QRoundProgressBar
#include <QRoundProgressBar.h>
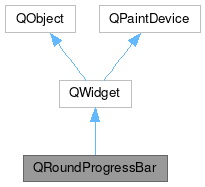
Public Types | |
enum | BarStyle { StyleDonut , StylePie , StyleLine } |
![]() | |
enum | RenderFlag |
![]() | |
enum | PaintDeviceMetric |
Public Slots | |
void | setMaximum (double max) |
void | setMinimum (double min) |
void | setRange (double min, double max) |
void | setValue (double val) |
void | setValue (int val) |
Public Member Functions | |
QRoundProgressBar (QWidget *parent=nullptr) | |
BarStyle | barStyle () const |
double | dataPenWidth () const |
int | decimals () const |
QString | format () const |
double | maximum () const |
double | minimum () const |
double | nullPosition () const |
double | outlinePenWidth () const |
void | resetFormat () |
void | setBarStyle (BarStyle style) |
void | setDataColors (const QGradientStops &stopPoints) |
void | setDataPenWidth (double penWidth) |
void | setDecimals (int count) |
void | setFormat (const QString &format) |
void | setNullPosition (double position) |
void | setOutlinePenWidth (double penWidth) |
double | value () const |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
virtual QSize | sizeHint () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
Static Public Attributes | |
static const int | PositionBottom = -90 |
static const int | PositionLeft = 180 |
static const int | PositionRight = 0 |
static const int | PositionTop = 90 |
Protected Member Functions | |
virtual void | calculateInnerRect (const QRectF &baseRect, double outerRadius, QRectF &innerRect, double &innerRadius) |
virtual void | drawBackground (QPainter &p, const QRectF &baseRect) |
virtual void | drawBase (QPainter &p, const QRectF &baseRect) |
virtual void | drawInnerBackground (QPainter &p, const QRectF &innerRect) |
virtual void | drawText (QPainter &p, const QRectF &innerRect, double innerRadius, double value) |
virtual void | drawValue (QPainter &p, const QRectF &baseRect, double value, double arcLength) |
bool | hasHeightForWidth () const override |
int | heightForWidth (int w) const override |
QSize | minimumSizeHint () const override |
void | paintEvent (QPaintEvent *event) override |
void | rebuildDataBrushIfNeeded () |
virtual void | valueFormatChanged () |
virtual QString | valueToText (double value) const |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | changeEvent (QEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
virtual void | contextMenuEvent (QContextMenuEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEnterEvent *event) |
virtual bool | event (QEvent *event) override |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | initPainter (QPainter *painter) const const override |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | leaveEvent (QEvent *event) |
virtual int | metric (PaintDeviceMetric m) const const override |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | nativeEvent (const QByteArray &eventType, void *message, qintptr *result) |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus (Qt::InputMethodQuery query) |
virtual void | wheelEvent (QWheelEvent *event) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
BarStyle | m_barStyle |
double | m_dataPenWidth |
int | m_decimals |
QString | m_format |
QGradientStops | m_gradientData |
double | m_max |
double | m_min |
double | m_nullPosition |
double | m_outlinePenWidth |
bool | m_rebuildBrush |
int | m_updateFlags |
double | m_value |
Static Protected Attributes | |
static const int | UF_MAX = 4 |
static const int | UF_PERCENT = 2 |
static const int | UF_VALUE = 1 |
Detailed Description
The QRoundProgressBar class represents a circular progress bar and maintains its API similar to the QProgressBar.
Styles
QRoundProgressBar currently supports Donut, Pie and Line styles. See setBarStyle() for more details.
Colors
Generally QRoundProgressBar uses its palette and font attributes to define how it will look.
The following QPalette members are considered:
- QPalette::Window background of the whole widget (normally should be set to Qt::NoBrush)
- QPalette::Base background of the non-filled progress bar area (should be set to Qt::NoBrush to make it transparent)
- QPalette::AlternateBase background of the central circle where the text is shown (for Donut style)
- QPalette::Shadow foreground of the non-filled progress bar area (i.e. border color)
- QPalette::Highlight background of the filled progress bar area
- QPalette::Text color of the text shown in the center
Create a QPalette with given attributes and apply it via setPalette()
.
Color gradient
Donut and Pie styles allow to use color gradient for currernt value area instead of plain brush fill. See setDataColors() for more details.
Value text
Value text is generally drawn inside the QRoundProgressBar using its font()
and QPalette::Text role from its palette()
.
To define pattern of the text, use setFormat() function (see Qt's QProgressBar for more details).
To define number of decimals to be shown, use setDecimals() function.
Font
To use own font for value text, apply it via setFont()
.
By default, font size will be adjusted automatically to fit the inner circle of the widget.
Definition at line 60 of file QRoundProgressBar.h.
Member Enumeration Documentation
◆ BarStyle
The BarStyle enum defines general look of the progress bar.
Enumerator | |
---|---|
StyleDonut | Donut style (filled torus around the text) |
StylePie | Pie style (filled pie segment with the text in center) |
StyleLine | Line style (thin round line around the text) |
Definition at line 86 of file QRoundProgressBar.h.
Constructor & Destructor Documentation
◆ QRoundProgressBar()
|
explicit |
Definition at line 24 of file QRoundProgressBar.cpp.
Member Function Documentation
◆ barStyle()
|
inline |
Returns current progree bar style.
- See also
- setBarStyle
Definition at line 104 of file QRoundProgressBar.h.
◆ calculateInnerRect()
|
protectedvirtual |
Definition at line 284 of file QRoundProgressBar.cpp.
◆ dataPenWidth()
|
inline |
Returns width of the data circle pen.
Definition at line 124 of file QRoundProgressBar.h.
◆ decimals()
|
inline |
Returns number of decimals to show after the comma (default is 1).
- See also
- setFormat, setDecimals
Definition at line 164 of file QRoundProgressBar.h.
◆ drawBackground()
Definition at line 221 of file QRoundProgressBar.cpp.
◆ drawBase()
Definition at line 226 of file QRoundProgressBar.cpp.
◆ drawInnerBackground()
|
protectedvirtual |
Definition at line 301 of file QRoundProgressBar.cpp.
◆ drawText()
|
protectedvirtual |
Definition at line 310 of file QRoundProgressBar.cpp.
◆ drawValue()
|
protectedvirtual |
Definition at line 253 of file QRoundProgressBar.cpp.
◆ format()
|
inline |
Returns the string used to generate the current text.
Definition at line 153 of file QRoundProgressBar.h.
◆ hasHeightForWidth()
|
inlineoverrideprotectedvirtual |
Reimplemented from QWidget.
Definition at line 228 of file QRoundProgressBar.h.
◆ heightForWidth()
|
inlineoverrideprotectedvirtual |
Reimplemented from QWidget.
Definition at line 229 of file QRoundProgressBar.h.
◆ maximum()
|
inline |
Returns maximum of the allowed value range.
- See also
- setMaximum, setRange
Definition at line 180 of file QRoundProgressBar.h.
◆ minimum()
|
inline |
Returns minimum of the allowed value range.
- See also
- setMinimum, setRange
Definition at line 175 of file QRoundProgressBar.h.
◆ minimumSizeHint()
|
inlineoverrideprotectedvirtual |
Reimplemented from QWidget.
Definition at line 226 of file QRoundProgressBar.h.
◆ nullPosition()
|
inline |
Return position (in degrees) of minimum value.
- See also
- setNullPosition
Definition at line 75 of file QRoundProgressBar.h.
◆ outlinePenWidth()
|
inline |
Returns width of the outline circle pen.
Definition at line 114 of file QRoundProgressBar.h.
◆ paintEvent()
|
overrideprotectedvirtual |
Reimplemented from QWidget.
Definition at line 160 of file QRoundProgressBar.cpp.
◆ rebuildDataBrushIfNeeded()
|
protected |
Definition at line 360 of file QRoundProgressBar.cpp.
◆ resetFormat()
void QRoundProgressBar::resetFormat | ( | ) |
Sets format string to empty string.
No text will be shown therefore. See setFormat() for more information.
Definition at line 144 of file QRoundProgressBar.cpp.
◆ setBarStyle()
void QRoundProgressBar::setBarStyle | ( | QRoundProgressBar::BarStyle | style | ) |
Sets visual style of the widget.
- See also
- barStyle
Definition at line 93 of file QRoundProgressBar.cpp.
◆ setDataColors()
void QRoundProgressBar::setDataColors | ( | const QGradientStops & | stopPoints | ) |
Sets colors of the visible data and makes gradient brush from them.
Gradient colors can be set for Donut and Pie styles (see setBarStyle() function).
Warning: this function will override widget's palette()
to set dynamically created gradient brush.
- Parameters
-
stopPoints List of colors (should have at least 2 values, see Qt's QGradientStops for more details). Color value at point 0 corresponds to the minimum() value, while color value at point 1 corresponds to the maximum(). Other colors will be distributed accordingly to the defined ranges (see setRange()).
Definition at line 123 of file QRoundProgressBar.cpp.
◆ setDataPenWidth()
void QRoundProgressBar::setDataPenWidth | ( | double | penWidth | ) |
Sets width of the data circle pen.
- Parameters
-
penWidth width of the data circle pen (in pixels)
Definition at line 113 of file QRoundProgressBar.cpp.
◆ setDecimals()
void QRoundProgressBar::setDecimals | ( | int | count | ) |
Sets number of decimals to show after the comma (default is 1).
- See also
- setFormat
Definition at line 150 of file QRoundProgressBar.cpp.
◆ setFormat()
void QRoundProgressBar::setFormat | ( | const QString & | format | ) |
Defines the string used to generate the current text.
If no format is set, no text will be shown.
- Parameters
-
format see QProgressBar's format description
- See also
- setDecimals
Definition at line 134 of file QRoundProgressBar.cpp.
◆ setMaximum
|
slot |
Defines maximum of the allowed value range.
If the current value does not fit into the range, it will be automatically adjusted.
- Parameters
-
max maximum of the allowed value range
- See also
- setRange
Definition at line 55 of file QRoundProgressBar.cpp.
◆ setMinimum
|
slot |
Defines minimum of the allowed value range.
If the current value does not fit into the range, it will be automatically adjusted.
- Parameters
-
min minimum of the allowed value range
- See also
- setRange
Definition at line 50 of file QRoundProgressBar.cpp.
◆ setNullPosition()
void QRoundProgressBar::setNullPosition | ( | double | position | ) |
Defines position of minimum value.
- Parameters
-
position position on the circle (in degrees) of minimum value
- See also
- nullPosition
Definition at line 80 of file QRoundProgressBar.cpp.
◆ setOutlinePenWidth()
void QRoundProgressBar::setOutlinePenWidth | ( | double | penWidth | ) |
Sets width of the outline circle pen.
- Parameters
-
penWidth width of the outline circle pen (in pixels)
Definition at line 103 of file QRoundProgressBar.cpp.
◆ setRange
|
slot |
Defines minimum und maximum of the allowed value range.
If the current value does not fit into the range, it will be automatically adjusted.
- Parameters
-
min minimum of the allowed value range max maximum of the allowed value range
Definition at line 31 of file QRoundProgressBar.cpp.
◆ setValue [1/2]
|
slot |
Sets a value which will be shown on the widget.
Definition at line 60 of file QRoundProgressBar.cpp.
◆ setValue [2/2]
|
slot |
Integer version of the previous slot.
Definition at line 75 of file QRoundProgressBar.cpp.
◆ value()
|
inline |
Returns current value shown on the widget.
- See also
- setValue()
Definition at line 170 of file QRoundProgressBar.h.
◆ valueFormatChanged()
|
protectedvirtual |
Definition at line 344 of file QRoundProgressBar.cpp.
◆ valueToText()
|
protectedvirtual |
Definition at line 325 of file QRoundProgressBar.cpp.
Member Data Documentation
◆ m_barStyle
|
protected |
Definition at line 237 of file QRoundProgressBar.h.
◆ m_dataPenWidth
|
protected |
Definition at line 238 of file QRoundProgressBar.h.
◆ m_decimals
|
protected |
Definition at line 244 of file QRoundProgressBar.h.
◆ m_format
|
protected |
Definition at line 243 of file QRoundProgressBar.h.
◆ m_gradientData
|
protected |
Definition at line 240 of file QRoundProgressBar.h.
◆ m_max
|
protected |
Definition at line 233 of file QRoundProgressBar.h.
◆ m_min
|
protected |
Definition at line 233 of file QRoundProgressBar.h.
◆ m_nullPosition
|
protected |
Definition at line 236 of file QRoundProgressBar.h.
◆ m_outlinePenWidth
|
protected |
Definition at line 238 of file QRoundProgressBar.h.
◆ m_rebuildBrush
|
protected |
Definition at line 241 of file QRoundProgressBar.h.
◆ m_updateFlags
|
protected |
Definition at line 249 of file QRoundProgressBar.h.
◆ m_value
|
protected |
Definition at line 234 of file QRoundProgressBar.h.
◆ PositionBottom
|
static |
Definition at line 69 of file QRoundProgressBar.h.
◆ PositionLeft
|
static |
Definition at line 66 of file QRoundProgressBar.h.
◆ PositionRight
|
static |
Definition at line 68 of file QRoundProgressBar.h.
◆ PositionTop
|
static |
Definition at line 67 of file QRoundProgressBar.h.
◆ UF_MAX
|
staticprotected |
Definition at line 248 of file QRoundProgressBar.h.
◆ UF_PERCENT
|
staticprotected |
Definition at line 247 of file QRoundProgressBar.h.
◆ UF_VALUE
|
staticprotected |
Definition at line 246 of file QRoundProgressBar.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.