FOV
FOV Class Reference
#include <fov.h>
Inheritance diagram for FOV:
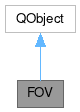
Public Types | |
enum | Shape { SQUARE , CIRCLE , CROSSHAIRS , BULLSEYE , SOLIDCIRCLE , UNKNOWN } |
![]() | |
typedef | QObjectList |
Properties | |
QString | color |
bool | cpLock |
QString | name |
float | offsetX |
float | offsetY |
float | PA |
FOV::Shape | shape |
float | sizeX |
float | sizeY |
![]() | |
objectName | |
Public Member Functions | |
FOV () | |
FOV (const FOV &other) | |
FOV (const QString &name, float a, float b=-1, float xoffset=0, float yoffset=0, float rot=0, Shape shape=SQUARE, const QString &color="#FFFFFF", bool useLockedCP=false) | |
SkyPoint | center () const |
QString | color () const |
void | draw (QPainter &p, float x, float y) |
void | draw (QPainter &p, float zoomFactor) |
bool | lockCelestialPole () const |
Q_SCRIPTABLE QString | name () const |
float | northPA () const |
float | offsetX () const |
float | offsetY () const |
float | PA () const |
void | setCenter (const SkyPoint ¢er) |
void | setColor (const QString &c) |
void | setImage (const QImage &image) |
void | setImageDisplay (bool value) |
void | setLockCelestialPole (bool lockCelestialPole) |
void | setName (const QString &n) |
void | setNorthPA (float northPA) |
void | setOffset (float fx, float fy) |
void | setPA (float rt) |
void | setShape (FOV::Shape s) |
void | setSize (float s) |
void | setSize (float sx, float sy) |
FOV::Shape | shape () const |
float | sizeX () const |
float | sizeY () const |
void | sync (const FOV &other) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
A simple class encapsulating a Field-of-View symbol.
The FOV size, shape, name, and color can be customized. The rotation is by default 0 degrees East Of North.
- Version
- 1.1
Member Enumeration Documentation
◆ Shape
Property Documentation
◆ color
◆ cpLock
◆ name
◆ offsetX
◆ offsetY
◆ PA
◆ shape
◆ sizeX
◆ sizeY
Constructor & Destructor Documentation
◆ FOV() [1/3]
◆ FOV() [2/3]
◆ FOV() [3/3]
Member Function Documentation
◆ center()
◆ color()
◆ draw() [1/2]
void FOV::draw | ( | QPainter & | p, |
float | x, | ||
float | y ) |
◆ draw() [2/2]
void FOV::draw | ( | QPainter & | p, |
float | zoomFactor ) |
◆ lockCelestialPole()
◆ name()
◆ northPA()
◆ offsetX()
◆ offsetY()
◆ PA()
◆ setCenter()
◆ setColor()
◆ setImage()
◆ setImageDisplay()
◆ setLockCelestialPole()
◆ setName()
◆ setNorthPA()
◆ setOffset()
◆ setPA()
◆ setShape()
◆ setSize() [1/2]
◆ setSize() [2/2]
◆ shape()
◆ sizeX()
◆ sizeY()
◆ sync()
The documentation for this class was generated from the following files:
This file is part of the KDE documentation.
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Dec 20 2024 11:53:01 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Dec 20 2024 11:53:01 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.