KDECore
KShortcutList Class Reference
KShortcutList is an abstract base class for KAccelShortcutList and KStdAccel::ShortcutList. More...
#include <kshortcutlist.h>
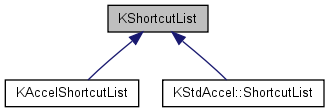
Public Types | |
enum | Other |
Public Member Functions | |
virtual uint | count () const =0 |
virtual QVariant | getOther (Other, uint index) const =0 |
virtual int | index (const KKeySequence &keySeq) const |
virtual int | index (const QString &sName) const |
virtual const KInstance * | instance () const |
virtual bool | isConfigurable (uint index) const =0 |
virtual bool | isGlobal (uint index) const |
KShortcutList () | |
virtual QString | label (uint index) const =0 |
virtual QString | name (uint index) const =0 |
virtual bool | readSettings (const QString &sConfigGroup=QString::null, KConfigBase *pConfig=0) |
virtual bool | save () const =0 |
virtual bool | setOther (Other, uint index, QVariant)=0 |
virtual bool | setShortcut (uint index, const KShortcut &shortcut)=0 |
virtual const KShortcut & | shortcut (uint index) const =0 |
virtual const KShortcut & | shortcutDefault (uint index) const =0 |
virtual QString | whatsThis (uint index) const =0 |
virtual bool | writeSettings (const QString &sConfigGroup=QString::null, KConfigBase *pConfig=0, bool bWriteAll=false, bool bGlobal=false) const |
virtual | ~KShortcutList () |
Protected Member Functions | |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
KShortcutList is an abstract base class for KAccelShortcutList and KStdAccel::ShortcutList.It gives you an unified interface for accessing the accelerator lists of KAccel (using KAccelShortcutList), KGlobalAccel (using KAccelShortcutList), and KStdAccel (using KStdAccel::ShortcutList).
Base class for accessing accelerator lists
Definition at line 48 of file kshortcutlist.h.
Member Enumeration Documentation
enum KShortcutList::Other |
Definition at line 146 of file kshortcutlist.h.
Constructor & Destructor Documentation
KShortcutList::KShortcutList | ( | ) |
KShortcutList::~KShortcutList | ( | ) | [virtual] |
Definition at line 22 of file kshortcutlist.cpp.
Member Function Documentation
virtual uint KShortcutList::count | ( | ) | const [pure virtual] |
Returns the number of entries.
- Returns:
- the number of entries
Implemented in KAccelShortcutList, and KStdAccel::ShortcutList.
For internal use only.
Implemented in KAccelShortcutList, and KStdAccel::ShortcutList.
Definition at line 61 of file kshortcutlist.cpp.
int KShortcutList::index | ( | const KKeySequence & | keySeq | ) | const [virtual] |
Returns the index of the shortcut with he given key sequence.
- Parameters:
-
keySeq the key sequence to search for
- Returns:
- the index of the shortcut, of -1 if not found
Definition at line 42 of file kshortcutlist.cpp.
int KShortcutList::index | ( | const QString & | sName | ) | const [virtual] |
Returns the index of the shortcut with he given name.
- Parameters:
-
sName the name of the shortcut to search
- Returns:
- the index of the shortcut, of -1 if not found
Definition at line 31 of file kshortcutlist.cpp.
const KInstance * KShortcutList::instance | ( | ) | const [virtual] |
The KInstance.
- Returns:
- the KInstance of the list, can be 0 if not available
Definition at line 56 of file kshortcutlist.cpp.
virtual bool KShortcutList::isConfigurable | ( | uint | index | ) | const [pure virtual] |
Checks whether the shortcut with the given index
is configurable.
- Parameters:
-
index the index of the shortcut (must be < count())
- Returns:
- true if configurable, false otherwise
Implemented in KAccelShortcutList, and KStdAccel::ShortcutList.
bool KShortcutList::isGlobal | ( | uint | index | ) | const [virtual] |
Checks whether the shortcut with the given index
is saved in the global configuration.
