kviewshell
GMapArea Class Reference
This is the base object for all map areas. More...
#include <GMapAreas.h>
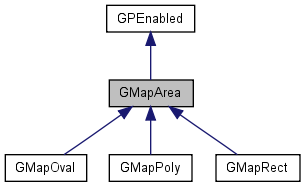
Public Types | |
enum | BorderType { NO_BORDER = 0, XOR_BORDER = 1, SOLID_BORDER = 2, SHADOW_IN_BORDER = 3, SHADOW_OUT_BORDER = 4, SHADOW_EIN_BORDER = 5, SHADOW_EOUT_BORDER = 6 } |
enum | MapAreaType { UNKNOWN, RECT, OVAL, POLY } |
enum | Special_Hilite_Color { NO_HILITE = 0xFFFFFFFF, XOR_HILITE = 0xFF000000 } |
Public Member Functions | |
char const *const | check_object (void) |
GRect | get_bound_rect (void) const |
virtual void | get_coords (GList< int > &CoordList) const |
virtual GP< GMapArea > | get_copy (void) const =0 |
virtual char const *const | get_shape_name (void) const =0 |
virtual MapAreaType const | get_shape_type (void) const |
int | get_xmax (void) const |
int | get_xmin (void) const |
virtual GUTF8String | get_xmltag (const int height) const =0 |
int | get_ymax (void) const |
int | get_ymin (void) const |
bool | is_point_inside (int x, int y) const |
virtual void | map (GRectMapper &mapper)=0 |
void | move (int dx, int dy) |
GUTF8String | print (void) |
void | resize (int new_width, int new_height) |
void | transform (const GRect &grect) |
virtual void | unmap (GRectMapper &mapper)=0 |
virtual | ~GMapArea () |
Public Attributes | |
bool | border_always_visible |
unsigned long int | border_color |
BorderType | border_type |
int | border_width |
GUTF8String | comment |
unsigned long int | hilite_color |
GUTF8String | target |
GUTF8String | url |
Static Public Attributes | |
static const char | BORDER_AVIS_TAG [] = "border_avis" |
static const char | HILITE_TAG [] = "hilite" |
static const char | MAPAREA_TAG [] = "maparea" |
static const char | NO_BORDER_TAG [] = "none" |
static const char | OVAL_TAG [] = "oval" |
static const char | POLY_TAG [] = "poly" |
static const char | RECT_TAG [] = "rect" |
static const char | SHADOW_EIN_BORDER_TAG [] = "shadow_ein" |
static const char | SHADOW_EOUT_BORDER_TAG [] = "shadow_eout" |
static const char | SHADOW_IN_BORDER_TAG [] = "shadow_in" |
static const char | SHADOW_OUT_BORDER_TAG [] = "shadow_out" |
static const char | SOLID_BORDER_TAG [] = "border" |
static const char | TARGET_SELF [] = "_self" |
static const char | URL_TAG [] = "url" |
static const char | XOR_BORDER_TAG [] = "xor" |
Protected Member Functions | |
void | clear_bounds (void) |
virtual char const *const | gma_check_object (void) const =0 |
virtual int | gma_get_xmax (void) const =0 |
virtual int | gma_get_xmin (void) const =0 |
virtual int | gma_get_ymax (void) const =0 |
virtual int | gma_get_ymin (void) const =0 |
virtual bool | gma_is_point_inside (const int x, const int y) const =0 |
virtual void | gma_move (int dx, int dy)=0 |
virtual GUTF8String | gma_print (void)=0 |
virtual void | gma_resize (int new_width, int new_height)=0 |
virtual void | gma_transform (const GRect &grect)=0 |
GMapArea (void) |
Detailed Description
This is the base object for all map areas.It defines some standard interface to access the geometrical properties of the areas and describes the area itsef: {itemize} url# If the optional URL# is specified, the map area will also work as a hyperlink meaning that if you click it with your mouse pointer, the browser will be advised to load the page referenced by the URL#. target# Defines where the specified URL# should be loaded comment# This is a string displayed in a status line or in a popup window when the mouse pointer moves over the hyperlink area border_type#, border_color# and border_width# describes how the area border should be drawn area_color# describes how the area should be highlighted. {itemize}
The map areas can be displayed using two different techniques, which can be combined together: {itemize} Visible border. The border of a map area can be drawn in several different ways (like XOR_BORDER# or SHADOW_IN_BORDER#). It can be made always visible, or appearing only when the mouse pointer moves over the map area. Highlighted contents. Contents of rectangular map areas can also be highlighted with some given color. {itemize}
Definition at line 135 of file GMapAreas.h.
