SkyMesh
#include <skymesh.h>
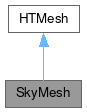
Public Member Functions | |
void | aperture (SkyPoint *center, double radius, MeshBufNum_t bufNum=DRAW_BUF) |
int | debug () const |
void | debug (int debug) |
void | draw (QPainter &psky, MeshBufNum_t bufNum=DRAW_BUF) |
DrawID | drawID () const |
int | incDrawID () |
Trixel | index (const SkyPoint *p) |
bool | inDraw () const |
void | inDraw (bool inDraw) |
const SkyRegion & | skyRegion (const SkyPoint &p1, const SkyPoint &p2) |
Stars and CLines | |
Routines used for indexing stars and CLines. The following four routines are used for indexing stars and CLines. They differ from the normal routines because they take proper motion into account while the normal routines always index the J2000 position. Since the proper motion depends on time, it is essential to call setKSNumbers to set the time before doing the indexing. The default value is J2000. | |
void | setKSNumbers (KSNumbers *num) |
Trixel | indexStar (StarObject *star) |
void | indexStar (StarObject *star1, StarObject *star2) |
const IndexHash & | indexStarLine (SkyList *points) |
Multi Shape Index Routines | |
void | index (const QPointF &p1, const QPointF &p2, const QPointF &p3) |
void | index (const QPointF &p1, const QPointF &p2, const QPointF &p3, const QPointF &p4) |
void | index (const SkyPoint *center, double radius, MeshBufNum_t bufNum=DRAW_BUF) |
void | index (const SkyPoint *p1, const SkyPoint *p2) |
void | index (const SkyPoint *p1, const SkyPoint *p2, const SkyPoint *p3) |
void | index (const SkyPoint *p1, const SkyPoint *p2, const SkyPoint *p3, const SkyPoint *p4) |
IndexHash Routines | |
The follow routines are used to index SkyList data structures. They fill a QHash with the indices of the trixels that cover the data structure. These are all used as callbacks in the LineListIndex subclasses so they can use the same indexing code to index different data structures. See also indexStarLine() above. | |
const IndexHash & | indexLine (SkyList *points) |
const IndexHash & | indexLine (SkyList *points, IndexHash *skip) |
const IndexHash & | indexPoly (SkyList *points) |
const IndexHash & | indexPoly (const QPolygonF *points) |
![]() | |
HTMesh (int level, int buildLevel, int numBuffers=1) | |
Trixel | index (double ra, double dec) const |
void | intersect (double ra, double dec, double radius, BufNum bufNum=0) |
void | intersect (double ra1, double dec1, double ra2, double dec2, BufNum bufNum=0) |
void | intersect (double ra1, double dec1, double ra2, double dec2, double ra3, double dec3, BufNum bufNum=0) |
void | intersect (double ra1, double dec1, double ra2, double dec2, double ra3, double dec3, double ra4, double dec4, BufNum bufNum=0) |
int | intersectSize (BufNum bufNum=0) |
int | level () const |
MeshBuffer * | meshBuffer (BufNum bufNum=0) |
void | setDebug (int debug) |
int | size () const |
void | vertices (Trixel id, double *ra1, double *dec1, double *ra2, double *dec2, double *ra3, double *dec3) |
Static Public Member Functions | |
static SkyMesh * | Create (int level) |
static SkyMesh * | Instance () |
static SkyMesh * | Instance (int level) |
Protected Member Functions | |
SkyMesh (int level) | |
SkyMesh (SkyMesh &skyMesh) | |
Detailed Description
Provides an interface to the Hierarchical Triangular Mesh (HTM) library written by A.
Szalay, John Doug Reynolds, Jim Gray, and Peter Z. Kunszt.
HTM divides the celestial sphere up into little triangles (trixels) and gives each triangle a unique integer id. We index objects by putting a pointer to the object in a data structure accessible by the id number, for example QList<QList<StarObject*> or QHash<int, QList<LineListComponent*>.
Use aperture(centerPoint, radius) to get a list of trixels visible in the circle. Then use a MeshIterator to iterate over the indices of the visible trixels. Look up each index in the top level QHash or QList data structure and the union of all the elements of the QList's returned will contain all the objects visible in the circle in the sky specified in the aperture() call.
The drawID is used for extended objects that may be covered by more than one trixel. Every time aperture() is called, drawID is incremented by one. Extended objects should each contain their own drawID. If an object's drawID is the same as the current drawID then it shouldn't be drawn again because it was already drawn in this draw cycle. If the drawID is different then it should be drawn and then the drawID should be set to the current drawID() so it doesn't get drawn again this cycle.
The list of visible trixels found from an aperture() call or one of the primitive index() by creating a new MeshIterator instance:
MeshIterator( HTMesh *mesh )
The MeshIterator has its own bool hasNext(), int next(), and int size() methods for iterating through the integer indices of the found trixels or for just getting the total number of found trixels.
Constructor & Destructor Documentation
◆ SkyMesh()
|
protected |
Definition at line 49 of file skymesh.cpp.
Member Function Documentation
◆ aperture()
void SkyMesh::aperture | ( | SkyPoint * | center, |
double | radius, | ||
MeshBufNum_t | bufNum = DRAW_BUF ) |
finds the set of trixels that cover the circular aperture specified after first performing a reverse precession correction on the center so we don't have to re-index objects simply due to precession.
The drawID also gets incremented which is useful for drawing extended objects. Typically a safety factor of about one degree is added to the radius to account for proper motion, refraction and other imperfections.
- Parameters
-
center Center of the aperture radius Radius of the aperture in degrees bufNum Buffer to use
- Note
- See HTMesh.h for more
Definition at line 56 of file skymesh.cpp.
◆ Create()
|
static |
creates the single instance of SkyMesh.
The level indicates how fine a mesh we will use. The number of triangles (trixels) in the mesh will be 8 * 4^level so a mesh of level 5 will have 8 * 4^5 = 8 * 2^10 = 8192 trixels. The size of the triangles are roughly pi / * 2^(level + 1) so a level 5 mesh will have triangles size roughly of .05 radians or 2.8 degrees.
Definition at line 25 of file skymesh.cpp.
◆ debug() [1/2]
|
inline |
Returns the debug level.
This is used as a global debug level for LineListIndex and its subclasses.
◆ debug() [2/2]
|
inline |
◆ draw()
void SkyMesh::draw | ( | QPainter & | psky, |
MeshBufNum_t | bufNum = DRAW_BUF ) |
Draws the outline of all the trixels in the specified buffer.
This was very useful during debugging. I don't precess the points because I mainly use it with the IN_CONSTELL_BUF which is not precessed. We will probably have buffers serve double and triple duty in the production code to save space in which case this function may become less useful.
Definition at line 276 of file skymesh.cpp.
◆ drawID()
|
inline |
- Returns
- the current drawID which gets incremented each time aperture() is called.
◆ incDrawID()
|
inline |
◆ index() [1/7]
finds the indices of the trixels that cover the triangle specified by the three QPointF's.
Definition at line 116 of file skymesh.cpp.
◆ index() [2/7]
void SkyMesh::index | ( | const QPointF & | p1, |
const QPointF & | p2, | ||
const QPointF & | p3, | ||
const QPointF & | p4 ) |
Finds the trixels needed to cover the quadrilateral specified by the four QPointF's.
Definition at line 121 of file skymesh.cpp.
◆ index() [3/7]
void SkyMesh::index | ( | const SkyPoint * | center, |
double | radius, | ||
MeshBufNum_t | bufNum = DRAW_BUF ) |
finds the indices of the trixels covering the circle specified by center and radius.
Definition at line 94 of file skymesh.cpp.
◆ index() [4/7]
Trixel SkyMesh::index | ( | const SkyPoint * | p | ) |
returns the index of the trixel containing p.
Definition at line 74 of file skymesh.cpp.
◆ index() [5/7]
finds the indices of the trixels covering the line segment connecting p1 and p2.
Definition at line 99 of file skymesh.cpp.
◆ index() [6/7]
finds the indices of the trixels covering the triangle specified by vertices: p1, p2, and p3.
Definition at line 104 of file skymesh.cpp.
◆ index() [7/7]
void SkyMesh::index | ( | const SkyPoint * | p1, |
const SkyPoint * | p2, | ||
const SkyPoint * | p3, | ||
const SkyPoint * | p4 ) |
finds the indices of the trixels covering the quadralateral specified by the vertices: p1, p2, p3, and p4.
Definition at line 110 of file skymesh.cpp.
◆ indexLine() [1/2]
fills a QHash with the trixel indices needed to cover all the line segments specified in the QVector<SkyPoints*> points.
- Parameters
-
points the points of the line segment. The debug mode causes extra info to be printed.
Definition at line 126 of file skymesh.cpp.
◆ indexLine() [2/2]
as above but allows for skipping the indexing of some of the points.
- Parameters
-
points the line segment to be indexed. skip a hash indicating which points to skip debugging info.
Definition at line 157 of file skymesh.cpp.
◆ indexPoly() [1/2]
does the same as above but with a QPolygonF as the container for the points.
Definition at line 240 of file skymesh.cpp.
◆ indexPoly() [2/2]
fills a QHash with the trixel indices needed to cover the polygon specified in the QList<SkyPoints*> points.
There is no version with a skip parameter because it does not make sense to skip some of the edges of a filled polygon.
- Parameters
-
points the points of the line segment. The debug mode causes extra info to be printed.
Definition at line 208 of file skymesh.cpp.
◆ indexStar() [1/2]
Trixel SkyMesh::indexStar | ( | StarObject * | star | ) |
returns the trixel that contains the star at the set time with proper motion taken into account but not precession.
This is a feature not a bug.
Definition at line 79 of file skymesh.cpp.
◆ indexStar() [2/2]
void SkyMesh::indexStar | ( | StarObject * | star1, |
StarObject * | star2 ) |
fills the default buffer with all the trixels needed to cover the line connecting the two stars.
Definition at line 86 of file skymesh.cpp.
◆ indexStarLine()
Fills a hash with all the trixels needed to cover all the line segments in the SkyList where each SkyPoint is assumed to be a star and proper motion is taken into account.
Used only by ConstellationsLines.
Definition at line 131 of file skymesh.cpp.
◆ inDraw() [1/2]
◆ inDraw() [2/2]
◆ Instance() [1/2]
|
static |
returns the default instance of SkyMesh or null if it has not yet been created.
Definition at line 39 of file skymesh.cpp.
◆ Instance() [2/2]
|
static |
returns the instance of SkyMesh corresponding to the given level
- Returns
- the instance of SkyMesh at the given level, or nullptr if it has not yet been created.
Definition at line 44 of file skymesh.cpp.
◆ setKSNumbers()
|
inline |
◆ skyRegion()
returns the sky region needed to cover the rectangle defined by two SkyPoints p1 and p2
Definition at line 312 of file skymesh.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.