kio
KURLCompletion Class Reference
This class does completion of URLs including user directories (~user) and environment variables. More...
#include <kurlcompletion.h>
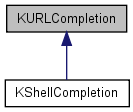
Public Types | |
enum | Mode { ExeCompletion = 1, FileCompletion, DirCompletion } |
Public Member Functions | |
virtual QString | dir () const |
virtual bool | isRunning () const |
KURLCompletion (Mode) | |
KURLCompletion () | |
virtual QString | makeCompletion (const QString &text) |
virtual Mode | mode () const |
QString | replacedPath (const QString &text) |
virtual bool | replaceEnv () const |
virtual bool | replaceHome () const |
virtual void | setDir (const QString &dir) |
virtual void | setMode (Mode mode) |
virtual void | setReplaceEnv (bool replace) |
virtual void | setReplaceHome (bool replace) |
virtual void | stop () |
virtual | ~KURLCompletion () |
Static Public Member Functions | |
static QString | replacedPath (const QString &text, bool replaceHome, bool replaceEnv=true) |
Protected Slots | |
void | slotEntries (KIO::Job *, const KIO::UDSEntryList &) |
void | slotIOFinished (KIO::Job *) |
Protected Member Functions | |
virtual void | customEvent (QCustomEvent *e) |
void | postProcessMatch (QString *match) const |
void | postProcessMatches (KCompletionMatches *matches) const |
void | postProcessMatches (QStringList *matches) const |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
This class does completion of URLs including user directories (~user) and environment variables.Remote URLs are passed to KIO.
Completion of a single URL
Definition at line 41 of file kurlcompletion.h.
Member Enumeration Documentation
enum KURLCompletion::Mode |
Determines how completion is done.
- ExeCompletion - executables in $PATH or with full path.
- FileCompletion - all files with full path or in dir(), URLs are listed using KIO.
- DirCompletion - Same as FileCompletion but only returns directories.
Definition at line 53 of file kurlcompletion.h.
Constructor & Destructor Documentation
KURLCompletion::KURLCompletion | ( | ) |
Constructs a KURLCompletion object in FileCompletion mode.
Definition at line 486 of file kurlcompletion.cpp.
KURLCompletion::KURLCompletion | ( | Mode | mode | ) |
This overloaded constructor allows you to set the Mode to ExeCompletion or FileCompletion without using setMode.
Default is FileCompletion.
Definition at line 492 of file kurlcompletion.cpp.
KURLCompletion::~KURLCompletion | ( | ) | [virtual] |
Member Function Documentation
void KURLCompletion::customEvent | ( | QCustomEvent * | e | ) | [protected, virtual] |
Definition at line 1343 of file kurlcompletion.cpp.
QString KURLCompletion::dir | ( | ) | const [virtual] |
Returns the current directory, as it was given in setDir.
- Returns:
- the current directory (path or URL)
Definition at line 532 of file kurlcompletion.cpp.
bool KURLCompletion::isRunning | ( | ) | const [virtual] |
Check whether asynchronous completion is in progress.
- Returns:
- true if asynchronous completion is in progress
Definition at line 658 of file kurlcompletion.cpp.
Finds completions to the given text.
Remote URLs are listed with KIO. For performance reasons, local files are listed with KIO only if KURLCOMPLETION_LOCAL_KIO is set. The completion is done asyncronously if KIO is used.
Returns the first match for user, environment, and local dir completion and QString::null for asynchronous completion (KIO or threaded).
- Parameters:
-
text the text to complete
- Returns:
- the first match, or QString::null if not found
Reimplemented in KShellCompletion.
Definition at line 572 of file kurlcompletion.cpp.
KURLCompletion::Mode KURLCompletion::mode | ( | ) | const [virtual] |
Returns the completion mode: exe or file completion (default FileCompletion).
- Returns:
- the completion mode
Definition at line 537 of file kurlcompletion.cpp.
void KURLCompletion::postProcessMatch | ( | QString * | match | ) | const [protected] |
void KURLCompletion::postProcessMatches | ( | KCompletionMatches * | matches | ) | const [protected] |
void KURLCompletion::postProcessMatches | ( | QStringList * | matches | ) | const [protected] |
QString KURLCompletion::replacedPath | ( | const QString & | text, | |
bool | replaceHome, | |||
bool | replaceEnv = true | |||
) | [static] |
For internal use only.
I'll let ossi add a real one to KShell :)
- Since:
- 3.2
Definition at line 1370 of file kurlcompletion.cpp.
Replaces username and/or environment variables, depending on the current settings and returns the filtered url.
Only works with local files, i.e. returns back the original string for non-local urls.
- Parameters:
-
text the text to process
- Returns:
- the path or URL resulting from this operation. If you want to convert it to a KURL, use KURL::fromPathOrURL.
Definition at line 1384 of file kurlcompletion.cpp.
bool KURLCompletion::replaceEnv | ( | ) | const [virtual] |
Checks whether environment variables are completed and whether they are replaced internally while finding completions.
Default is enabled.
- Returns:
- true if environment vvariables will be replaced
Definition at line 547 of file kurlcompletion.cpp.
bool KURLCompletion::replaceHome | ( | ) | const [virtual] |
Returns whether ~username is completed and whether ~username is replaced internally with the user's home directory while finding completions.
Default is enabled.
- Returns:
- true to replace tilde with the home directory
Definition at line 557 of file kurlcompletion.cpp.
void KURLCompletion::setDir | ( | const QString & | dir | ) | [virtual] |
Sets the current directory (used as base for completion).
Default = $HOME.
- Parameters:
-
dir the current directory, either as a path or URL
Definition at line 527 of file kurlcompletion.cpp.
void KURLCompletion::setMode | ( | Mode | mode | ) | [virtual] |
Changes the completion mode: exe or file completion.
- Parameters:
-
mode the new completion mode
Definition at line 542 of file kurlcompletion.cpp.
void KURLCompletion::setReplaceEnv | ( | bool | replace | ) | [virtual] |
Enables/disables completion and replacement (internally) of environment variables in URLs.
Default is enabled.
- Parameters:
-
replace true to replace environment variables
Definition at line 552 of file kurlcompletion.cpp.
void KURLCompletion::setReplaceHome | ( | bool | replace | ) | [virtual] |
Enables/disables completion of ~username and replacement (internally) of ~username with the user's home directory.
Default is enabled.
- Parameters:
-
replace true to replace tilde with the home directory
Definition at line 562 of file kurlcompletion.cpp.
void KURLCompletion::slotEntries | ( | KIO::Job * | , | |
const KIO::UDSEntryList & | entries | |||
) | [protected, slot] |
Definition at line 1154 of file kurlcompletion.cpp.
void KURLCompletion::slotIOFinished | ( | KIO::Job * | job | ) | [protected, slot] |
Definition at line 1229 of file kurlcompletion.cpp.
void KURLCompletion::stop | ( | ) | [virtual] |
void KURLCompletion::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
The documentation for this class was generated from the following files: