kio
KFilterDev Class Reference
A class for reading and writing compressed data onto a device (e.g. More...
#include <kfilterdev.h>
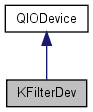
Public Member Functions | |
virtual bool | at (QIODevice::Offset) |
virtual QIODevice::Offset | at () const |
virtual bool | atEnd () const |
virtual void | close () |
virtual void | flush () |
virtual int | getch () |
KFilterDev (KFilterBase *filter, bool autoDeleteFilterBase=false) | |
virtual bool | open (int mode) |
virtual int | putch (int) |
virtual Q_LONG | readBlock (char *data, Q_ULONG maxlen) |
void | setOrigFileName (const QCString &fileName) |
void | setSkipHeaders () |
virtual QIODevice::Offset | size () const |
virtual int | ungetch (int) |
virtual Q_LONG | writeBlock (const char *data, Q_ULONG len) |
virtual | ~KFilterDev () |
Static Public Member Functions | |
static QIODevice * | createFilterDevice (KFilterBase *base, QFile *file) KDE_DEPRECATED |
static QIODevice * | device (QIODevice *inDevice, const QString &mimetype, bool autoDeleteInDevice) |
static QIODevice * | device (QIODevice *inDevice, const QString &mimetype) |
static QIODevice * | deviceForFile (const QString &fileName, const QString &mimetype=QString::null, bool forceFilter=false) |
Detailed Description
A class for reading and writing compressed data onto a device (e.g.file, but other usages are possible, like a buffer or a socket).
To simply read/write compressed files, see deviceForFile.
Definition at line 36 of file kfilterdev.h.
Constructor & Destructor Documentation
KFilterDev::KFilterDev | ( | KFilterBase * | filter, | |
bool | autoDeleteFilterBase = false | |||
) |
Constructs a KFilterDev for a given filter (e.g.
gzip, bzip2 etc.).
- Parameters:
-
filter the KFilterBase to use autoDeleteFilterBase when true this object will become the owner of filter
.
Definition at line 46 of file kfilterdev.cpp.
KFilterDev::~KFilterDev | ( | ) | [virtual] |
Destructs the KFilterDev.
Calls close() if the filter device is still open.
Definition at line 54 of file kfilterdev.cpp.
Member Function Documentation
bool KFilterDev::at | ( | QIODevice::Offset | pos | ) | [virtual] |
That one can be quite slow, when going back.
Use with care.
Definition at line 190 of file kfilterdev.cpp.
QIODevice::Offset KFilterDev::at | ( | ) | const [virtual] |
bool KFilterDev::atEnd | ( | ) | const [virtual] |
void KFilterDev::close | ( | ) | [virtual] |
Close after reading or writing.
If the KFilterBase's device was opened by open(), it will be closed.
Reimplemented from QIODevice.
Definition at line 149 of file kfilterdev.cpp.
QIODevice * KFilterDev::createFilterDevice | ( | KFilterBase * | base, | |
QFile * | file | |||
) | [static] |
Call this to create the appropriate filter device for base
working on file
.
The returned QIODevice has to be deleted after using.
- Deprecated:
- . Use deviceForFile instead. To be removed in KDE 3.0
Definition at line 66 of file kfilterdev.cpp.
QIODevice * KFilterDev::device | ( | QIODevice * | inDevice, | |
const QString & | mimetype, | |||
bool | autoDeleteInDevice | |||
) | [static] |
Creates an i/o device that is able to read from the QIODevice inDevice
, whether the data is compressed or not.
Available compression filters (gzip/bzip2 etc.) will automatically be used.
The compression filter to be used is determined mimetype
. Pass "application/x-gzip" or "application/x-bzip2" to use the corresponding decompression filter.
Warning: application/x-bzip2 may not be available. In that case 0 will be returned !
The returned QIODevice has to be deleted after using.
- Parameters:
-
inDevice input device. Won't be deleted if autoDeleteInDevice
= falsemimetype the mime type for the filter autoDeleteInDevice if true, inDevice
will be deleted automatically
- Returns:
- a QIODevice that filters the original stream. Must be deleted after using
- Since:
- 3.1
Definition at line 106 of file kfilterdev.cpp.
Creates an i/o device that is able to read from the QIODevice inDevice
, whether the data is compressed or not.
Available compression filters (gzip/bzip2 etc.) will automatically be used.
The compression filter to be used is determined mimetype
. Pass "application/x-gzip" or "application/x-bzip2" to use the corresponding decompression filter.
Warning: application/x-bzip2 may not be available. In that case 0 will be returned !
The returned QIODevice has to be deleted after using.
- Parameters:
-
inDevice input device, becomes owned by this device! Automatically deleted! mimetype the mime type for the filter
- Returns:
- a QIODevice that filters the original stream. Must be deleted after using
Definition at line 101 of file kfilterdev.cpp.
QIODevice * KFilterDev::deviceForFile | ( | const QString & | fileName, | |
const QString & | mimetype = QString::null , |
|||
bool | forceFilter = false | |||
) | [static] |
Creates an i/o device that is able to read from fileName
, whether it's compressed or not.
Available compression filters (gzip/bzip2 etc.) will automatically be used.
The compression filter to be used is determined from the fileName
if mimetype
is empty. Pass "application/x-gzip" or "application/x-bzip2" to force the corresponding decompression filter, if available.
Warning: application/x-bzip2 may not be available. In that case a QFile opened on the compressed data will be returned ! Use KFilterBase::findFilterByMimeType and code similar to what deviceForFile is doing, to better control what's happening.
The returned QIODevice has to be deleted after using.
- Parameters:
-
fileName the name of the file to filter mimetype the mime type of the file to filter, or QString::null if unknown forceFilter if true, the function will either find a compression filter, or return 0. If false, it will always return a QIODevice. If no filter is available it will return a simple QFile. This can be useful if the file is usable without a filter.
- Returns:
- if a filter has been found, the QIODevice for the filter. If the filter does not exist, the return value depends on
forceFilter
. The returned QIODevice has to be deleted after using.
Definition at line 81 of file kfilterdev.cpp.
void KFilterDev::flush | ( | ) | [virtual] |
int KFilterDev::getch | ( | ) | [virtual] |
bool KFilterDev::open | ( | int | mode | ) | [virtual] |
Open for reading or writing.
If the KFilterBase's device is not opened, it will be opened.
Reimplemented from QIODevice.
Definition at line 119 of file kfilterdev.cpp.
int KFilterDev::putch | ( | int | c | ) | [virtual] |
Q_LONG KFilterDev::readBlock | ( | char * | data, | |
Q_ULONG | maxlen | |||
) | [virtual] |
void KFilterDev::setOrigFileName | ( | const QCString & | fileName | ) |
For writing gzip compressed files only: set the name of the original file, to be used in the gzip header.
- Parameters:
-
fileName the name of the original file
Definition at line 472 of file kfilterdev.cpp.
void KFilterDev::setSkipHeaders | ( | ) |
Call this let this device skip the gzip headers when reading/writing.
This way KFilterDev (with gzip filter) can be used as a direct wrapper around zlib - this is used by KZip.
- Since:
- 3.1
Definition at line 477 of file kfilterdev.cpp.
QIODevice::Offset KFilterDev::size | ( | ) | const [virtual] |
int KFilterDev::ungetch | ( | int | ch | ) | [virtual] |
Q_LONG KFilterDev::writeBlock | ( | const char * | data, | |
Q_ULONG | len | |||
) | [virtual] |
The documentation for this class was generated from the following files: