Controls
#include <controls.h>
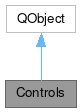
Public Types | |
enum | Level { Undefined , Primary , Secondary } |
Properties | |
QString | badgeText |
QString | color |
QString | iconName |
Level | level |
bool | showCSD |
QML_ELEMENTQString | title |
QString | toolTipText |
![]() | |
objectName | |
Signals | |
void | badgeTextChanged () |
void | colorChanged () |
void | iconNameChanged () |
void | levelChanged () |
void | showCSDChanged () |
void | titleChanged () |
void | toolTipTextChanged () |
Public Member Functions | |
QString | badgeText () const |
QString | color () const |
QString | iconName () const |
Controls::Level | level () const |
Q_ENUM (Level) explicit Controls(QObject *parent | |
void | setBadgeText (const QString &newBadgeText) |
void | setColor (const QString &newColor) |
void | setIconName (const QString &newIconName) |
void | setLevel (Level level) |
void | setShowCSD (bool newShowCSD) |
void | setTitle (const QString &title) |
void | setToolTipText (const QString &newToolTipText) |
bool | showCSD () const |
QString | title () const |
QString | toolTipText () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static Controls * | qmlAttachedProperties (QObject *object) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The Controls class.
This object exposes a series of attached properties useful for the MauiKit controls as a sort of common set metadata.
- Note
- This is mean to be used as an attached property. It can be consumed as
Maui.Controls
, for example,Maui.Controls.showCSD
Definition at line 13 of file controls.h.
Member Enumeration Documentation
◆ Level
enum Controls::Level |
A property hint for UI elements to be styled as a flat surface, for example, without a background.
By default this is set to false.
Definition at line 66 of file controls.h.
Property Documentation
◆ badgeText
|
readwrite |
The text to be used as a badge for notification purposes.
If this is left empty, then not badge will be shown.
Definition at line 35 of file controls.h.
◆ color
|
readwrite |
The color to be used as an indicator in the tab button representing the view.
Definition at line 40 of file controls.h.
◆ iconName
|
readwrite |
The icon name to be used in the AppViews button port.
Definition at line 28 of file controls.h.
◆ level
|
readwrite |
Set a UI element hierarchy level.
For example, in a page with two toolbars one could be Level::Primary, and the other one Level::Secunday, this will allow to better style the toolbars for differentiation. By default Level::Primary
is set.
Definition at line 56 of file controls.h.
◆ showCSD
|
readwrite |
Whether a supported MauiKit controls should display the window control buttons when using CSD.
Definition at line 50 of file controls.h.
◆ title
|
readwrite |
A title text that can be attached to any control.
Definition at line 23 of file controls.h.
◆ toolTipText
|
readwrite |
The text to be shown in the tool-tip when hovering over the tab button representing the view.
Definition at line 45 of file controls.h.
Member Function Documentation
◆ badgeText()
QString Controls::badgeText | ( | ) | const |
Definition at line 55 of file controls.cpp.
◆ color()
QString Controls::color | ( | ) | const |
Definition at line 81 of file controls.cpp.
◆ iconName()
QString Controls::iconName | ( | ) | const |
Definition at line 42 of file controls.cpp.
◆ level()
Controls::Level Controls::level | ( | ) | const |
Definition at line 95 of file controls.cpp.
◆ qmlAttachedProperties()
Definition at line 9 of file controls.cpp.
◆ setBadgeText()
void Controls::setBadgeText | ( | const QString & | newBadgeText | ) |
Definition at line 60 of file controls.cpp.
◆ setColor()
void Controls::setColor | ( | const QString & | newColor | ) |
Definition at line 86 of file controls.cpp.
◆ setIconName()
void Controls::setIconName | ( | const QString & | newIconName | ) |
Definition at line 47 of file controls.cpp.
◆ setLevel()
void Controls::setLevel | ( | Controls::Level | level | ) |
Definition at line 100 of file controls.cpp.
◆ setShowCSD()
void Controls::setShowCSD | ( | bool | newShowCSD | ) |
Definition at line 21 of file controls.cpp.
◆ setTitle()
void Controls::setTitle | ( | const QString & | title | ) |
Definition at line 34 of file controls.cpp.
◆ setToolTipText()
void Controls::setToolTipText | ( | const QString & | newToolTipText | ) |
Definition at line 73 of file controls.cpp.
◆ showCSD()
bool Controls::showCSD | ( | ) | const |
Definition at line 16 of file controls.cpp.
◆ title()
QString Controls::title | ( | ) | const |
Definition at line 29 of file controls.cpp.
◆ toolTipText()
QString Controls::toolTipText | ( | ) | const |
Definition at line 68 of file controls.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:50:53 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.