kio
KTar Class Reference
A class for reading / writing (optionally compressed) tar archives. More...
#include <ktar.h>
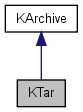
Public Member Functions | |
virtual bool | doneWriting (uint size) |
QString | fileName () |
KTar (QIODevice *dev) | |
KTar (const QString &filename, const QString &mimetype=QString::null) | |
bool | prepareWriting (const QString &name, const QString &user, const QString &group, uint size, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
virtual bool | prepareWriting (const QString &name, const QString &user, const QString &group, uint size) |
void | setOrigFileName (const QCString &fileName) |
bool | writeDir (const QString &name, const QString &user, const QString &group, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
virtual bool | writeDir (const QString &name, const QString &user, const QString &group) |
bool | writeSymLink (const QString &name, const QString &target, const QString &user, const QString &group, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
virtual | ~KTar () |
Protected Member Functions | |
virtual bool | closeArchive () |
virtual bool | openArchive (int mode) |
bool | prepareWriting_impl (const QString &name, const QString &user, const QString &group, uint size, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
virtual void | virtual_hook (int id, void *data) |
bool | writeDir_impl (const QString &name, const QString &user, const QString &group, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
bool | writeSymLink_impl (const QString &name, const QString &target, const QString &user, const QString &group, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
Detailed Description
A class for reading / writing (optionally compressed) tar archives.KTar allows you to read and write tar archives, including those that are compressed using gzip or bzip2.
Definition at line 40 of file ktar.h.
Constructor & Destructor Documentation
Creates an instance that operates on the given filename using the compression filter associated to given mimetype.
- Parameters:
-
filename is a local path (e.g. "/home/weis/myfile.tgz") mimetype "application/x-gzip" or "application/x-bzip2" Do not use application/x-tgz or similar - you only need to specify the compression layer ! If the mimetype is omitted, it will be determined from the filename.
KTar::KTar | ( | QIODevice * | dev | ) |
Creates an instance that operates on the given device.
The device can be compressed (KFilterDev) or not (QFile, etc.).
- Warning:
- Do not assume that giving a QFile here will decompress the file, in case it's compressed!
- Parameters:
-
dev the device to read from. If the source is compressed, the QIODevice must take care of decompression
KTar::~KTar | ( | ) | [virtual] |
Member Function Documentation
bool KTar::closeArchive | ( | ) | [protected, virtual] |
bool KTar::doneWriting | ( | uint | size | ) | [virtual] |
Call doneWriting after writing the data.
- Parameters:
-
size the size of the file
- See also:
- prepareWriting()
Implements KArchive.
QString KTar::fileName | ( | ) | [inline] |
bool KTar::openArchive | ( | int | mode | ) | [protected, virtual] |
bool KTar::prepareWriting | ( | const QString & | name, | |
const QString & | user, | |||
const QString & | group, | |||
uint | size, | |||
mode_t | perm, | |||
time_t | atime, | |||
time_t | mtime, | |||
time_t | ctime | |||
) |
Here's another way of writing a file into an archive: Call prepareWriting, then call writeData() as many times as wanted then call doneWriting( totalSize ).
For tar.gz files, you need to know the size before hand, it is needed in the header! For zip files, size isn't used.
This method also allows some file metadata to be set. However, depending on the archive type not all metadata might be regarded.
- Parameters:
-
name the name of the file user the user that owns the file group the group that owns the file size the size of the file perm permissions of the file atime time the file was last accessed mtime modification time of the file ctime creation time of the file
- Since:
- 3.2
- Todo:
- TODO(BIC): make this virtual. For now use virtual hook.
Reimplemented from KArchive.
bool KTar::prepareWriting | ( | const QString & | name, | |
const QString & | user, | |||
const QString & | group, | |||
uint | size | |||
) | [virtual] |
Here's another way of writing a file into an archive: Call prepareWriting, then call writeData() as many times as wanted then call doneWriting( totalSize ).
For tar.gz files, you need to know the size before hand, since it is needed in the header. For zip files, size isn't used.
- Parameters:
-
name the name of the file user the user that owns the file group the group that owns the file size the size of the file
- Todo:
- TODO(BIC): make this a thin non-virtual wrapper around prepareWriting(name,user,group,size,perm,atime,mtime,ctime) or eliminate it.
Implements KArchive.
void KTar::setOrigFileName | ( | const QCString & | fileName | ) |
Special function for setting the "original file name" in the gzip header, when writing a tar.gz file.
It appears when using in the "file" command, for instance. Should only be called if the underlying device is a KFilterDev!
- Parameters:
-
fileName the original file name
void KTar::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
bool KTar::writeDir | ( | const QString & | name, | |
const QString & | user, | |||
const QString & | group, | |||
mode_t | perm, | |||
time_t | atime, | |||
time_t | mtime, | |||
time_t | ctime | |||
) |
If an archive is opened for writing then you can add new directories using this function.
KArchive won't write one directory twice.
This method also allows some file metadata to be set. However, depending on the archive type not all metadata might be regarded.
- Parameters:
-
name the name of the directory user the user that owns the directory group the group that owns the directory perm permissions of the directory atime time the file was last accessed mtime modification time of the file ctime creation time of the file
- Since:
- 3.2
- Todo:
- TODO(BIC): make this virtual. For now use virtual hook
Reimplemented from KArchive.
If an archive is opened for writing then you can add new directories using this function.
KArchive won't write one directory twice.
- Parameters:
-
name the name of the directory user the user that owns the directory group the group that owns the directory
- Todo:
- TODO(BIC): make this a thin wrapper around writeDir(name,user,group,perm,atime,mtime,ctime) or eliminate it
Implements KArchive.
bool KTar::writeSymLink | ( | const QString & | name, | |
const QString & | target, | |||
const QString & | user, | |||
const QString & | group, | |||
mode_t | perm, | |||
time_t | atime, | |||
time_t | mtime, | |||
time_t | ctime | |||
) |
Writes a symbolic link to the archive if the archive must be opened for writing.
- Parameters:
-
name name of symbolic link target target of symbolic link user the user that owns the directory group the group that owns the directory perm permissions of the directory atime time the file was last accessed mtime modification time of the file ctime creation time of the file
- Since:
- 3.2
- Todo:
- TODO(BIC) make virtual. For now it must be implemented by virtual_hook.
Reimplemented from KArchive.
The documentation for this class was generated from the following files: