kio
KFileDialog Class Reference
Provides a user (and developer) friendly way to select files and directories. More...
#include <kfiledialog.h>
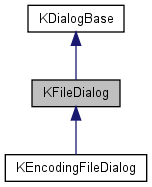
Public Types | |
enum | OperationMode { Other = 0, Opening, Saving } |
Signals | |
void | fileHighlighted (const QString &) |
void | fileSelected (const QString &) |
void | filterChanged (const QString &filter) |
void | selectionChanged () |
Public Member Functions | |
KActionCollection * | actionCollection () const |
KURL | baseURL () const |
KPushButton * | cancelButton () const |
void | clearFilter () |
QString | currentFilter () const |
KMimeType::Ptr | currentFilterMimeType () |
QString | currentMimeFilter () const |
bool | keepsLocation () const |
KFileDialog (const QString &startDir, const QString &filter, QWidget *parent, const char *name, bool modal, QWidget *widget) | |
KFileDialog (const QString &startDir, const QString &filter, QWidget *parent, const char *name, bool modal) | |
KFile::Mode | mode () const |
KPushButton * | okButton () const |
OperationMode | operationMode () const |
int | pathComboIndex () |
QString | selectedFile () const |
QStringList | selectedFiles () const |
KURL | selectedURL () const |
KURL::List | selectedURLs () const |
void | setFilter (const QString &filter) |
void | setFilterMimeType (const QString &label, const KMimeType::List &types, const KMimeType::Ptr &defaultType) KDE_DEPRECATED |
void | setKeepLocation (bool keep) |
void | setLocationLabel (const QString &text) |
void | setMimeFilter (const QStringList &types, const QString &defaultType=QString::null) |
void | setMode (unsigned int m) |
void | setMode (KFile::Mode m) |
void | setOperationMode (KFileDialog::OperationMode) |
void | setPreviewWidget (const KPreviewWidgetBase *w) |
void | setPreviewWidget (const QWidget *w) KDE_DEPRECATED |
void | setSelection (const QString &name) |
void | setURL (const KURL &url, bool clearforward=true) |
virtual void | show () |
KURLBar * | speedBar () |
KToolBar * | toolBar () const |
~KFileDialog () | |
Static Public Member Functions | |
static QString | getExistingDirectory (const QString &startDir=QString::null, QWidget *parent=0, const QString &caption=QString::null) |
static KURL | getExistingURL (const QString &startDir=QString::null, QWidget *parent=0, const QString &caption=QString::null) |
static KURL | getImageOpenURL (const QString &startDir=QString::null, QWidget *parent=0, const QString &caption=QString::null) |
static QString | getOpenFileName (const QString &startDir=QString::null, const QString &filter=QString::null, QWidget *parent=0, const QString &caption=QString::null) |
static QStringList | getOpenFileNames (const QString &startDir=QString::null, const QString &filter=QString::null, QWidget *parent=0, const QString &caption=QString::null) |
static QString | getOpenFileNameWId (const QString &startDir, const QString &filter, WId parent_id, const QString &caption) |
static KURL | getOpenURL (const QString &startDir=QString::null, const QString &filter=QString::null, QWidget *parent=0, const QString &caption=QString::null) |
static KURL::List | getOpenURLs (const QString &startDir=QString::null, const QString &filter=QString::null, QWidget *parent=0, const QString &caption=QString::null) |
static QString | getSaveFileName (const QString &startDir=QString::null, const QString &filter=QString::null, QWidget *parent=0, const QString &caption=QString::null) |
static QString | getSaveFileNameWId (const QString &dir, const QString &filter, WId parent_id, const QString &caption) |
static KURL | getSaveURL (const QString &startDir=QString::null, const QString &filter=QString::null, QWidget *parent=0, const QString &caption=QString::null) |
static KURL | getStartURL (const QString &startDir, QString &recentDirClass) |
static void | setStartDir (const KURL &directory) |
Protected Slots | |
virtual void | accept () |
void | addToRecentDocuments () |
void | dirCompletion (const QString &) |
void | enterURL (const QString &url) |
void | enterURL (const KURL &url) |
void | fileCompletion (const QString &) |
void | fileHighlighted (const KFileItem *i) |
void | fileSelected (const KFileItem *i) |
void | initSpeedbar () |
void | locationActivated (const QString &url) |
void | pathComboChanged (const QString &) |
void | slotAutoSelectExtClicked () |
virtual void | slotCancel () |
void | slotFilterChanged () |
void | slotLoadingFinished () |
virtual void | slotOk () |
void | slotStatResult (KIO::Job *job) |
void | toggleBookmarks (bool show) |
void | toggleSpeedbar (bool) |
void | toolbarCallback (int) |
virtual void | updateStatusLine (int dirs, int files) |
void | urlEntered (const KURL &) |
Protected Member Functions | |
QString | currentFilterExtension () |
KURL | getCompleteURL (const QString &) |
void | init (const QString &startDir, const QString &filter, QWidget *widget) |
virtual void | initGUI () |
virtual void | keyPressEvent (QKeyEvent *e) |
void | multiSelectionChanged () |
KURL::List & | parseSelectedURLs () const |
virtual void | readConfig (KConfig *, const QString &group=QString::null) |
virtual void | readRecentFiles (KConfig *) |
virtual void | saveRecentFiles (KConfig *) |
KURL::List | tokenize (const QString &line) const |
void | updateAutoSelectExtension () |
virtual void | virtual_hook (int id, void *data) |
virtual void | writeConfig (KConfig *, const QString &group=QString::null) |
Protected Attributes | |
bool | autoDirectoryFollowing |
KFileFilterCombo * | filterWidget |
KURLComboBox * | locationEdit |
KDirOperator * | ops |
KToolBar * | toolbar |
Static Protected Attributes | |
static KURL * | lastDirectory |
Detailed Description
Provides a user (and developer) friendly way to select files and directories.The widget can be used as a drop in replacement for the QFileDialog widget, but has greater functionality and a nicer GUI.
You will usually want to use one of the static methods getOpenFileName(), getSaveFileName(), getOpenURL() or for multiple files getOpenFileNames() or getOpenURLs().
The dialog has been designed to allow applications to customise it by subclassing. It uses geometry management to ensure that subclasses can easily add children that will be incorporated into the layout.
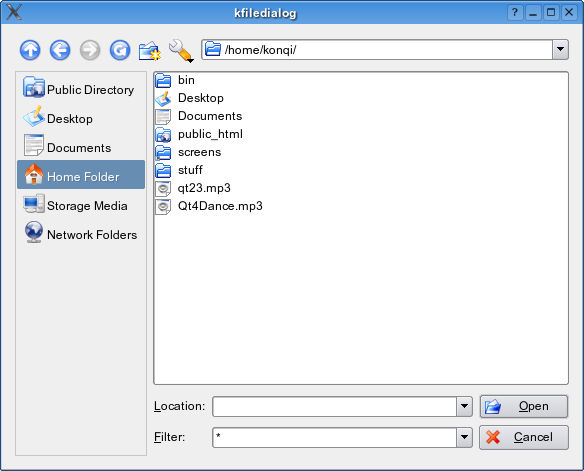
KDE File Dialog
Definition at line 77 of file kfiledialog.h.
Member Enumeration Documentation
Defines some default behavior of the filedialog.
E.g. in mode Opening
and Saving
, the selected files/urls will be added to the "recent documents" list. The Saving mode also implies setKeepLocation() being set.
Other
means that no default actions are performed.
- See also:
- setOperationMode
Definition at line 94 of file kfiledialog.h.
Constructor & Destructor Documentation
KFileDialog::KFileDialog | ( | const QString & | startDir, | |
const QString & | filter, | |||
QWidget * | parent, | |||
const char * | name, | |||
bool | modal | |||
) |
Constructs a file dialog.
- Parameters:
-
startDir This can either be - The URL of the directory to start in.
- QString::null to start in the current working directory, or the last directory where a file has been selected.
- ':<keyword>' to start in the directory last used by a filedialog in the same application that specified the same keyword.
- '::<keyword>' to start in the directory last used by a filedialog in any application that specified the same keyword.
filter A shell glob or a mime-type-filter that specifies which files to display. parent The parent widget of this dialog name The name of this object modal Whether to create a modal dialog or not See setFilter() for details on how to use this argument.
Definition at line 169 of file kfiledialog.cpp.
KFileDialog::KFileDialog | ( | const QString & | startDir, | |
const QString & | filter, | |||
QWidget * | parent, | |||
const char * | name, | |||
bool | modal, | |||
QWidget * | widget | |||
) |
Constructs a file dialog.
The parameters here are identical to the first constructor except for the addition of a QWidget parameter.
Historical note: The original version of KFileDialog did not have this extra parameter. It was added later, and, in order to maintain binary compatibility, it was placed in a new constructor instead of added to the original one.
- Parameters:
-
startDir This can either be - The URL of the directory to start in.
- QString::null to start in the current working directory, or the last directory where a file has been selected.
- ':<keyword>' to start in the directory last used by a filedialog in the same application that specified the same keyword.
- '::<keyword>' to start in the directory last used by a filedialog in any application that specified the same keyword.
filter A shell glob or a mime-type-filter that specifies which files to display. See setFilter() for details on how to use this argument. widget A widget, or a widget of widgets, for displaying custom data in the dialog. This can be used, for example, to display a check box with the caption "Open as read-only". When creating this widget, you don't need to specify a parent, since the widget's parent will be set automatically by KFileDialog. parent The parent widget of this dialog name The name of this object modal Whether to create a modal dialog or not
- Since:
- 3.1
Definition at line 176 of file kfiledialog.cpp.
KFileDialog::~KFileDialog | ( | ) |
Member Function Documentation
void KFileDialog::accept | ( | ) | [protected, virtual, slot] |
Definition at line 645 of file kfiledialog.cpp.
KActionCollection * KFileDialog::actionCollection | ( | ) | const |
- Returns:
- a pointer to the action collection, holding all the used KActions.
Definition at line 2197 of file kfiledialog.cpp.
void KFileDialog::addToRecentDocuments | ( | ) | [protected, slot] |
Definition at line 2176 of file kfiledialog.cpp.
KURL KFileDialog::baseURL | ( | ) | const |
KPushButton * KFileDialog::cancelButton | ( | ) | const |
- Returns:
- a pointer to the Cancel-Button in the filedialog. You may use it e.g. to set a custom text to it.
Definition at line 1796 of file kfiledialog.cpp.
void KFileDialog::clearFilter | ( | ) |
Clears any mime- or namefilter.
Does not reload the directory.
Definition at line 271 of file kfiledialog.cpp.
QString KFileDialog::currentFilter | ( | ) | const |
Returns the current filter as entered by the user or one of the predefined set via setFilter().
- See also:
- setFilter()
Definition at line 234 of file kfiledialog.cpp.
QString KFileDialog::currentFilterExtension | ( | void | ) | [protected] |
Returns the filename extension associated with the currentFilter().
QString::null is returned if an extension is not available or if operationMode() != Saving.
- Since:
- 3.2
Definition at line 1903 of file kfiledialog.cpp.
KMimeType::Ptr KFileDialog::currentFilterMimeType | ( | ) |
Returns the mimetype for the desired output format.
This is only valid if setFilterMimeType() has been called previously.
- See also:
- setFilterMimeType()
Definition at line 293 of file kfiledialog.cpp.
QString KFileDialog::currentMimeFilter | ( | ) | const |
The mimetype for the desired output format.
This is only valid if setMimeFilter() has been called previously.
- See also:
- setMimeFilter()
Definition at line 282 of file kfiledialog.cpp.
void KFileDialog::dirCompletion | ( | const QString & | ) | [protected, slot] |
void KFileDialog::enterURL | ( | const QString & | url | ) | [protected, slot] |
Definition at line 1199 of file kfiledialog.cpp.
void KFileDialog::enterURL | ( | const KURL & | url | ) | [protected, slot] |
Definition at line 1194 of file kfiledialog.cpp.
void KFileDialog::fileCompletion | ( | const QString & | match | ) | [protected, slot] |
Definition at line 1291 of file kfiledialog.cpp.
void KFileDialog::fileHighlighted | ( | const KFileItem * | i | ) | [protected, slot] |
Definition at line 695 of file kfiledialog.cpp.
void KFileDialog::fileHighlighted | ( | const QString & | ) | [signal] |
Emitted when the user highlights a file.
void KFileDialog::fileSelected | ( | const KFileItem * | i | ) | [protected, slot] |
Definition at line 719 of file kfiledialog.cpp.
void KFileDialog::fileSelected | ( | const QString & | ) | [signal] |
Emitted when the user selects a file.
It is only emitted in single- selection mode. The best way to get notified about selected file(s) is to connect to the okClicked() signal inherited from KDialogBase and call selectedFile(), selectedFiles(), selectedURL() or selectedURLs().
void KFileDialog::filterChanged | ( | const QString & | filter | ) | [signal] |
Emitted when the filter changed, i.e.
the user entered an own filter or chose one of the predefined set via setFilter().
- Parameters:
-
filter contains the new filter (only the extension part, not the explanation), i.e. "*.cpp" or "*.cpp *.cc".
- See also:
- setFilter()
KURL KFileDialog::getCompleteURL | ( | const QString & | _url | ) | [protected] |
Returns the absolute version of the URL specified in locationEdit.
- Since:
- 3.2
Definition at line 310 of file kfiledialog.cpp.
QString KFileDialog::getExistingDirectory | ( | const QString & | startDir = QString::null , |
|
QWidget * | parent = 0 , |
|||
const QString & | caption = QString::null | |||
) | [static] |
Creates a modal file dialog and returns the selected directory or an empty string if none was chosen.
- Parameters:
-
startDir This can either be - The URL of the directory to start in.
- QString::null to start in the current working directory, or the last directory where a file has been selected.
- ':<keyword>' to start in the directory last used by a filedialog in the same application that specified the same keyword.
- '::<keyword>' to start in the directory last used by a filedialog in any application that specified the same keyword.
parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
Definition at line 1405 of file kfiledialog.cpp.
KURL KFileDialog::getExistingURL | ( | const QString & | startDir = QString::null , |
|
QWidget * | parent = 0 , |
|||
const QString & | caption = QString::null | |||
) | [static] |
Creates a modal file dialog and returns the selected directory or an empty string if none was chosen.
Contrary to getExistingDirectory(), this method allows the selection of a remote directory.
- Parameters:
-
startDir This can either be - The URL of the directory to start in.
- QString::null to start in the current working directory, or the last directory where a file has been selected.
- ':<keyword>' to start in the directory last used by a filedialog in the same application that specified the same keyword.
- '::<keyword>' to start in the directory last used by a filedialog in any application that specified the same keyword.
parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
- Since:
- 3.1
Definition at line 1398 of file kfiledialog.cpp.
KURL KFileDialog::getImageOpenURL | ( | const QString & | startDir = QString::null , |
|
QWidget * | parent = 0 , |
|||
const QString & | caption = QString::null | |||
) | [static] |
Creates a modal file dialog with an image previewer and returns the selected url or an empty string if none was chosen.
- Parameters:
-
startDir This can either be - The URL of the directory to start in.
- QString::null to start in the current working directory, or the last directory where a file has been selected.
- ':<keyword>' to start in the directory last used by a filedialog in the same application that specified the same keyword.
- '::<keyword>' to start in the directory last used by a filedialog in any application that specified the same keyword.
parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
Definition at line 1422 of file kfiledialog.cpp.
QString KFileDialog::getOpenFileName | ( | const QString & | startDir = QString::null , |
|
const QString & | filter = QString::null , |
|||
QWidget * | parent = 0 , |
|||
const QString & | caption = QString::null | |||
) | [static] |
Creates a modal file dialog and return the selected filename or an empty string if none was chosen.
Note that with this method the user must select an existing filename.
- Parameters:
-
startDir This can either be - The URL of the directory to start in.
- QString::null to start in the current working directory, or the last directory where a file has been selected.
- ':<keyword>' to start in the directory last used by a filedialog in the same application that specified the same keyword.
- '::<keyword>' to start in the directory last used by a filedialog in any application that specified the same keyword.
filter This is a space separated list of shell globs. You can set the text to be displayed for the glob, and provide multiple globs. See setFilter() for details on how to do this... parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
Definition at line 1312 of file kfiledialog.cpp.
QStringList KFileDialog::getOpenFileNames | ( | const QString & | startDir = QString::null , |
|
const QString & | filter = QString::null , |
|||
QWidget * | parent = 0 , |
|||
const QString & | caption = QString::null | |||
) | [static] |
Creates a modal file dialog and returns the selected filenames or an empty list if none was chosen.
Note that with this method the user must select an existing filename.
- Parameters:
-
startDir This can either be - The URL of the directory to start in.
- QString::null to start in the current working directory, or the last directory where a file has been selected.
- ':<keyword>' to start in the directory last used by a filedialog in the same application that specified the same keyword.
- '::<keyword>' to start in the directory last used by a filedialog in any application that specified the same keyword.
filter This is a space separated list of shell globs. You can set the text to be displayed for the glob, and provide multiple globs. See setFilter() for details on how to do this... parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
Definition at line 1352 of file kfiledialog.cpp.
QString KFileDialog::getOpenFileNameWId | ( | const QString & | startDir, | |
const QString & | filter, | |||
WId | parent_id, | |||
const QString & | caption | |||
) | [static] |
Use this version only if you have no QWidget available as parent widget.
This can be the case if the parent widget is a widget in another process or if the parent widget is a non-Qt widget. For example, in a GTK program.
- Since:
- 3.4
Definition at line 1328 of file kfiledialog.cpp.
KURL KFileDialog::getOpenURL | ( | const QString & | startDir = QString::null , |
|
const QString & | filter = QString::null , |
|||
QWidget * | parent = 0 , |
|||
const QString & | caption = QString::null | |||
) | [static] |
Creates a modal file dialog and returns the selected URL or an empty string if none was chosen.
Note that with this method the user must select an existing URL.
- Parameters:
-
startDir This can either be - The URL of the directory to start in.
- QString::null to start in the current working directory, or the last directory where a file has been selected.
- ':<keyword>' to start in the directory last used by a filedialog in the same application that specified the same keyword.
- '::<keyword>' to start in the directory last used by a filedialog in any application that specified the same keyword.
filter This is a space separated list of shell globs. You can set the text to be displayed for the glob, and provide multiple globs. See setFilter() for details on how to do this... parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
Definition at line 1368 of file kfiledialog.cpp.
KURL::List KFileDialog::getOpenURLs | ( | const QString & | startDir = QString::null , |
|
const QString & | filter = QString::null , |
|||
QWidget * | parent = 0 , |
|||
const QString & | caption = QString::null | |||
) | [static] |
Creates a modal file dialog and returns the selected URLs or an empty list if none was chosen.
Note that with this method the user must select an existing filename.
- Parameters:
-
startDir This can either be - The URL of the directory to start in.
- QString::null to start in the current working directory, or the last directory where a file has been selected.
- ':<keyword>' to start in the directory last used by a filedialog in the same application that specified the same keyword.
- '::<keyword>' to start in the directory last used by a filedialog in any application that specified the same keyword.
filter This is a space separated list of shell globs. You can set the text to be displayed for the glob, and provide multiple globs. See setFilter() for details on how to do this... parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
Definition at line 1382 of file kfiledialog.cpp.
QString KFileDialog::getSaveFileName | ( | const QString & | startDir = QString::null , |
|
const QString & | filter = QString::null , |
|||
QWidget * | parent = 0 , |
|||
const QString & | caption = QString::null | |||
) | [static] |
Creates a modal file dialog and returns the selected filename or an empty string if none was chosen.
Note that with this method the user need not select an existing filename.
- Parameters:
-
startDir This can either be - The URL of the directory to start in.
- a relative path or a filename determining the directory to start in and the file to be selected.
- QString::null to start in the current working directory, or the last directory where a file has been selected.
- ':<keyword>' to start in the directory last used by a filedialog in the same application that specified the same keyword.
- '::<keyword>' to start in the directory last used by a filedialog in any application that specified the same keyword.
filter This is a space separated list of shell globs. You can set the text to be displayed for the glob, and provide multiple globs. See setFilter() for details on how to do this... parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
Definition at line 1588 of file kfiledialog.cpp.
QString KFileDialog::getSaveFileNameWId | ( | const QString & | dir, | |
const QString & | filter, | |||
WId | parent_id, | |||
const QString & | caption | |||
) | [static] |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
- Since:
- 3.4
Definition at line 1609 of file kfiledialog.cpp.
KURL KFileDialog::getSaveURL | ( | const QString & | startDir = QString::null , |
|
const QString & | filter = QString::null , |
|||
QWidget * | parent = 0 , |
|||
const QString & | caption = QString::null | |||
) | [static] |
Creates a modal file dialog and returns the selected filename or an empty string if none was chosen.
Note that with this method the user need not select an existing filename.
- Parameters:
-
startDir This can either be - The URL of the directory to start in.
- a relative path or a filename determining the directory to start in and the file to be selected.
- QString::null to start in the current working directory, or the last directory where a file has been selected.
- ':<keyword>' to start in the directory last used by a filedialog in the same application that specified the same keyword.
- '::<keyword>' to start in the directory last used by a filedialog in any application that specified the same keyword.
filter This is a space separated list of shell globs. You can set the text to be displayed for the glob, and provide multiple globs. See setFilter() for details on how to do this... parent The widget the dialog will be centered on initially. caption The name of the dialog widget.
Definition at line 1638 of file kfiledialog.cpp.
This method implements the logic to determine the user's default directory to be listed.
E.g. the documents direcory, home directory or a recently used directory.
- Parameters:
-
startDir A url/directory, to be used. May use the ':' and '::' syntax as documented in the KFileDialog() constructor. recentDirClass If the ':' or '::' syntax is used, recentDirClass will contain the string to be used later for KRecentDir::dir()
- Returns:
- The URL that should be listed by default (e.g. by KFileDialog or KDirSelectDialog).
- Since:
- 3.1
Definition at line 2299 of file kfiledialog.cpp.
void KFileDialog::init | ( | const QString & | startDir, | |
const QString & | filter, | |||
QWidget * | widget | |||
) | [protected] |
Perform basic initialization tasks.
Called by constructors.
- Since:
- 3.1
Definition at line 811 of file kfiledialog.cpp.
void KFileDialog::initGUI | ( | ) | [protected, virtual] |
void KFileDialog::initSpeedbar | ( | ) | [protected, slot] |
Definition at line 1049 of file kfiledialog.cpp.
bool KFileDialog::keepsLocation | ( | ) | const |
- Returns:
- whether the contents of the location edit are kept when changing directories.
Definition at line 1822 of file kfiledialog.cpp.
void KFileDialog::keyPressEvent | ( | QKeyEvent * | e | ) | [protected, virtual] |
void KFileDialog::locationActivated | ( | const QString & | url | ) | [protected, slot] |
Definition at line 1183 of file kfiledialog.cpp.
KFile::Mode KFileDialog::mode | ( | ) | const |
Returns the mode of the filedialog.
- See also:
- setMode()
Definition at line 1687 of file kfiledialog.cpp.
void KFileDialog::multiSelectionChanged | ( | ) | [protected] |
called when an item is highlighted/selected in multiselection mode.
handles setting the locationEdit.
Definition at line 741 of file kfiledialog.cpp.
KPushButton * KFileDialog::okButton | ( | ) | const |
- Returns:
- a pointer to the OK-Button in the filedialog. You may use it e.g. to set a custom text to it.
Definition at line 1791 of file kfiledialog.cpp.
KFileDialog::OperationMode KFileDialog::operationMode | ( | ) | const |
- Returns:
- the current operation mode, Opening, Saving or Other. Default is Other.
- See also:
- operationMode
Definition at line 1844 of file kfiledialog.cpp.
KURL::List & KFileDialog::parseSelectedURLs | ( | ) | const [protected] |
Definition at line 1461 of file kfiledialog.cpp.
void KFileDialog::pathComboChanged | ( | const QString & | ) | [protected, slot] |
int KFileDialog::pathComboIndex | ( | ) |
- Returns:
- the index of the path combobox so when inserting widgets into the dialog (e.g. subclasses) they can do so without hardcoding in an index
Definition at line 2284 of file kfiledialog.cpp.
void KFileDialog::readConfig | ( | KConfig * | kc, | |
const QString & | group = QString::null | |||
) | [protected, virtual] |
Reads configuration and applies it (size, recent directories, .
..)
Definition at line 1693 of file kfiledialog.cpp.
void KFileDialog::readRecentFiles | ( | KConfig * | kc | ) | [protected, virtual] |
Reads the recent used files and inserts them into the location combobox.
Definition at line 1766 of file kfiledialog.cpp.
void KFileDialog::saveRecentFiles | ( | KConfig * | kc | ) | [protected, virtual] |
QString KFileDialog::selectedFile | ( | ) | const |
Returns the full path of the selected file in the local filesystem.
(Local files only)
Definition at line 1541 of file kfiledialog.cpp.
QStringList KFileDialog::selectedFiles | ( | ) | const |
KURL KFileDialog::selectedURL | ( | ) | const |
KURL::List KFileDialog::selectedURLs | ( | ) | const |
void KFileDialog::selectionChanged | ( | ) | [signal] |
Emitted when the user hilights one or more files in multiselection mode.
Note: fileHighlighted() or fileSelected() are not emitted in multiselection mode. You may use selectedItems() to ask for the current highlighted items.
- See also:
- fileSelected
void KFileDialog::setFilter | ( | const QString & | filter | ) |
Sets the filter to be used to filter
.
You can set more filters for the user to select separated by '
'. Every filter entry is defined through namefilter|text to diplay. If no | is found in the expression, just the namefilter is shown. Examples:
kfile->setFilter("*.cpp|C++ Source Files\n*.h|Header files"); kfile->setFilter("*.cpp"); kfile->setFilter("*.cpp|Sources (*.cpp)"); kfile->setFilter("*.cpp|" + i18n("Sources (*.cpp)")); kfile->setFilter("*.cpp *.cc *.C|C++ Source Files\n*.h *.H|Header files");
Note: The text to display is not parsed in any way. So, if you want to show the suffix to select by a specific filter, you must repeat it.
If the filter contains an unescaped '/', a mimetype-filter is assumed. If you would like a '/' visible in your filter it can be escaped with a '\'. You can specify multiple mimetypes like this (separated with space):
kfile->setFilter( "image/png text/html text/plain" ); kfile->setFilter( "*.cue|CUE\\/BIN Files (*.cue)" );
- See also:
- filterChanged
Definition at line 205 of file kfiledialog.cpp.
void KFileDialog::setFilterMimeType | ( | const QString & | label, | |
const KMimeType::List & | types, | |||
const KMimeType::Ptr & | defaultType | |||
) |
Sets the filter up to specify the output type.
- Parameters:
-
label the label to use instead of "Filter:" types a list of mimetypes that can be used as output format defaultType the default mimetype to use as output format.
Definition at line 240 of file kfiledialog.cpp.
void KFileDialog::setKeepLocation | ( | bool | keep | ) |
Sets whether the filename/url should be kept when changing directories.
This is for example useful when having a predefined filename where the full path for that file is searched.
This is implicitly set when operationMode() is KFileDialog::Saving
getSaveFileName() and getSaveURL() set this to true by default, so that you can type in the filename and change the directory without having to type the name again.
Definition at line 1817 of file kfiledialog.cpp.
void KFileDialog::setLocationLabel | ( | const QString & | text | ) |
Sets the text to be displayed in front of the selection.
The default is "Location". Most useful if you want to make clear what the location is used for.
Definition at line 200 of file kfiledialog.cpp.
void KFileDialog::setMimeFilter | ( | const QStringList & | types, | |
const QString & | defaultType = QString::null | |||
) |
Sets the filter up to specify the output type.
- Parameters:
-
types a list of mimetypes that can be used as output format defaultType the default mimetype to use as output format, if any. If defaultType
is set, it will be set as the current item. Otherwise, a first item showing all the mimetypes will be created. Typically,defaultType
should be empty for loading and set for saving.
Definition at line 254 of file kfiledialog.cpp.
void KFileDialog::setMode | ( | unsigned int | m | ) |
Sets the mode of the dialog.
The mode is defined as (in kfile.h):
enum Mode {
File = 1,
Directory = 2,
Files = 4,
ExistingOnly = 8,
LocalOnly = 16
};
KFile::Mode mode = static_cast<KFile::Mode>( KFile::Files | KFile::ExistingOnly | KFile::LocalOnly ); setMode( mode );
Definition at line 1682 of file kfiledialog.cpp.
void KFileDialog::setMode | ( | KFile::Mode | m | ) |
Convenient overload of the other setMode(unsigned int) method.
Definition at line 1669 of file kfiledialog.cpp.
void KFileDialog::setOperationMode | ( | KFileDialog::OperationMode | mode | ) |
Sets the operational mode of the filedialog to Saving
, Opening
or Other
.
This will set some flags that are specific to loading or saving files. E.g. setKeepLocation() makes mostly sense for a save-as dialog. So setOperationMode( KFileDialog::Saving ); sets setKeepLocation for example.
The mode Saving
, together with a default filter set via setMimeFilter() will make the filter combobox read-only.
The default mode is Opening
.
Call this method right after instantiating KFileDialog.
- See also:
- operationMode
Definition at line 1827 of file kfiledialog.cpp.
void KFileDialog::setPreviewWidget | ( | const KPreviewWidgetBase * | w | ) |
Adds a preview widget and enters the preview mode.
In this mode the dialog is split and the right part contains your preview widget.
Ownership is transferred to KFileDialog. You need to create the preview-widget with "new", i.e. on the heap.
- Parameters:
-
w The widget to be used for the preview.
Definition at line 304 of file kfiledialog.cpp.
void KFileDialog::setPreviewWidget | ( | const QWidget * | w | ) |
- Deprecated:
- Add a preview widget and enter the preview mode.
Ownership is transferred to KFileDialog. You need to create the preview-widget with "new", i.e. on the heap.
Definition at line 298 of file kfiledialog.cpp.
void KFileDialog::setSelection | ( | const QString & | name | ) |
Sets the file name to preselect to name
.
This takes absolute URLs and relative file names.
Definition at line 1213 of file kfiledialog.cpp.
void KFileDialog::setStartDir | ( | const KURL & | directory | ) | [static] |
For internal use only.
Used by KDirSelectDialog to share the dialog's start directory.
Definition at line 2345 of file kfiledialog.cpp.
void KFileDialog::setURL | ( | const KURL & | url, | |
bool | clearforward = true | |||
) |
Sets the directory to view.
- Parameters:
-
url URL to show. clearforward Indicates whether the forward queue should be cleared.
Definition at line 1152 of file kfiledialog.cpp.
void KFileDialog::show | ( | ) | [virtual] |
Definition at line 1658 of file kfiledialog.cpp.
void KFileDialog::slotAutoSelectExtClicked | ( | ) | [protected, slot] |
Definition at line 1849 of file kfiledialog.cpp.
void KFileDialog::slotCancel | ( | ) | [protected, virtual, slot] |
Definition at line 1806 of file kfiledialog.cpp.
void KFileDialog::slotFilterChanged | ( | ) | [protected, slot] |
Definition at line 1131 of file kfiledialog.cpp.
void KFileDialog::slotLoadingFinished | ( | ) | [protected, slot] |
Definition at line 1278 of file kfiledialog.cpp.
void KFileDialog::slotOk | ( | ) | [protected, virtual, slot] |
Definition at line 333 of file kfiledialog.cpp.
void KFileDialog::slotStatResult | ( | KIO::Job * | job | ) | [protected, slot] |
Definition at line 595 of file kfiledialog.cpp.
KURLBar * KFileDialog::speedBar | ( | ) |
- Returns:
- the KURLBar object used as the "speed bar". You can add custom entries to it like that:
KURLBar *urlBar = fileDialog->speedBar(); if ( urlBar ) urlBar->insertDynamicItem( someURL, i18n("The URL's description") );
- See also:
- KURLBar
- Since:
- 3.2
Definition at line 1801 of file kfiledialog.cpp.
void KFileDialog::toggleBookmarks | ( | bool | show | ) | [protected, slot] |
void KFileDialog::toggleSpeedbar | ( | bool | show | ) | [protected, slot] |
KURL::List KFileDialog::tokenize | ( | const QString & | line | ) | const [protected] |
Parses the string "line" for files.
If line doesn't contain any ", the whole line will be interpreted as one file. If the number of " is odd, an empty list will be returned. Otherwise, all items enclosed in " " will be returned as correct urls.
Definition at line 1495 of file kfiledialog.cpp.
KToolBar* KFileDialog::toolBar | ( | ) | const [inline] |
Returns a pointer to the toolbar.
You can use this to insert custom items into it, e.g.:
yourAction = new KAction( i18n("Your Action"), 0, this, SLOT( yourSlot() ), this, "action name" ); yourAction->plug( kfileDialog->toolBar() );
Definition at line 722 of file kfiledialog.h.
void KFileDialog::toolbarCallback | ( | int | ) | [protected, slot] |
void KFileDialog::updateAutoSelectExtension | ( | void | ) | [protected] |
Updates the currentFilterExtension and the availability of the Automatically Select Extension Checkbox (visible if operationMode() == Saving and enabled if an extension _will_ be associated with the currentFilter(), _after_ this call).
You should call this after filterWidget->setCurrentItem().
- Since:
- 3.2
Definition at line 1908 of file kfiledialog.cpp.
void KFileDialog::updateStatusLine | ( | int | dirs, | |
int | files | |||
) | [protected, virtual, slot] |
void KFileDialog::urlEntered | ( | const KURL & | url | ) | [protected, slot] |
Definition at line 1159 of file kfiledialog.cpp.
void KFileDialog::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
void KFileDialog::writeConfig | ( | KConfig * | kc, | |
const QString & | group = QString::null | |||
) | [protected, virtual] |
Member Data Documentation
bool KFileDialog::autoDirectoryFollowing [protected] |
Definition at line 979 of file kfiledialog.h.
KFileFilterCombo* KFileDialog::filterWidget [protected] |
Definition at line 829 of file kfiledialog.h.
KURL * KFileDialog::lastDirectory [static, protected] |
Definition at line 825 of file kfiledialog.h.
KURLComboBox* KFileDialog::locationEdit [protected] |
Definition at line 827 of file kfiledialog.h.
KDirOperator* KFileDialog::ops [protected] |
Definition at line 978 of file kfiledialog.h.
KToolBar* KFileDialog::toolbar [protected] |
Definition at line 823 of file kfiledialog.h.
The documentation for this class was generated from the following files: