KTextEditor
#include <document.h>
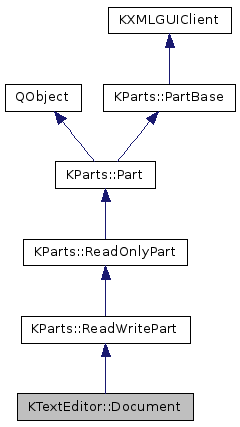
Public Member Functions | |
Document (QObject *parent=0) | |
virtual | ~Document () |
virtual View * | activeView () const =0 |
virtual QChar | character (const Cursor &position) const =0 |
virtual bool | clear ()=0 |
virtual View * | createView (QWidget *parent)=0 |
virtual bool | cursorInText (const Cursor &cursor) |
virtual Cursor | documentEnd () const =0 |
virtual const QString & | documentName () const =0 |
Range | documentRange () const |
virtual bool | documentReload ()=0 |
virtual bool | documentSave ()=0 |
virtual bool | documentSaveAs ()=0 |
virtual Editor * | editor ()=0 |
virtual const QString & | encoding () const =0 |
virtual bool | endEditing ()=0 |
Cursor | endOfLine (int line) const |
virtual QString | highlightingMode () const =0 |
virtual QStringList | highlightingModes () const =0 |
virtual QString | highlightingModeSection (int index) const =0 |
virtual bool | insertLine (int line, const QString &text)=0 |
virtual bool | insertLines (int line, const QStringList &text)=0 |
virtual bool | insertText (const Cursor &position, const QString &text, bool block=false)=0 |
virtual bool | insertText (const Cursor &position, const QStringList &text, bool block=false)=0 |
virtual bool | isEmpty () const |
virtual QString | line (int line) const =0 |
virtual int | lineLength (int line) const =0 |
virtual int | lines () const =0 |
virtual QString | mimeType ()=0 |
virtual QString | mode () const =0 |
virtual QStringList | modes () const =0 |
virtual QString | modeSection (int index) const =0 |
bool | openingError () const |
QString | openingErrorMessage () const |
virtual bool | removeLine (int line)=0 |
virtual bool | removeText (const Range &range, bool block=false)=0 |
virtual bool | replaceText (const Range &range, const QString &text, bool block=false) |
virtual bool | replaceText (const Range &range, const QStringList &text, bool block=false) |
virtual bool | setEncoding (const QString &encoding)=0 |
virtual bool | setHighlightingMode (const QString &name)=0 |
virtual bool | setMode (const QString &name)=0 |
void | setSuppressOpeningErrorDialogs (bool suppress) |
virtual bool | setText (const QString &text)=0 |
virtual bool | setText (const QStringList &text)=0 |
virtual bool | startEditing ()=0 |
bool | suppressOpeningErrorDialogs () const |
virtual QString | text () const =0 |
virtual QString | text (const Range &range, bool block=false) const =0 |
virtual QStringList | textLines (const Range &range, bool block=false) const =0 |
virtual int | totalCharacters () const =0 |
virtual const QList< View * > & | views () const =0 |
![]() | |
ReadWritePart (QObject *parent=0) | |
virtual | ~ReadWritePart () |
virtual bool | closeUrl (bool promptToSave) |
virtual bool | closeUrl () |
bool | isModified () const |
bool | isReadWrite () const |
virtual bool | queryClose () |
virtual bool | saveAs (const KUrl &url) |
virtual void | setModified (bool modified) |
virtual void | setReadWrite (bool readwrite=true) |
![]() | |
ReadOnlyPart (QObject *parent=0) | |
virtual | ~ReadOnlyPart () |
OpenUrlArguments | arguments () const |
BrowserExtension * | browserExtension () const |
bool | closeStream () |
bool | isProgressInfoEnabled () const |
bool | openStream (const QString &mimeType, const KUrl &url) |
void | setArguments (const OpenUrlArguments &arguments) |
void | setProgressInfoEnabled (bool show) |
void | showProgressInfo (bool show) |
KUrl | url () const |
bool | writeStream (const QByteArray &data) |
![]() | |
Part (QObject *parent=0) | |
virtual | ~Part () |
virtual void | embed (QWidget *parentWidget) |
virtual Part * | hitTest (QWidget *widget, const QPoint &globalPos) |
KIconLoader * | iconLoader () |
bool | isSelectable () const |
PartManager * | manager () const |
void | setAutoDeletePart (bool autoDeletePart) |
void | setAutoDeleteWidget (bool autoDeleteWidget) |
virtual void | setManager (PartManager *manager) |
virtual void | setSelectable (bool selectable) |
virtual QWidget * | widget () |
![]() | |
KXMLGUIClient () | |
KXMLGUIClient (KXMLGUIClient *parent) | |
virtual | ~KXMLGUIClient () |
QAction * | action (const char *name) const |
virtual QAction * | action (const QDomElement &element) const |
virtual KActionCollection * | actionCollection () const |
void | addStateActionDisabled (const QString &state, const QString &action) |
void | addStateActionEnabled (const QString &state, const QString &action) |
void | beginXMLPlug (QWidget *) |
QList< KXMLGUIClient * > | childClients () |
KXMLGUIBuilder * | clientBuilder () const |
virtual KComponentData | componentData () const |
virtual QDomDocument | domDocument () const |
void | endXMLPlug () |
KXMLGUIFactory * | factory () const |
StateChange | getActionsToChangeForState (const QString &state) |
void | insertChildClient (KXMLGUIClient *child) |
virtual QString | localXMLFile () const |
KXMLGUIClient * | parentClient () const |
void | plugActionList (const QString &name, const QList< QAction * > &actionList) |
void | prepareXMLUnplug (QWidget *) |
void | reloadXML () |
void | removeChildClient (KXMLGUIClient *child) |
void | replaceXMLFile (const QString &xmlfile, const QString &localxmlfile, bool merge=false) |
void | setClientBuilder (KXMLGUIBuilder *builder) |
void | setFactory (KXMLGUIFactory *factory) |
void | setXMLGUIBuildDocument (const QDomDocument &doc) |
void | unplugActionList (const QString &name) |
virtual QString | xmlFile () const |
QDomDocument | xmlguiBuildDocument () const |
Protected Member Functions | |
void | setOpeningError (bool errors) |
void | setOpeningErrorMessage (const QString &message) |
![]() | |
virtual bool | saveFile ()=0 |
virtual bool | saveToUrl () |
![]() | |
ReadOnlyPart (ReadOnlyPartPrivate &dd, QObject *parent) | |
void | abortLoad () |
virtual void | guiActivateEvent (GUIActivateEvent *event) |
bool | isLocalFileTemporary () const |
QString | localFilePath () const |
virtual bool | openFile () |
void | setLocalFilePath (const QString &localFilePath) |
void | setLocalFileTemporary (bool temp) |
void | setUrl (const KUrl &url) |
![]() | |
Part (PartPrivate &dd, QObject *parent) | |
virtual void | customEvent (QEvent *event) |
QWidget * | hostContainer (const QString &containerName) |
void | loadPlugins () |
virtual void | partActivateEvent (PartActivateEvent *event) |
virtual void | partSelectEvent (PartSelectEvent *event) |
virtual void | setWidget (QWidget *widget) |
![]() | |
void | loadStandardsXmlFile () |
virtual void | setComponentData (const KComponentData &componentData) |
virtual void | setDOMDocument (const QDomDocument &document, bool merge=false) |
virtual void | setLocalXMLFile (const QString &file) |
virtual void | setXML (const QString &document, bool merge=false) |
virtual void | setXMLFile (const QString &file, bool merge=false, bool setXMLDoc=true) |
virtual void | stateChanged (const QString &newstate, ReverseStateChange reverse=StateNoReverse) |
virtual void | virtual_hook (int id, void *data) |
Additional Inherited Members | |
![]() | |
enum | ReverseStateChange |
![]() | |
virtual bool | save () |
void | setModified () |
bool | waitSaveComplete () |
![]() | |
virtual bool | openUrl (const KUrl &url) |
![]() | |
static QString | findMostRecentXMLFile (const QStringList &files, QString &doc) |
![]() | |
void | slotWidgetDestroyed () |
![]() | |
KUrl | url |
Detailed Description
A KParts derived class representing a text document.
Topics:
Introduction
The Document class represents a pure text document providing methods to modify the content and create views. A document can have any number of views, each view representing the same content, i.e. all views are synchronized. Support for text selection is handled by a View and text format attributes by the Attribute class.
To load a document call KParts::ReadOnlyPart::openUrl(). To reload a document from a file call documentReload(), to save the document call documentSave() or documentSaveAs(). Whenever the modified state of the document changes the signal modifiedChanged() is emitted. Check the modified state with KParts::ReadWritePart::isModified(). Further signals are documentUrlChanged(). The encoding can be specified with setEncoding(), however this will only take effect on file reload and file save.
Text Manipulation
Get the whole content with text() and set new content with setText(). Call insertText() or insertLine() to insert new text or removeText() and removeLine() to remove content. Whenever the document's content changed the signal textChanged() is emitted. Additional signals are textInserted() and textRemoved(). Note, that the first line in the document is line 0.
If the editor part supports it, a document provides full undo/redo history. Text manipulation actions can be grouped together using startEditing() and endEditing(). All actions in between are grouped together to only one undo/redo action. Due to internal reference counting you can call startEditing() and endEditing() as often as you wish, but make sure you call endEditing() exactly as often as you call startEditing(), otherwise the reference counter gets confused.
Document Views
A View displays the document's content. As already mentioned, a document can have any number of views, all synchronized. Get a list of all views with views(). Only one of the views can be active (i.e. has focus), get it by using activeView(). Create a new view with createView(). Every time a new view is created the signal viewCreated() is emitted.
Document Extension Interfaces
A simple document represents text and provides text manipulation methods. However, a real text editor should support advanced concepts like session support, textsearch support, bookmark/general mark support etc. That is why the KTextEditor library provides several additional interfaces to extend a document's capabilities via multiple inheritance.
More information about interfaces for the document can be found in Document Extension Interfaces.
- See also
- KParts::ReadWritePart, KTextEditor::Editor, KTextEditor::View, KTextEditor::MarkInterface, KTextEditor::ModificationInterface, KTextEditor::SearchInterface, KTextEditor::SessionConfigInterface, KTextEditor::MovingInterface, KTextEditor::VariableInterface
Definition at line 111 of file document.h.
Constructor & Destructor Documentation
Document::Document | ( | QObject * | parent = 0 | ) |
Constructor.
Create a new document with parent
.
- Parameters
-
parent parent object
- See also
- Editor::createDocument()
Definition at line 127 of file document.cpp.
|
virtual |
Virtual destructor.
Definition at line 135 of file document.cpp.
Member Function Documentation
|
signal |
Warn anyone listening that the current document is about to close.
At this point all of the information is still accessible, such as the text, cursors and ranges.
Any modifications made to the document at this point will be lost.
- Parameters
-
document the document being closed
|
signal |
Warn anyone listening that the current document is about to reload.
At this point all of the information is still accessible, such as the text, cursors and ranges.
Any modifications made to the document at this point will be lost.
- Parameters
-
document the document being reloaded
|
pure virtual |
Return the view which currently has user focus, if any.
|
pure virtual |
|
pure virtual |
Remove the whole content of the document.
- Returns
- true on success, otherwise false
- See also
- removeText(), removeLine()
Create a new view attached to parent
.
- Parameters
-
parent parent widget
- Returns
- the new view
Checks whether the cursor
specifies a valid position in a document.
It can optionally be overridden by an implementation.
- Parameters
-
cursor which should be checked
- Returns
- true, if the cursor is valid, otherwise false
- See also
- DocumentCursor::isValid()
Definition at line 164 of file document.cpp.
|
pure virtual |
End position of the document.
- Returns
- The last column on the last line of the document
- See also
- all()
|
pure virtual |
Get this document's name.
The editor part should provide some meaningful name, like some unique "Untitled XYZ" for the document - without URL or basename for documents with url.
- Returns
- readable document name
|
signal |
This signal is emitted whenever the document
name changes.
- Parameters
-
document document which changed its name
- See also
- documentName()
|
inline |
A Range which encompasses the whole document.
- Returns
- A range from the start to the end of the document
Definition at line 435 of file document.h.
|
pure virtual |
Reload the current file.
The user will be prompted by the part on changes and more and can cancel this action if it can harm.
- Returns
- true if the reload has been done, otherwise false. If the document has no url set, it will just return false.
|
pure virtual |
Save the current file.
The user will be asked for a filename if needed and more.
- Returns
- true on success, i.e. the save has been done, otherwise false
|
pure virtual |
Save the current file to another location.
The user will be asked for a filename and more.
- Returns
- true on success, i.e. the save has been done, otherwise false
|
signal |
This signal should be emitted after a document has been saved to disk or for remote files uploaded.
saveAs should be set to true, if the operation is a save as operation
|
signal |
This signal is emitted whenever the document
URL changes.
- Parameters
-
document document which changed its URL
- See also
- KParts::ReadOnlyPart::url()
|
pure virtual |
Get the global editor object.
The editor part implementation must ensure that this object exists as long as any factory or document object exists.
- Returns
- global KTextEditor::Editor object
- See also
- KTextEditor::Editor
|
pure virtual |
Get the current chosen encoding.
The return value is an empty string, if the document uses the default encoding of the editor and no own special encoding.
- Returns
- current encoding of the document
- See also
- setEncoding()
|
pure virtual |
End an editing sequence.
- Returns
- true on success, otherwise false. Parts not supporting it should return false.
- See also
- startEditing() for more details
|
inline |
Get the end cursor position of line line
.
- Parameters
-
line line
- See also
- lineLength(), line()
Definition at line 464 of file document.h.
|
signal |
In conjunction with exclusiveEditStart(), signals that the document's content may be changed again without restriction.
- Since
- 4.5
|
signal |
Upon emission, the document's content may only be changed by the initiator of this signal until exclusiveEditEnd() is signalled.
It is, however, possible to listen to changes of the content.
Signalled e.g. on undo or redo.
- Since
- 4.5
|
pure virtual |
Return the name of the currently used mode.
- Returns
- name of the used mode
- See also
- highlightingModes(), setHighlightingMode()
|
signal |
Warn anyone listening that the current document's highlighting mode has changed.
- Parameters
-
document the document which's mode has changed
- See also
- setHighlightingMode()
|
pure virtual |
Return a list of the names of all possible modes.
- Returns
- list of mode names
- See also
- highlightingMode(), setHighlightingMode()
|
pure virtual |
Returns the name of the section for a highlight given its index in the highlight list (as returned by highlightModes()).
You can use this function to build a tree of the highlight names, organized in sections.
- Parameters
-
index the index of the highlight in the list returned by modes()
Insert line(s) at the given line number.
The newline character '\n' is treated as line delimiter, so it is possible to insert multiple lines. To append lines at the end of the document, use
- Parameters
-
line line where to insert the text text text which should be inserted
- Returns
- true on success, otherwise false
- See also
- insertText()
|
pure virtual |
Insert line(s) at the given line number.
The newline character '\n' is treated as line delimiter, so it is possible to insert multiple lines. To append lines at the end of the document, use
- Parameters
-
line line where to insert the text text text which should be inserted
- Returns
- true on success, otherwise false
- See also
- insertText()
|
pure virtual |
Insert text
at position
.
- Parameters
-
position position to insert the text text text to insert block insert this text as a visual block of text rather than a linear sequence
- Returns
- true on success, otherwise false
- See also
- setText(), removeText()
|
pure virtual |
Insert text
at position
.
- Parameters
-
position position to insert the text text text to insert block insert this text as a visual block of text rather than a linear sequence
- Returns
- true on success, otherwise false
- See also
- setText(), removeText()
|
virtual |
Returns if the document is empty.
Definition at line 190 of file document.cpp.
|
pure virtual |
Get a single text line.
- Parameters
-
line the wanted line
- Returns
- the requested line, or "" for invalid line numbers
- See also
- text(), lineLength()
|
pure virtual |
Get the length of a given line in characters.
- Parameters
-
line line to get length from
- Returns
- the number of characters in the line or -1 if the line was invalid
- See also
- line()
|
pure virtual |
Get the count of lines of the document.
- Returns
- the current number of lines in the document
- See also
- length()
|
pure virtual |
Get this document's mimetype.
- Returns
- mimetype
|
pure virtual |
|
signal |
Warn anyone listening that the current document's mode has changed.
- Parameters
-
document the document whose mode has changed
- See also
- setMode()
|
pure virtual |
|
pure virtual |
|
signal |
This signal is emitted whenever the document's
buffer changed from either state unmodified to modified or vice versa.
- Parameters
-
document document which changed its modified state
bool Document::openingError | ( | ) | const |
True, eg if the file for opening could not be read This doesn't have to handle the KPart job cancled cases.
Definition at line 148 of file document.cpp.
QString Document::openingErrorMessage | ( | ) | const |
Definition at line 152 of file document.cpp.
|
signal |
Emitted after the current document was reloaded.
At this point, some information might have been invalidated, like for example the editing history.
- Parameters
-
document the document that was reloaded.
- Since
- 4.6
|
pure virtual |
Remove line
from the document.
- Parameters
-
line line to remove
- Returns
- true on success, otherwise false
- See also
- removeText(), clear()
|
pure virtual |
Remove the text specified in range
.
- Parameters
-
range range of text to remove block set this to true to remove a text block on the basis of columns, rather than everything inside range
- Returns
- true on success, otherwise false
- See also
- setText(), insertText()
|
virtual |
Replace text from range
with specified text
.
- Parameters
-
range range of text to replace text text to replace with block replace text as a visual block of text rather than a linear sequence
- Returns
- true on success, otherwise false
- See also
- setText(), removeText(), insertText()
Definition at line 170 of file document.cpp.
|
virtual |
Replace text from range
with specified text
.
- Parameters
-
range range of text to replace text text to replace with block replace text as a visual block of text rather than a linear sequence
- Returns
- true on success, otherwise false
- See also
- setText(), removeText(), insertText()
Definition at line 180 of file document.cpp.
This signal is emitted whenever the readWrite state of a document changes.
Set the encoding for this document. This encoding will be used while loading and saving files, it will not affect the already existing content of the document, e.g. if the file has already been opened without the correct encoding, this will not fix it, you would for example need to trigger a reload for this.
- Parameters
-
encoding new encoding for the document, the name must be accepted by QTextCodec, if an empty encoding name is given, the part should fallback to its own default encoding, e.g. the system encoding or the global user settings
- Returns
- true on success, or false, if the encoding could not be set.
- See also
- encoding()
Set the current mode of the document by giving its name.
- Parameters
-
name name of the mode to use for this document
- Returns
- true on success, otherwise false
Set the current mode of the document by giving its name.
- Parameters
-
name name of the mode to use for this document
- Returns
- true on success, otherwise false
- See also
- mode(), modes(), modeChanged()
|
protected |
Definition at line 156 of file document.cpp.
|
protected |
Definition at line 160 of file document.cpp.
void Document::setSuppressOpeningErrorDialogs | ( | bool | suppress | ) |
by default dialogs should be displayed.
In any case (dialog shown or suppressed) openingErrors and openingErrorMessage should have meaningfull values
- Parameters
-
suppress true/false value if dialogs should be displayed
Definition at line 140 of file document.cpp.
Set the given text as new document content.
- Parameters
-
text new content for the document
- Returns
- true on success, otherwise false
- See also
- text()
|
pure virtual |
Set the given text as new document content.
- Parameters
-
text new content for the document
- Returns
- true on success, otherwise false
- See also
- text()
|
pure virtual |
Begin an editing sequence.
Edit commands during this sequence will be bunched together so that they represent a single undo command in the editor, and so that repaint events do not occur inbetween.
Your application should not return control to the event loop while it has an unterminated (i.e. no matching endEditing() call) editing sequence (result undefined) - so do all of your work in one go!
This call stacks, like the endEditing() calls, this means you can safely call it three times in a row for example if you call endEditing() three times, too, it internaly just does counting the running editing sessions.
If the texteditor part does not support these transactions, both calls just do nothing.
- Returns
- true on success, otherwise false. Parts not supporting it should return false
- See also
- endEditing()
bool Document::suppressOpeningErrorDialogs | ( | ) | const |
Definition at line 144 of file document.cpp.
|
pure virtual |
|
signal |
The document
emits this signal whenever its text changes.
- Parameters
-
document document which emitted this signal
- See also
- text(), textLine()
|
signal |
The document
emits this signal whenever the text in range oldRange
was removed and replaced with the text now in newRange, e.g.
the user selects text and pastes new text to replace the selection.
- Note
oldRange.start()
is guaranteed to equalnewRange.start()
.
- Parameters
-
document document which emitted this signal oldRange range that the text previously occupied newRange range that the changed text now occupies
- See also
- insertText(), insertLine(), removeText(), removeLine(), clear()
|
signal |
The document
emits this signal whenever the text in range oldRange
was removed and replaced with the text now in newRange, e.g.
the user selects text and pastes new text to replace the selection.
- Note
oldRange.start()
is guaranteed to equalnewRange.start()
.
- Parameters
-
document document which emitted this signal oldRange range that the text previously occupied oldText old text that has been replaced newRange range that the changed text now occupies
- See also
- insertText(), insertLine(), removeText(), removeLine(), clear()
|
signal |
The document
emits this signal whenever text was inserted.
The insertion occurred at range.start(), and new text now occupies up to range.end().
- Parameters
-
document document which emitted this signal range range that the newly inserted text occupies
- See also
- insertText(), insertLine()
|
pure virtual |
Get the document content within the given range
.
- Parameters
-
range the range of text to retrieve block Set this to true to receive text in a visual block, rather than everything inside range
.
- Returns
- the requested text lines, or QStringList() for invalid ranges. no end of line termination is included.
- See also
- setText()
|
signal |
The document
emits this signal whenever range
was removed, i.e.
text was removed.
- Parameters
-
document document which emitted this signal range range that the removed text previously occupied
- See also
- removeText(), removeLine(), clear()
|
signal |
The document
emits this signal whenever range
was removed, i.e.
text was removed.
- Parameters
-
document document which emitted this signal range range that the removed text previously occupied oldText the text that has been removed
- See also
- removeText(), removeLine(), clear()
|
pure virtual |
Get the count of characters in the document.
A TAB character counts as only one character.
- Returns
- the number of characters in the document
- See also
- lines()
|
signal |
This signal is emitted whenever the document
creates a new view
.
It should be called for every view to help applications / plugins to attach to the view
.
- Attention
- This signal should be emitted after the view constructor is completed, e.g. in the createView() method.
- Parameters
-
document the document for which a new view is created view the new view
- See also
- createView()
Returns the views pre-casted to KTextEditor::Views.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:42 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.