kio
KFilePreview Class Reference
#include <kfilepreview.h>
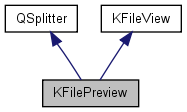
Signals | |
void | clearPreview () |
void | showPreview (const KURL &) |
Public Member Functions | |
virtual KActionCollection * | actionCollection () const |
virtual void | clear () |
virtual void | clearSelection () |
virtual void | clearView () |
virtual KFileItem * | currentFileItem () const |
void | ensureItemVisible (const KFileItem *) |
KFileView * | fileView () const |
virtual KFileItem * | firstFileItem () const |
virtual void | insertItem (KFileItem *) |
virtual void | invertSelection () |
virtual bool | isSelected (const KFileItem *) const |
KFilePreview (KFileView *view, QWidget *parent, const char *name) | |
KFilePreview (QWidget *parent, const char *name) | |
virtual void | listingCompleted () |
virtual KFileItem * | nextItem (const KFileItem *) const |
virtual KFileItem * | prevItem (const KFileItem *) const |
virtual void | readConfig (KConfig *, const QString &group=QString::null) |
virtual void | removeItem (const KFileItem *) |
virtual void | selectAll () |
virtual void | setCurrentItem (const KFileItem *) |
void | setFileView (KFileView *view) |
void | setPreviewWidget (const QWidget *w, const KURL &u) |
virtual void | setSelected (const KFileItem *, bool) |
virtual void | setSelectionMode (KFile::SelectionMode sm) |
virtual void | setSorting (QDir::SortSpec sort) |
virtual void | updateView (const KFileItem *) |
virtual void | updateView (bool) |
virtual QWidget * | widget () |
virtual void | writeConfig (KConfig *, const QString &group=QString::null) |
virtual | ~KFilePreview () |
Protected Slots | |
virtual void | slotHighlighted (const KFileItem *) |
Protected Member Functions | |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
This KFileView is an empbedded preview for some file types.Definition at line 39 of file kfilepreview.h.
Constructor & Destructor Documentation
KFilePreview::KFilePreview | ( | QWidget * | parent, | |
const char * | name | |||
) |
Definition at line 41 of file kfilepreview.cpp.
Definition at line 31 of file kfilepreview.cpp.
KFilePreview::~KFilePreview | ( | ) | [virtual] |
Definition at line 47 of file kfilepreview.cpp.
Member Function Documentation
KActionCollection * KFilePreview::actionCollection | ( | ) | const [virtual] |
This overrides KFileView::actionCollection() by returning the actionCollection() of the KFileView (member left) it contains.
This means that KFilePreview will never create a KActionCollection object of its own.
Reimplemented from KFileView.
Definition at line 257 of file kfilepreview.cpp.
void KFilePreview::clear | ( | ) | [virtual] |
Clears the view and all item lists.
Reimplemented from KFileView.
Definition at line 174 of file kfilepreview.cpp.
void KFilePreview::clearPreview | ( | ) | [signal] |
void KFilePreview::clearSelection | ( | ) | [virtual] |
Clears any selection, unhighlights everything.
Must be implemented by the view.
Implements KFileView.
Definition at line 180 of file kfilepreview.cpp.
void KFilePreview::clearView | ( | ) | [virtual] |
pure virtual function, that should be implemented to clear the view.
At this moment the list is already empty
Implements KFileView.
Definition at line 142 of file kfilepreview.cpp.
KFileItem * KFilePreview::currentFileItem | ( | ) | const [virtual] |
- Returns:
- the "current" KFileItem, e.g. where the cursor is. Returns 0L when there is no current item (e.g. in an empty view). Subclasses have to implement this.
Implements KFileView.
Definition at line 214 of file kfilepreview.cpp.
void KFilePreview::ensureItemVisible | ( | const KFileItem * | i | ) | [virtual] |
pure virtual function, that should be implemented to make item i visible, i.e.
by scrolling the view appropriately.
Implements KFileView.
Definition at line 237 of file kfilepreview.cpp.
KFileView* KFilePreview::fileView | ( | ) | const [inline] |
KFileItem * KFilePreview::firstFileItem | ( | ) | const [virtual] |
void KFilePreview::insertItem | ( | KFileItem * | i | ) | [virtual] |
The derived view must implement this function to add the file in the widget.
Make sure to call this implementation, i.e. KFileView::insertItem( i );
Reimplemented from KFileView.
Definition at line 130 of file kfilepreview.cpp.
void KFilePreview::invertSelection | ( | ) | [virtual] |
Inverts the current selection, i.e.
selects all items, that were up to now not selected and deselects the other.
Reimplemented from KFileView.
Definition at line 191 of file kfilepreview.cpp.
bool KFilePreview::isSelected | ( | const KFileItem * | ) | const [virtual] |
- Returns:
- whether the given item is currently selected. Must be implemented by the view.
Implements KFileView.
Definition at line 196 of file kfilepreview.cpp.
void KFilePreview::listingCompleted | ( | ) | [virtual] |
This hook is called when all items of the currently listed directory are listed and inserted into the view, i.e.
there won't come any new items anymore.
Reimplemented from KFileView.
Definition at line 169 of file kfilepreview.cpp.
void KFilePreview::readConfig | ( | KConfig * | config, | |
const QString & | group = QString::null | |||
) | [virtual] |
void KFilePreview::removeItem | ( | const KFileItem * | item | ) | [virtual] |
Removes an item from the list; has to be implemented by the view.
Call KFileView::removeItem( item ) after removing it.
Reimplemented from KFileView.
Definition at line 160 of file kfilepreview.cpp.
void KFilePreview::selectAll | ( | ) | [virtual] |
Selects all items.
You may want to override this, if you can implement it more efficiently than calling setSelected() with every item. This works only in Multiselection mode of course.
Reimplemented from KFileView.
Definition at line 186 of file kfilepreview.cpp.
void KFilePreview::setCurrentItem | ( | const KFileItem * | item | ) | [virtual] |
Reimplement this to set item
the current item in the view, e.g.
the item having focus.
Implements KFileView.
Definition at line 209 of file kfilepreview.cpp.
void KFilePreview::setFileView | ( | KFileView * | view | ) |
Delets the current view and sets the view to the given view
.
The view is reparented to have this as parent, if necessary.
Definition at line 81 of file kfilepreview.cpp.
void KFilePreview::setPreviewWidget | ( | const QWidget * | w, | |
const KURL & | u | |||
) |
Definition at line 108 of file kfilepreview.cpp.
void KFilePreview::setSelected | ( | const KFileItem * | , | |
bool | enable | |||
) | [virtual] |
Tells the view that it should highlight the item.
This function must be implemented by the view.
Implements KFileView.
Definition at line 205 of file kfilepreview.cpp.
void KFilePreview::setSelectionMode | ( | KFile::SelectionMode | sm | ) | [virtual] |
void KFilePreview::setSorting | ( | QDir::SortSpec | sort | ) | [virtual] |
Sets the sorting order of the view.
Default is QDir::Name | QDir::IgnoreCase | QDir::DirsFirst Override this in your subclass and sort accordingly (usually by setting the sorting-key for every item and telling QIconView or QListView to sort.
A view may choose to use a different sorting than QDir::Name, Time or Size. E.g. to sort by mimetype or any possible string. Set the sorting to QDir::Unsorted for that and do the rest internally.
- See also:
- sortingKey
Reimplemented from KFileView.
Definition at line 136 of file kfilepreview.cpp.
void KFilePreview::showPreview | ( | const KURL & | ) | [signal] |
void KFilePreview::slotHighlighted | ( | const KFileItem * | item | ) | [protected, virtual, slot] |
Definition at line 219 of file kfilepreview.cpp.
void KFilePreview::updateView | ( | const KFileItem * | i | ) | [virtual] |
void KFilePreview::updateView | ( | bool | f | ) | [virtual] |
void KFilePreview::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
For internal use only.
Reimplemented from KFileView.
Definition at line 277 of file kfilepreview.cpp.
virtual QWidget* KFilePreview::widget | ( | ) | [inline, virtual] |
a pure virtual function to get a QWidget, that can be added to other widgets.
This function is needed to make it possible for derived classes to derive from other widgets.
Implements KFileView.
Definition at line 48 of file kfilepreview.h.
void KFilePreview::writeConfig | ( | KConfig * | config, | |
const QString & | group = QString::null | |||
) | [virtual] |
The documentation for this class was generated from the following files: