QCPAxisTickerTime
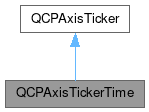
Public Types | |
enum | TimeUnit { tuMilliseconds , tuSeconds , tuMinutes , tuHours , tuDays } |
![]() | |
enum | TickStepStrategy { tssReadability , tssMeetTickCount } |
Public Member Functions | |
QCPAxisTickerTime () | |
int | fieldWidth (TimeUnit unit) const |
void | setFieldWidth (TimeUnit unit, int width) |
void | setTimeFormat (const QString &format) |
QString | timeFormat () const |
![]() | |
QCPAxisTicker () | |
virtual void | generate (const QCPRange &range, const QLocale &locale, QChar formatChar, int precision, QVector< double > &ticks, QVector< double > *subTicks, QVector< QString > *tickLabels) |
void | setTickCount (int count) |
void | setTickOrigin (double origin) |
void | setTickStepStrategy (TickStepStrategy strategy) |
int | tickCount () const |
double | tickOrigin () const |
TickStepStrategy | tickStepStrategy () const |
Protected Member Functions | |
virtual int | getSubTickCount (double tickStep) override |
virtual QString | getTickLabel (double tick, const QLocale &locale, QChar formatChar, int precision) override |
virtual double | getTickStep (const QCPRange &range) override |
void | replaceUnit (QString &text, TimeUnit unit, int value) const |
![]() | |
double | cleanMantissa (double input) const |
virtual QVector< QString > | createLabelVector (const QVector< double > &ticks, const QLocale &locale, QChar formatChar, int precision) |
virtual QVector< double > | createSubTickVector (int subTickCount, const QVector< double > &ticks) |
virtual QVector< double > | createTickVector (double tickStep, const QCPRange &range) |
double | getMantissa (double input, double *magnitude=nullptr) const |
double | pickClosest (double target, const QVector< double > &candidates) const |
void | trimTicks (const QCPRange &range, QVector< double > &ticks, bool keepOneOutlier) const |
Protected Attributes | |
TimeUnit | mBiggestUnit |
QHash< TimeUnit, int > | mFieldWidth |
QHash< TimeUnit, QString > | mFormatPattern |
TimeUnit | mSmallestUnit |
QString | mTimeFormat |
![]() | |
int | mTickCount |
double | mTickOrigin |
TickStepStrategy | mTickStepStrategy |
Detailed Description
Specialized axis ticker for time spans in units of milliseconds to days.

This QCPAxisTicker subclass generates ticks that corresponds to time intervals.
The format of the time display in the tick labels is controlled with setTimeFormat and setFieldWidth. The time coordinate is in the unit of seconds with respect to the time coordinate zero. Unlike with QCPAxisTickerDateTime, the ticks don't correspond to a specific calendar date and time.
The time can be displayed in milliseconds, seconds, minutes, hours and days. Depending on the largest available unit in the format specified with setTimeFormat, any time spans above will be carried in that largest unit. So for example if the format string is "%m:%s" and a tick at coordinate value 7815 (being 2 hours, 10 minutes and 15 seconds) is created, the resulting tick label will show "130:15" (130 minutes, 15 seconds). If the format string is "%h:%m:%s", the hour unit will be used and the label will thus be "02:10:15". Negative times with respect to the axis zero will carry a leading minus sign.
The ticker can be created and assigned to an axis like this:
Here is an example of a time axis providing time information in days, hours and minutes. Due to the axis range spanning a few days and the wanted tick count (setTickCount), the ticker decided to use tick steps of 12 hours:

The format string for this example is
- Note
- If you rather wish to display calendar dates and times, have a look at QCPAxisTickerDateTime instead.
Definition at line 1789 of file qcustomplot.h.
Member Enumeration Documentation
◆ TimeUnit
Defines the logical units in which fractions of time spans can be expressed.
- See also
- setFieldWidth, setTimeFormat
Enumerator | |
---|---|
tuMilliseconds | Milliseconds, one thousandth of a second (%z in setTimeFormat) |
tuSeconds | Seconds (%s in setTimeFormat) |
tuMinutes | Minutes (%m in setTimeFormat) |
tuHours | Hours (%h in setTimeFormat) |
tuDays | Days (%d in setTimeFormat) |
Definition at line 1798 of file qcustomplot.h.
Constructor & Destructor Documentation
◆ QCPAxisTickerTime()
QCPAxisTickerTime::QCPAxisTickerTime | ( | ) |
Constructs the ticker and sets reasonable default values. Axis tickers are commonly created managed by a QSharedPointer, which then can be passed to QCPAxis::setTicker.
Definition at line 6862 of file qcustomplot.cpp.
Member Function Documentation
◆ fieldWidth()
|
inline |
Definition at line 1810 of file qcustomplot.h.
◆ getSubTickCount()
|
overrideprotectedvirtual |
Returns the sub tick count appropriate for the provided tickStep and time displays.
\seebaseclassmethod
Reimplemented from QCPAxisTicker.
Definition at line 6995 of file qcustomplot.cpp.
◆ getTickLabel()
|
overrideprotectedvirtual |
Returns the tick label corresponding to the provided tick and the configured format and field widths (setTimeFormat, setFieldWidth).
\seebaseclassmethod
Reimplemented from QCPAxisTicker.
Definition at line 7021 of file qcustomplot.cpp.
◆ getTickStep()
|
overrideprotectedvirtual |
Returns the tick step appropriate for time displays, depending on the provided range and the smallest available time unit in the current format (setTimeFormat). For example if the unit of seconds isn't available in the format, this method will not generate steps (like 2.5 minutes) that require sub-minute precision to be displayed correctly.
\seebaseclassmethod
Reimplemented from QCPAxisTicker.
Definition at line 6944 of file qcustomplot.cpp.
◆ replaceUnit()
|
protected |
Replaces all occurrences of the format pattern belonging to unit in text with the specified value, using the field width as specified with setFieldWidth for the unit.
Definition at line 7058 of file qcustomplot.cpp.
◆ setFieldWidth()
void QCPAxisTickerTime::setFieldWidth | ( | QCPAxisTickerTime::TimeUnit | unit, |
int | width ) |
Sets the field widh of the specified unit to be width digits, when displayed in the tick label. If the number for the specific unit is shorter than width, it will be padded with an according number of zeros to the left in order to reach the field width.
- See also
- setTimeFormat
Definition at line 6930 of file qcustomplot.cpp.
◆ setTimeFormat()
void QCPAxisTickerTime::setTimeFormat | ( | const QString & | format | ) |
Sets the format that will be used to display time in the tick labels.
The available patterns are:
- %z for milliseconds
- %s for seconds
- %m for minutes
- %h for hours
- %d for days
The field width (zero padding) can be controlled for each unit with setFieldWidth.
The largest unit that appears in format will carry all the remaining time of a certain tick coordinate, even if it overflows the natural limit of the unit. For example, if %m is the largest unit it might become larger than 59 in order to consume larger time values. If on the other hand %h is available, the minutes will wrap around to zero after 59 and the time will carry to the hour digit.
Definition at line 6899 of file qcustomplot.cpp.
◆ timeFormat()
|
inline |
Definition at line 1809 of file qcustomplot.h.
Member Data Documentation
◆ mBiggestUnit
|
protected |
Definition at line 1822 of file qcustomplot.h.
◆ mFieldWidth
Definition at line 1819 of file qcustomplot.h.
◆ mFormatPattern
Definition at line 1823 of file qcustomplot.h.
◆ mSmallestUnit
|
protected |
Definition at line 1822 of file qcustomplot.h.
◆ mTimeFormat
|
protected |
Definition at line 1818 of file qcustomplot.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:59:53 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.