kio
KFileView Class Reference
This class defines an interface to all file views. More...
#include <kfileview.h>
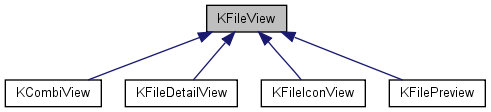
Public Types | |
enum | DropOptions { AutoOpenDirs = 1 } |
enum | ViewMode { Files = 1, Directories = 2, All = Files | Directories } |
Public Member Functions | |
virtual KActionCollection * | actionCollection () const |
void | addItemList (const KFileItemList &list) |
virtual void | clear () |
virtual void | clearSelection ()=0 |
virtual void | clearView ()=0 |
uint | count () const |
virtual KFileItem * | currentFileItem () const =0 |
int | dropOptions () |
virtual void | ensureItemVisible (const KFileItem *i)=0 |
virtual KFileItem * | firstFileItem () const =0 |
virtual void | insertItem (KFileItem *i) |
virtual void | invertSelection () |
bool | isReversed () const |
virtual bool | isSelected (const KFileItem *) const =0 |
const KFileItemList * | items () const |
KFileView () | |
virtual void | listingCompleted () |
virtual KFileItem * | nextItem (const KFileItem *) const =0 |
uint | numDirs () const |
uint | numFiles () const |
bool | onlyDoubleClickSelectsFiles () const |
virtual KFileItem * | prevItem (const KFileItem *) const =0 |
virtual void | readConfig (KConfig *, const QString &group=QString::null) |
virtual void | removeItem (const KFileItem *item) |
virtual void | selectAll () |
const KFileItemList * | selectedItems () const |
virtual KFile::SelectionMode | selectionMode () const |
virtual void | setCurrentItem (const KFileItem *item)=0 |
void | setCurrentItem (const QString &filename) |
void | setDropOptions (int options) |
void | setOnlyDoubleClickSelectsFiles (bool enable) |
virtual void | setParentView (KFileView *parent) |
virtual void | setSelected (const KFileItem *, bool enable)=0 |
virtual void | setSelectionMode (KFile::SelectionMode sm) |
virtual void | setSorting (QDir::SortSpec sort) |
virtual void | setViewMode (ViewMode vm) |
void | setViewName (const QString &name) |
KFileViewSignaler * | signaler () const |
QDir::SortSpec | sorting () const |
void | sortReversed () |
bool | updateNumbers (const KFileItem *i) |
virtual void | updateView (const KFileItem *) |
virtual void | updateView (bool f=true) |
virtual ViewMode | viewMode () const |
QString | viewName () const |
QWidget * | widget () const |
virtual QWidget * | widget ()=0 |
virtual void | writeConfig (KConfig *, const QString &group=QString::null) |
virtual | ~KFileView () |
Static Public Member Functions | |
static int | autoOpenDelay () |
static QString | sortingKey (KIO::filesize_t value, bool isDir, int sortSpec) |
static QString | sortingKey (const QString &value, bool isDir, int sortSpec) |
Protected Types | |
enum | { VIRTUAL_SET_DROP_OPTIONS = 1 } |
Protected Member Functions | |
void | setDropOptions_impl (int options) |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
KFileViewSignaler * | sig |
Detailed Description
This class defines an interface to all file views.Its intent is to allow to switch the view of the files in the selector very easily. It defines some pure virtual functions, that must be implemented to make a file view working.
Since this class is not a widget, but it's meant to be added to other widgets, its most important function is widget. This should return a pointer to the implemented widget.
A base class for views of the KDE file selector
Definition at line 98 of file kfileview.h.
Member Enumeration Documentation
anonymous enum [protected] |
Various options for drag and drop support.
These values can be or'd together.
AutoOpenDirs
Automatically open directory after hovering above it for a short while while dragging.- Since:
- 3.2
Definition at line 359 of file kfileview.h.
enum KFileView::ViewMode |
Constructor & Destructor Documentation
KFileView::KFileView | ( | ) |
Definition at line 63 of file kfileview.cpp.
KFileView::~KFileView | ( | ) | [virtual] |
Member Function Documentation
KActionCollection * KFileView::actionCollection | ( | ) | const [virtual] |
- Returns:
- the view-specific action-collection. Every view should add its actions here (if it has any) to make them available to e.g. the KDirOperator's popup-menu.
Reimplemented in KCombiView, and KFilePreview.
Definition at line 365 of file kfileview.cpp.
void KFileView::addItemList | ( | const KFileItemList & | list | ) |
int KFileView::autoOpenDelay | ( | ) | [static] |
For internal use only.
delay before auto opening a directory
Definition at line 412 of file kfileview.cpp.
void KFileView::clear | ( | ) | [virtual] |
Clears the view and all item lists.
