kviewshell
GContainer.h File Reference
#include "GException.h"
#include "GSmartPointer.h"
#include <string.h>
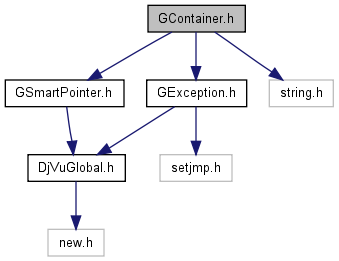

Go to the source code of this file.
Classes | |
class | GArray< TYPE > |
Dynamic array for general types. More... | |
class | GArrayBase |
class | GArrayTemplate< TYPE > |
Common base class for all dynamic arrays. More... | |
class | GCont |
class | GList< TYPE > |
Doubly linked lists. More... | |
class | GListBase |
class | GListImpl< TI > |
class | GListTemplate< TYPE, TI > |
Common base class for all doubly linked lists. More... | |
class | GMap< KTYPE, VTYPE > |
Associative maps. More... | |
class | GMapImpl< K, TI > |
class | GMapTemplate< KTYPE, VTYPE, TI > |
Common base class for all associative maps. More... | |
class | GPArray< TYPE > |
Dynamic array for smart pointers. More... | |
class | GPList< TYPE > |
Doubly linked lists for smart pointers. More... | |
class | GPMap< KTYPE, VTYPE > |
Associative maps for smart-pointers. More... | |
class | GPosition |
Generic iterator class. More... | |
class | GSetBase |
class | GSetImpl< K > |
class | GTArray< TYPE > |
Dynamic array for simple types. More... | |
struct | GCont::HNode |
struct | GCont::ListNode< T > |
struct | GCont::MapNode< K, T > |
struct | GCont::Node |
class | GCont::NormTraits< T > |
struct | GCont::SetNode< K > |
struct | GCont::Traits |
class | GCont::TrivTraits< SZ > |
Defines | |
#define | GCONTAINER_BOUNDS_CHECK 1 |
#define | GCONTAINER_NO_MEMBER_TEMPLATES 0 |
#define | GCONTAINER_NO_TYPENAME 0 |
#define | GCONTAINER_OLD_ITERATORS 1 |
#define | GCONTAINER_ZERO_FILL 1 |
GContainer.h | |
Files #"GContainer.h"# and #"GContainer.cpp"# implement three main template class for generic containers. Class GArray# (see {Dynamic Arrays}) implements an array of objects with variable bounds. Class GList# (see {Doubly Linked Lists}) implements a doubly linked list of objects. Class GMap# (see {Associative Maps}) implements a hashed associative map. The container templates are not thread-safe. Thread safety can be implemented using the facilities provided in {GThreads.h}. Template class for generic containers.
| |
#define | GCONT GCont:: |
Functions | |
Hash functions | |
These functions let you use template class {GMap} with the corresponding elementary types.
The returned hash code may be reduced to an arbitrary range by computing its remainder modulo the upper bound of the range. Hash functions for elementary types. | |
static unsigned int | hash (const double &x) |
static unsigned int | hash (const float &x) |
static unsigned int | hash (const void *const &x) |
static unsigned int | hash (void *const &x) |
static unsigned int | hash (const unsigned long &x) |
static unsigned int | hash (const long &x) |
static unsigned int | hash (const int &x) |
static unsigned int | hash (const unsigned int &x) |
Define Documentation
#define GCONT GCont:: |
Definition at line 248 of file GContainer.h.
#define GCONTAINER_BOUNDS_CHECK 1 |
Definition at line 86 of file GContainer.h.
#define GCONTAINER_NO_MEMBER_TEMPLATES 0 |
Definition at line 103 of file GContainer.h.
#define GCONTAINER_NO_TYPENAME 0 |
Definition at line 109 of file GContainer.h.
#define GCONTAINER_OLD_ITERATORS 1 |
Definition at line 81 of file GContainer.h.
#define GCONTAINER_ZERO_FILL 1 |
Definition at line 91 of file GContainer.h.
Function Documentation
static unsigned int hash | ( | const double & | x | ) | [inline, static] |
static unsigned int hash | ( | const float & | x | ) | [inline, static] |
static unsigned int hash | ( | const void *const & | x | ) | [inline, static] |
static unsigned int hash | ( | void *const & | x | ) | [inline, static] |
static unsigned int hash | ( | const unsigned long & | x | ) | [inline, static] |
static unsigned int hash | ( | const long & | x | ) | [inline, static] |
static unsigned int hash | ( | const int & | x | ) | [inline, static] |
static unsigned int hash | ( | const unsigned int & | x | ) | [inline, static] |