Member Enumeration Documentation
enum GMapArea::BorderType |
- Enumerator:
-
NO_BORDER XOR_BORDER SOLID_BORDER SHADOW_IN_BORDER SHADOW_OUT_BORDER SHADOW_EIN_BORDER SHADOW_EOUT_BORDER
Definition at line 162 of file GMapAreas.h.
Constructor & Destructor Documentation
GMapArea::GMapArea | ( | void | ) | [protected] |
Definition at line 888 of file GMapAreas.cpp.
GMapArea::~GMapArea | ( | ) | [virtual] |
Member Function Documentation
char const *const GMapArea::check_object | ( | void | ) |
Checks if the object is OK.
Especially useful with {GMapPoly} where edges may intersect. If there is a problem it returns a string describing it.
Definition at line 206 of file GMapAreas.cpp.
void GMapArea::clear_bounds | ( | void | ) | [inline, protected] |
Definition at line 276 of file GMapAreas.h.
GRect GMapArea::get_bound_rect | ( | void | ) | const |
void GMapArea::get_coords | ( | GList< int > & | CoordList | ) | const [virtual] |
Virtual function generating a list of defining coordinates (default are the opposite corners of the enclosing rectangle).
Reimplemented in GMapPoly.
Definition at line 378 of file GMapAreas.cpp.
virtual char const* const GMapArea::get_shape_name | ( | void | ) | const [pure virtual] |
virtual MapAreaType const GMapArea::get_shape_type | ( | void | ) | const [inline, virtual] |
Virtual function returning the shape type.
Reimplemented in GMapRect, GMapPoly, and GMapOval.
Definition at line 251 of file GMapAreas.h.
int GMapArea::get_xmax | ( | void | ) | const |
Returns xmax of the bounding rectangle.
In other words, if X# is a coordinate of the last point in the right direction, the function will return X+1#
Definition at line 145 of file GMapAreas.cpp.
int GMapArea::get_xmin | ( | void | ) | const |
virtual GUTF8String GMapArea::get_xmltag | ( | const int | height | ) | const [pure virtual] |
int GMapArea::get_ymax | ( | void | ) | const |
Returns xmax of the bounding rectangle.
In other words, if Y# is a coordinate of the last point in the top direction, the function will return Y+1#
Definition at line 153 of file GMapAreas.cpp.
int GMapArea::get_ymin | ( | void | ) | const |
virtual char const* const GMapArea::gma_check_object | ( | void | ) | const [protected, pure virtual] |
virtual int GMapArea::gma_get_xmax | ( | void | ) | const [protected, pure virtual] |
virtual int GMapArea::gma_get_xmin | ( | void | ) | const [protected, pure virtual] |
virtual int GMapArea::gma_get_ymax | ( | void | ) | const [protected, pure virtual] |
virtual int GMapArea::gma_get_ymin | ( | void | ) | const [protected, pure virtual] |
virtual bool GMapArea::gma_is_point_inside | ( | const int | x, | |
const int | y | |||
) | const [protected, pure virtual] |
virtual void GMapArea::gma_move | ( | int | dx, | |
int | dy | |||
) | [protected, pure virtual] |
virtual GUTF8String GMapArea::gma_print | ( | void | ) | [protected, pure virtual] |
virtual void GMapArea::gma_resize | ( | int | new_width, | |
int | new_height | |||
) | [protected, pure virtual] |
virtual void GMapArea::gma_transform | ( | const GRect & | grect | ) | [protected, pure virtual] |
bool GMapArea::is_point_inside | ( | int | x, | |
int | y | |||
) | const |
Returns 1 if the given point is inside the hyperlink area.
Definition at line 237 of file GMapAreas.cpp.
virtual void GMapArea::map | ( | GRectMapper & | mapper | ) | [pure virtual] |
void GMapArea::move | ( | int | dx, | |
int | dy | |||
) |
Moves the hyperlink along the given vector.
Is used by the hyperlinks editor.
Definition at line 168 of file GMapAreas.cpp.
GUTF8String GMapArea::print | ( | void | ) |
Stores the contents of the hyperlink object in a lisp-like format for saving into ANTa# chunk (see {DjVuAnno}).
