KTextEditor
#include <messageinterface.h>
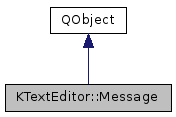
Public Types | |
enum | AutoHideMode { Immediate = 0, AfterUserInteraction } |
enum | MessagePosition { AboveView = 0, BelowView, TopInView, BottomInView } |
enum | MessageType { Positive = 0, Information, Warning, Error } |
Public Slots | |
void | setIcon (const QIcon &icon) |
void | setText (const QString &richtext) |
Signals | |
void | closed (KTextEditor::Message *message) |
void | iconChanged (const QIcon &icon) |
void | textChanged (const QString &text) |
Public Member Functions | |
Message (const QString &richtext, MessageType type=Message::Information) | |
virtual | ~Message () |
QList< QAction * > | actions () const |
void | addAction (QAction *action, bool closeOnTrigger=true) |
int | autoHide () const |
KTextEditor::Message::AutoHideMode | autoHideMode () const |
KTextEditor::Document * | document () const |
QIcon | icon () const |
MessageType | messageType () const |
MessagePosition | position () const |
int | priority () const |
void | setAutoHide (int autoHideTimer=0) |
void | setAutoHideMode (KTextEditor::Message::AutoHideMode mode) |
void | setDocument (KTextEditor::Document *document) |
void | setPosition (MessagePosition position) |
void | setPriority (int priority) |
void | setView (KTextEditor::View *view) |
void | setWordWrap (bool wordWrap) |
QString | text () const |
KTextEditor::View * | view () const |
bool | wordWrap () const |
Detailed Description
This class holds a Message to display in Views.
Introduction
The Message class holds the data used to display interactive message widgets in the editor. To post a message, use the MessageInterface.
Message Creation and Deletion
To create a new Message, use code like this:
Although discouraged in general, the text of the Message can be changed on the fly when it is already visible with setText().
Once you posted the Message through MessageInterface::postMessage(), the lifetime depends on the user interaction. The Message gets automatically deleted either if the user clicks a closing action in the message, or for instance if the document is reloaded.
If you posted a message but want to remove it yourself again, just delete the message. But beware of the following warning!
- Warning
- Always use QPointer<Message> to guard the message pointer from getting invalid, if you need to access the Message after you posted it.
Positioning
By default, the Message appears right above of the View. However, if desired, the position can be changed through setPosition(). For instance, the search-and-replace code in Kate Part shows the number of performed replacements in a message floating in the view. For further information, have a look at the enum MessagePosition.
Autohiding Messages
Messages can be shown for only a short amount of time by using the autohide feature. With setAutoHide() a timeout in milliseconds can be set after which the Message is automatically hidden. Further, use setAutoHideMode() to either trigger the autohide timer as soon as the widget is shown (AutoHideMode::Immediate), or only after user interaction with the view (AutoHideMode::AfterUserInteraction).
The default autohide mode is set to AutoHideMode::AfterUserInteraction. This way, it is unlikely the user misses a notification.
- See also
- MessageInterface
- Since
- KDE 4.11
Definition at line 91 of file messageinterface.h.
Member Enumeration Documentation
The AutoHideMode determines when to trigger the autoHide timer.
- See also
- setAutoHide(), autoHide()
Enumerator | |
---|---|
Immediate |
auto-hide is triggered as soon as the message is shown |
AfterUserInteraction |
auto-hide is triggered only after the user interacted with the view |
Definition at line 126 of file messageinterface.h.
Message position used to place the message either above or below of the KTextEditor::View.
Definition at line 115 of file messageinterface.h.
Message types used as visual indicator.
The message types match exactly the behavior of KMessageWidget::MessageType. For simple notifications either use Positive or Information.
Enumerator | |
---|---|
Positive |
positive information message |
Information |
information message type |
Warning |
warning message type |
Error |
error message type |
Definition at line 104 of file messageinterface.h.
Constructor & Destructor Documentation
KTextEditor::Message::Message | ( | const QString & | richtext, |
MessageType | type = Message::Information |
||
) |
Constructor for new messages.
- Parameters
-
type the message type, e.g. MessageType::Information richtext text to be displayed
Definition at line 41 of file messageinterface.cpp.
|
virtual |
Destructor.
Definition at line 55 of file messageinterface.cpp.
Member Function Documentation
Accessor to all actions, mainly used in the internal implementation to add the actions into the gui.
Definition at line 102 of file messageinterface.cpp.
Adds an action to the message.
By default (closeOnTrigger
= true), the action closes the message displayed in all Views. If closeOnTrigger
is false, the message is stays open.
The actions will be displayed in the order you added the actions.
To connect to an action, use the following code:
- Parameters
-
action action to be added closeOnTrigger when triggered, the message widget is closed
- Warning
- The added actions are deleted automatically. So do not delete the added actions yourself.
Definition at line 91 of file messageinterface.cpp.
int KTextEditor::Message::autoHide | ( | ) | const |
Returns the auto hide time in milliseconds.
Please refer to setAutoHide() for an explanation of the return value.
- See also
- setAutoHide(), autoHideMode()
Definition at line 112 of file messageinterface.cpp.
KTextEditor::Message::AutoHideMode KTextEditor::Message::autoHideMode | ( | ) | const |
Get the Message's autoHide mode.
The default mode is set to AutoHideMode::AfterUserInteraction.
- See also
- setAutoHideMode(), autoHide()
Definition at line 122 of file messageinterface.cpp.
|
signal |
This signal is emitted before the message is deleted.
Afterwards, this pointer is invalid.
Use the function document() to access the associated Document.
- Parameters
-
message closed/processed message
KTextEditor::Document * KTextEditor::Message::document | ( | ) | const |
Returns the document pointer this message was posted in.
This pointer is 0 as long as the message was not posted.
Definition at line 162 of file messageinterface.cpp.
QIcon KTextEditor::Message::icon | ( | ) | const |
Returns the icon of this message.
If the message has no icon set, a null icon is returned.
Definition at line 81 of file messageinterface.cpp.
|
signal |
This signal is emitted whenever setIcon() is called.
If the message was already sent through MessageInterface::postMessage(), the displayed icon changes on the fly.
- Note
- Change the icon on the fly with care, since changing the text may resize the notification widget, which may result in a distracting user experience.
- Parameters
-
icon new notification icon
- See also
- setIcon()
Message::MessageType KTextEditor::Message::messageType | ( | ) | const |
Returns the message type set in the constructor.
Definition at line 86 of file messageinterface.cpp.
Message::MessagePosition KTextEditor::Message::position | ( | ) | const |
Returns the desired message position of this message.
Definition at line 172 of file messageinterface.cpp.
int KTextEditor::Message::priority | ( | ) | const |
Returns the priority of the message.
Default is 0.
- See also
- setPriority()
Definition at line 142 of file messageinterface.cpp.
void KTextEditor::Message::setAutoHide | ( | int | autoHideTimer = 0 | ) |
Set the auto hide timer to autoHideTimer
milliseconds.
If autoHideTimer
< 0, auto hide is disabled. If autoHideTimer
= 0, auto hide is enabled and set to a sane default value of several seconds.
By default, auto hide is disabled.
- See also
- autoHide(), setAutoHideMode()
Definition at line 107 of file messageinterface.cpp.
void KTextEditor::Message::setAutoHideMode | ( | KTextEditor::Message::AutoHideMode | mode | ) |
Sets the autoHide mode to mode
.
The default mode is set to AutoHideMode::AfterUserInteraction.
- Parameters
-
mode autoHide mode
- See also
- autoHideMode(), setAutoHide()
Definition at line 117 of file messageinterface.cpp.
void KTextEditor::Message::setDocument | ( | KTextEditor::Document * | document | ) |
Set the document pointer to document
.
This is called by the implementation, as soon as you post a message through MessageInterface::postMessage(), so that you do not have to call this yourself.
- See also
- MessageInterface, document()
Definition at line 157 of file messageinterface.cpp.
|
slot |
Optionally set an icon for this notification.
The icon is shown next to the message text.
- Parameters
-
icon the icon to be displayed
Definition at line 75 of file messageinterface.cpp.
void KTextEditor::Message::setPosition | ( | Message::MessagePosition | position | ) |
Sets the position
either to AboveView or BelowView.
By default, the position is set to MessagePosition::AboveView.
- See also
- MessagePosition
Definition at line 167 of file messageinterface.cpp.
void KTextEditor::Message::setPriority | ( | int | priority | ) |
Set the priority of this message to priority
.
Messages with higher priority are shown first. The default priority is 0.
- See also
- priority()
Definition at line 137 of file messageinterface.cpp.
|
slot |
Sets the notification contents to text
.
If the message was already sent through MessageInterface::postMessage(), the displayed text changes on the fly.
- Note
- Change text on the fly with care, since changing the text may resize the notification widget, which may result in a distracting user experience.
- Parameters
-
richtext new notification text (rich text supported)
- See also
- textChanged()
Definition at line 67 of file messageinterface.cpp.
void KTextEditor::Message::setView | ( | KTextEditor::View * | view | ) |
Set the associated view of the message.
If view
is 0, the message is shown in all Views of the Document. If view
is given, i.e. non-zero, the message is shown only in this view.
- Parameters
-
view the associated view the message should be displayed in
Definition at line 147 of file messageinterface.cpp.
void KTextEditor::Message::setWordWrap | ( | bool | wordWrap | ) |
Enabled word wrap according to wordWrap
.
By default, auto wrap is disabled.
Word wrap is enabled automatically, if the Message's width is larger than the parent widget's width to avoid breaking the gui layout.
- See also
- wordWrap()
Definition at line 127 of file messageinterface.cpp.
QString KTextEditor::Message::text | ( | ) | const |
Returns the text set in the constructor.
Definition at line 62 of file messageinterface.cpp.
|
signal |
This signal is emitted whenever setText() is called.
If the message was already sent through MessageInterface::postMessage(), the displayed text changes on the fly.
- Note
- Change text on the fly with care, since changing the text may resize the notification widget, which may result in a distracting user experience.
- Parameters
-
text new notification text (rich text supported)
- See also
- setText()
KTextEditor::View * KTextEditor::Message::view | ( | ) | const |
This function returns the view you set by setView().
If setView() was not called, the return value is 0.
Definition at line 152 of file messageinterface.cpp.
bool KTextEditor::Message::wordWrap | ( | ) | const |
Check, whether word wrap is enabled or not.
- See also
- setWordWrap()
Definition at line 132 of file messageinterface.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:42 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.