Reimplemented in KCombiView, and KFilePreview.
Definition at line 156 of file kfileview.cpp.
virtual void KFileView::clearSelection | ( | ) | [pure virtual] |
Clears any selection, unhighlights everything.
Must be implemented by the view.
Implemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
virtual void KFileView::clearView | ( | ) | [pure virtual] |
pure virtual function, that should be implemented to clear the view.
At this moment the list is already empty
Implemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
uint KFileView::count | ( | ) | const [inline] |
virtual KFileItem* KFileView::currentFileItem | ( | ) | const [pure virtual] |
- Returns:
- the "current" KFileItem, e.g. where the cursor is. Returns 0L when there is no current item (e.g. in an empty view). Subclasses have to implement this.
Implemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
int KFileView::dropOptions | ( | ) |
Returns the DND options in effect.
See DropOptions for details.
- Since:
- 3.2
Definition at line 407 of file kfileview.cpp.
virtual void KFileView::ensureItemVisible | ( | const KFileItem * | i | ) | [pure virtual] |
pure virtual function, that should be implemented to make item i visible, i.e.
by scrolling the view appropriately.
Implemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
virtual KFileItem* KFileView::firstFileItem | ( | ) | const [pure virtual] |
Implemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
void KFileView::insertItem | ( | KFileItem * | i | ) | [virtual] |
The derived view must implement this function to add the file in the widget.
Make sure to call this implementation, i.e. KFileView::insertItem( i );
Reimplemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
Definition at line 147 of file kfileview.cpp.
void KFileView::invertSelection | ( | ) | [virtual] |
Inverts the current selection, i.e.
selects all items, that were up to now not selected and deselects the other.
Reimplemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
Definition at line 323 of file kfileview.cpp.
bool KFileView::isReversed | ( | ) | const [inline] |
Tells whether the current items are in reversed order (shortcut to sorting() & QDir::Reversed).
Definition at line 199 of file kfileview.h.
virtual bool KFileView::isSelected | ( | const KFileItem * | ) | const [pure virtual] |
- Returns:
- whether the given item is currently selected. Must be implemented by the view.
Implemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
const KFileItemList * KFileView::items | ( | ) | const |
- Returns:
- all items currently available in the current sort-order
Definition at line 283 of file kfileview.cpp.
void KFileView::listingCompleted | ( | ) | [virtual] |
This hook is called when all items of the currently listed directory are listed and inserted into the view, i.e.
there won't come any new items anymore.
Reimplemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
Definition at line 360 of file kfileview.cpp.
Implemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
uint KFileView::numDirs | ( | ) | const [inline] |
uint KFileView::numFiles | ( | ) | const [inline] |
bool KFileView::onlyDoubleClickSelectsFiles | ( | ) | const [inline] |
- Returns:
- whether files (not directories) should only be select()ed by double-clicks.
- See also:
- setOnlyDoubleClickSelectsFiles
Definition at line 330 of file kfileview.h.
Implemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
void KFileView::readConfig | ( | KConfig * | , | |
const QString & | group = QString::null | |||
) | [virtual] |
Reimplemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
Definition at line 372 of file kfileview.cpp.
void KFileView::removeItem | ( | const KFileItem * | item | ) | [virtual] |
Removes an item from the list; has to be implemented by the view.
Call KFileView::removeItem( item ) after removing it.
Reimplemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
Definition at line 346 of file kfileview.cpp.
void KFileView::selectAll | ( | ) | [virtual] |
Selects all items.
You may want to override this, if you can implement it more efficiently than calling setSelected() with every item. This works only in Multiselection mode of course.
Reimplemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
Definition at line 312 of file kfileview.cpp.
const KFileItemList * KFileView::selectedItems | ( | ) | const |
KFile::SelectionMode KFileView::selectionMode | ( | ) | const [virtual] |
Definition at line 336 of file kfileview.cpp.
virtual void KFileView::setCurrentItem | ( | const KFileItem * | item | ) | [pure virtual] |
Reimplement this to set item
the current item in the view, e.g.
the item having focus.
Implemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
void KFileView::setCurrentItem | ( | const QString & | filename | ) |
Sets filename
the current item in the view, if available.
Definition at line 268 of file kfileview.cpp.
void KFileView::setDropOptions | ( | int | options | ) |
Specify DND options.
See DropOptions for details. All options are disabled by default.
- Since:
- 3.2
Definition at line 397 of file kfileview.cpp.
void KFileView::setDropOptions_impl | ( | int | options | ) | [protected] |
void KFileView::setOnlyDoubleClickSelectsFiles | ( | bool | enable | ) | [inline] |
This is a KFileDialog specific hack: we want to select directories with single click, but not files.
But as a generic class, we have to be able to select files on single click as well.
This gives us the opportunity to do both.
Every view has to decide when to call select( item ) when a file was single-clicked, based on onlyDoubleClickSelectsFiles().
Definition at line 321 of file kfileview.h.
void KFileView::setParentView | ( | KFileView * | parent | ) | [virtual] |
Definition at line 90 of file kfileview.cpp.
virtual void KFileView::setSelected | ( | const KFileItem * | , | |
bool | enable | |||
) | [pure virtual] |
Tells the view that it should highlight the item.
This function must be implemented by the view.
Implemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
void KFileView::setSelectionMode | ( | KFile::SelectionMode | sm | ) | [virtual] |
Reimplemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
Definition at line 331 of file kfileview.cpp.
void KFileView::setSorting | ( | QDir::SortSpec | sort | ) | [virtual] |
Sets the sorting order of the view.
Default is QDir::Name | QDir::IgnoreCase | QDir::DirsFirst Override this in your subclass and sort accordingly (usually by setting the sorting-key for every item and telling QIconView or QListView to sort.
A view may choose to use a different sorting than QDir::Name, Time or Size. E.g. to sort by mimetype or any possible string. Set the sorting to QDir::Unsorted for that and do the rest internally.
- See also:
- sortingKey
Reimplemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
Definition at line 151 of file kfileview.cpp.
void KFileView::setViewMode | ( | ViewMode | vm | ) | [virtual] |
Definition at line 341 of file kfileview.cpp.
void KFileView::setViewName | ( | const QString & | name | ) | [inline] |
Sets the name of the view, which could be displayed somewhere.
E.g. "Image Preview".
Definition at line 240 of file kfileview.h.
KFileViewSignaler* KFileView::signaler | ( | ) | const [inline] |
Definition at line 347 of file kfileview.h.
QDir::SortSpec KFileView::sorting | ( | ) | const [inline] |
Returns the sorting order of the internal list.
Newly added files are added through this sorting.
Definition at line 177 of file kfileview.h.
QString KFileView::sortingKey | ( | KIO::filesize_t | value, | |
bool | isDir, | |||
int | sortSpec | |||
) | [static] |
An overloaded method that takes not a QString, but a number as sort criterion.
You can use this for file-sizes or dates/times for example. If you use a time_t, you need to cast that to KIO::filesize_t because of ambiguity problems.
Definition at line 389 of file kfileview.cpp.
This method calculates a QString from the given parameters, that is suitable for sorting with e.g.
QIconView or QListView. Their Item-classes usually have a setKey( const QString& ) method or a virtual method QString key() that is used for sorting.
- Parameters:
-
value Any string that should be used as sort criterion isDir Tells whether the key is computed for an item representing a directory (directories are usually sorted before files) sortSpec An ORed combination of QDir::SortSpec flags. Currently, the values IgnoreCase, Reversed and DirsFirst are taken into account.
Definition at line 380 of file kfileview.cpp.
void KFileView::sortReversed | ( | ) |
Definition at line 164 of file kfileview.cpp.
bool KFileView::updateNumbers | ( | const KFileItem * | i | ) |
increases the number of dirs and files.
- Returns:
- true if the item fits the view mode
Definition at line 110 of file kfileview.cpp.
void KFileView::updateView | ( | const KFileItem * | ) | [virtual] |
Reimplemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
Definition at line 264 of file kfileview.cpp.
void KFileView::updateView | ( | bool | f = true |
) | [virtual] |
does a repaint of the view.
The default implementation calls
Reimplemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
Definition at line 259 of file kfileview.cpp.
virtual ViewMode KFileView::viewMode | ( | ) | const [inline, virtual] |
Definition at line 227 of file kfileview.h.
QString KFileView::viewName | ( | ) | const [inline] |
- Returns:
- the localized name of the view, which could be displayed somewhere, e.g. in a menu, where the user can choose between views.
- See also:
- setViewName
Definition at line 234 of file kfileview.h.
void KFileView::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Reimplemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
Definition at line 417 of file kfileview.cpp.
QWidget* KFileView::widget | ( | ) | const [inline] |
virtual QWidget* KFileView::widget | ( | ) | [pure virtual] |
a pure virtual function to get a QWidget, that can be added to other widgets.
This function is needed to make it possible for derived classes to derive from other widgets.
Implemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
void KFileView::writeConfig | ( | KConfig * | , | |
const QString & | group = QString::null | |||
) | [virtual] |
Reimplemented in KCombiView, KFileDetailView, KFileIconView, and KFilePreview.
Definition at line 376 of file kfileview.cpp.
Member Data Documentation
KFileViewSignaler* KFileView::sig [protected] |
The documentation for this class was generated from the following files: