kio
KCombiView Class Reference
This view is designed to combine two KFileViews into one widget, to show directories on the left side and files on the right side. More...
#include <kcombiview.h>
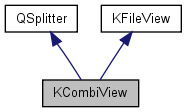
Public Member Functions | |
virtual KActionCollection * | actionCollection () const |
virtual void | clear () |
virtual void | clearSelection () |
virtual void | clearView () |
virtual KFileItem * | currentFileItem () const |
void | ensureItemVisible (const KFileItem *) |
virtual KFileItem * | firstFileItem () const |
virtual void | insertItem (KFileItem *i) |
virtual void | invertSelection () |
virtual bool | isSelected (const KFileItem *) const |
KCombiView (QWidget *parent, const char *name) | |
virtual void | listingCompleted () |
virtual KFileItem * | nextItem (const KFileItem *) const |
virtual KFileItem * | prevItem (const KFileItem *) const |
virtual void | readConfig (KConfig *, const QString &group=QString::null) |
virtual void | removeItem (const KFileItem *) |
virtual void | selectAll () |
virtual void | setAcceptDrops (bool b) |
virtual void | setCurrentItem (const KFileItem *) |
void | setRight (KFileView *view) |
virtual void | setSelected (const KFileItem *, bool) |
virtual void | setSelectionMode (KFile::SelectionMode sm) |
virtual void | setSorting (QDir::SortSpec sort) |
virtual void | updateView (const KFileItem *) |
virtual void | updateView (bool) |
virtual QWidget * | widget () |
virtual void | writeConfig (KConfig *, const QString &group=QString::null) |
virtual | ~KCombiView () |
Protected Slots | |
void | slotSortingChanged (QDir::SortSpec) |
Protected Member Functions | |
virtual bool | eventFilter (QObject *o, QEvent *e) |
void | setDropOptions_impl (int options) |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
KFileIconView * | left |
KFileView * | right |
Detailed Description
This view is designed to combine two KFileViews into one widget, to show directories on the left side and files on the right side.Methods like selectedItems() to query status _only_ work on the right side, i.e. on the files.
After creating the KCombiView, you need to supply the view shown in the right, (see setRight()). Available KFileView implementations are KFileIconView and KFileDetailView.
Most of the below methods are just implementations of the baseclass KFileView, so look there for documentation.
Definition at line 54 of file kcombiview.h.
Constructor & Destructor Documentation
KCombiView::KCombiView | ( | QWidget * | parent, | |
const char * | name | |||
) |
Definition at line 42 of file kcombiview.cpp.
KCombiView::~KCombiView | ( | ) | [virtual] |
Definition at line 63 of file kcombiview.cpp.
Member Function Documentation
KActionCollection * KCombiView::actionCollection | ( | ) | const [virtual] |
- Returns:
- the view-specific action-collection. Every view should add its actions here (if it has any) to make them available to e.g. the KDirOperator's popup-menu.
Reimplemented from KFileView.
Definition at line 322 of file kcombiview.cpp.
void KCombiView::clear | ( | ) | [virtual] |
Clears the view and all item lists.
Reimplemented from KFileView.
Definition at line 147 of file kcombiview.cpp.
void KCombiView::clearSelection | ( | ) | [virtual] |
Clears any selection, unhighlights everything.
Must be implemented by the view.
Implements KFileView.
Definition at line 155 of file kcombiview.cpp.
void KCombiView::clearView | ( | ) | [virtual] |
pure virtual function, that should be implemented to clear the view.
At this moment the list is already empty
Implements KFileView.
Definition at line 111 of file kcombiview.cpp.
KFileItem * KCombiView::currentFileItem | ( | ) | const [virtual] |
- Returns:
- the "current" KFileItem, e.g. where the cursor is. Returns 0L when there is no current item (e.g. in an empty view). Subclasses have to implement this.
Implements KFileView.
Definition at line 206 of file kcombiview.cpp.
void KCombiView::ensureItemVisible | ( | const KFileItem * | i | ) | [virtual] |
pure virtual function, that should be implemented to make item i visible, i.e.
by scrolling the view appropriately.
Implements KFileView.
Definition at line 222 of file kcombiview.cpp.
Definition at line 354 of file kcombiview.cpp.
KFileItem * KCombiView::firstFileItem | ( | ) | const [virtual] |
void KCombiView::insertItem | ( | KFileItem * | i | ) | [virtual] |
The derived view must implement this function to add the file in the widget.
Make sure to call this implementation, i.e. KFileView::insertItem( i );
Reimplemented from KFileView.
Definition at line 87 of file kcombiview.cpp.
void KCombiView::invertSelection | ( | ) | [virtual] |
Inverts the current selection, i.e.
selects all items, that were up to now not selected and deselects the other.
Reimplemented from KFileView.
Definition at line 169 of file kcombiview.cpp.
bool KCombiView::isSelected | ( | const KFileItem * | ) | const [virtual] |
- Returns:
- whether the given item is currently selected. Must be implemented by the view.
Implements KFileView.
Definition at line 176 of file kcombiview.cpp.
void KCombiView::listingCompleted | ( | ) | [virtual] |
This hook is called when all items of the currently listed directory are listed and inserted into the view, i.e.
there won't come any new items anymore.
Reimplemented from KFileView.
Definition at line 140 of file kcombiview.cpp.
void KCombiView::readConfig | ( | KConfig * | config, | |
const QString & | group = QString::null | |||
) | [virtual] |
void KCombiView::removeItem | ( | const KFileItem * | item | ) | [virtual] |
Removes an item from the list; has to be implemented by the view.
Call KFileView::removeItem( item ) after removing it.
Reimplemented from KFileView.
Definition at line 132 of file kcombiview.cpp.
void KCombiView::selectAll | ( | ) | [virtual] |
Selects all items.
You may want to override this, if you can implement it more efficiently than calling setSelected() with every item. This works only in Multiselection mode of course.
Reimplemented from KFileView.
Definition at line 162 of file kcombiview.cpp.
void KCombiView::setAcceptDrops | ( | bool | b | ) | [virtual] |
Definition at line 327 of file kcombiview.cpp.
void KCombiView::setCurrentItem | ( | const KFileItem * | item | ) | [virtual] |
Reimplement this to set item
the current item in the view, e.g.
the item having focus.
Implements KFileView.
Definition at line 199 of file kcombiview.cpp.
void KCombiView::setDropOptions_impl | ( | int | options | ) | [protected] |
void KCombiView::setRight | ( | KFileView * | view | ) |
Sets the view to be shown in the right.
You need to call this before doing anything else with this widget.
Definition at line 68 of file kcombiview.cpp.
void KCombiView::setSelected | ( | const KFileItem * | , | |
bool | enable | |||
) | [virtual] |
Tells the view that it should highlight the item.
This function must be implemented by the view.
Implements KFileView.
Definition at line 192 of file kcombiview.cpp.
void KCombiView::setSelectionMode | ( | KFile::SelectionMode | sm | ) | [virtual] |
void KCombiView::setSorting | ( | QDir::SortSpec | sort | ) | [virtual] |
Sets the sorting order of the view.
Default is QDir::Name | QDir::IgnoreCase | QDir::DirsFirst Override this in your subclass and sort accordingly (usually by setting the sorting-key for every item and telling QIconView or QListView to sort.
A view may choose to use a different sorting than QDir::Name, Time or Size. E.g. to sort by mimetype or any possible string. Set the sorting to QDir::Unsorted for that and do the rest internally.
- See also:
- sortingKey
Reimplemented from KFileView.
Definition at line 101 of file kcombiview.cpp.
void KCombiView::slotSortingChanged | ( | QDir::SortSpec | sorting | ) | [protected, slot] |
Definition at line 296 of file kcombiview.cpp.
void KCombiView::updateView | ( | const KFileItem * | i | ) | [virtual] |
void KCombiView::updateView | ( | bool | f | ) | [virtual] |
void KCombiView::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
virtual QWidget* KCombiView::widget | ( | ) | [inline, virtual] |
a pure virtual function to get a QWidget, that can be added to other widgets.
This function is needed to make it possible for derived classes to derive from other widgets.
Implements KFileView.
Definition at line 63 of file kcombiview.h.
void KCombiView::writeConfig | ( | KConfig * | config, | |
const QString & | group = QString::null | |||
) | [virtual] |
Member Data Documentation
KFileIconView* KCombiView::left [protected] |
Definition at line 106 of file kcombiview.h.
KFileView* KCombiView::right [protected] |
Definition at line 107 of file kcombiview.h.
The documentation for this class was generated from the following files: