kio
KZip Class Reference
This class implements a kioslave to access zip files from KDE. More...
#include <kzip.h>
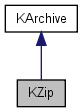
Public Types | |
enum | Compression { NoCompression = 0, DeflateCompression = 1 } |
enum | ExtraField { NoExtraField = 0, ModificationTime = 1, DefaultExtraField = 1 } |
Public Member Functions | |
Compression | compression () const |
virtual bool | doneWriting (uint size) |
ExtraField | extraField () const |
QString | fileName () |
KZip (QIODevice *dev) | |
KZip (const QString &filename) | |
bool | prepareWriting (const QString &name, const QString &user, const QString &group, uint size, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
virtual bool | prepareWriting (const QString &name, const QString &user, const QString &group, uint size) |
void | setCompression (Compression c) |
void | setExtraField (ExtraField ef) |
bool | writeData (const char *data, uint size) |
bool | writeFile (const QString &name, const QString &user, const QString &group, uint size, mode_t perm, time_t atime, time_t mtime, time_t ctime, const char *data) |
virtual bool | writeFile (const QString &name, const QString &user, const QString &group, uint size, const char *data) |
bool | writeSymLink (const QString &name, const QString &target, const QString &user, const QString &group, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
virtual | ~KZip () |
Protected Member Functions | |
virtual bool | closeArchive () |
virtual bool | openArchive (int mode) |
bool | prepareWriting_impl (const QString &name, const QString &user, const QString &group, uint size, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
virtual void | virtual_hook (int id, void *data) |
bool | writeData_impl (const char *data, uint size) |
virtual bool | writeDir (const QString &name, const QString &user, const QString &group) |
bool | writeSymLink_impl (const QString &name, const QString &target, const QString &user, const QString &group, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
Detailed Description
This class implements a kioslave to access zip files from KDE.You can use it in IO_ReadOnly or in IO_WriteOnly mode, and it behaves just as expected. It can also be used in IO_ReadWrite mode, in this case one can append files to an existing zip archive. When you append new files, which are not yet in the zip, it works as expected, i.e. the files are appended at the end. When you append a file, which is already in the file, the reference to the old file is dropped and the new one is added to the zip - but the old data from the file itself is not deleted, it is still in the zipfile. so when you want to have a small and garbage-free zipfile, just read the contents of the appended zip file and write it to a new one in IO_WriteOnly mode. This is especially important when you don't want to leak information of how intermediate versions of files in the zip were looking. For more information on the zip fileformat go to http://www.pkware.com/products/enterprise/white_papers/appnote.html A class for reading/writing zip archives.
- Since:
- 3.1
Definition at line 53 of file kzip.h.
Member Enumeration Documentation
enum KZip::Compression |
enum KZip::ExtraField |
Constructor & Destructor Documentation
KZip::KZip | ( | const QString & | filename | ) |
KZip::KZip | ( | QIODevice * | dev | ) |
Creates an instance that operates on the given device.
The device can be compressed (KFilterDev) or not (QFile, etc.).
- Warning:
- Do not assume that giving a QFile here will decompress the file, in case it's compressed!
- Parameters:
-
dev the device to access
KZip::~KZip | ( | ) | [virtual] |
Member Function Documentation
bool KZip::closeArchive | ( | ) | [protected, virtual] |
KZip::Compression KZip::compression | ( | ) | const |
The current compression mode that will be used for new files.
- Returns:
- the current compression mode
- See also:
- setCompression()
bool KZip::doneWriting | ( | uint | size | ) | [virtual] |
Write data to a file that has been created using prepareWriting().
- Parameters:
-
size the size of the file
- Returns:
- true if successful, false otherwise
Implements KArchive.
KZip::ExtraField KZip::extraField | ( | ) | const |
The current type of "extra field" that will be used for new files.
- Returns:
- the current type of "extra field"
- See also:
- setExtraField()
QString KZip::fileName | ( | ) | [inline] |
bool KZip::openArchive | ( | int | mode | ) | [protected, virtual] |
bool KZip::prepareWriting | ( | const QString & | name, | |
const QString & | user, | |||
const QString & | group, | |||
uint | size, | |||
mode_t | perm, | |||
time_t | atime, | |||
time_t | mtime, | |||
time_t | ctime | |||
) |
Here's another way of writing a file into an archive: Call prepareWriting, then call writeData() as many times as wanted then call doneWriting( totalSize ).
For tar.gz files, you need to know the size before hand, it is needed in the header! For zip files, size isn't used.
This method also allows some file metadata to be set. However, depending on the archive type not all metadata might be regarded.
- Parameters:
-
name the name of the file user the user that owns the file group the group that owns the file size the size of the file perm permissions of the file atime time the file was last accessed mtime modification time of the file ctime creation time of the file
- Since:
- 3.2
- Todo:
- TODO(BIC): make this virtual. For now use virtual hook.
Reimplemented from KArchive.
bool KZip::prepareWriting | ( | const QString & | name, | |
const QString & | user, | |||
const QString & | group, | |||
uint | size | |||
) | [virtual] |
Alternative method for writing: call prepareWriting(), then feed the data in small chunks using writeData(), and call doneWriting() when done.
- Parameters:
-
name can include subdirs e.g. path/to/the/file user the user owning the file group the group owning the file size unused argument
- Returns:
- true if successful, false otherwise
Implements KArchive.
void KZip::setCompression | ( | Compression | c | ) |
Call this before writeFile or prepareWriting, to define whether the next files to be written should be compressed or not.
- Parameters:
-
c the new compression mode
- See also:
- compression()
void KZip::setExtraField | ( | ExtraField | ef | ) |
Call this before writeFile or prepareWriting, to define what the next file to be written should have in its extra field.
- Parameters:
-
ef the type of "extra field"
- See also:
- extraField()
void KZip::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
bool KZip::writeData | ( | const char * | data, | |
uint | size | |||
) |
Write data to a file that has been created using prepareWriting().
- Parameters:
-
data a pointer to the data size the size of the chunk
- Returns:
- true if successful, false otherwise
Reimplemented from KArchive.
bool KZip::writeData_impl | ( | const char * | data, | |
uint | size | |||
) | [protected] |
bool KZip::writeDir | ( | const QString & | name, | |
const QString & | user, | |||
const QString & | group | |||
) | [protected, virtual] |
If an archive is opened for writing then you can add new directories using this function.
KArchive won't write one directory twice.
- Parameters:
-
name the name of the directory user the user that owns the directory group the group that owns the directory
- Todo:
- TODO(BIC): make this a thin wrapper around writeDir(name,user,group,perm,atime,mtime,ctime) or eliminate it
Implements KArchive.
bool KZip::writeFile | ( | const QString & | name, | |
const QString & | user, | |||
const QString & | group, | |||
uint | size, | |||
mode_t | perm, | |||
time_t | atime, | |||
time_t | mtime, | |||
time_t | ctime, | |||
const char * | data | |||
) |
If an archive is opened for writing then you can add a new file using this function.
If the file name is for example "mydir/test1" then the directory "mydir" is automatically appended first if that did not happen yet.
This method also allows some file metadata to be set. However, depending on the archive type not all metadata might be regarded.
- Parameters:
-
name the name of the file user the user that owns the file group the group that owns the file size the size of the file perm permissions of the file atime time the file was last accessed mtime modification time of the file ctime creation time of the file data the data to write ( size
bytes)
- Since:
- 3.2
- Todo:
- TODO(BIC): make virtual. For now use virtual hook
Reimplemented from KArchive.
bool KZip::writeFile | ( | const QString & | name, | |
const QString & | user, | |||
const QString & | group, | |||
uint | size, | |||
const char * | data | |||
) | [virtual] |
If an archive is opened for writing then you can add a new file using this function.
This method takes the whole data at once.
- Parameters:
-
name can include subdirs e.g. path/to/the/file user the user owning the file group the group owning the file size the size of the file data a pointer to the data
- Returns:
- true if successful, false otherwise
Reimplemented from KArchive.
bool KZip::writeSymLink | ( | const QString & | name, | |
const QString & | target, | |||
const QString & | user, | |||
const QString & | group, | |||
mode_t | perm, | |||
time_t | atime, | |||
time_t | mtime, | |||
time_t | ctime | |||
) |
Writes a symbolic link to the archive if the archive must be opened for writing.
- Parameters:
-
name name of symbolic link target target of symbolic link user the user that owns the directory group the group that owns the directory perm permissions of the directory atime time the file was last accessed mtime modification time of the file ctime creation time of the file
- Since:
- 3.2
- Todo:
- TODO(BIC) make virtual. For now it must be implemented by virtual_hook.
Reimplemented from KArchive.
The documentation for this class was generated from the following files: