kio
KDirOperator Class Reference
This widget works as a network transparent filebrowser. More...
#include <kdiroperator.h>
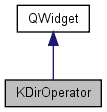
Public Types | |
enum | ActionTypes { SortActions = 1, ViewActions = 2, NavActions = 4, FileActions = 8, AllActions = 15 } |
Public Slots | |
void | back () |
void | cdUp () |
void | deleteSelected () |
void | forward () |
void | home () |
QString | makeCompletion (const QString &) |
QString | makeDirCompletion (const QString &) |
void | mkdir () |
void | rereadDir () |
void | trashSelected (KAction::ActivationReason, Qt::ButtonState) |
void | updateDir () |
void | updateSelectionDependentActions () |
Signals | |
void | completion (const QString &) |
void | dirActivated (const KFileItem *item) |
void | dropped (const KFileItem *item, QDropEvent *event, const KURL::List &urls) |
void | fileHighlighted (const KFileItem *item) |
void | fileSelected (const KFileItem *item) |
void | finishedLoading () |
void | updateInformation (int files, int dirs) |
void | urlEntered (const KURL &) |
void | viewChanged (KFileView *newView) |
Public Member Functions | |
KActionCollection * | actionCollection () const |
void | clearFilter () |
void | clearHistory () |
virtual bool | close (bool alsoDelete) |
void | close () |
KCompletion * | completionObject () const |
KIO::DeleteJob * | del (const KFileItemList &items, QWidget *parent, bool ask=true, bool showProgress=true) |
KIO::DeleteJob * | del (const KFileItemList &items, bool ask=true, bool showProgress=true) |
KCompletion * | dirCompletionObject () const |
bool | dirHighlighting () const |
KDirLister * | dirLister () const |
bool | dirOnlyMode () const |
bool | isRoot () const |
bool | isSelected (const KFileItem *item) const |
KDirOperator (const KURL &urlName=KURL(), QWidget *parent=0, const char *name=0) | |
QStringList | mimeFilter () const |
bool | mkdir (const QString &directory, bool enterDirectory=true) |
KFile::Mode | mode () const |
const QString & | nameFilter () const |
int | numDirs () const |
int | numFiles () const |
bool | onlyDoubleClickSelectsFiles () const |
KProgress * | progressBar () const |
virtual void | readConfig (KConfig *, const QString &group=QString::null) |
const KFileItemList * | selectedItems () const |
virtual void | setAcceptDrops (bool b) |
void | setCurrentItem (const QString &filename) |
void | setDropOptions (int options) |
void | setEnableDirHighlighting (bool enable) |
void | setMimeFilter (const QStringList &mimetypes) |
void | setMode (KFile::Mode m) |
void | setNameFilter (const QString &filter) |
void | setOnlyDoubleClickSelectsFiles (bool enable) |
void | setPreviewWidget (const QWidget *w) |
void | setShowHiddenFiles (bool s) |
void | setSorting (QDir::SortSpec) |
void | setupMenu (int whichActions) |
void | setURL (const KURL &url, bool clearforward) |
void | setView (KFile::FileView view) |
void | setView (KFileView *view) |
void | setViewConfig (KConfig *config, const QString &group) |
bool | showHiddenFiles () const |
QDir::SortSpec | sorting () const |
KIO::CopyJob * | trash (const KFileItemList &items, QWidget *parent, bool ask=true, bool showProgress=true) |
KURL | url () const |
KFileView * | view () const |
KConfig * | viewConfig () |
QString | viewConfigGroup () const |
QWidget * | viewWidget () const |
virtual void | writeConfig (KConfig *, const QString &group=QString::null) |
virtual | ~KDirOperator () |
Static Public Member Functions | |
static bool | dirOnlyMode (uint mode) |
Protected Slots | |
virtual void | activatedMenu (const KFileItem *, const QPoint &pos) |
void | highlightFile (const KFileItem *i) |
void | insertNewFiles (const KFileItemList &newone) |
void | itemDeleted (KFileItem *) |
void | pathChanged () |
void | resetCursor () |
void | selectDir (const KFileItem *item) |
void | selectFile (const KFileItem *item) |
void | slotCompletionMatch (const QString &match) |
void | sortByDate () |
void | sortByName () |
void | sortBySize () |
void | sortReversed () |
void | toggleDirsFirst () |
void | toggleIgnoreCase () |
Protected Member Functions | |
bool | checkPreviewSupport () |
virtual KFileView * | createView (QWidget *parent, KFile::FileView view) |
void | prepareCompletionObjects () |
virtual void | resizeEvent (QResizeEvent *) |
void | setDirLister (KDirLister *lister) |
void | setupActions () |
void | setupMenu () |
void | updateSortActions () |
void | updateViewActions () |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
This widget works as a network transparent filebrowser.You specify a URL to display and this url will be loaded via KDirLister. The user can browse through directories, highlight and select files, delete or rename files.
It supports different views, e.g. a detailed view (see KFileDetailView), a simple icon view (see KFileIconView), a combination of two views, separating directories and files ( KCombiView).
Additionally, a preview view is available (see KFilePreview), which can show either a simple or detailed view and additionally a preview widget (see setPreviewWidget()). KImageFilePreview is one implementation of a preview widget, that displays previews for all supported filetypes utilizing KIO::PreviewJob.
Currently, those classes don't support Drag&Drop out of the box -- there you have to use your own view-classes. You can use some DnD-aware views from Bj�n Sahlstr� <bjorn@kbear.org> until they will be integrated into this library. See http://devel-home.kde.org/~pfeiffer/DnD-classes.tar.gz
This widget is the one used in the KFileDialog.
Basic usage is like this:
KDirOperator *op = new KDirOperator( KURL( "file:/home/gis" ), this ); // some signals you might be interested in connect(op, SIGNAL(urlEntered(const KURL&)), SLOT(urlEntered(const KURL&))); connect(op, SIGNAL(fileHighlighted(const KFileItem *)), SLOT(fileHighlighted(const KFileItem *))); connect(op, SIGNAL(fileSelected(const KFileItem *)), SLOT(fileSelected(const KFileItem *))); connect(op, SIGNAL(finishedLoading()), SLOT(slotLoadingFinished())); op->readConfig( KGlobal::config(), "Your KDiroperator ConfigGroup" ); op->setView(KFile::Default);
This will create a childwidget of 'this' showing the directory contents of /home/gis in the default-view. The view is determined by the readConfig() call, which will read the KDirOperator settings, the user left your program with (and which you saved with op->writeConfig()).
A widget for displaying files and browsing directories.
Definition at line 97 of file kdiroperator.h.
Member Enumeration Documentation
The various action types.
These values can be or'd together
- Since:
- 3.1
Definition at line 106 of file kdiroperator.h.
Constructor & Destructor Documentation
KDirOperator::KDirOperator | ( | const KURL & | urlName = KURL() , |
|
QWidget * | parent = 0 , |
|||
const char * | name = 0 | |||
) |
Constructs the KDirOperator with no initial view.
As the views are configurable, call readConfig() to load the user's configuration and then setView to explicitly set a view.
This constructor doesn't start loading the url, setView will do it.
Definition at line 97 of file kdiroperator.cpp.
KDirOperator::~KDirOperator | ( | ) | [virtual] |
Member Function Documentation
KActionCollection* KDirOperator::actionCollection | ( | ) | const [inline] |
an accessor to a collection of all available Actions.
The actions are static, they will be there all the time (no need to connect to the signals KActionCollection::inserted() or removed().
There are the following actions:
- popupMenu : an ActionMenu presenting a popupmenu with all actions
- up : changes to the parent directory
- back : goes back to the previous directory
- forward : goes forward in the history
- home : changes to the user's home directory
- reload : reloads the current directory
- separator : a separator
- mkdir : opens a dialog box to create a directory
- delete : deletes the selected files/directories
- sorting menu : an ActionMenu containing all sort-options
- by name : sorts by name
- by date : sorts by date
- by size : sorts by size
- reversed : reverses the sort order
- dirs first : sorts directories before files
- case insensitive : sorts case insensitively
- view menu : an ActionMenu containing all actions concerning the view
- short view : shows a simple fileview
- detailed view : shows a detailed fileview (dates, permissions ,...)
- show hidden : shows hidden files
- separate dirs : shows directories in a separate pane
- preview : shows a preview next to the fileview
- single : hides the separate view for directories or the preview
- properties : shows a KPropertiesDialog for the selected files
You can e.g. use
actionCollection()->action( "up" )->plug( someToolBar );
- Returns:
- all available Actions
Definition at line 390 of file kdiroperator.h.
void KDirOperator::back | ( | ) | [slot] |
Goes one step back in the history and opens that url.
Definition at line 746 of file kdiroperator.cpp.
void KDirOperator::cdUp | ( | ) | [slot] |
bool KDirOperator::checkPreviewSupport | ( | ) | [protected] |
Checks if there support from KIO::PreviewJob for the currently shown files, taking mimeFilter() and nameFilter() into account Enables/disables the preview-action accordingly.
Definition at line 810 of file kdiroperator.cpp.
void KDirOperator::clearFilter | ( | ) |
Clears both the namefilter and mimetype filter, so that all files and directories will be shown.
Call updateDir() to apply it.
- See also:
- setMimeFilter
Definition at line 791 of file kdiroperator.cpp.
void KDirOperator::clearHistory | ( | ) |
virtual bool KDirOperator::close | ( | bool | alsoDelete | ) | [inline, virtual] |
Reimplemented to avoid "hidden virtual" warnings.
Reimplemented from QWidget.
Definition at line 141 of file kdiroperator.h.
void KDirOperator::close | ( | ) |
Stops loading immediately.
You don't need to call this, usually.
Reimplemented from QWidget.
Definition at line 573 of file kdiroperator.cpp.
void KDirOperator::completion | ( | const QString & | ) | [signal] |
KCompletion* KDirOperator::completionObject | ( | ) | const [inline] |
- Returns:
- a KCompletion object, containing all filenames and directories of the current directory/URL. You can use it to insert it into a KLineEdit or KComboBox Note: it will only contain files, after prepareCompletionObjects() has been called. It will be implicitly called from makeCompletion() or makeDirCompletion()
Definition at line 329 of file kdiroperator.h.
KFileView * KDirOperator::createView | ( | QWidget * | parent, | |
KFile::FileView | view | |||
) | [protected, virtual] |
A view factory for creating predefined fileviews.
Called internally by setView , but you can also call it directly. Reimplement this if you depend on self defined fileviews.
- Parameters:
-
parent is the QWidget to be set as parent view is the predefined view to be set, note: this can be several ones OR:ed together.
- Returns:
- the created KFileView
Definition at line 884 of file kdiroperator.cpp.
KIO::DeleteJob * KDirOperator::del | ( | const KFileItemList & | items, | |
QWidget * | parent, | |||
bool | ask = true , |
|||
bool | showProgress = true | |||
) |
Starts and returns a KIO::DeleteJob to delete the given items
.
- Parameters:
-
items the list of items to be deleted parent the parent widget used for the confirmation dialog ask specifies whether a confirmation dialog should be shown showProgress passed to the DeleteJob to show a progress dialog
- Since:
- 3.1
Definition at line 442 of file kdiroperator.cpp.
KIO::DeleteJob * KDirOperator::del | ( | const KFileItemList & | items, | |
bool | ask = true , |
|||
bool | showProgress = true | |||
) |
Starts and returns a KIO::DeleteJob to delete the given items
.
- Parameters:
-
items the list of items to be deleted ask specifies whether a confirmation dialog should be shown showProgress passed to the DeleteJob to show a progress dialog
Definition at line 436 of file kdiroperator.cpp.
void KDirOperator::deleteSelected | ( | ) | [slot] |
void KDirOperator::dirActivated | ( | const KFileItem * | item | ) | [signal] |
KCompletion* KDirOperator::dirCompletionObject | ( | ) | const [inline] |
- Returns:
- a KCompletion object, containing only all directories of the current directory/URL. You can use it to insert it into a KLineEdit or KComboBox Note: it will only contain directories, after prepareCompletionObjects() has been called. It will be implicitly called from makeCompletion() or makeDirCompletion()
Definition at line 341 of file kdiroperator.h.
bool KDirOperator::dirHighlighting | ( | ) | const |
- Returns:
- whether the last directory will be made the current item when going up in the directory hierarchy.
Definition at line 1663 of file kdiroperator.cpp.
KDirLister* KDirOperator::dirLister | ( | ) | const [inline] |
static bool KDirOperator::dirOnlyMode | ( | uint | mode | ) | [inline, static] |
Definition at line 540 of file kdiroperator.h.
bool KDirOperator::dirOnlyMode | ( | ) | const [inline] |
- Returns:
- true if we are in directory-only mode, that is, no files are shown.
Definition at line 538 of file kdiroperator.h.
void KDirOperator::dropped | ( | const KFileItem * | item, | |
QDropEvent * | event, | |||
const KURL::List & | urls | |||
) | [signal] |
Emitted when files are dropped.
Dropping files is disabled by default. You need to enable it with setAcceptDrops()
- Parameters:
-
item the item on which the drop occurred or 0. event the drop event itself. urls the urls that where dropped.
- Since:
- 3.2
void KDirOperator::fileHighlighted | ( | const KFileItem * | item | ) | [signal] |
Emitted when a file is highlighted or generally the selection changes in multiselection mode.
In the latter case, item
is 0L. You can access the selected items with selectedItems().
void KDirOperator::fileSelected | ( | const KFileItem * | item | ) | [signal] |
void KDirOperator::finishedLoading | ( | ) | [signal] |
void KDirOperator::forward | ( | ) | [slot] |
Goes one step forward in the history and opens that url.
Definition at line 760 of file kdiroperator.cpp.
void KDirOperator::highlightFile | ( | const KFileItem * | i | ) | [inline, protected, slot] |
void KDirOperator::home | ( | ) | [slot] |
void KDirOperator::insertNewFiles | ( | const KFileItemList & | newone | ) | [protected, slot] |
Adds a new list of KFileItems to the view (coming from KDirLister).
Definition at line 1143 of file kdiroperator.cpp.
bool KDirOperator::isRoot | ( | ) | const [inline] |
- Returns:
- true if we are displaying the root directory of the current url
Definition at line 255 of file kdiroperator.h.
bool KDirOperator::isSelected | ( | const KFileItem * | item | ) | const [inline] |
- Returns:
- true if
item
is currently selected, or false otherwise.
Definition at line 305 of file kdiroperator.h.
void KDirOperator::itemDeleted | ( | KFileItem * | item | ) | [protected, slot] |
Removes the given KFileItem item from the view (usually called from KDirLister).
Definition at line 1174 of file kdiroperator.cpp.
Tries to complete the given string (only completes files).
Definition at line 1208 of file kdiroperator.cpp.
Tries to complete the given string (only completes directores).
Definition at line 1219 of file kdiroperator.cpp.
QStringList KDirOperator::mimeFilter | ( | ) | const [inline] |
void KDirOperator::mkdir | ( | ) | [slot] |
bool KDirOperator::mkdir | ( | const QString & | directory, | |
bool | enterDirectory = true | |||
) |
Creates the given directory/url.
If it is a relative path, it will be completed with the current directory. If enterDirectory is true, the directory will be entered after a successful operation. If unsuccessful, a messagebox will be presented to the user.
- Returns:
- true if the directory could be created.
Definition at line 400 of file kdiroperator.cpp.
KFile::Mode KDirOperator::mode | ( | ) | const |
const QString& KDirOperator::nameFilter | ( | ) | const [inline] |
- Returns:
- the current namefilter.
- See also:
- setNameFilter
Definition at line 156 of file kdiroperator.h.
int KDirOperator::numDirs | ( | ) | const |
- Returns:
- the number of directories in the currently listed url. Returns 0 if there is no view.
Definition at line 280 of file kdiroperator.cpp.
int KDirOperator::numFiles | ( | ) | const |
- Returns:
- the number of files in the currently listed url. Returns 0 if there is no view.
Definition at line 285 of file kdiroperator.cpp.
bool KDirOperator::onlyDoubleClickSelectsFiles | ( | ) | const |
- Returns:
- whether files (not directories) should only be select()ed by double-clicks.
- See also:
- setOnlyDoubleClickSelectsFiles
Definition at line 1574 of file kdiroperator.cpp.
void KDirOperator::pathChanged | ( | ) | [protected, slot] |
Called after setURL() to load the directory, update the history, etc.
Definition at line 708 of file kdiroperator.cpp.
void KDirOperator::prepareCompletionObjects | ( | ) | [protected] |
Synchronizes the completion objects with the entries of the currently listed url.
Automatically called from makeCompletion() and makeDirCompletion()
Definition at line 1230 of file kdiroperator.cpp.
KProgress * KDirOperator::progressBar | ( | ) | const |
- Returns:
- the progress widget, that is shown during directory listing. You can for example reparent() it to put it into a statusbar.
Definition at line 1623 of file kdiroperator.cpp.
void KDirOperator::readConfig | ( | KConfig * | kc, | |
const QString & | group = QString::null | |||
) | [virtual] |
Reads the default settings for a view, i.e.
the default KFile::FileView. Also reads the sorting and whether hidden files should be shown. Note: the default view will not be set - you have to call
to apply it.
- See also:
- setView
Definition at line 1442 of file kdiroperator.cpp.
void KDirOperator::rereadDir | ( | ) | [slot] |
void KDirOperator::resetCursor | ( | ) | [protected, slot] |
Restores the normal cursor after showing the busy-cursor.
Also hides the progressbar.
Definition at line 176 of file kdiroperator.cpp.
void KDirOperator::resizeEvent | ( | QResizeEvent * | ) | [protected, virtual] |
void KDirOperator::selectDir | ( | const KFileItem * | item | ) | [protected, slot] |
const KFileItemList* KDirOperator::selectedItems | ( | ) | const [inline] |
- Returns:
- a list of all currently selected items. If there is no view, then 0L is returned.
Definition at line 298 of file kdiroperator.h.
void KDirOperator::selectFile | ( | const KFileItem * | item | ) | [protected, slot] |
void KDirOperator::setAcceptDrops | ( | bool | b | ) | [virtual] |
Reimplemented - allow dropping of files if b
is true.
- Parameters:
-
b true if the widget should allow dropping of files
Reimplemented from QWidget.
Definition at line 932 of file kdiroperator.cpp.
void KDirOperator::setCurrentItem | ( | const QString & | filename | ) |
Clears the current selection and attempts to set filename
the current file.
filename is just the name, no path or url.
Definition at line 1191 of file kdiroperator.cpp.
void KDirOperator::setDirLister | ( | KDirLister * | lister | ) | [protected] |
void KDirOperator::setDropOptions | ( | int | options | ) |
Sets the options for dropping files.
- See also:
- KFileView::DropOptions
- Since:
- 3.2
Definition at line 939 of file kdiroperator.cpp.
void KDirOperator::setEnableDirHighlighting | ( | bool | enable | ) |
When going up in the directory hierarchy, KDirOperator can highlight the directory that was just left.
I.e. when you go from /home/gis/src to /home/gis, the item "src" will be made the current item.
Default is off, because this behavior introduces bug #136630. Don't enable until this bug is fixed.
Definition at line 1658 of file kdiroperator.cpp.
void KDirOperator::setMimeFilter | ( | const QStringList & | mimetypes | ) |
Sets a list of mimetypes as filter.
Only files of those mimetypes will be shown.
Example:
QStringList filter; filter << "text/html" << "image/png" << "inode/directory"; dirOperator->setMimefilter( filter );
Node: Without the mimetype inode/directory, only files would be shown. Call updateDir() to apply it.
- See also:
- KDirLister::setMimeFilter
Definition at line 804 of file kdiroperator.cpp.
void KDirOperator::setMode | ( | KFile::Mode | m | ) |
Sets the listing/selection mode for the views, an OR'ed combination of.
- File
- Directory
- Files
- ExistingOnly
- LocalOnly
Definition at line 1086 of file kdiroperator.cpp.
void KDirOperator::setNameFilter | ( | const QString & | filter | ) |
Sets a filter like "*.cpp *.h *.o".
Only files matching that filter will be shown. Call updateDir() to apply it.
- See also:
- KDirLister::setNameFilter
Definition at line 798 of file kdiroperator.cpp.
void KDirOperator::setOnlyDoubleClickSelectsFiles | ( | bool | enable | ) |
This is a KFileDialog specific hack: we want to select directories with single click, but not files.
But as a generic class, we have to be able to select files on single click as well.
This gives us the opportunity to do both.
The default is false, set it to true if you don't want files selected with single click.
Definition at line 1567 of file kdiroperator.cpp.
void KDirOperator::setPreviewWidget | ( | const QWidget * | w | ) |
Sets a preview-widget to be shown next to the file-view.
The ownership of w
is transferred to KDirOperator, so don't delete it yourself!
Definition at line 264 of file kdiroperator.cpp.
void KDirOperator::setShowHiddenFiles | ( | bool | s | ) | [inline] |
void KDirOperator::setSorting | ( | QDir::SortSpec | spec | ) |
void KDirOperator::setupActions | ( | ) | [protected] |
Sets up all the actions.
Called from the constructor, you usually better not call this.
Definition at line 1254 of file kdiroperator.cpp.
void KDirOperator::setupMenu | ( | ) | [protected] |
Sets up the context-menu with all the necessary actions.
Called from the constructor, you usually don't need to call this.
- Since:
- 3.1
Definition at line 1355 of file kdiroperator.cpp.
void KDirOperator::setupMenu | ( | int | whichActions | ) |
Sets up the action menu.
- Parameters:
-
whichActions is an value of OR'd ActionTypes that controls which actions to show in the action menu
Definition at line 1360 of file kdiroperator.cpp.
void KDirOperator::setURL | ( | const KURL & | url, | |
bool | clearforward | |||
) |
Sets a new url to list.
- Parameters:
-
clearforward specifies whether the "forward" history should be cleared. url the URL to set
Definition at line 636 of file kdiroperator.cpp.
void KDirOperator::setView | ( | KFile::FileView | view | ) |
Sets one of the predefined fileviews.
- See also:
- KFile::FileView
Definition at line 946 of file kdiroperator.cpp.
void KDirOperator::setView | ( | KFileView * | view | ) |
Sets a new KFileView to be used for showing and browsing files.
Note: this will read the current url() to fill the view.
- See also:
- KFileView
Definition at line 1099 of file kdiroperator.cpp.
void KDirOperator::setViewConfig | ( | KConfig * | config, | |
const QString & | group | |||
) |
Sets the config object and the to be used group in KDirOperator.
This will be used to store the view's configuration via KFileView::writeConfig() (and for KFileView::readConfig()). If you don't set this, the views cannot save and restore their configuration.
Usually you call this right after KDirOperator creation so that the view instantiation can make use of it already.
Note that KDirOperator does NOT take ownership of that object (typically it's KGlobal::config() anyway.
- See also:
- viewConfig
- Since:
- 3.1
Definition at line 1721 of file kdiroperator.cpp.
bool KDirOperator::showHiddenFiles | ( | ) | const [inline] |
- Returns:
- true when hidden files are shown or false otherwise.
Definition at line 134 of file kdiroperator.h.
void KDirOperator::slotCompletionMatch | ( | const QString & | match | ) | [protected, slot] |
Tries to make the given match
as current item in the view and emits completion( match ).
