QCPPlottableInterface1D
#include <qcustomplot.h>
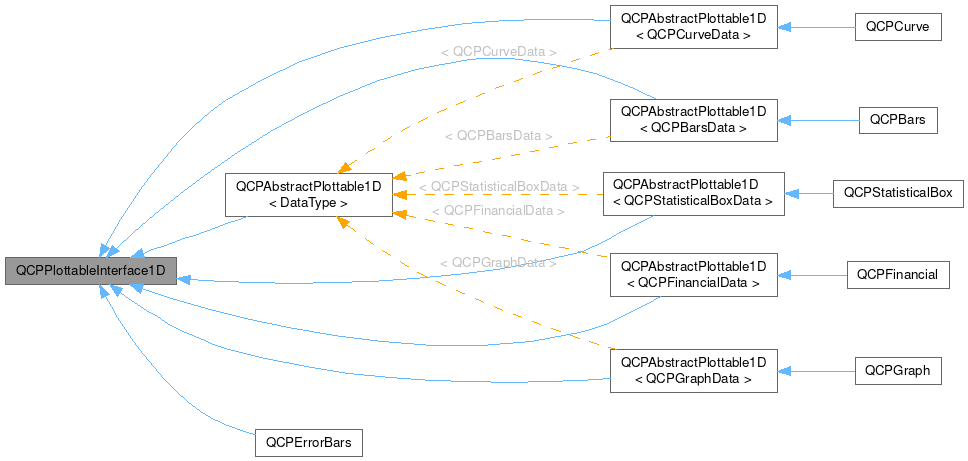
Public Member Functions | |
virtual int | dataCount () const =0 |
virtual double | dataMainKey (int index) const =0 |
virtual double | dataMainValue (int index) const =0 |
virtual QPointF | dataPixelPosition (int index) const =0 |
virtual double | dataSortKey (int index) const =0 |
virtual QCPRange | dataValueRange (int index) const =0 |
virtual int | findBegin (double sortKey, bool expandedRange=true) const =0 |
virtual int | findEnd (double sortKey, bool expandedRange=true) const =0 |
virtual QCPDataSelection | selectTestRect (const QRectF &rect, bool onlySelectable) const =0 |
virtual bool | sortKeyIsMainKey () const =0 |
Detailed Description
Defines an abstract interface for one-dimensional plottables.
This class contains only pure virtual methods which define a common interface to the data of one-dimensional plottables.
For example, it is implemented by the template class QCPAbstractPlottable1D (the preferred base class for one-dimensional plottables). So if you use that template class as base class of your one-dimensional plottable, you won't have to care about implementing the 1d interface yourself.
If your plottable doesn't derive from QCPAbstractPlottable1D but still wants to provide a 1d interface (e.g. like QCPErrorBars does), you should inherit from both QCPAbstractPlottable and QCPPlottableInterface1D and accordingly reimplement the pure virtual methods of the 1d interface, matching your data container. Also, reimplement QCPAbstractPlottable::interface1D to return the this
pointer.
If you have a QCPAbstractPlottable pointer, you can check whether it implements this interface by calling QCPAbstractPlottable::interface1D and testing it for a non-zero return value. If it indeed implements this interface, you may use it to access the plottable's data without needing to know the exact type of the plottable or its data point type.
Definition at line 4160 of file qcustomplot.h.
Member Function Documentation
◆ dataCount()
|
pure virtual |
Returns the number of data points of the plottable.
Implemented in QCPAbstractPlottable1D< DataType >, QCPAbstractPlottable1D< QCPBarsData >, QCPAbstractPlottable1D< QCPCurveData >, QCPAbstractPlottable1D< QCPFinancialData >, QCPAbstractPlottable1D< QCPGraphData >, QCPAbstractPlottable1D< QCPStatisticalBoxData >, and QCPErrorBars.
◆ dataMainKey()
|
pure virtual |
Returns the main key of the data point at the given index.
What the main key is, is defined by the plottable's data type. See the QCPDataContainer DataType documentation for details about this naming convention.
Implemented in QCPAbstractPlottable1D< DataType >, QCPAbstractPlottable1D< QCPBarsData >, QCPAbstractPlottable1D< QCPCurveData >, QCPAbstractPlottable1D< QCPFinancialData >, QCPAbstractPlottable1D< QCPGraphData >, QCPAbstractPlottable1D< QCPStatisticalBoxData >, and QCPErrorBars.
◆ dataMainValue()
|
pure virtual |
Returns the main value of the data point at the given index.
What the main value is, is defined by the plottable's data type. See the QCPDataContainer DataType documentation for details about this naming convention.
Implemented in QCPAbstractPlottable1D< DataType >, QCPAbstractPlottable1D< QCPBarsData >, QCPAbstractPlottable1D< QCPCurveData >, QCPAbstractPlottable1D< QCPFinancialData >, QCPAbstractPlottable1D< QCPGraphData >, QCPAbstractPlottable1D< QCPStatisticalBoxData >, and QCPErrorBars.
◆ dataPixelPosition()
|
pure virtual |
Returns the pixel position on the widget surface at which the data point at the given index appears.
Usually this corresponds to the point of dataMainKey/ dataMainValue, in pixel coordinates. However, depending on the plottable, this might be a different apparent position than just a coord-to-pixel transform of those values. For example, QCPBars apparent data values can be shifted depending on their stacking, bar grouping or configured base value.
Implemented in QCPAbstractPlottable1D< DataType >, QCPAbstractPlottable1D< QCPBarsData >, QCPAbstractPlottable1D< QCPCurveData >, QCPAbstractPlottable1D< QCPFinancialData >, QCPAbstractPlottable1D< QCPGraphData >, QCPAbstractPlottable1D< QCPStatisticalBoxData >, QCPBars, and QCPErrorBars.
◆ dataSortKey()
|
pure virtual |
Returns the sort key of the data point at the given index.
What the sort key is, is defined by the plottable's data type. See the QCPDataContainer DataType documentation for details about this naming convention.
Implemented in QCPAbstractPlottable1D< DataType >, QCPAbstractPlottable1D< QCPBarsData >, QCPAbstractPlottable1D< QCPCurveData >, QCPAbstractPlottable1D< QCPFinancialData >, QCPAbstractPlottable1D< QCPGraphData >, QCPAbstractPlottable1D< QCPStatisticalBoxData >, and QCPErrorBars.
◆ dataValueRange()
|
pure virtual |
Returns the value range of the data point at the given index.
What the value range is, is defined by the plottable's data type. See the QCPDataContainer DataType documentation for details about this naming convention.
Implemented in QCPAbstractPlottable1D< DataType >, QCPAbstractPlottable1D< QCPBarsData >, QCPAbstractPlottable1D< QCPCurveData >, QCPAbstractPlottable1D< QCPFinancialData >, QCPAbstractPlottable1D< QCPGraphData >, QCPAbstractPlottable1D< QCPStatisticalBoxData >, and QCPErrorBars.
◆ findBegin()
|
pure virtual |
Returns the index of the data point with a (sort-)key that is equal to, just below, or just above sortKey. If expandedRange is true, the data point just below sortKey will be considered, otherwise the one just above.
This can be used in conjunction with findEnd to iterate over data points within a given key range, including or excluding the bounding data points that are just beyond the specified range.
If expandedRange is true but there are no data points below sortKey, 0 is returned.
If the container is empty, returns 0 (in that case, findEnd will also return 0, so a loop using these methods will not iterate over the index 0).
- See also
- findEnd, QCPDataContainer::findBegin
Implemented in QCPAbstractPlottable1D< DataType >, QCPAbstractPlottable1D< QCPBarsData >, QCPAbstractPlottable1D< QCPCurveData >, QCPAbstractPlottable1D< QCPFinancialData >, QCPAbstractPlottable1D< QCPGraphData >, QCPAbstractPlottable1D< QCPStatisticalBoxData >, and QCPErrorBars.
◆ findEnd()
|
pure virtual |
Returns the index one after the data point with a (sort-)key that is equal to, just above, or just below sortKey. If expandedRange is true, the data point just above sortKey will be considered, otherwise the one just below.
This can be used in conjunction with findBegin to iterate over data points within a given key range, including the bounding data points that are just below and above the specified range.
If expandedRange is true but there are no data points above sortKey, the index just above the highest data point is returned.
If the container is empty, returns 0.
- See also
- findBegin, QCPDataContainer::findEnd
Implemented in QCPAbstractPlottable1D< DataType >, QCPAbstractPlottable1D< QCPBarsData >, QCPAbstractPlottable1D< QCPCurveData >, QCPAbstractPlottable1D< QCPFinancialData >, QCPAbstractPlottable1D< QCPGraphData >, QCPAbstractPlottable1D< QCPStatisticalBoxData >, and QCPErrorBars.
◆ selectTestRect()
|
pure virtual |
Returns a data selection containing all the data points of this plottable which are contained (or hit by) rect. This is used mainly in the selection rect interaction for data selection (data selection mechanism).
If onlySelectable is true, an empty QCPDataSelection is returned if this plottable is not selectable (i.e. if QCPAbstractPlottable::setSelectable is QCP::stNone).
- Note
- rect must be a normalized rect (positive or zero width and height). This is especially important when using the rect of QCPSelectionRect::accepted, which is not necessarily normalized. Use
QRect::normalized()
when passing a rect which might not be normalized.
Implemented in QCPAbstractPlottable1D< DataType >, QCPAbstractPlottable1D< QCPBarsData >, QCPAbstractPlottable1D< QCPCurveData >, QCPAbstractPlottable1D< QCPFinancialData >, QCPAbstractPlottable1D< QCPGraphData >, QCPAbstractPlottable1D< QCPStatisticalBoxData >, QCPBars, QCPErrorBars, QCPFinancial, and QCPStatisticalBox.
◆ sortKeyIsMainKey()
|
pure virtual |
Returns whether the sort key (dataSortKey) is identical to the main key (dataMainKey).
What the sort and main keys are, is defined by the plottable's data type. See the QCPDataContainer DataType documentation for details about this naming convention.
Implemented in QCPAbstractPlottable1D< DataType >, QCPAbstractPlottable1D< QCPBarsData >, QCPAbstractPlottable1D< QCPCurveData >, QCPAbstractPlottable1D< QCPFinancialData >, QCPAbstractPlottable1D< QCPGraphData >, QCPAbstractPlottable1D< QCPStatisticalBoxData >, and QCPErrorBars.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.