CatalogObject
#include <catalogobject.h>
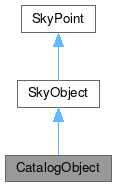
Public Types | |
using | oid = QByteArray |
![]() | |
enum | TYPE { STAR = 0 , CATALOG_STAR = 1 , PLANET = 2 , OPEN_CLUSTER = 3 , GLOBULAR_CLUSTER = 4 , GASEOUS_NEBULA = 5 , PLANETARY_NEBULA = 6 , SUPERNOVA_REMNANT = 7 , GALAXY = 8 , COMET = 9 , ASTEROID = 10 , CONSTELLATION = 11 , MOON = 12 , ASTERISM = 13 , GALAXY_CLUSTER = 14 , DARK_NEBULA = 15 , QUASAR = 16 , MULT_STAR = 17 , RADIO_SOURCE = 18 , SATELLITE = 19 , SUPERNOVA = 20 , NUMBER_OF_KNOWN_TYPES = 21 , TYPE_UNKNOWN = 255 } |
typedef qint64 | UID |
Public Member Functions | |
CatalogObject (oid id={}, const SkyObject::TYPE t=SkyObject::STAR, const dms &r=dms(0.0), const dms &d=dms(0.0), const float m=NaN::f, const QString &n=QString(), const QString &lname=QString(), const QString &catalog_identifier=QString(), const int catalog_id=-1, const float a=0.0, const float b=0.0, const double pa=0.0, const float flux=0, const QString &database_path="") | |
float | a () const |
float | b () const |
int | catalogId () const |
const QString & | catalogIdentifier () const |
CatalogObject * | clone () const override |
float | e () const |
float | flux () const |
const CatalogsDB::Catalog | getCatalog () const |
const oid | getId () const |
const oid | getObjectId () const |
SkyObject::UID | getUID () const override |
std::pair< bool, const QImage & > | image () const |
void | initPopupMenu (KSPopupMenu *pmenu) override |
void | JITupdate () |
double | labelOffset () const override |
QString | labelString () const override |
void | load_image () |
double | pa () const override |
void | setCatalogIdentifier (const QString &cat_ident) |
void | setFlux (const float flux) |
void | setMag (const double mag) |
void | setMaj (const float a) |
void | setMin (const float b) |
void | setPA (const double pa) |
![]() | |
SkyObject (int t, double r, double d, float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
SkyObject (int t=TYPE_UNKNOWN, dms r=dms(0.0), dms d=dms(0.0), float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
virtual | ~SkyObject () override=default |
bool | hashBeenUpdated () |
bool | hasLongName () const |
bool | hasName () const |
bool | hasName2 () const |
bool | isSolarSystem () const |
virtual QString | longname (void) const |
float | mag () const |
QString | messageFromTitle (const QString &imageTitle) const |
virtual QString | name (void) const |
QString | name2 (void) const |
SkyPoint | recomputeCoords (const KStarsDateTime &dt, const GeoLocation *geo=nullptr) const |
SkyPoint | recomputeHorizontalCoords (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | riseSetTime (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) const |
dms | riseSetTimeAz (const KStarsDateTime &dt, const GeoLocation *geo, bool rst) const |
QTime | riseSetTimeUT (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) const |
void | setLongName (const QString &longname=QString()) |
void | setType (int t) |
void | showPopupMenu (KSPopupMenu *pmenu, const QPoint &pos) |
dms | transitAltitude (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | transitTime (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | transitTimeUT (const KStarsDateTime &dt, const GeoLocation *geo) const |
QString | translatedLongName () const |
QString | translatedName () const |
QString | translatedName2 () const |
int | type (void) const |
QString | typeName () const |
![]() | |
SkyPoint () | |
SkyPoint (const CachingDms &r, const CachingDms &d) | |
SkyPoint (const dms &r, const dms &d) | |
SkyPoint (double r, double d) | |
void | aberrate (const KSNumbers *num, bool reverse=false) |
void | addEterms (void) |
double | airmass () const |
const dms & | alt () const |
dms | altRefracted () const |
dms | angularDistanceTo (const SkyPoint *sp, double *const positionAngle=nullptr) const |
void | apparentCoord (long double jd0, long double jdf) |
const dms & | az () const |
void | B1950ToJ2000 (void) |
bool | bendlight () |
SkyPoint | catalogueCoord (long double jdf) |
bool | checkBendLight () |
bool | checkCircumpolar (const dms *gLat) const |
const CachingDms & | dec () const |
const CachingDms & | dec0 () const |
SkyPoint | deprecess (const KSNumbers *num, long double epoch=J2000) |
void | Equatorial1950ToGalactic (dms &galLong, dms &galLat) |
void | EquatorialToHorizontal (const CachingDms *LST, const CachingDms *lat) |
void | EquatorialToHorizontal (const dms *LST, const dms *lat) |
SkyPoint | Eterms (void) |
void | findEcliptic (const CachingDms *Obliquity, dms &EcLong, dms &EcLat) |
void | GalacticToEquatorial1950 (const dms *galLong, const dms *galLat) |
long double | getLastPrecessJD () const |
void | HorizontalToEquatorial (const dms *LST, const dms *lat) |
bool | isValid () const |
void | J2000ToB1950 (void) |
double | maxAlt (const dms &lat) const |
double | minAlt (const dms &lat) const |
SkyPoint | moveAway (const SkyPoint &from, double dist) const |
void | nutate (const KSNumbers *num, const bool reverse=false) |
bool | operator== (SkyPoint &p) const |
dms | parallacticAngle (const CachingDms &LST, const CachingDms &lat) |
void | precessFromAnyEpoch (long double jd0, long double jdf) |
const CachingDms & | ra () const |
const CachingDms & | ra0 () const |
void | set (const dms &r, const dms &d) |
void | setAlt (dms alt) |
void | setAlt (double alt) |
void | setAltRefracted (dms alt_apparent) |
void | setAltRefracted (double alt_apparent) |
void | setAz (dms az) |
void | setAz (double az) |
void | setDec (const CachingDms &d) |
void | setDec (dms d) |
void | setDec (double d) |
void | setDec0 (const CachingDms &d) |
void | setDec0 (dms d) |
void | setDec0 (double d) |
void | setFromEcliptic (const CachingDms *Obliquity, const dms &EcLong, const dms &EcLat) |
void | setRA (const CachingDms &r) |
void | setRA (dms &r) |
void | setRA (double r) |
void | setRA0 (CachingDms r) |
void | setRA0 (dms r) |
void | setRA0 (double r) |
void | subtractEterms (void) |
virtual void | updateCoords (const KSNumbers *num, bool includePlanets=true, const CachingDms *lat=nullptr, const CachingDms *LST=nullptr, bool forceRecompute=false) |
virtual void | updateCoordsNow (const KSNumbers *num) |
double | vGeocentric (double vhelio, long double jd) |
double | vGeoToVHelio (double vgeo, long double jd) |
double | vHeliocentric (double vlsr, long double jd) |
double | vHelioToVlsr (double vhelio, long double jd) |
double | vREarth (long double jd0) |
double | vRSite (double vsite[3]) |
double | vRSun (long double jd) |
double | vTopocentric (double vgeo, double vsite[3]) |
double | vTopoToVGeo (double vtopo, double vsite[3]) |
Static Public Member Functions | |
static const oid | getId (const SkyObject::TYPE type, const double ra, const double dec, const QString &name, const QString &catalog_identifier) |
![]() | |
static QString | typeName (const int t) |
static QString | typeShortName (const int t) |
![]() | |
static dms | findAltitude (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | refract (const dms alt, bool conditional=true) |
static double | refract (const double alt, bool conditional=true) |
static double | refractionCorr (double alt) |
static SkyPoint | timeTransformed (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | unrefract (const dms alt, bool conditional=true) |
static double | unrefract (const double alt, bool conditional=true) |
Additional Inherited Members | |
![]() | |
static const UID | invalidUID = ~0 |
static const UID | UID_DEEPSKY = 2 |
static const UID | UID_GALAXY = 1 |
static const UID | UID_SOLARSYS = 3 |
static const UID | UID_STAR = 0 |
![]() | |
static const double | altCrit = -1.0 |
static bool | implementationIsLibnova = false |
![]() | |
void | setMag (float m) |
void | setName (const QString &name) |
void | setName2 (const QString &name2=QString()) |
![]() | |
void | precess (const KSNumbers *num) |
![]() | |
bool | has_been_updated = true |
QString | LongName |
QString | Name |
QString | Name2 |
![]() | |
long double | lastPrecessJD { 0 } |
Detailed Description
A simple container object to hold the minimum information for a Deep Sky Object to be drawn on the skymap.
Detailed information about the object (like Catalog
information) will be loaded from it's birthing database when needed. Based on the original DeepSkyObject by Jason Harris.
You shouldn't create a CatalogObject manually!
- Todo
- maybe just turn the const members public
Definition at line 40 of file catalogobject.h.
Member Typedef Documentation
◆ oid
using CatalogObject::oid = QByteArray |
Definition at line 45 of file catalogobject.h.
Constructor & Destructor Documentation
◆ CatalogObject()
|
inline |
- Parameters
-
id oid (hash) of the object t Type of object r Right Ascension d Declination m magnitude (brightness) n Primary name lname Long name (common name) catalog_identifier a catalog specific identifier catalog_id catalog id a major axis (arcminutes) b minor axis (arcminutes) pa position angle (degrees) flux integrated flux (optional) database_path the path to the birthing database of this object, used to load additional information on-demand
Definition at line 64 of file catalogobject.h.
Member Function Documentation
◆ a()
|
inline |
- Returns
- the object's major axis length, in arcminutes.
Definition at line 112 of file catalogobject.h.
◆ b()
|
inline |
- Returns
- the object's minor axis length, in arcminutes.
Definition at line 117 of file catalogobject.h.
◆ catalogId()
|
inline |
Get the id of the catalog this object belongs to.
Definition at line 141 of file catalogobject.h.
◆ catalogIdentifier()
|
inline |
- Returns
- the catalog specific identifier. (For example UGC ...)
Definition at line 163 of file catalogobject.h.
◆ clone()
|
overridevirtual |
Clones the object and returns a pointer to it.
This is legacy code.
- Deprecated
- do not use in new code
Reimplemented from SkyObject.
Definition at line 19 of file catalogobject.cpp.
◆ e()
float CatalogObject::e | ( | ) | const |
- Returns
- the object's aspect ratio (MinorAxis/MajorAxis). Returns 1.0 if the object's MinorAxis=0.0.
Definition at line 26 of file catalogobject.cpp.
◆ flux()
|
inline |
- Returns
- the object's integrated flux, unit value is stored in the custom catalog component.
Definition at line 107 of file catalogobject.h.
◆ getCatalog()
const CatalogsDB::Catalog CatalogObject::getCatalog | ( | ) | const |
Get information about the catalog that this objects stems from.
The catalog information will be loaded from the catalog database on demand.
Definition at line 104 of file catalogobject.cpp.
◆ getId() [1/2]
const CatalogObject::oid CatalogObject::getId | ( | ) | const |
- Returns
- the unique ID of this object (not the physical ID = m_object_id) by hashing unique properties of the object.
This method provides the reference implementation for the oid hash.
Definition at line 115 of file catalogobject.cpp.
◆ getId() [2/2]
|
static |
Definition at line 122 of file catalogobject.cpp.
◆ getObjectId()
|
inline |
- Returns
- the physical object id of the catalogobject
Definition at line 188 of file catalogobject.h.
◆ getUID()
|
overridevirtual |
Generates a KStars internal UID from the object id.
Reimplemented from SkyObject.
Definition at line 72 of file catalogobject.cpp.
◆ image()
|
inline |
Get the image for this object.
- Returns
- [has image?, the image]
Definition at line 233 of file catalogobject.h.
◆ initPopupMenu()
|
overridevirtual |
Initialize the popup menu for a CatalogObject
.
Reimplemented from SkyObject.
Definition at line 95 of file catalogobject.cpp.
◆ JITupdate()
void CatalogObject::JITupdate | ( | ) |
Update the cooridnates and the horizontal coordinates if updateID or updateNumID have changed (global).
Definition at line 77 of file catalogobject.cpp.
◆ labelOffset()
|
overridevirtual |
- Returns
- the pixel distance for offseting the object's name label
Reimplemented from SkyObject.
Definition at line 33 of file catalogobject.cpp.
◆ labelString()
|
overridevirtual |
- Returns
- the string for the skymap label of the object.
Reimplemented from SkyObject.
Definition at line 51 of file catalogobject.cpp.
◆ load_image()
void CatalogObject::load_image | ( | ) |
Load the image for this object.
Definition at line 139 of file catalogobject.cpp.
◆ pa()
|
inlineoverridevirtual |
- Returns
- the object's position angle in degrees, measured clockwise from North.
Reimplemented from SkyObject.
Definition at line 128 of file catalogobject.h.
◆ setCatalogIdentifier()
|
inline |
Set the catalog identifier to `cat_ident`
.
Definition at line 193 of file catalogobject.h.
◆ setFlux()
|
inline |
Set the flux to `flux`
.
Definition at line 211 of file catalogobject.h.
◆ setMag()
|
inline |
Set the magnitude of the object.
Definition at line 221 of file catalogobject.h.
◆ setMaj()
|
inline |
Set the major axis to `a`
.
Definition at line 201 of file catalogobject.h.
◆ setMin()
|
inline |
Set the minor axis to `b`
.
Definition at line 206 of file catalogobject.h.
◆ setPA()
|
inline |
Set the position angle to `pa`
.
Definition at line 216 of file catalogobject.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:16:42 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.