Definition at line 1248 of file kdiroperator.cpp.
void KDirOperator::sortByDate | ( | ) | [inline, protected, slot] |
void KDirOperator::sortByName | ( | ) | [inline, protected, slot] |
void KDirOperator::sortBySize | ( | ) | [inline, protected, slot] |
QDir::SortSpec KDirOperator::sorting | ( | ) | const [inline] |
- Returns:
- the current way of sorting files and directories
Definition at line 250 of file kdiroperator.h.
void KDirOperator::sortReversed | ( | ) | [inline, protected, slot] |
void KDirOperator::toggleDirsFirst | ( | ) | [inline, protected, slot] |
Toggles showing directories first / having them sorted like files.
Definition at line 784 of file kdiroperator.h.
void KDirOperator::toggleIgnoreCase | ( | ) | [inline, protected, slot] |
KIO::CopyJob * KDirOperator::trash | ( | const KFileItemList & | items, | |
QWidget * | parent, | |||
bool | ask = true , |
|||
bool | showProgress = true | |||
) |
Starts and returns a KIO::CopyJob to trash the given items
.
- Parameters:
-
items the list of items to be trashed parent the parent widget used for the confirmation dialog ask specifies whether a confirmation dialog should be shown showProgress passed to the CopyJob to show a progress dialog
- Since:
- 3.4
Definition at line 505 of file kdiroperator.cpp.
void KDirOperator::trashSelected | ( | KAction::ActivationReason | reason, | |
Qt::ButtonState | state | |||
) | [slot] |
Trashes the currently selected files/directories.
- Since:
- 3.4
Definition at line 558 of file kdiroperator.cpp.
void KDirOperator::updateDir | ( | ) | [slot] |
void KDirOperator::updateInformation | ( | int | files, | |
int | dirs | |||
) | [signal] |
void KDirOperator::updateSelectionDependentActions | ( | ) | [slot] |
Enables/disables actions that are selection dependent.
Call this e.g. when you are about to show a popup menu using some of KDirOperators actions.
Definition at line 255 of file kdiroperator.cpp.
void KDirOperator::updateSortActions | ( | ) | [protected] |
Updates the sorting-related actions to comply with the current sorting.
- See also:
- sorting
Definition at line 1414 of file kdiroperator.cpp.
void KDirOperator::updateViewActions | ( | ) | [protected] |
Updates the view-related actions to comply with the current KFile::FileView.
Definition at line 1431 of file kdiroperator.cpp.
KURL KDirOperator::url | ( | ) | const |
void KDirOperator::urlEntered | ( | const KURL & | ) | [signal] |
KFileView* KDirOperator::view | ( | ) | const [inline] |
void KDirOperator::viewChanged | ( | KFileView * | newView | ) | [signal] |
Emitted whenever the current fileview is changed, either by an explicit call to setView() or by the user selecting a different view thru the GUI.
KConfig * KDirOperator::viewConfig | ( | ) |
Returns the KConfig object used for saving and restoring view's configuration.
- Returns:
- the KConfig object used for saving and restoring view's configuration.
- Since:
- 3.1
Definition at line 1727 of file kdiroperator.cpp.
QString KDirOperator::viewConfigGroup | ( | ) | const |
Returns the group name used for saving and restoring view's configuration.
- Returns:
- the group name used for saving and restoring view's configuration.
- Since:
- 3.1
Definition at line 1732 of file kdiroperator.cpp.
QWidget* KDirOperator::viewWidget | ( | ) | const [inline] |
Returns the widget of the current view.
0L if there is no view/widget. (KFileView itself is not a widget.)
Definition at line 233 of file kdiroperator.h.
void KDirOperator::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Definition at line 1737 of file kdiroperator.cpp.
void KDirOperator::writeConfig | ( | KConfig * | kc, | |
const QString & | group = QString::null | |||
) | [virtual] |
Saves the current settings like sorting, simple or detailed view.
- See also:
- readConfig
Definition at line 1498 of file kdiroperator.cpp.
The documentation for this class was generated from the following files: