LineListIndex
#include <linelistindex.h>
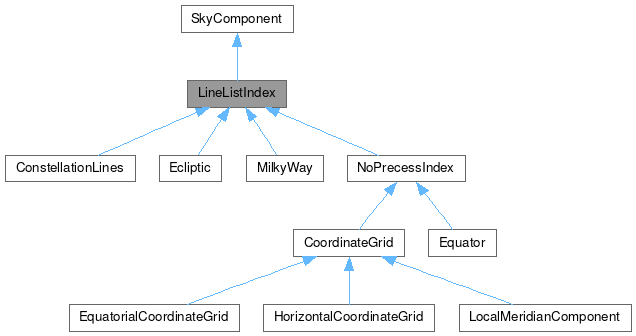
Public Member Functions | |
LineListIndex (SkyComposite *parent, const QString &name="") | |
void | draw (SkyPainter *skyp) override |
virtual void | JITupdate (LineList *lineList) |
![]() | |
SkyComponent (SkyComposite *parent=nullptr) | |
virtual void | drawTrails (SkyPainter *skyp) |
virtual void | emitProgressText (const QString &message) |
virtual SkyObject * | findByName (const QString &name, bool exact=true) |
QHash< int, QVector< QPair< QString, const SkyObject * > > > & | objectLists () |
QVector< QPair< QString, const SkyObject * > > & | objectLists (int type) |
QHash< int, QStringList > & | objectNames () |
QStringList & | objectNames (int type) |
virtual SkyObject * | objectNearest (SkyPoint *p, double &maxrad) |
virtual void | objectsInArea (QList< SkyObject * > &list, const SkyRegion ®ion) |
SkyComposite * | parent () |
void | removeFromLists (const SkyObject *obj) |
void | removeFromNames (const SkyObject *obj) |
virtual bool | selected () |
virtual void | update (KSNumbers *) |
virtual void | updateMoons (KSNumbers *) |
virtual void | updateSolarSystemBodies (KSNumbers *) |
Protected Member Functions | |
void | appendBoth (const std::shared_ptr< LineList > &lineList) |
void | appendLine (const std::shared_ptr< LineList > &lineList) |
void | appendPoly (const std::shared_ptr< LineList > &lineList) |
virtual MeshBufNum_t | drawBuffer () |
void | drawFilled (SkyPainter *skyp) |
void | drawLines (SkyPainter *skyp) |
virtual const IndexHash & | getIndexHash (LineList *lineList) |
void | intro () |
virtual LineListLabel * | label () |
LineListList | listList () const |
QString | name () const |
virtual void | preDraw (SkyPainter *skyp) |
void | reindexLines () |
void | removeLine (const std::shared_ptr< LineList > &lineList) |
virtual SkipHashList * | skipList (LineList *lineList) |
SkyMesh * | skyMesh () |
void | summary () |
Detailed Description
Contains almost all the code needed for indexing and drawing and clipping lines and polygons.
- Version
- 0.1
Definition at line 29 of file linelistindex.h.
Constructor & Destructor Documentation
◆ LineListIndex()
|
explicit |
Constructor Simply set the internal skyMesh, parent, and name.
- Parameters
-
parent Pointer to the parent SkyComponent object name name of the subclass used for debugging
Definition at line 34 of file linelistindex.cpp.
Member Function Documentation
◆ appendBoth()
|
protected |
a convenience method that adds a lineList to both the lineIndex and the polyIndex.
Definition at line 100 of file linelistindex.cpp.
◆ appendLine()
|
protected |
Typically called from within a subclasses constructors.
Adds the trixels covering the outline of lineList to the lineIndex.
Definition at line 63 of file linelistindex.cpp.
◆ appendPoly()
|
protected |
Typically called from within a subclasses constructors.
Adds the trixels covering the full lineList to the polyIndex.
Definition at line 82 of file linelistindex.cpp.
◆ draw()
|
overridevirtual |
The top level draw routine.
Draws all the LineLists for any subclass in one fell swoop which minimizes some of the loop overhead. Overridden by MilkWay so it can decide whether to draw outlines or filled. Therefore MilkyWay does not need to override preDraw(). The MilkyWay draw() routine calls all of the more specific draw() routines below.
Implements SkyComponent.
Reimplemented in MilkyWay.
Definition at line 158 of file linelistindex.cpp.
◆ drawBuffer()
|
inlineprotectedvirtual |
a callback overridden by NoPrecessIndex so it can use the drawing code with the non-reverse-precessed mesh buffer.
Reimplemented in NoPrecessIndex.
Definition at line 142 of file linelistindex.h.
◆ drawFilled()
|
protected |
Draws all the lines in m_listList as filled polygons in float mode.
Definition at line 203 of file linelistindex.cpp.
◆ drawLines()
|
protected |
Draws all the lines in m_listList as simple lines in float mode.
Definition at line 180 of file linelistindex.cpp.
◆ getIndexHash()
Returns an IndexHash from the SkyMesh that contains the set of trixels that cover lineList.
Overridden by SkipListIndex so it can pass SkyMesh an IndexHash indicating which line segments should not be indexed
- Parameters
-
lineList contains the list of points to be covered.
Reimplemented in ConstellationLines, and MilkyWay.
Definition at line 42 of file linelistindex.cpp.
◆ intro()
|
protected |
displays a message that we are loading m_name.
Also prints out the message if skyMesh debug is greater than zero.
Definition at line 234 of file linelistindex.cpp.
◆ JITupdate()
|
virtual |
this is called from within the draw routines when the updateID of the lineList is stale.
It is virtual because different subclasses have different update routines. NoPrecessIndex doesn't precess in the updates and ConstellationLines must update its points as stars, not points. that doesn't precess the points.
Reimplemented in ConstellationLines, and NoPrecessIndex.
Definition at line 129 of file linelistindex.cpp.
◆ label()
|
inlineprotectedvirtual |
Definition at line 161 of file linelistindex.h.
◆ listList()
|
inlineprotected |
Definition at line 163 of file linelistindex.h.
◆ name()
|
inlineprotected |
retrieve name of object
Definition at line 84 of file linelistindex.h.
◆ preDraw()
|
protectedvirtual |
Gives the subclasses access to the top of the draw() method.
Typically used for setting the QPen, etc. in the QPainter being passed in. Defaults to setting a thin white pen.
Reimplemented in ConstellationLines, CoordinateGrid, Equator, EquatorialCoordinateGrid, HorizontalCoordinateGrid, and LocalMeridianComponent.
Definition at line 153 of file linelistindex.cpp.
◆ reindexLines()
|
protected |
as the name says, recreates the lineIndex using the LineLists in the previous index.
Since we are indexing everything at J2000 this is only used by ConstellationLines which needs to reindex because of the proper motion of the stars.
Definition at line 108 of file linelistindex.cpp.
◆ removeLine()
|
protected |
Definition at line 47 of file linelistindex.cpp.
◆ skipList()
|
protectedvirtual |
Also overridden by SkipListIndex.
Controls skipping inside of the draw() routines. The default behavior is to simply return a null pointer.
FIXME: I don't think that the SkipListIndex class even exists – hdevalence
Reimplemented in MilkyWay.
Definition at line 174 of file linelistindex.cpp.
◆ skyMesh()
|
inlineprotected |
Returns the SkyMesh object.
Definition at line 99 of file linelistindex.h.
◆ summary()
|
protected |
prints out some summary statistics if the skyMesh debug is greater than 1.
Definition at line 241 of file linelistindex.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.