- Parameters:
-
index the index of the shortcut (must be < count())
- Returns:
- true if global, false otherwise
Reimplemented in KAccelShortcutList.
Definition at line 26 of file kshortcutlist.cpp.
virtual QString KShortcutList::label | ( | uint | index | ) | const [pure virtual] |
Returns the (i18n'd) label of the shortcut with the given index
.
- Parameters:
-
index the index of the shortcut (must be < count())
- Returns:
- the label (i18n'd) of the shortcut
Implemented in KAccelShortcutList, and KStdAccel::ShortcutList.
virtual QString KShortcutList::name | ( | uint | index | ) | const [pure virtual] |
Returns the name of the shortcut with the given index
.
- Parameters:
-
index the index of the shortcut (must be < count())
- Returns:
- the name of the shortcut
Implemented in KAccelShortcutList, and KStdAccel::ShortcutList.
bool KShortcutList::readSettings | ( | const QString & | sConfigGroup = QString::null , |
|
KConfigBase * | pConfig = 0 | |||
) | [virtual] |
Loads the shortcuts from the given configuration file.
- Parameters:
-
sConfigGroup the group in the configuration file pConfig the configuration file to load from
- Returns:
- true if successful, false otherwise
Definition at line 71 of file kshortcutlist.cpp.
virtual bool KShortcutList::save | ( | ) | const [pure virtual] |
Save the shortcut list.
- Returns:
- true if successful, false otherwise
Implemented in KAccelShortcutList, and KStdAccel::ShortcutList.
For internal use only.
Implemented in KAccelShortcutList, and KStdAccel::ShortcutList.
Definition at line 66 of file kshortcutlist.cpp.
Sets the shortcut of the given entry.
- Parameters:
-
index the index of the shortcut (must be < count()) shortcut the shortcut
Implemented in KAccelShortcutList, and KStdAccel::ShortcutList.
virtual const KShortcut& KShortcutList::shortcut | ( | uint | index | ) | const [pure virtual] |
Returns the shortcut with the given index
.
- Parameters:
-
index the index of the shortcut (must be < count())
- Returns:
- the shortcut
- See also:
- shortcutDefault()
Implemented in KAccelShortcutList, and KStdAccel::ShortcutList.
virtual const KShortcut& KShortcutList::shortcutDefault | ( | uint | index | ) | const [pure virtual] |
Returns default shortcut with the given index
.
- Parameters:
-
index the index of the shortcut (must be < count())
- Returns:
- the default shortcut
- See also:
- shortcut()
Implemented in KAccelShortcutList, and KStdAccel::ShortcutList.
void KShortcutList::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
used to extend the interface with virtuals without breaking binary compatibility
Reimplemented in KAccelShortcutList, and KStdAccel::ShortcutList.
Definition at line 212 of file kshortcutlist.cpp.
virtual QString KShortcutList::whatsThis | ( | uint | index | ) | const [pure virtual] |
Returns the (i18n'd) What's This text of the shortcut with the given index
.
- Parameters:
-
index the index of the shortcut (must be < count())
- Returns:
- the What's This text (i18n'd) of the shortcut
Implemented in KAccelShortcutList, and KStdAccel::ShortcutList.
bool KShortcutList::writeSettings | ( | const QString & | sConfigGroup = QString::null , |
|
KConfigBase * | pConfig = 0 , |
|||
bool | bWriteAll = false , |
|||
bool | bGlobal = false | |||
) | const [virtual] |
Writes the shortcuts to the given configuration file.
- Parameters:
-
sConfigGroup the group in the configuration file pConfig the configuration file to save to bWriteAll true to write all actions bGlobal true to write to the global configuration file
- Returns:
- true if successful, false otherwise
Definition at line 109 of file kshortcutlist.cpp.
The documentation for this class was generated from the following files: