TrixelCache
#include <trixelcache.h>
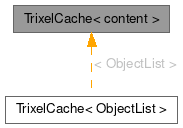
Classes | |
class | element |
Public Member Functions | |
TrixelCache (const size_t data_size, const size_t cache_size) | |
void | clear () noexcept |
size_t | current_usage () |
bool | noop () const |
element & | operator[] (const size_t index) noexcept |
std::list< size_t > | primed_indices () |
void | prune (size_t keep=0) noexcept |
void | set_size (const size_t size) |
size_t | size () const |
Detailed Description
class TrixelCache< content >
A simple integer indexed elastically cached wrapper around std::vector to hold container types content
which are cheap to construct empty and provide a default constructor, as well as []
, size
and swap
members (think std::vector
and std::list
).
The caching mechanism will be short-circuited if the cache size equals the data size.
The container is resized to a given data_size by default initializing its elements. The elements are stored as TrixelCache::element
objects and retrieved by index and LRU cached on the fly. To test if a given element is cached (or not empty) the TrixelCache::element::is_set()
can be queried. Setting an element works by just assigning it with the new data. The data from an element can be retrieved through the TrixelCache::element::data()
member. Modifying the data through that reference is allowed.
Setting an element is done by assigning it the new data.
When it is convenient TrixelCache::prune()
may be called, which clears the least recently used elements (by default initializing them) until the number of elements does not exceed the cache size. This is expensive relative to setting an elment which has almost no cost.
- Template Parameters
-
content The content type to use. Most likely a QList, std::vector or std::list.
- Todo
- Use
std::optional
when C++17 is adopted in kstars.
Example
Definition at line 59 of file trixelcache.h.
Constructor & Destructor Documentation
◆ TrixelCache()
|
inline |
Constructs a cache with data_size
default constructed elements with an elastic ceiling capacity of cache_size
.
Definition at line 108 of file trixelcache.h.
Member Function Documentation
◆ clear()
|
inlinenoexcept |
Clear the cache without changing it's size.
Definition at line 187 of file trixelcache.h.
◆ current_usage()
|
inline |
- Returns
- the number of set elements in the cache, slow
Definition at line 170 of file trixelcache.h.
◆ noop()
|
inline |
- Returns
- wether the cache is just a wrapped vector
Definition at line 184 of file trixelcache.h.
◆ operator[]()
|
inlinenoexcept |
Retrieve an element at index
.
Definition at line 118 of file trixelcache.h.
◆ primed_indices()
|
inline |
- Returns
- a list of currently primed indices, slow
Definition at line 177 of file trixelcache.h.
◆ prune()
|
inlinenoexcept |
Remove excess elements from the cache The capacity can be temporarily readjusted to keep
.
keep
must be greater than the cache size to be of effect.
Definition at line 131 of file trixelcache.h.
◆ set_size()
|
inline |
Reset the cache size to size
.
This does clear the cache.
Definition at line 155 of file trixelcache.h.
◆ size()
|
inline |
- Returns
- the size of the cache
Definition at line 167 of file trixelcache.h.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Apr 27 2024 22:13:29 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.