HTMesh
#include <HTMesh.h>
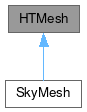
Public Member Functions | |
HTMesh (int level, int buildLevel, int numBuffers=1) | |
Trixel | index (double ra, double dec) const |
void | intersect (double ra, double dec, double radius, BufNum bufNum=0) |
void | intersect (double ra1, double dec1, double ra2, double dec2, BufNum bufNum=0) |
void | intersect (double ra1, double dec1, double ra2, double dec2, double ra3, double dec3, BufNum bufNum=0) |
void | intersect (double ra1, double dec1, double ra2, double dec2, double ra3, double dec3, double ra4, double dec4, BufNum bufNum=0) |
int | intersectSize (BufNum bufNum=0) |
int | level () const |
MeshBuffer * | meshBuffer (BufNum bufNum=0) |
void | setDebug (int debug) |
int | size () const |
void | vertices (Trixel id, double *ra1, double *dec1, double *ra2, double *dec2, double *ra3, double *dec3) |
Detailed Description
HTMesh was originally intended to be a simple interface to the HTM library for the KStars project that would hide some of the complexity.
But it has gained some complexity of its own.
The most complex addition was the routine that performs an intersection on a line segment, finding all the trixels that cover the line. Perhaps there is an easier and faster way to do it. Right now we convert to cartesian coordinates to take the cross product of the two input vectors in order to create a perpendicular which is used to form a very narrow triangle that contains the original line segment as one of its long legs.
Error detection and prevention added a little bit more complexity. The raw HTM library is vulnerable to misbehavior if the polygon intersection routines are given duplicate consecutive points, including the first point of a polygon duplicating the last point. We test for duplicated points now. If they are found, we drop down to the intersection routine with one fewer vertices, ending at the line segment. If the two ends of a line segment are much close together than the typical edge of a trixel then we simply index each point separately and the result set is consists of the union of these two trixels.
The final (I hope) level of complexity was the addition of multiple results buffers. You can set the number of results buffers in the HTMesh constructor. Since each buffer has to be able to hold one integer for each trixel in the mesh, multiple buffers can quickly eat up memory. The default is just one buffer and all routines that use the buffers default to using the just the first buffer.
NOTE: all Right Ascensions (ra) and Declinations (dec) are in degrees.
Constructor & Destructor Documentation
◆ HTMesh()
HTMesh::HTMesh | ( | int | level, |
int | buildLevel, | ||
int | numBuffers = 1 ) |
constructor.
- Parameters
-
level is passed on to the underlying SpatialIndex buildLevel is also passed on to the SpatialIndex numBuffers controls how many output buffers are created. Don't use more than require because they eat up mucho RAM. The default is just one output buffer.
Definition at line 22 of file HTMesh.cpp.
◆ ~HTMesh()
HTMesh::~HTMesh | ( | ) |
Definition at line 64 of file HTMesh.cpp.
Member Function Documentation
◆ index()
Trixel HTMesh::index | ( | double | ra, |
double | dec ) const |
returns the index of the trixel that contains the specified point.
Definition at line 72 of file HTMesh.cpp.
◆ intersect() [1/4]
void HTMesh::intersect | ( | double | ra, |
double | dec, | ||
double | radius, | ||
BufNum | bufNum = 0 ) |
NOTE: The intersect() routines below are all used to find the trixels needed to cover a geometric object: circle, line, triangle, and quadrilateral.
Since the number of trixels needed can be large and is not known a priori, you must construct a MeshIterator to iterate over the trixels that are the result of any of these 4 calculations.
The HTMesh is created with one or more output buffers used for storing the results. You can specify which output buffer is to be used to hold the results by supplying an optional integer bufNum parameter.
finds the trixels that cover the specified circle
- Parameters
-
ra Central ra in degrees dec Central dec in degrees radius Radius of the circle in degrees bufNum the output buffer to hold the results
- Note
- You will need to supply unprecessed (ra, dec) in most situations. Please see SkyMesh::aperture()'s code before messing with this method.
Definition at line 104 of file HTMesh.cpp.
◆ intersect() [2/4]
void HTMesh::intersect | ( | double | ra1, |
double | dec1, | ||
double | ra2, | ||
double | dec2, | ||
BufNum | bufNum = 0 ) |
finds the trixels that cover the specified line segment
Definition at line 181 of file HTMesh.cpp.
◆ intersect() [3/4]
void HTMesh::intersect | ( | double | ra1, |
double | dec1, | ||
double | ra2, | ||
double | dec2, | ||
double | ra3, | ||
double | dec3, | ||
BufNum | bufNum = 0 ) |
find the trixels that cover the specified triangle
Definition at line 116 of file HTMesh.cpp.
◆ intersect() [4/4]
void HTMesh::intersect | ( | double | ra1, |
double | dec1, | ||
double | ra2, | ||
double | dec2, | ||
double | ra3, | ||
double | dec3, | ||
double | ra4, | ||
double | dec4, | ||
BufNum | bufNum = 0 ) |
finds the trixels that cover the specified quadrilateral
Definition at line 137 of file HTMesh.cpp.
◆ intersectSize()
int HTMesh::intersectSize | ( | BufNum | bufNum = 0 | ) |
returns the number of trixels in the result buffer bufNum.
Definition at line 261 of file HTMesh.cpp.
◆ level()
◆ meshBuffer()
MeshBuffer * HTMesh::meshBuffer | ( | BufNum | bufNum = 0 | ) |
returns a pointer to the MeshBuffer specified by bufNum.
Currently this is only used in the MeshIterator constructor.
Definition at line 254 of file HTMesh.cpp.
◆ setDebug()
|
inline |
◆ size()
|
inline |
◆ vertices()
void HTMesh::vertices | ( | Trixel | id, |
double * | ra1, | ||
double * | dec1, | ||
double * | ra2, | ||
double * | dec2, | ||
double * | ra3, | ||
double * | dec3 ) |
Definition at line 268 of file HTMesh.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:47:16 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.