Definition at line 246 of file GMapAreas.cpp.
void GMapArea::resize | ( | int | new_width, | |
int | new_height | |||
) |
Resizes the hyperlink to fit new bounding rectangle while keeping the (xmin, ymin) points at rest.
Definition at line 184 of file GMapAreas.cpp.
void GMapArea::transform | ( | const GRect & | grect | ) |
Transforms the hyperlink to be within the specified rectangle.
Definition at line 195 of file GMapAreas.cpp.
virtual void GMapArea::unmap | ( | GRectMapper & | mapper | ) | [pure virtual] |
Member Data Documentation
If TRUE#, the border will be made always visible.
Otherwise it will be drawn when the mouse moves over the map area.
Definition at line 204 of file GMapAreas.h.
const char GMapArea::BORDER_AVIS_TAG = "border_avis" [static] |
Definition at line 157 of file GMapAreas.h.
unsigned long int GMapArea::border_color |
Border type.
Defines how the map area border should be drawn {itemize} NO_BORDER# - No border drawn XOR_BORDER# - The border is drawn using XOR method. SOLID_BORDER# - The border is drawn as a solid line of a given color. SHADOW_IN_BORDER# - Supported for {GMapRect} only. The map area area looks as if it was "pushed-in". SHADOW_OUT_BORDER# - The opposite of SHADOW_OUT_BORDER# SHADOW_EIN_BORDER# - Also for {GMapRect} only. Is translated as "shadow etched in" SHADOW_EOUT_BORDER# - The opposite of SHADOW_EIN_BORDER#. {itemize}
Definition at line 201 of file GMapAreas.h.
Comment (displayed in a status line or as a popup hint when the mouse pointer moves over the map area.
Definition at line 187 of file GMapAreas.h.
unsigned long int GMapArea::hilite_color |
Specified a color for highlighting the internal area of the map area.
Will work with rectangular map areas only. The color is specified in #00RRGGBB format. A special value of #FFFFFFFF disables highlighting and #FF000000 is for XOR highlighting.
Definition at line 213 of file GMapAreas.h.
const char GMapArea::HILITE_TAG = "hilite" [static] |
Definition at line 158 of file GMapAreas.h.
const char GMapArea::MAPAREA_TAG = "maparea" [static] |
Definition at line 146 of file GMapAreas.h.
const char GMapArea::NO_BORDER_TAG = "none" [static] |
Definition at line 150 of file GMapAreas.h.
const char GMapArea::OVAL_TAG = "oval" [static] |
Definition at line 149 of file GMapAreas.h.
const char GMapArea::POLY_TAG = "poly" [static] |
Definition at line 148 of file GMapAreas.h.
const char GMapArea::RECT_TAG = "rect" [static] |
Definition at line 147 of file GMapAreas.h.
const char GMapArea::SHADOW_EIN_BORDER_TAG = "shadow_ein" [static] |
Definition at line 155 of file GMapAreas.h.
const char GMapArea::SHADOW_EOUT_BORDER_TAG = "shadow_eout" [static] |
Definition at line 156 of file GMapAreas.h.
const char GMapArea::SHADOW_IN_BORDER_TAG = "shadow_in" [static] |
Definition at line 153 of file GMapAreas.h.
const char GMapArea::SHADOW_OUT_BORDER_TAG = "shadow_out" [static] |
Definition at line 154 of file GMapAreas.h.
const char GMapArea::SOLID_BORDER_TAG = "border" [static] |
Definition at line 152 of file GMapAreas.h.
The target for the URL#.
Standard targets are: {itemize} _blank# - Load the link in a new blank window _self# - Load the link into the plugin window _top# - Load the link into the top-level frame {itemize}
Definition at line 184 of file GMapAreas.h.
const char GMapArea::TARGET_SELF = "_self" [static] |
Definition at line 160 of file GMapAreas.h.
Optional URL which this map area can be associated with.
If it's not empty then clicking this map area with the mouse will make the browser load the HTML page referenced by this url#. Note: This may also be a relative URL, so the GURL class is not used.
Definition at line 177 of file GMapAreas.h.
const char GMapArea::URL_TAG = "url" [static] |
Definition at line 159 of file GMapAreas.h.
const char GMapArea::XOR_BORDER_TAG = "xor" [static] |
Definition at line 151 of file GMapAreas.h.
The documentation for this class was generated from the following files: