kio
KURLBar Class Reference
KURLBar is a widget that displays icons together with a description. More...
#include <kurlbar.h>
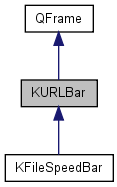
Public Slots | |
virtual void | setCurrentItem (const KURL &url) |
Signals | |
void | activated (const KURL &url) |
Public Member Functions | |
virtual void | clear () |
KURLBarItem * | currentItem () const |
KURL | currentURL () const |
int | iconSize () const |
virtual KURLBarItem * | insertDynamicItem (const KURL &url, const QString &description, const QString &icon=QString::null, KIcon::Group group=KIcon::Panel) |
virtual KURLBarItem * | insertItem (const KURL &url, const QString &description, bool applicationLocal=true, const QString &icon=QString::null, KIcon::Group group=KIcon::Panel) |
bool | isImmutable () const |
bool | isModified () const |
bool | isVertical () const |
KURLBar (bool useGlobalItems, QWidget *parent=0, const char *name=0, WFlags f=0) | |
KURLBarListBox * | listBox () const |
virtual QSize | minimumSizeHint () const |
Orientation | orientation () const |
virtual void | readConfig (KConfig *config, const QString &itemGroup) |
virtual void | readItem (int i, KConfig *config, bool applicationLocal) |
virtual void | setIconSize (int size) |
virtual void | setListBox (KURLBarListBox *) |
virtual void | setOrientation (Qt::Orientation orient) |
virtual QSize | sizeHint () const |
virtual void | writeConfig (KConfig *config, const QString &itemGroup) |
virtual void | writeItem (KURLBarItem *item, int i, KConfig *, bool global) |
~KURLBar () | |
Protected Slots | |
virtual void | slotContextMenuRequested (QListBoxItem *, const QPoint &pos) |
virtual void | slotDropped (QDropEvent *) |
virtual void | slotSelected (QListBoxItem *) |
Protected Member Functions | |
virtual bool | addNewItem () |
virtual bool | editItem (KURLBarItem *item) |
virtual void | paletteChange (const QPalette &) |
virtual void | resizeEvent (QResizeEvent *) |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
KURLBarItem * | m_activeItem |
bool | m_isImmutable:1 |
bool | m_isModified:1 |
bool | m_useGlobal:1 |
Detailed Description
KURLBar is a widget that displays icons together with a description.They can be arranged either horizontally or vertically. Clicking on an item will cause the activated() signal to be emitted. The user can edit existing items by choosing "Edit entry" in the contextmenu. He can also remove or add new entries (via drag&drop or the context menu).
KURLBar offers the methods readConfig() and writeConfig() to read and write the configuration of all the entries. It can differentiate between global and local entries -- global entries will be saved in the global configuration (kdeglobals), while local entries will be saved in your application's KConfig object.
Due to the configurability, you usually only insert some default entries once and then solely use the read and writeConfig methods to preserve the user's configuration.
The widget has a "current" item, that is visualized to differentiate it from others.
Definition at line 230 of file kurlbar.h.
Constructor & Destructor Documentation
KURLBar::KURLBar | ( | bool | useGlobalItems, | |
QWidget * | parent = 0 , |
|||
const char * | name = 0 , |
|||
WFlags | f = 0 | |||
) |
Constructs a KURLBar.
Set useGlobalItems
to true if you want to allow global/local item separation.
Definition at line 330 of file kurlbar.cpp.
KURLBar::~KURLBar | ( | ) |
Member Function Documentation
void KURLBar::activated | ( | const KURL & | url | ) | [signal] |
This signal is emitted when the user activated an item, e.g., by clicking on it.
bool KURLBar::addNewItem | ( | ) | [protected, virtual] |
Pops up a KURLBarItemDialog to let the user add a new item.
Uses editItem() to do the job.
- Returns:
- false if the user aborted the dialog and no item is added.
Definition at line 773 of file kurlbar.cpp.
void KURLBar::clear | ( | ) | [virtual] |
KURLBarItem * KURLBar::currentItem | ( | ) | const |
- Returns:
- the current KURLBarItem, or 0L if none.
- See also:
- setCurrentItem
Definition at line 564 of file kurlbar.cpp.
KURL KURLBar::currentURL | ( | ) | const |
- Returns:
- the url of the current item or an invalid url, if there is no current item.
- See also:
- currentItem
Definition at line 572 of file kurlbar.cpp.
bool KURLBar::editItem | ( | KURLBarItem * | item | ) | [protected, virtual] |
Pops up a KURLBarItemDialog to let the user edit the properties of item
.
Invoked e.g. by addNewItem(), when the user drops a url onto the bar or from the contextmenu.
- Returns:
- false if the user aborted the dialog and
item
is not changed.
Definition at line 786 of file kurlbar.cpp.
int KURLBar::iconSize | ( | ) | const [inline] |
- Returns:
- the default iconsize used for items inserted with insertItem. By default KIcon::SizeMedium
- See also:
- setIconSize
KURLBarItem * KURLBar::insertDynamicItem | ( | const KURL & | url, | |
const QString & | description, | |||
const QString & | icon = QString::null , |
|||
KIcon::Group | group = KIcon::Panel | |||
) | [virtual] |
Inserts a new dynamic item into the KURLBar and returns the created KURLBarItem.
url
the url of the item description
the description of the item (shown in the view) icon
an icon -- if empty, the default icon for the url will be used group
the icon-group for using icon-effects
- Since:
- 3.2
Definition at line 368 of file kurlbar.cpp.
KURLBarItem * KURLBar::insertItem | ( | const KURL & | url, | |
const QString & | description, | |||
bool | applicationLocal = true , |
|||
const QString & | icon = QString::null , |
|||
KIcon::Group | group = KIcon::Panel | |||
) | [virtual] |
Inserts a new item into the KURLBar and returns the created KURLBarItem.
url
the url of the item description
the description of the item (shown in the view) applicationLocal
whether this should be a global or a local item icon
an icon -- if empty, the default icon for the url will be used group
the icon-group for using icon-effects
Definition at line 358 of file kurlbar.cpp.
bool KURLBar::isImmutable | ( | ) | const [inline] |
bool KURLBar::isModified | ( | ) | const [inline] |
- Returns:
- true when the urlbar was modified by the user (e.g. by editing/adding/removing one or more entries). Will be reset to false after calling writeConfig().
bool KURLBar::isVertical | ( | ) | const [inline] |
KURLBarListBox* KURLBar::listBox | ( | ) | const [inline] |
QSize KURLBar::minimumSizeHint | ( | ) | const [virtual] |
Qt::Orientation KURLBar::orientation | ( | ) | const |
- Returns:
- the current orientation mode.
- See also:
- setOrientation
Definition at line 387 of file kurlbar.cpp.
void KURLBar::paletteChange | ( | const QPalette & | ) | [protected, virtual] |
Definition at line 451 of file kurlbar.cpp.
void KURLBar::readConfig | ( | KConfig * | config, | |
const QString & | itemGroup | |||
) | [virtual] |
Call this method to read a saved configuration from config
, inside the group itemGroup
.
All items in there will be restored. The reading of every item is delegated to the readItem() method.
Definition at line 578 of file kurlbar.cpp.
void KURLBar::readItem | ( | int | i, | |
KConfig * | config, | |||
bool | applicationLocal | |||
) | [virtual] |
Called from readConfig() to read the i'th from config
.
After reading a KURLBarItem is created and initialized with the read values (as well as the given applicationLocal
).
Definition at line 601 of file kurlbar.cpp.
void KURLBar::resizeEvent | ( | QResizeEvent * | e | ) | [protected, virtual] |
void KURLBar::setCurrentItem | ( | const KURL & | url | ) | [virtual, slot] |
Makes the item with the url url
the current item.
Does nothing if no item with that url is available.
- See also:
- currentItem
Definition at line 536 of file kurlbar.cpp.
void KURLBar::setIconSize | ( | int | size | ) | [virtual] |
Sets the default iconsize to be used for items inserted with insertItem.
By default KIcon::SizeMedium.
- See also:
- iconsize
Definition at line 422 of file kurlbar.cpp.
void KURLBar::setListBox | ( | KURLBarListBox * | view | ) | [virtual] |
Allows to set a custom KURLBarListBox.
Note: The previous listbox will be deleted. Items of the previous listbox will not be moved to the new box.
- See also:
- listBox
Definition at line 392 of file kurlbar.cpp.
void KURLBar::setOrientation | ( | Qt::Orientation | orient | ) | [virtual] |
The items can be arranged either vertically in one column or horizontally in one row.
- See also:
- orientation
Definition at line 376 of file kurlbar.cpp.
QSize KURLBar::sizeHint | ( | ) | const [virtual] |
- Returns:
- a proper sizehint, depending on the orientation and the number of items available.
Reimplemented in KFileSpeedBar.
Definition at line 467 of file kurlbar.cpp.
void KURLBar::slotContextMenuRequested | ( | QListBoxItem * | _item, | |
const QPoint & | pos | |||
) | [protected, virtual, slot] |
Reimplemented to show a contextmenu, allowing the user to add, edit or remove items, or change the iconsize.
Definition at line 713 of file kurlbar.cpp.
void KURLBar::slotDropped | ( | QDropEvent * | e | ) | [protected, virtual, slot] |
Called when a url was dropped onto the bar to show a KURLBarItemDialog.
Definition at line 695 of file kurlbar.cpp.
void KURLBar::slotSelected | ( | QListBoxItem * | item | ) | [protected, virtual, slot] |
Called when an item has been selected.
Emits the activated() signal.
Definition at line 525 of file kurlbar.cpp.
void KURLBar::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Definition at line 1029 of file kurlbar.cpp.
void KURLBar::writeConfig | ( | KConfig * | config, | |
const QString & | itemGroup | |||
) | [virtual] |
Call this method to save the current configuration into config
, inside the group iconGroup
.
The writeItem() method is used to save each item.
Definition at line 616 of file kurlbar.cpp.
void KURLBar::writeItem | ( | KURLBarItem * | item, | |
int | i, | |||
KConfig * | config, | |||
bool | global | |||
) | [virtual] |
Called from writeConfig() to save the KURLBarItem item
as the i'th entry in the config-object.
global
tell whether it should be saved in the global configuration or not (using KConfig::writeEntry( key, value, true, global ) ).
Definition at line 675 of file kurlbar.cpp.
Member Data Documentation
KURLBarItem* KURLBar::m_activeItem [protected] |
bool KURLBar::m_isImmutable [protected] |
bool KURLBar::m_isModified [protected] |
bool KURLBar::m_useGlobal [protected] |
The documentation for this class was generated from the following files: