kviewshell
GPixmap Class Reference
RGB Color images. More...
#include <GPixmap.h>
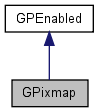
Public Member Functions | |
void | destroy (void) |
int | get_grays (void) const |
void | set_grays (int) |
virtual | ~GPixmap () |
Blitting and applying stencils. | |
These function is essential for rendering DjVu images.
The elementary functions are {attenuate} and {blit}. The combined functions {blend} and {stencil} should be viewed as optimizations. | |
void | attenuate (const GBitmap *bm, int x, int y) |
void | blend (const GBitmap *bm, int x, int y, const GPixmap *color) |
void | blit (const GBitmap *bm, int x, int y, const GPixmap *color) |
void | blit (const GBitmap *bm, int x, int y, const GPixel *color) |
void | stencil (const GBitmap *bm, const GPixmap *pm, int pms, const GRect *pmr, double corr=1.0) |
Stealing or borrowing the memory buffer (advanced). | |
void | borrow_data (GPixel &data, int w, int h) |
void | donate_data (GPixel *data, int w, int h) |
GP< GPixmap > | rotate (int count=0) |
GPixel * | take_data (size_t &offset) |
Accessing pixels. | |
unsigned int | columns () const |
GPixel * | operator[] (int row) |
const GPixel * | operator[] (int row) const |
unsigned int | rows () const |
unsigned int | rowsize () const |
Resampling images. | |
void | downsample (const GPixmap *src, int factor, const GRect *rect=0) |
void | downsample43 (const GPixmap *src, const GRect *rect=0) |
void | upsample (const GPixmap *src, int factor, const GRect *rect=0) |
void | upsample23 (const GPixmap *src, const GRect *rect=0) |
Miscellaneous. | |
unsigned int | get_memory_usage () const |
void | save_ppm (ByteStream &bs, int raw=1) const |
Initialization. | |
void | init (ByteStream &ref) |
void | init (const GBitmap &ref, const GRect &rect, const GPixel *ramp=0) |
void | init (const GBitmap &ref, const GPixel *ramp=0) |
void | init (const GPixmap &ref, const GRect &rect) |
void | init (const GPixmap &ref) |
void | init (int nrows, int ncolumns, const GPixel *filler=0) |
GPixmap & | operator= (const GPixmap &ref) |
GPixmap & | operator= (const GBitmap &ref) |
Static Public Member Functions | |
Construction. | |
static GP< GPixmap > | create (ByteStream &ref) |
static GP< GPixmap > | create (const GPixmap &ref, const GRect &rect) |
static GP< GPixmap > | create (const GPixmap &ref) |
static GP< GPixmap > | create (const GBitmap &ref, const GRect &rect) |
static GP< GPixmap > | create (const GBitmap &ref) |
static GP< GPixmap > | create (const int nrows, const int ncolumns, const GPixel *filler=0) |
static GP< GPixmap > | create (void) |
Protected Member Functions | |
GPixmap (ByteStream &ref) | |
GPixmap (const GPixmap &ref, const GRect &rect) | |
GPixmap (const GPixmap &ref) | |
GPixmap (const GBitmap &ref, const GRect &rect) | |
GPixmap (const GBitmap &ref) | |
GPixmap (int nrows, int ncolumns, const GPixel *filler=0) | |
GPixmap (void) | |
Protected Attributes | |
unsigned short | ncolumns |
unsigned short | nrows |
unsigned short | nrowsize |
GPixel * | pixels |
GPixel * | pixels_data |
Manipulating colors. | |
void | color_correct (double corr) |
void | ordered_32k_dither (int xmin=0, int ymin=0) |
void | ordered_666_dither (int xmin=0, int ymin=0) |
static void | color_correct (double corr, GPixel *pixels, int npixels) |
Detailed Description
RGB Color images.Instances of class GPixmap# represent color images as a two dimensional array of pixels {GPixel}. The bracket operator returns a pointer to the pixels composing one line of the image. This pointer can be used as an array to read or write the pixels of this particular line. Following the general convention of the DjVu Reference Library, line zero is always the bottom line of the image.
Definition at line 148 of file GPixmap.h.
Constructor & Destructor Documentation
GPixmap::GPixmap | ( | void | ) | [protected] |
Definition at line 181 of file GPixmap.cpp.
GPixmap::GPixmap | ( | int | nrows, | |
int | ncolumns, | |||
const GPixel * | filler = 0 | |||
) | [protected] |
Definition at line 186 of file GPixmap.cpp.
GPixmap::GPixmap | ( | const GBitmap & | ref | ) | [protected] |
Definition at line 216 of file GPixmap.cpp.
Definition at line 231 of file GPixmap.cpp.
GPixmap::GPixmap | ( | const GPixmap & | ref | ) | [protected] |
Definition at line 246 of file GPixmap.cpp.
Definition at line 261 of file GPixmap.cpp.
GPixmap::GPixmap | ( | ByteStream & | ref | ) | [protected] |
Definition at line 201 of file GPixmap.cpp.
GPixmap::~GPixmap | ( | ) | [virtual] |
Member Function Documentation
void GPixmap::attenuate | ( | const GBitmap * | bm, | |
int | x, | |||
int | y | |||
) |
Attenuates the color image in preparation for a blit.
Bitmap bm# is positionned at location x#,y# over this color image. The matching color image pixels are then multiplied by #1.0-Alpha# where Alpha# denotes the gray value, in range #[0,1]#, represented by the corresponding pixel of bitmap bm#.
Definition at line 1295 of file GPixmap.cpp.
Performs alpha blending.
This function is similar to first calling {attenuate} with alpha map bm# and then calling {blit} with alpha map bm# and color map color#. Both operations are performed together for efficiency reasons.
Definition at line 1459 of file GPixmap.cpp.
Blits pixmap color# through transparency mask bm#.
Bitmap bm# is positionned at location x#,y# over this color image. The matching color image pixels are then modified by adding the corresponding pixel color in pixmap color#, multiplied by Alpha#, where Alpha# denotes the gray value, in range #[0,1]#, represented by the corresponding pixel of bitmap bm#.
Definition at line 1401 of file GPixmap.cpp.
Blits solid color color# through transparency mask bm#.
Bitmap bm# is positionned at location x#,y# over this color image. The matching color image pixels are then modified by adding color color# multiplied by Alpha#, where Alpha# denotes the gray value, in range #[0,1]#, represented by the corresponding pixel of bitmap bm#.
Definition at line 1345 of file GPixmap.cpp.
void GPixmap::borrow_data | ( | GPixel & | data, | |
int | w, | |||
int | h | |||
) | [inline] |
Initializes this GPixmap by borrowing a memory segment.
The GPixmap then directly addresses the memory buffer data# provided by the user. This buffer must be large enough to hold w*h# GPixels. The GPixmap object does not ``own'' the buffer: you must explicitly de-allocate the buffer using operator delete []#. This de-allocation should take place after the destruction or the re-initialization of the GPixmap object.
void GPixmap::color_correct | ( | double | corr, | |
GPixel * | pixels, | |||
int | npixels | |||
) | [static] |
Applies a luminance gamma correction to an array of pixels.
This function is {static} and does not modify this pixmap.
Definition at line 673 of file GPixmap.cpp.
void GPixmap::color_correct | ( | double | corr | ) |
Applies a luminance gamma correction factor of corr#.
Values greater than #1.0# make the image brighter. Values smaller than #1.0# make the image darker. The documentation of program {ppmcoco} explains how to properly use this function.
Definition at line 650 of file GPixmap.cpp.
unsigned int GPixmap::columns | ( | ) | const [inline] |
static GP<GPixmap> GPixmap::create | ( | ByteStream & | ref | ) | [inline, static] |
Creates a GPixmap by reading PPM data from ByteStream ref#.
See {PNM and RLE file formats} for more information.
Creates a GPixmap by copying the rectangle rect# of the gray level image ref#.
The constructed GPixmap has the same size as rectangle rect#. The pixels are initialized with shades of grays converted from the ink levels represented in ref#. This conversion depends on the number of gray levels in ref#.
void GPixmap::destroy | ( | void | ) |
void GPixmap::donate_data | ( | GPixel * | data, | |
int | w, | |||
int | h | |||
) |
Identical to the above, but GPixmap will do the delete [].
Definition at line 410 of file GPixmap.cpp.
Resets this GPixmap with a subsampled segment of color image src#.
This function conceptually rescales image src# by a factor #1:factor#, and copies rectangle rect# of the subsampled image into the current GPixmap. The full subsampled image is copied if rect# is a null pointer. Both operations are however performed together for efficiency reasons. Subsampling works by averaging the colors of the source pixels located in small squares of size factor# times factor#.
Definition at line 812 of file GPixmap.cpp.
Resets this GPixmap with a rescaled segment of src# (zoom 75%).
This function conceptually rescales image src# by a factor #3:4#, and copies rectangle rect# of the rescaled image into the current GPixmap. The full rescaled image is copied if rect# is a null pointer. Both operations are however performed together for efficiency reasons. This function has been superseded by class {GPixmapScaler}.
Definition at line 1098 of file GPixmap.cpp.
unsigned int GPixmap::get_memory_usage | ( | void | ) | const [inline] |
void GPixmap::init | ( | ByteStream & | ref | ) |
Resets the GPixmap by reading PPM data from ByteStream ref#.
See {PNM and RLE file formats} for more information.
Definition at line 463 of file GPixmap.cpp.
Resets the GPixmap by copying the rectangle rect# of the gray level image ref#.
The optional argument ramp# is an array of 256 pixel values used for mapping the gray levels to color values. Setting ramp# to zero selects a linear ramp computed according to the maximal number of gray levels in ref#.
Definition at line 335 of file GPixmap.cpp.
Resets the GPixmap by copying the size and the contents of the gray level image ref#.
The optional argument ramp# is an array of 256 pixel values used for mapping the gray levels to color values. Setting ramp# to zero selects a linear ramp of shades of gray.
Definition at line 304 of file GPixmap.cpp.
Resets the GPixmap by copying the rectangle rect# of the color image ref#.
The previous content of the GPixmap is discarded.
Definition at line 388 of file GPixmap.cpp.
void GPixmap::init | ( | const GPixmap & | ref | ) |
Resets the GPixmap by copying the size and the contents of the color image ref#.
The previous content of the GPixmap is discarded.
Definition at line 371 of file GPixmap.cpp.
void GPixmap::init | ( | int | nrows, | |
int | ncolumns, | |||
const GPixel * | filler = 0 | |||
) |
GPixel * GPixmap::operator[] | ( | int | row | ) | [inline] |
const GPixel * GPixmap::operator[] | ( | int | row | ) | const [inline] |
void GPixmap::ordered_32k_dither | ( | int | xmin = 0 , |
|
int | ymin = 0 | |||
) |
Dithers the image to 32768 colors.
This function applies an ordered dithering algorithm to reduce the image to 32768 predefined colors. These predefined colors are located on a color cube of 32x32x32 colors: the color RGB coordinates can only take values in which the three least significant bits are set to #1#. This is useful for displaying images with less than 24 bits per pixel. Arguments xmin# and ymin# control the position of the dithering grids. This is useful for dithering tiled images. Arguments xmin# and ymin# must be the position of the bottom left corner of the tile contained in this GPixmap. Properly setting these arguments eliminates dithering artifacts on the tile boundaries.
Definition at line 753 of file GPixmap.cpp.
void GPixmap::ordered_666_dither | ( | int | xmin = 0 , |
|
int | ymin = 0 | |||
) |
Dithers the image to 216 colors.
This function applies an ordered dithering algorithm to reduce the image to 216 predefined colors. These predefined colors are located on a color cube of 6x6x6 colors: the color RGB coordinates can only take the following values: #0#, #51#, #102#, #163#, #214# or #255#. This is useful for displaying images on a device supporting a maximum of 256 colors. Arguments xmin# and ymin# control the position of the dithering grids. This is useful for dithering tiled images. Arguments xmin# and ymin# must be the position of the bottom left corner of the tile contained in this GPixmap. Properly setting these arguments eliminates dithering artifacts on the tile boundaries.
Definition at line 699 of file GPixmap.cpp.
Rotates pixmap by 90, 180 or 270 degrees anticlockwise and returns a new pixmap, input pixmap is not changed.
count can be 1, 2, or 3 for 90, 180, 270 degree rotation. It returns the same pixmap if not rotated.
Definition at line 1604 of file GPixmap.cpp.
unsigned int GPixmap::rows | ( | ) | const [inline] |
unsigned int GPixmap::rowsize | ( | ) | const [inline] |
void GPixmap::save_ppm | ( | ByteStream & | bs, | |
int | raw = 1 | |||
) | const |
Saves the image into ByteStream bs# using the PPM format.
Argument raw# selects the ``Raw PPM'' (1) or the ``Ascii PPM'' (0) format. See {PNM and RLE file formats} for more information.
Definition at line 547 of file GPixmap.cpp.
void GPixmap::stencil | ( | const GBitmap * | bm, | |
const GPixmap * | pm, | |||
int | pms, | |||
const GRect * | pmr, | |||
double | corr = 1.0 | |||
) |
Resample color pixmap and performs color corrected alpha blending.
This function conceptually computes an intermediate color image by first upsampling the GPixmap pm# by a factor pms:1# (see {upsample}), extracting the sub-image designated by rectangle pmr# and applying color correction corr# (see {color_correct}). This intermediate color image is then blended into this pixel map according to the alpha map bm# (see {blend}).
Definition at line 1518 of file GPixmap.cpp.
GPixel * GPixmap::take_data | ( | size_t & | offset | ) |
Steals the memory buffer of a GPixmap.
This function returns the address of the memory buffer allocated by this GPixmap object. The offset of the first pixel in the bottom line is written into variable offset#. Other lines can be accessed using pointer arithmetic (see {rowsize}). The GPixmap object no longer ``owns'' the buffer: you must explicitly de-allocate the buffer using operator delete []#. This de-allocation should take place after the destruction or the re-initialization of the GPixmap object. This function will return a null pointer if the GPixmap object does not ``own'' the buffer in the first place.
Definition at line 421 of file GPixmap.cpp.
Resets this GPixmap with a oversampled segment of color image src#.
This function conceptually rescales image src# by a factor factor:1#, and copies rectangle rect# of the oversampled image into the current GPixmap. The full oversampled image is copied if rect# is a null pointer. Both operations are however performed together for efficiency reasons. Oversampling works by replicating the color of the source pixels into squares of size factor# times factor#.
Definition at line 898 of file GPixmap.cpp.
Resets this GPixmap with a rescaled segment of src# (zoom 150%).
This function conceptually rescales image src# by a factor #3:2# and copies rectangle rect# of the rescaled image into the current GPixmap. The full rescaled image is copied if rect# is a null pointer. Both operations are however performed together for efficiency reasons. This function has been superseded by class {GPixmapScaler}.
Definition at line 1188 of file GPixmap.cpp.
Member Data Documentation
unsigned short GPixmap::ncolumns [protected] |
unsigned short GPixmap::nrows [protected] |
unsigned short GPixmap::nrowsize [protected] |
GPixel* GPixmap::pixels [protected] |
GPixel* GPixmap::pixels_data [protected] |
The documentation for this class was generated from the following files: