kio
KArchive Class Reference
KArchive is a base class for reading and writing archives. More...
#include <karchive.h>
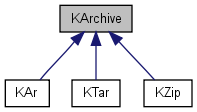
Classes | |
struct | PrepareWritingParams |
struct | WriteDataParams |
struct | WriteDirParams |
struct | WriteFileParams |
struct | WriteSymlinkParams |
Public Member Functions | |
bool | addLocalDirectory (const QString &path, const QString &destName) |
bool | addLocalFile (const QString &fileName, const QString &destName) |
virtual void | close () |
bool | closeSucceeded () const |
QIODevice * | device () const |
const KArchiveDirectory * | directory () const |
virtual bool | doneWriting (uint size)=0 |
bool | isOpened () const |
int | mode () const |
virtual bool | open (int mode) |
bool | prepareWriting (const QString &name, const QString &user, const QString &group, uint size, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
virtual bool | prepareWriting (const QString &name, const QString &user, const QString &group, uint size)=0 |
bool | writeData (const char *data, uint size) |
bool | writeDir (const QString &name, const QString &user, const QString &group, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
virtual bool | writeDir (const QString &name, const QString &user, const QString &group)=0 |
bool | writeFile (const QString &name, const QString &user, const QString &group, uint size, mode_t perm, time_t atime, time_t mtime, time_t ctime, const char *data) |
virtual bool | writeFile (const QString &name, const QString &user, const QString &group, uint size, const char *data) |
bool | writeSymLink (const QString &name, const QString &target, const QString &user, const QString &group, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
virtual | ~KArchive () |
Protected Types | |
enum | { VIRTUAL_WRITE_DATA = 1, VIRTUAL_WRITE_SYMLINK, VIRTUAL_WRITE_DIR, VIRTUAL_WRITE_FILE, VIRTUAL_PREPARE_WRITING } |
Protected Member Functions | |
virtual bool | closeArchive ()=0 |
KArchiveDirectory * | findOrCreate (const QString &path) |
KArchive (QIODevice *dev) | |
virtual bool | openArchive (int mode)=0 |
bool | prepareWriting_impl (const QString &name, const QString &user, const QString &group, uint size, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
virtual KArchiveDirectory * | rootDir () |
void | setDevice (QIODevice *dev) |
void | setRootDir (KArchiveDirectory *rootDir) |
virtual void | virtual_hook (int id, void *data) |
bool | writeData_impl (const char *data, uint size) |
bool | writeDir_impl (const QString &name, const QString &user, const QString &group, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
bool | writeFile_impl (const QString &name, const QString &user, const QString &group, uint size, mode_t perm, time_t atime, time_t mtime, time_t ctime, const char *data) |
bool | writeSymLink_impl (const QString &name, const QString &target, const QString &user, const QString &group, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
Detailed Description
KArchive is a base class for reading and writing archives.generic class for reading/writing archives
Definition at line 42 of file karchive.h.
Member Enumeration Documentation
anonymous enum [protected] |
- Enumerator:
-
VIRTUAL_WRITE_DATA VIRTUAL_WRITE_SYMLINK VIRTUAL_WRITE_DIR VIRTUAL_WRITE_FILE VIRTUAL_PREPARE_WRITING
Definition at line 326 of file karchive.h.
Constructor & Destructor Documentation
KArchive::KArchive | ( | QIODevice * | dev | ) | [protected] |
Base constructor (protected since this is a pure virtual class).
- Parameters:
-
dev the I/O device where the archive reads its data Note that this can be a file, but also a data buffer, a compression filter, etc.
Definition at line 75 of file karchive.cpp.
KArchive::~KArchive | ( | ) | [virtual] |
Definition at line 83 of file karchive.cpp.
Member Function Documentation
Writes a local directory into the archive, including all its contents, recursively.
Calls addLocalFile for each file to be added.
Since KDE 3.2 it will also add a path
that is a symbolic link to a directory. The symbolic link will be dereferenced and the content of the directory it is pointing to added recursively. However, symbolic links *under* path
will be stored as is.
- Parameters:
-
path full path to an existing local directory, to be added to the archive. destName the resulting name (or relative path) of the file in the archive.
Definition at line 200 of file karchive.cpp.
Writes a local file into the archive.
The main difference with writeFile, is that this method minimizes memory usage, by not loading the whole file into memory in one go.
If fileName
is a symbolic link, it will be written as is, i. e. it will not be resolved before.
- Parameters:
-
fileName full path to an existing local file, to be added to the archive. destName the resulting name (or relative path) of the file in the archive.
Definition at line 136 of file karchive.cpp.
void KArchive::close | ( | ) | [virtual] |
Closes the archive.
Inherited classes might want to reimplement closeArchive instead.
- See also:
- open
Definition at line 108 of file karchive.cpp.
virtual bool KArchive::closeArchive | ( | ) | [protected, pure virtual] |
bool KArchive::closeSucceeded | ( | ) | const |
Use to check if close had any problem.
- Returns:
- true if close succeded without problems
- Since:
- 3.5
Definition at line 124 of file karchive.cpp.
QIODevice* KArchive::device | ( | ) | const [inline] |
const KArchiveDirectory * KArchive::directory | ( | ) | const |
If an archive is opened for reading, then the contents of the archive can be accessed via this function.
- Returns:
- the directory of the archive
Definition at line 129 of file karchive.cpp.
virtual bool KArchive::doneWriting | ( | uint | size | ) | [pure virtual] |
Call doneWriting after writing the data.
- Parameters:
-
size the size of the file
- See also:
- prepareWriting()
KArchiveDirectory * KArchive::findOrCreate | ( | const QString & | path | ) | [protected] |
Ensures that path
exists, create otherwise.
This handles e.g. tar files missing directory entries, like mico-2.3.0.tar.gz :)
- Parameters:
-
path the path of the directory
- Returns:
- the directory with the given
path
Definition at line 383 of file karchive.cpp.
bool KArchive::isOpened | ( | ) | const [inline] |
Checks whether the archive is open.
- Returns:
- true if the archive is opened
Definition at line 83 of file karchive.h.
int KArchive::mode | ( | ) | const [inline] |
Returns the mode in which the archive was opened.
- Returns:
- the mode in which the archive was opened (IO_ReadOnly or IO_WriteOnly)
- See also:
- open()
Definition at line 90 of file karchive.h.
bool KArchive::open | ( | int | mode | ) | [virtual] |
Opens the archive for reading or writing.
Inherited classes might want to reimplement openArchive instead.
- Parameters:
-
mode may be IO_ReadOnly or IO_WriteOnly
- See also:
- close
Definition at line 91 of file karchive.cpp.
virtual bool KArchive::openArchive | ( | int | mode | ) | [protected, pure virtual] |
bool KArchive::prepareWriting | ( | const QString & | name, | |
const QString & | user, | |||
const QString & | group, | |||
uint | size, | |||
mode_t | perm, | |||
time_t | atime, | |||
time_t | mtime, | |||
time_t | ctime | |||
) |
Here's another way of writing a file into an archive: Call prepareWriting, then call writeData() as many times as wanted then call doneWriting( totalSize ).
For tar.gz files, you need to know the size before hand, it is needed in the header! For zip files, size isn't used.
This method also allows some file metadata to be set. However, depending on the archive type not all metadata might be regarded.
- Parameters:
-
name the name of the file user the user that owns the file group the group that owns the file size the size of the file perm permissions of the file atime time the file was last accessed mtime modification time of the file ctime creation time of the file
- Since:
- 3.2
- Todo:
- TODO(BIC): make this virtual. For now use virtual hook.
Reimplemented in KTar, and KZip.
Definition at line 235 of file karchive.cpp.
virtual bool KArchive::prepareWriting | ( | const QString & | name, | |
const QString & | user, | |||
const QString & | group, | |||
uint | size | |||
) | [pure virtual] |
Here's another way of writing a file into an archive: Call prepareWriting, then call writeData() as many times as wanted then call doneWriting( totalSize ).
For tar.gz files, you need to know the size before hand, since it is needed in the header. For zip files, size isn't used.
- Parameters:
-
name the name of the file user the user that owns the file group the group that owns the file size the size of the file
- Todo:
- TODO(BIC): make this a thin non-virtual wrapper around prepareWriting(name,user,group,size,perm,atime,mtime,ctime) or eliminate it.
KArchiveDirectory * KArchive::rootDir | ( | ) | [protected, virtual] |
Retrieves or create the root directory.
The default implementation assumes that openArchive() did the parsing, so it creates a dummy rootdir if none was set (write mode, or no '/' in the archive). Reimplement this to provide parsing/listing on demand.
- Returns:
- the root directory
Definition at line 368 of file karchive.cpp.
void KArchive::setDevice | ( | QIODevice * | dev | ) | [protected] |
void KArchive::setRootDir | ( | KArchiveDirectory * | rootDir | ) | [protected] |
void KArchive::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
bool KArchive::writeData | ( | const char * | data, | |
uint | size | |||
) |
Write data into the current file - to be called after calling prepareWriting.
- Todo:
- TODO(BIC) make virtual. For now virtual_hook allows reimplementing it.
Reimplemented in KZip.
Definition at line 353 of file karchive.cpp.
bool KArchive::writeData_impl | ( | const char * | data, | |
uint | size | |||
) | [protected] |
bool KArchive::writeDir | ( | const QString & | name, | |
const QString & | user, | |||
const QString & | group, | |||
mode_t | perm, | |||
time_t | atime, | |||
time_t | mtime, | |||
time_t | ctime | |||
) |
If an archive is opened for writing then you can add new directories using this function.
KArchive won't write one directory twice.
This method also allows some file metadata to be set. However, depending on the archive type not all metadata might be regarded.
- Parameters:
-
name the name of the directory user the user that owns the directory group the group that owns the directory perm permissions of the directory atime time the file was last accessed mtime modification time of the file ctime creation time of the file
- Since:
- 3.2
- Todo:
- TODO(BIC): make this virtual. For now use virtual hook
Reimplemented in KTar.
Definition at line 304 of file karchive.cpp.
virtual bool KArchive::writeDir | ( | const QString & | name, | |
const QString & | user, | |||
const QString & | group | |||
) | [pure virtual] |
If an archive is opened for writing then you can add new directories using this function.
KArchive won't write one directory twice.
- Parameters:
-
name the name of the directory user the user that owns the directory group the group that owns the directory
- Todo:
- TODO(BIC): make this a thin wrapper around writeDir(name,user,group,perm,atime,mtime,ctime) or eliminate it
bool KArchive::writeFile | ( | const QString & | name, | |
const QString & | user, | |||
const QString & | group, | |||
uint | size, | |||
mode_t | perm, | |||
time_t | atime, | |||
time_t | mtime, | |||
time_t | ctime, | |||
const char * | data | |||
) |
If an archive is opened for writing then you can add a new file using this function.
If the file name is for example "mydir/test1" then the directory "mydir" is automatically appended first if that did not happen yet.
This method also allows some file metadata to be set. However, depending on the archive type not all metadata might be regarded.
- Parameters:
-
name the name of the file user the user that owns the file group the group that owns the file size the size of the file perm permissions of the file atime time the file was last accessed mtime modification time of the file ctime creation time of the file data the data to write ( size
bytes)
- Since:
- 3.2
- Todo:
- TODO(BIC): make virtual. For now use virtual hook
Reimplemented in KZip.
Definition at line 259 of file karchive.cpp.
bool KArchive::writeFile | ( | const QString & | name, | |
const QString & | user, | |||
const QString & | group, | |||
uint | size, | |||
const char * | data | |||
) | [virtual] |
If an archive is opened for writing then you can add a new file using this function.
If the file name is for example "mydir/test1" then the directory "mydir" is automatically appended first if that did not happen yet.
- Parameters:
-
name the name of the file user the user that owns the file group the group that owns the file size the size of the file data the data to write ( size
bytes)
- Todo:
- TODO(BIC): make this a thin non-virtual wrapper around writeFile(name,user,group,size,perm,atime,mtime,ctime,data)
Reimplemented in KZip.
Definition at line 228 of file karchive.cpp.
bool KArchive::writeFile_impl | ( | const QString & | name, | |
const QString & | user, | |||
const QString & | group, | |||
uint | size, | |||
mode_t | perm, | |||
time_t | atime, | |||
time_t | mtime, | |||
time_t | ctime, | |||
const char * | data | |||
) | [protected] |
Definition at line 277 of file karchive.cpp.
bool KArchive::writeSymLink | ( | const QString & | name, | |
const QString & | target, | |||
const QString & | user, | |||
const QString & | group, | |||
mode_t | perm, | |||
time_t | atime, | |||
time_t | mtime, | |||
time_t | ctime | |||
) |
Writes a symbolic link to the archive if the archive must be opened for writing.
- Parameters:
-
name name of symbolic link target target of symbolic link user the user that owns the directory group the group that owns the directory perm permissions of the directory atime time the file was last accessed mtime modification time of the file ctime creation time of the file
- Since:
- 3.2
- Todo:
- TODO(BIC) make virtual. For now it must be implemented by virtual_hook.
Reimplemented in KTar, and KZip.
Definition at line 327 of file karchive.cpp.
The documentation for this class was generated from the following files: