of file skycomponent.cpp.
Member Function Documentation
◆ draw()
|
pure virtual |
Draw the object on the SkyMap skyp
a pointer to the SkyPainter to use.
Implemented in AsteroidsComponent, CatalogsComponent, CometsComponent, ConstellationArtComponent, ConstellationNamesComponent, DeepStarComponent, EarthShadowComponent, Ecliptic, Equator, FlagComponent, HIPSComponent, HorizonComponent, ImageOverlayComponent, LineListIndex, MilkyWay, MosaicComponent, PlanetMoonsComponent, SatellitesComponent, SkyComposite, SkyMapComposite, SolarSystemSingleComponent, StarComponent, SupernovaeComponent, TargetListComponent, and TerrainComponent.
◆ drawTrails()
|
virtual |
Draw trails for objects.
Reimplemented in PlanetMoonsComponent, SatellitesComponent, SolarSystemComposite, SolarSystemListComponent, and SolarSystemSingleComponent.
Definition at line 33 of file skycomponent.cpp.
◆ emitProgressText()
|
virtual |
Emit signal about progress.
Reimplemented in SkyMapComposite.
Definition at line 18 of file skycomponent.cpp.
◆ findByName()
Search the children of this SkyComponent for a SkyObject whose name matches the argument name
the name to be matched exact
If true, it will return an exact match, otherwise it can return a partial match.
- Returns
- a pointer to the SkyObject whose name matches the argument, or a nullptr pointer if no match was found.
- Note
- This function simply returns the nullptr pointer; it is reimplemented in various sub-classes
Reimplemented in CatalogsComponent, ListComponent, PlanetMoonsComponent, SatellitesComponent, SkyComposite, SkyMapComposite, and SolarSystemSingleComponent.
Definition at line 23 of file skycomponent.cpp.
◆ objectLists() [1/2]
Definition at line 198 of file skycomponent.h.
◆ objectLists() [2/2]
Definition at line 203 of file skycomponent.h.
◆ objectNames() [1/2]
|
inline |
Definition at line 188 of file skycomponent.h.
◆ objectNames() [2/2]
|
inline |
Definition at line 193 of file skycomponent.h.
◆ objectNearest()
Find the SkyObject nearest the given SkyPoint.
Look for a SkyObject that is nearer to point p than maxrad. If one is found, then maxrad is reset to the separation of the new nearest object. p
pointer to the SkyPoint to search around maxrad
reference to current search radius in degrees
- Returns
- a pointer to the nearest SkyObject
- Note
- This function simply returns a nullptr pointer; it is reimplemented in various sub-classes.
Reimplemented in AsteroidsComponent, CatalogsComponent, DeepStarComponent, ListComponent, PlanetMoonsComponent, SatellitesComponent, SkyComposite, SkyMapComposite, SolarSystemSingleComponent, StarComponent, and SupernovaeComponent.
Definition at line 28 of file skycomponent.cpp.
◆ objectsInArea()
Searches the region(s) and appends the SkyObjects found to the list of sky objects.
Look for a SkyObject that is in one of the regions If found, then append to the list of sky objects list
list of SkyObject to which matching list has to be appended to region
defines the regions in which the search for SkyObject should be done within
- Returns
- void
- Note
- This function simply returns; it is reimplemented in various sub-classes.
Reimplemented in CatalogsComponent, and StarComponent.
Definition at line 37 of file skycomponent.cpp.
◆ parent()
|
inline |
- Returns
- Parent of component. If there is no parent returns nullptr.
Definition at line 137 of file skycomponent.h.
◆ removeFromLists()
void SkyComponent::removeFromLists | ( | const SkyObject * | obj | ) |
Definition at line 78 of file skycomponent.cpp.
◆ removeFromNames()
void SkyComponent::removeFromNames | ( | const SkyObject * | obj | ) |
Definition at line 65 of file skycomponent.cpp.
◆ selected()
|
inlinevirtual |
- Returns
- true if component is to be drawn on the map.
Reimplemented in AsteroidsComponent, CatalogsComponent, CometsComponent, ConstellationLines, ConstellationNamesComponent, CoordinateGrid, DeepStarComponent, EarthShadowComponent, Ecliptic, Equator, EquatorialCoordinateGrid, FlagComponent, HIPSComponent, HorizonComponent, HorizontalCoordinateGrid, ImageOverlayComponent, LocalMeridianComponent, MilkyWay, MosaicComponent, PlanetMoonsComponent, SatellitesComponent, SolarSystemComposite, SolarSystemSingleComponent, StarComponent, SupernovaeComponent, and TerrainComponent.
Definition at line 131 of file skycomponent.h.
◆ update()
|
inlinevirtual |
Update the sky position(s) of this component.
This function usually just updates the Horizontal (Azimuth/Altitude) coordinates of its member object(s). However, the precession and nutation must also be recomputed periodically. num
Pointer to the KSNumbers object
- See also
- SingleComponent::update()
- ListComponent::update()
- ConstellationBoundaryComponent::update()
Reimplemented in ConstellationNamesComponent, DeepStarComponent, EarthShadowComponent, FlagComponent, HorizonComponent, HorizontalCoordinateGrid, ListComponent, LocalMeridianComponent, PlanetMoonsComponent, PointListComponent, SatellitesComponent, SkyComposite, SkyMapComposite, SolarSystemComposite, SolarSystemListComponent, SolarSystemSingleComponent, StarComponent, and SupernovaeComponent.
Definition at line 126 of file skycomponent.h.
◆ updateMoons()
|
inlinevirtual |
Reimplemented in SkyMapComposite, and SolarSystemSingleComponent.
Definition at line 128 of file skycomponent.h.
◆ updateSolarSystemBodies()
|
inlinevirtual |
Reimplemented in SkyMapComposite, SolarSystemListComponent, and SolarSystemSingleComponent.
Definition at line 127 of file skycomponent.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.