CatalogsComponent
#include <catalogscomponent.h>
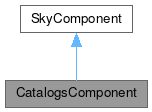
Public Types | |
using | ObjectList = std::vector<CatalogObject> |
Public Member Functions | |
CatalogsComponent (SkyComposite *parent, const QString &db_filename, bool load_default=false) | |
void | draw (SkyPainter *skyp) override |
void | dropCache () |
SkyObject * | findByName (const QString &name, bool exact=true) override |
CatalogObject & | insertStaticObject (const CatalogObject &obj) |
SkyObject * | objectNearest (SkyPoint *p, double &maxrad) override |
void | objectsInArea (QList< SkyObject * > &list, const SkyRegion ®ion) override |
void | resizeCache (const int percentage) |
bool | selected () override |
![]() | |
SkyComponent (SkyComposite *parent=nullptr) | |
virtual void | drawTrails (SkyPainter *skyp) |
virtual void | emitProgressText (const QString &message) |
QHash< int, QVector< QPair< QString, const SkyObject * > > > & | objectLists () |
QVector< QPair< QString, const SkyObject * > > & | objectLists (int type) |
QHash< int, QStringList > & | objectNames () |
QStringList & | objectNames (int type) |
SkyComposite * | parent () |
void | removeFromLists (const SkyObject *obj) |
void | removeFromNames (const SkyObject *obj) |
virtual void | update (KSNumbers *) |
virtual void | updateMoons (KSNumbers *) |
virtual void | updateSolarSystemBodies (KSNumbers *) |
Detailed Description
Represents objects loaded from an sqlite backed, trixel indexed catalog.
The component doesn't follow the traditional list approach and loads it's skyobjects into an LRU cache (TrixelCache
). For puproses of compatiblility with object search etc. some of the brightest objects are loaded into m_static_objects
and registered within the component system. Furthermore, if some part of the code demands a pointer to a CatalogObject, it will be allocated into m_static_objects
on demand.
If you want to access DSOs in new code you should use a local instance of CatalogsDB::DBManager
instead and call dropCache
if necessary.
- See also
- CatalogsDB::DBManager
Definition at line 41 of file catalogscomponent.h.
Member Typedef Documentation
◆ ObjectList
using CatalogsComponent::ObjectList = std::vector<CatalogObject> |
Definition at line 44 of file catalogscomponent.h.
Constructor & Destructor Documentation
◆ CatalogsComponent()
|
explicit |
Constructs the Catalogscomponent with a parent
and a database file under the path db_filename
.
If load_ngc
is specified, an attempt is made to load the default catalog from the default location into the db.
The lru cache for the objects will be initialized to a capacity configurable by Options::dSOCachePercentage.
Definition at line 29 of file catalogscomponent.cpp.
Member Function Documentation
◆ draw()
|
overridevirtual |
Draws the objects in the currently visible trixels by dynamically loading them from the database.
Implements SkyComponent.
Definition at line 69 of file catalogscomponent.cpp.
◆ dropCache()
|
inline |
Clear the internal cache and effectively reload all objects from the database.
Definition at line 111 of file catalogscomponent.h.
◆ findByName()
|
overridevirtual |
Search the underlying database for an object with the name
.
- See also
CatalogsDB::DBManager::find_object_by_name
for details.
If multiple objects match, the one with the hightest magnitude is returned.
- Returns
- a pointer to the SkyObject whose name matches the argument, or a nullptr pointer if no match was found. (Due to way KStars works)
Reimplemented from SkyComponent.
Definition at line 334 of file catalogscomponent.cpp.
◆ insertStaticObject()
CatalogObject & CatalogsComponent::insertStaticObject | ( | const CatalogObject & | obj | ) |
Insert an object obj
into m_static_objects
and return a reference to the newly inserted object.
If the object is already present in the list, return a reference to that. Furthermore the object will be updated (CatalogObject::JITupdate
) and inserted into the parent's objectLists
.
Definition at line 300 of file catalogscomponent.cpp.
◆ objectNearest()
Find the SkyObject nearest the given SkyPoint.
Look for a SkyObject that is nearer to point p than maxrad. If one is found, then maxrad is reset to the separation of the new nearest object. p
pointer to the SkyPoint to search around maxrad
reference to current search radius in degrees
- Returns
- a pointer to the nearest SkyObject
- Note
- This function simply returns a nullptr pointer; it is reimplemented in various sub-classes.
Reimplemented from SkyComponent.
Definition at line 375 of file catalogscomponent.cpp.
◆ objectsInArea()
|
overridevirtual |
Searches the region(s) and appends the SkyObjects found to the list of sky objects.
Look for a SkyObject that is in one of the regions If found, then append to the list of sky objects list
list of SkyObject to which matching list has to be appended to region
defines the regions in which the search for SkyObject should be done within
- Returns
- void
- Note
- This function simply returns; it is reimplemented in various sub-classes.
Reimplemented from SkyComponent.
Definition at line 346 of file catalogscomponent.cpp.
◆ resizeCache()
|
inline |
Set the cache size to the new percentage
.
The cache stores the objects of a certain percentage
of all trixels. Setting percentage = 100
short circuits the cache and loads all the objects into memory. This is reasonable for catalog sizes up to 10_000
objects.
Definition at line 74 of file catalogscomponent.h.
◆ selected()
|
inlineoverridevirtual |
Wether to show the DSOs.
Reimplemented from SkyComponent.
Definition at line 121 of file catalogscomponent.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Dec 20 2024 11:53:01 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.