KSMoon
#include <ksmoon.h>
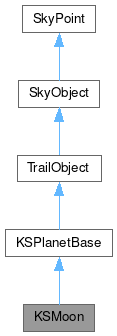
Public Member Functions | |
KSMoon () | |
KSMoon (const KSMoon &o) | |
KSMoon * | clone () const override |
bool | findGeocentricPosition (const KSNumbers *num, const KSPlanetBase *) override |
virtual void | findPhase () |
void | findPhase (const KSSun *Sun=nullptr) |
short int | getIPhase () const |
SkyObject::UID | getUID () const override |
double | illum () const |
void | initPopupMenu (KSPopupMenu *pmenu) override |
bool | loadData () override |
QString | phaseName () const |
void | updateMag () |
![]() | |
KSPlanetBase (const QString &s=i18n("unnamed"), const QString &image_file=QString(), const QColor &c=Qt::white, double pSize=0) | |
~KSPlanetBase () override=default | |
double | angSize () const |
QColor & | color () |
const dms & | ecLat () const |
void | EclipticToEquatorial (const CachingDms *Obliquity) |
const dms & | ecLong () const |
void | EquatorialToEcliptic (const CachingDms *Obliquity) |
void | findPosition (const KSNumbers *num, const CachingDms *lat=nullptr, const CachingDms *LST=nullptr, const KSPlanetBase *Earth=nullptr) |
const dms & | helEcLat () const |
const dms & | helEcLong () const |
const QImage & | image () const |
void | init (const QString &s, const QString &image_file, const QColor &c, double pSize) |
bool | isMajorPlanet () const |
double | labelOffset () const override |
double | pa () const override |
dms | phase () |
double | physicalSize () const |
double | rearth () const |
double | rsun () const |
void | setAngularSize (double size) |
void | setColor (const QColor &c) |
void | setEcLat (dms elat) |
void | setEcLong (dms elong) |
void | setPA (double p) |
void | setPhysicalSize (double size) |
void | setRearth (const KSPlanetBase *Earth) |
void | setRearth (double r) |
void | setRsun (double r) |
void | updateCoords (const KSNumbers *num, bool includePlanets=true, const CachingDms *lat=nullptr, const CachingDms *LST=nullptr, bool forceRecompute=false) override |
![]() | |
TrailObject (int t, double r, double d, float m=0.0, const QString &n=QString()) | |
TrailObject (int t=TYPE_UNKNOWN, dms r=dms(0.0), dms d=dms(0.0), float m=0.0, const QString &n=QString()) | |
void | addToTrail (const QString &label=QString()) |
void | clearTrail () |
void | clipTrail () |
TrailObject * | clone () const override |
void | drawTrail (SkyPainter *skyp) const |
bool | hasTrail () const |
void | initPopupMenu (KSPopupMenu *pmenu) override |
const QList< SkyPoint > & | trail () const |
void | updateTrail (dms *LST, const dms *lat) |
![]() | |
SkyObject (int t, double r, double d, float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
SkyObject (int t=TYPE_UNKNOWN, dms r=dms(0.0), dms d=dms(0.0), float m=0.0, const QString &n=QString(), const QString &n2=QString(), const QString &lname=QString()) | |
virtual | ~SkyObject () override=default |
bool | hashBeenUpdated () |
bool | hasLongName () const |
bool | hasName () const |
bool | hasName2 () const |
bool | isSolarSystem () const |
virtual QString | labelString () const |
virtual QString | longname (void) const |
float | mag () const |
QString | messageFromTitle (const QString &imageTitle) const |
virtual QString | name (void) const |
QString | name2 (void) const |
SkyPoint | recomputeCoords (const KStarsDateTime &dt, const GeoLocation *geo=nullptr) const |
SkyPoint | recomputeHorizontalCoords (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | riseSetTime (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) const |
dms | riseSetTimeAz (const KStarsDateTime &dt, const GeoLocation *geo, bool rst) const |
QTime | riseSetTimeUT (const KStarsDateTime &dt, const GeoLocation *geo, bool rst, bool exact=true) const |
void | setLongName (const QString &longname=QString()) |
void | setName (const QString &name) |
void | setName2 (const QString &name2=QString()) |
void | setType (int t) |
void | showPopupMenu (KSPopupMenu *pmenu, const QPoint &pos) |
dms | transitAltitude (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | transitTime (const KStarsDateTime &dt, const GeoLocation *geo) const |
QTime | transitTimeUT (const KStarsDateTime &dt, const GeoLocation *geo) const |
QString | translatedLongName () const |
QString | translatedName () const |
QString | translatedName2 () const |
int | type (void) const |
QString | typeName () const |
![]() | |
SkyPoint () | |
SkyPoint (const CachingDms &r, const CachingDms &d) | |
SkyPoint (const dms &r, const dms &d) | |
SkyPoint (double r, double d) | |
void | aberrate (const KSNumbers *num, bool reverse=false) |
void | addEterms (void) |
double | airmass () const |
const dms & | alt () const |
dms | altRefracted () const |
dms | angularDistanceTo (const SkyPoint *sp, double *const positionAngle=nullptr) const |
void | apparentCoord (long double jd0, long double jdf) |
const dms & | az () const |
void | B1950ToJ2000 (void) |
bool | bendlight () |
SkyPoint | catalogueCoord (long double jdf) |
bool | checkBendLight () |
bool | checkCircumpolar (const dms *gLat) const |
const CachingDms & | dec () const |
const CachingDms & | dec0 () const |
SkyPoint | deprecess (const KSNumbers *num, long double epoch=J2000) |
void | Equatorial1950ToGalactic (dms &galLong, dms &galLat) |
void | EquatorialToHorizontal (const CachingDms *LST, const CachingDms *lat) |
void | EquatorialToHorizontal (const dms *LST, const dms *lat) |
SkyPoint | Eterms (void) |
void | findEcliptic (const CachingDms *Obliquity, dms &EcLong, dms &EcLat) |
void | GalacticToEquatorial1950 (const dms *galLong, const dms *galLat) |
long double | getLastPrecessJD () const |
void | HorizontalToEquatorial (const dms *LST, const dms *lat) |
void | HorizontalToEquatorialICRS (const dms *LST, const dms *lat, const long double jdf) |
void | HorizontalToEquatorialNow () |
bool | isValid () const |
void | J2000ToB1950 (void) |
double | maxAlt (const dms &lat) const |
double | minAlt (const dms &lat) const |
SkyPoint | moveAway (const SkyPoint &from, double dist) const |
void | nutate (const KSNumbers *num, const bool reverse=false) |
bool | operator== (SkyPoint &p) const |
dms | parallacticAngle (const CachingDms &LST, const CachingDms &lat) |
void | precessFromAnyEpoch (long double jd0, long double jdf) |
const CachingDms & | ra () const |
const CachingDms & | ra0 () const |
void | set (const dms &r, const dms &d) |
void | setAlt (dms alt) |
void | setAlt (double alt) |
void | setAltRefracted (dms alt_apparent) |
void | setAltRefracted (double alt_apparent) |
void | setAz (dms az) |
void | setAz (double az) |
void | setDec (const CachingDms &d) |
void | setDec (dms d) |
void | setDec (double d) |
void | setDec0 (const CachingDms &d) |
void | setDec0 (dms d) |
void | setDec0 (double d) |
void | setFromEcliptic (const CachingDms *Obliquity, const dms &EcLong, const dms &EcLat) |
void | setRA (const CachingDms &r) |
void | setRA (dms &r) |
void | setRA (double r) |
void | setRA0 (CachingDms r) |
void | setRA0 (dms r) |
void | setRA0 (double r) |
void | subtractEterms (void) |
virtual void | updateCoordsNow (const KSNumbers *num) |
double | vGeocentric (double vhelio, long double jd) |
double | vGeoToVHelio (double vgeo, long double jd) |
double | vHeliocentric (double vlsr, long double jd) |
double | vHelioToVlsr (double vhelio, long double jd) |
double | vREarth (long double jd0) |
double | vRSite (double vsite[3]) |
double | vRSun (long double jd) |
double | vTopocentric (double vgeo, double vsite[3]) |
double | vTopoToVGeo (double vtopo, double vsite[3]) |
Additional Inherited Members | |
![]() | |
enum | Planets { MERCURY = 0 , VENUS = 1 , MARS = 2 , JUPITER = 3 , SATURN = 4 , URANUS = 5 , NEPTUNE = 6 , SUN = 7 , MOON = 8 , EARTH_SHADOW = 9 , UNKNOWN_PLANET } |
![]() | |
enum | TYPE { STAR = 0 , CATALOG_STAR = 1 , PLANET = 2 , OPEN_CLUSTER = 3 , GLOBULAR_CLUSTER = 4 , GASEOUS_NEBULA = 5 , PLANETARY_NEBULA = 6 , SUPERNOVA_REMNANT = 7 , GALAXY = 8 , COMET = 9 , ASTEROID = 10 , CONSTELLATION = 11 , MOON = 12 , ASTERISM = 13 , GALAXY_CLUSTER = 14 , DARK_NEBULA = 15 , QUASAR = 16 , MULT_STAR = 17 , RADIO_SOURCE = 18 , SATELLITE = 19 , SUPERNOVA = 20 , NUMBER_OF_KNOWN_TYPES = 21 , TYPE_UNKNOWN = 255 } |
typedef qint64 | UID |
![]() | |
static KSPlanetBase * | createPlanet (int n) |
![]() | |
static void | clearTrailsExcept (SkyObject *o) |
![]() | |
static QString | typeName (const int t) |
static QString | typeShortName (const int t) |
![]() | |
static dms | findAltitude (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | refract (const dms alt, bool conditional=true) |
static double | refract (const double alt, bool conditional=true) |
static double | refractionCorr (double alt) |
static SkyPoint | timeTransformed (const SkyPoint *p, const KStarsDateTime &dt, const GeoLocation *geo, const double hour=0) |
static dms | unrefract (const dms alt, bool conditional=true) |
static double | unrefract (const double alt, bool conditional=true) |
![]() | |
static QVector< QColor > | planetColor |
![]() | |
static const int | MaxTrail = 400 |
![]() | |
static const UID | invalidUID = ~0 |
static const UID | UID_DEEPSKY = 2 |
static const UID | UID_GALAXY = 1 |
static const UID | UID_SOLARSYS = 3 |
static const UID | UID_STAR = 0 |
![]() | |
static const double | altCrit = -1.0 |
static bool | implementationIsLibnova = false |
![]() | |
virtual double | findAngularSize () |
void | findPA (const KSNumbers *num) |
UID | solarsysUID (UID type) const |
![]() | |
void | setMag (float m) |
![]() | |
void | precess (const KSNumbers *num) |
![]() | |
EclipticPosition | ep |
EclipticPosition | helEcPos |
QImage | m_image |
double | Phase {NaN::d} |
double | Rearth {NaN::d} |
![]() | |
QList< QString > | m_TrailLabels |
QList< SkyPoint > | Trail |
![]() | |
bool | has_been_updated = true |
QString | LongName |
QString | Name |
QString | Name2 |
![]() | |
long double | lastPrecessJD { 0 } |
![]() | |
static const UID | UID_SOL_ASTEROID = 1 |
static const UID | UID_SOL_BIGOBJ = 0 |
static const UID | UID_SOL_COMET = 2 |
![]() | |
static QSet< TrailObject * > | trailObjects |
Detailed Description
Provides necessary information about the Moon.
A subclass of SkyObject that provides information needed for the Moon. Specifically, KSMoon provides a moon-specific findPosition() function. Also, there is a method findPhase(), which returns the lunar phase as a floating-point number between 0.0 and 1.0.
- Version
- 1.0
Constructor & Destructor Documentation
◆ KSMoon() [1/2]
KSMoon::KSMoon | ( | ) |
◆ KSMoon() [2/2]
KSMoon::KSMoon | ( | const KSMoon & | o | ) |
Copy constructor.
Definition at line 71 of file ksmoon.cpp.
◆ ~KSMoon()
|
override |
Definition at line 82 of file ksmoon.cpp.
Member Function Documentation
◆ clone()
|
overridevirtual |
Create copy of object.
This method is virtual copy constructor. It allows for safe copying of objects. In other words, KSPlanet object stored in SkyObject pointer will be copied as KSPlanet.
Each subclass of SkyObject MUST implement clone method. There is no checking to ensure this, though.
- Returns
- pointer to newly allocated object. Caller takes full responsibility for deallocating it.
Reimplemented from SkyObject.
Definition at line 76 of file ksmoon.cpp.
◆ findGeocentricPosition()
|
overridevirtual |
Reimplemented from KSPlanetBase, this function employs unique algorithms for estimating the lunar coordinates.
Finding the position of the moon is much more difficult than the other planets. For one thing, the Moon is a lot closer, so we can detect smaller deviations in its orbit. Also, the Earth has a significant effect on the Moon's orbit, and their interaction is complex and nonlinear. As a result, the positions as calculated by findPosition() are only accurate to about 10 arcseconds (10 times less precise than the planets' positions!)
moon-specific coordinate finder
- Parameters
-
num KSNumbers pointer for the target date/time
- Note
- we don't use the Earth pointer here
Implements KSPlanetBase.
Definition at line 153 of file ksmoon.cpp.
◆ findPhase() [1/2]
|
virtual |
Determine the phase of the planet.
Reimplemented from KSPlanetBase.
Definition at line 250 of file ksplanetbase.cpp.
◆ findPhase() [2/2]
void KSMoon::findPhase | ( | const KSSun * | Sun = nullptr | ) |
Determine the phase angle of the moon, and assign the appropriate moon image.
- Parameters
-
Sun a KSSun pointer with coordinates updated to the time of computation. If not supplied, the sun is retrieved via KStarsData
- Note
- Overrides KSPlanetBase::findPhase()
Definition at line 268 of file ksmoon.cpp.
◆ getIPhase()
|
inline |
◆ getUID()
|
overridevirtual |
◆ illum()
|
inline |
◆ initPopupMenu()
|
overridevirtual |
Initialize the popup menut.
This function should call correct initialization function in KSPopupMenu. By overloading the function, we don't have to check the object type when we need the menu.
Reimplemented from SkyObject.
Definition at line 320 of file ksmoon.cpp.
◆ loadData()
|
overridevirtual |
◆ phaseName()
QString KSMoon::phaseName | ( | ) | const |
- Returns
- a short string describing the moon's phase
Definition at line 288 of file ksmoon.cpp.
◆ updateMag()
|
inline |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:01:37 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.