EquirectangularProjector
#include <equirectangularprojector.h>
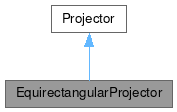
Public Member Functions | |
EquirectangularProjector (const ViewParams &p) | |
SkyPoint | fromScreen (const QPointF &p, dms *LST, const dms *lat, bool onlyAltAz=false) const override |
QVector< Eigen::Vector2f > | groundPoly (SkyPoint *labelpoint=nullptr, bool *drawLabel=nullptr) const override |
double | radius () const override |
Eigen::Vector2f | toScreenVec (const SkyPoint *o, bool oRefract=true, bool *onVisibleHemisphere=nullptr) const override |
Projection | type () const override |
bool | unusablePoint (const QPointF &p) const override |
void | updateClipPoly () override |
![]() | |
Projector (const ViewParams &p) | |
bool | checkVisibility (const SkyPoint *p) const |
QPointF | clipLine (SkyPoint *p1, SkyPoint *p2) const |
Eigen::Vector2f | clipLineVec (SkyPoint *p1, SkyPoint *p2) const |
virtual QPolygonF | clipPoly () const |
double | findNorthPA (const SkyPoint *o, float x, float y) const |
double | findPA (const SkyObject *o, float x, float y) const |
double | findZenithPA (const SkyPoint *o, float x, float y) const |
double | fov () const |
bool | onScreen (const Eigen::Vector2f &p) const |
bool | onScreen (const QPointF &p) const |
void | setViewParams (const ViewParams &p) |
QPointF | toScreen (const SkyPoint *o, bool oRefract=true, bool *onVisibleHemisphere=nullptr) const |
ViewParams | viewParams () const |
Additional Inherited Members | |
![]() | |
enum | Projection { Lambert , AzimuthalEquidistant , Orthographic , Equirectangular , Stereographic , Gnomonic , UnknownProjection } |
![]() | |
virtual double | cosMaxFieldAngle () const |
Eigen::Vector2f | derst (double x, double y) const |
virtual double | projectionK (double x) const |
virtual double | projectionL (double x) const |
Eigen::Vector2f | rst (double x, double y) const |
![]() | |
static SkyPoint | pointAt (double az) |
![]() | |
QPolygonF | m_clipPolygon |
double | m_cosY0 { 0 } |
KStarsData * | m_data { nullptr } |
double | m_fov { 0 } |
double | m_sinY0 { 0 } |
ViewParams | m_vp |
Detailed Description
Implememntation of Equirectangular projection
Definition at line 18 of file equirectangularprojector.h.
Constructor & Destructor Documentation
◆ EquirectangularProjector()
|
explicit |
Definition at line 13 of file equirectangularprojector.cpp.
Member Function Documentation
◆ fromScreen()
|
overridevirtual |
Determine RA, Dec coordinates of the pixel at (dx, dy), which are the screen pixel coordinate offsets from the center of the Sky pixmap.
- Parameters
-
p the screen pixel position to convert LST pointer to the local sidereal time, as a dms object. lat pointer to the current geographic laitude, as a dms object onlyAltAz the returned SkyPoint's RA & DEC are not computed, only Alt/Az.
N.B. We don't cache these sin/cos values in the inverse projection because it causes 'shaking' when moving the sky.
Reimplemented from Projector.
Definition at line 63 of file equirectangularprojector.cpp.
◆ groundPoly()
|
overridevirtual |
Get the ground polygon.
- Parameters
-
labelpoint This point will be set to something suitable for attaching a label drawLabel this tells whether to draw a label.
- Returns
- the ground polygon
Reimplemented from Projector.
Definition at line 105 of file equirectangularprojector.cpp.
◆ radius()
|
overridevirtual |
Get the radius of this projection's sky circle.
- Returns
- the radius in radians
Reimplemented from Projector.
Definition at line 23 of file equirectangularprojector.cpp.
◆ toScreenVec()
|
overridevirtual |
Given the coordinates of the SkyPoint argument, determine the pixel coordinates in the SkyMap.
Since most of the projections used by KStars are very similar, if this function were to be reimplemented in each projection subclass we would end up changing maybe 5 or 6 lines out of 150. Instead, we have a default implementation that uses the projectionK and projectionL functions to take care of the differences between e.g. Orthographic and Stereographic. There is also the cosMaxFieldAngle function, which is used for testing whether a point is on the visible part of the projection, and the radius function which gives the radius of the projection in screen coordinates.
While this seems ugly, it is less ugly than duplicating 150 loc to change 5.
- Returns
- Eigen::Vector2f containing screen pixel x, y coordinates of SkyPoint.
- Parameters
-
o pointer to the SkyPoint for which to calculate x, y coordinates. oRefract true = use Options::useRefraction() value. false = do not use refraction. This argument is only needed for the Horizon, which should never be refracted. onVisibleHemisphere pointer to a bool to indicate whether the point is on the visible part of the Celestial Sphere.
Reimplemented from Projector.
Definition at line 28 of file equirectangularprojector.cpp.
◆ type()
|
overridevirtual |
Return the type of this projection.
Implements Projector.
Definition at line 18 of file equirectangularprojector.cpp.
◆ unusablePoint()
|
overridevirtual |
Check if the current point on screen is a valid point on the sky.
This is needed to avoid a crash of the program if the user clicks on a point outside the sky (the corners of the sky map at the lowest zoom level are the invalid points).
- Parameters
-
p the screen pixel position
Reimplemented from Projector.
Definition at line 97 of file equirectangularprojector.cpp.
◆ updateClipPoly()
|
overridevirtual |
updateClipPoly calculate the clipping polygen given the current FOV.
Reimplemented from Projector.
Definition at line 251 of file equirectangularprojector.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:47:16 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.