KSFileReader
#include <ksfilereader.h>
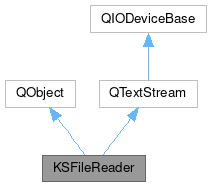
Signals | |
void | progressText (const QString &message) |
Public Member Functions | |
KSFileReader (QFile &file, qint64 maxLen=1024) | |
KSFileReader (qint64 maxLen=1024) | |
bool | hasMoreLines () const |
int | lineNumber () const |
bool | open (const QString &fname) |
bool | openFullPath (const QString &fname) |
QString | readLine () |
void | setProgress (QString label, unsigned int lastLine, unsigned int numUpdates=10) |
void | showProgress () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
QTextStream (const QByteArray &array, OpenMode openMode) | |
QTextStream (FILE *fileHandle, OpenMode openMode) | |
QTextStream (QByteArray *array, OpenMode openMode) | |
QTextStream (QIODevice *device) | |
QTextStream (QString *string, OpenMode openMode) | |
bool | atEnd () const const |
bool | autoDetectUnicode () const const |
QIODevice * | device () const const |
QStringConverter::Encoding | encoding () const const |
FieldAlignment | fieldAlignment () const const |
int | fieldWidth () const const |
void | flush () |
bool | generateByteOrderMark () const const |
int | integerBase () const const |
QLocale | locale () const const |
NumberFlags | numberFlags () const const |
QTextStream & | operator<< (char c) |
QTextStream & | operator<< (char16_t c) |
QTextStream & | operator<< (const char *string) |
QTextStream & | operator<< (const QByteArray &array) |
QTextStream & | operator<< (const QString &string) |
QTextStream & | operator<< (const void *ptr) |
QTextStream & | operator<< (double f) |
QTextStream & | operator<< (float f) |
QTextStream & | operator<< (int i) |
QTextStream & | operator<< (long i) |
QTextStream & | operator<< (QChar c) |
QTextStream & | operator<< (QLatin1StringView string) |
QTextStream & | operator<< (qlonglong i) |
QTextStream & | operator<< (QStringView string) |
QTextStream & | operator<< (qulonglong i) |
QTextStream & | operator<< (short i) |
QTextStream & | operator<< (unsigned int i) |
QTextStream & | operator<< (unsigned long i) |
QTextStream & | operator<< (unsigned short i) |
QTextStream & | operator>> (char &c) |
QTextStream & | operator>> (char *c) |
QTextStream & | operator>> (char16_t &c) |
QTextStream & | operator>> (double &f) |
QTextStream & | operator>> (float &f) |
QTextStream & | operator>> (int &i) |
QTextStream & | operator>> (long &i) |
QTextStream & | operator>> (QByteArray &array) |
QTextStream & | operator>> (QChar &c) |
QTextStream & | operator>> (qlonglong &i) |
QTextStream & | operator>> (QString &str) |
QTextStream & | operator>> (qulonglong &i) |
QTextStream & | operator>> (short &i) |
QTextStream & | operator>> (unsigned int &i) |
QTextStream & | operator>> (unsigned long &i) |
QTextStream & | operator>> (unsigned short &i) |
QChar | padChar () const const |
qint64 | pos () const const |
QTextStreamManipulator | qSetFieldWidth (int width) |
QTextStreamManipulator | qSetPadChar (QChar ch) |
QTextStreamManipulator | qSetRealNumberPrecision (int precision) |
QString | read (qint64 maxlen) |
QString | readAll () |
QString | readLine (qint64 maxlen) |
bool | readLineInto (QString *line, qint64 maxlen) |
RealNumberNotation | realNumberNotation () const const |
int | realNumberPrecision () const const |
void | reset () |
void | resetStatus () |
bool | seek (qint64 pos) |
void | setAutoDetectUnicode (bool enabled) |
void | setDevice (QIODevice *device) |
void | setEncoding (QStringConverter::Encoding encoding) |
void | setFieldAlignment (FieldAlignment mode) |
void | setFieldWidth (int width) |
void | setGenerateByteOrderMark (bool generate) |
void | setIntegerBase (int base) |
void | setLocale (const QLocale &locale) |
void | setNumberFlags (NumberFlags flags) |
void | setPadChar (QChar ch) |
void | setRealNumberNotation (RealNumberNotation notation) |
void | setRealNumberPrecision (int precision) |
void | setStatus (Status status) |
void | setString (QString *string, OpenMode openMode) |
void | skipWhiteSpace () |
Status | status () const const |
QString * | string () const const |
Additional Inherited Members | |
![]() | |
enum | FieldAlignment |
enum | NumberFlag |
enum | RealNumberNotation |
enum | Status |
![]() | |
enum | OpenModeFlag |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
AlignAccountingStyle | |
AlignCenter | |
AlignLeft | |
AlignRight | |
FixedNotation | |
ForcePoint | |
ForceSign | |
typedef | NumberFlags |
Ok | |
ReadCorruptData | |
ReadPastEnd | |
ScientificNotation | |
ShowBase | |
SmartNotation | |
UppercaseBase | |
UppercaseDigits | |
WriteFailed | |
![]() | |
Append | |
ExistingOnly | |
NewOnly | |
NotOpen | |
typedef | OpenMode |
ReadOnly | |
ReadWrite | |
Text | |
Truncate | |
Unbuffered | |
WriteOnly | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
I totally rewrote this because the earlier scheme of reading all the lines of a file into a buffer before processing is actually extremely inefficient because it makes it impossible to interleave file reading and data processing which all modern computers can do.
It also force large data files to be split up into many smaller files which made dealing with the data much more difficult and made the code to read in the data needlessly complicated. A simple subclassing of QTextStream fixed all of the above problems (IMO).
I had added periodic progress reports based on line number to several parts of the code that read in large files. So I combined that duplicated code into one place which was naturally here. The progress code causes almost nothing extra to take place when reading a file that does not use it. The only thing extra is incrementing the line number. For files that you want to emit periodic progress reports, you call setProgress() once setting up the message to display and the intervals the message will be emitted. Then inside the loop you call showProgress(). This is an extra call in the read loop but it just does an integer compare and almost always returns. We could inline it to reduce the call overhead but I don't think it makes a bit of difference considering all of the processing that takes place when we read in a line from a data file.
NOTE: We no longer close the file like the previous version did. I've changed the code where this was assumed.
There are two ways to use this class. One is pass in a QFile& in the constructor which is included only for backward compatibility. The preferred way is to just instantiate KSFileReader with no parameters and then use the open( QString fname ) method to let this class handle the file opening which helps take unneeded complexity out of the calling classes. I didn't make a constructor with the filename in it because we need to be able to inform the caller of an error opening the file, hence the bool open(filename) method.
– James B. Bowlin
Definition at line 54 of file ksfilereader.h.
Constructor & Destructor Documentation
◆ KSFileReader() [1/2]
|
explicit |
this is the preferred constructor.
You can then use the open() method to let this class open the file for you.
Definition at line 21 of file ksfilereader.cpp.
◆ KSFileReader() [2/2]
|
explicit |
Constructor.
- Parameters
-
file is a previously opened (for reading) file. maxLen sets the maximum line length before wrapping. Setting this parameter should help efficiency. The little max-length.pl script will tell you the maximum line length of files.
Definition at line 25 of file ksfilereader.cpp.
Member Function Documentation
◆ hasMoreLines()
|
inline |
- Returns
- true if we are not yet at the end of the file. (I added this to be compatible with existing code.)
Definition at line 101 of file ksfilereader.h.
◆ lineNumber()
|
inline |
returns the current line number
Definition at line 113 of file ksfilereader.h.
◆ open()
bool KSFileReader::open | ( | const QString & | fname | ) |
opens the file fname from the QStandardPaths::AppLocalDataLocation directory and uses that file for the QTextStream.
- Parameters
-
fname the name of the file to open
- Returns
- returns true on success. Prints an error message and returns false on failure.
Definition at line 40 of file ksfilereader.cpp.
◆ openFullPath()
bool KSFileReader::openFullPath | ( | const QString & | fname | ) |
opens the file with full path fname and uses that file for the QTextStream.
open() locates QStandardPaths::AppLocalDataLocation behind the scenes, so passing fname such that QString fname = KSPaths::locate(QStandardPaths::AppLocalDataLocation, "file_name" ); is equivalent
- Parameters
-
fname full path to directory + name of the file to open
- Returns
- returns true on success. Prints an error message and returns false on failure.
Definition at line 56 of file ksfilereader.cpp.
◆ readLine()
|
inline |
increments the line number and returns the next line from the file as a QString.
Definition at line 106 of file ksfilereader.h.
◆ setProgress()
void KSFileReader::setProgress | ( | QString | label, |
unsigned int | lastLine, | ||
unsigned int | numUpdates = 10 ) |
Prepares this instance to emit progress reports on how much of the file has been read (in percent).
- Parameters
-
label the label lastLine the number of lines to be read numUpdates the number of progress reports to send
Definition at line 73 of file ksfilereader.cpp.
◆ showProgress()
void KSFileReader::showProgress | ( | ) |
emits progress reports when required and updates bookkeeping for when to send the next report.
This might seem slow at first glance but almost all the time we are just doing an integer compare and returning. If you are worried about speed we can inline it. It could also safely be included in the readLine() method since m_targetLine is set to MAXUINT in the constructor.
Definition at line 85 of file ksfilereader.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.