KTextEditor
#include <smartrange.h>
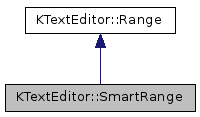
Public Types | |
enum | InsertBehavior { DoNotExpand = 0, ExpandLeft = 0x1, ExpandRight = 0x2 } |
Public Member Functions | |
virtual | ~SmartRange () |
virtual bool | isSmartRange () const |
SmartRange & | operator= (const SmartRange &rhs) |
SmartRange & | operator= (const Range &rhs) |
virtual SmartRange * | toSmartRange () const |
Position | |
The following functions provide access and manipulation of the range's position. | |
virtual void | setRange (const Range &range) |
SmartCursor & | smartStart () |
const SmartCursor & | smartStart () const |
SmartCursor & | smartEnd () |
const SmartCursor & | smartEnd () const |
virtual bool | confineToRange (const Range &range) |
virtual bool | expandToRange (const Range &range) |
Document-related functions | |
The following functions are provided for convenient access to the associated Document. | |
Document * | document () const |
virtual QStringList | text (bool block=false) const |
virtual bool | replaceText (const QStringList &text, bool block=false) |
virtual bool | removeText (bool block=false) |
Behavior | |
The following functions relate to the behavior of this SmartRange. | |
InsertBehaviors | insertBehavior () const |
void | setInsertBehavior (InsertBehaviors behavior) |
Tree structure | |
The following functions relate to the tree structure functionality. | |
SmartRange * | parentRange () const |
virtual void | setParentRange (SmartRange *r) |
bool | hasParent (SmartRange *parent) const |
int | depth () const |
SmartRange * | topParentRange () const |
const QList< SmartRange * > & | childRanges () const |
void | clearChildRanges () |
void | deleteChildRanges () |
void | clearAndDeleteChildRanges () |
SmartRange * | childBefore (const SmartRange *range) const |
SmartRange * | childAfter (const SmartRange *range) const |
SmartRange * | mostSpecificRange (const Range &input) const |
SmartRange * | firstRangeContaining (const Cursor &pos) const |
SmartRange * | deepestRangeContaining (const Cursor &pos, QStack< SmartRange * > *rangesEntered=0L, QStack< SmartRange * > *rangesExited=0L) const |
QList< SmartRange * > | deepestRangesContaining (const Cursor &pos) const |
int | overlapCount () const |
Arbitrary highlighting | |
The following functions relate to arbitrary highlighting capabilities. | |
Attribute::Ptr | attribute () const |
void | setAttribute (Attribute::Ptr attribute) |
Action binding | |
The following functions relate to action binding capabilities.
| |
void | associateAction (KAction *action) |
void | dissociateAction (KAction *action) |
const QList< KAction * > & | associatedActions () const |
void | clearAssociatedActions () |
Notification | |
The following functions allow for changes related to this range to be notified to 3rd party programs. | |
SmartRangeNotifier * | primaryNotifier () |
const QList< SmartRangeNotifier * > | notifiers () const |
void | addNotifier (SmartRangeNotifier *notifier) |
void | removeNotifier (SmartRangeNotifier *notifier) |
void | deletePrimaryNotifier () |
const QList< SmartRangeWatcher * > & | watchers () const |
void | addWatcher (SmartRangeWatcher *watcher) |
void | removeWatcher (SmartRangeWatcher *watcher) |
![]() | |
Range () | |
Range (const Cursor &start, const Cursor &end) | |
Range (const Cursor &start, int width) | |
Range (const Cursor &start, int endLine, int endColumn) | |
Range (int startLine, int startColumn, int endLine, int endColumn) | |
Range (const Range ©) | |
virtual | ~Range () |
Range | encompass (const Range &range) const |
Range | intersect (const Range &range) const |
virtual bool | isValid () const |
Range & | operator= (const Range &rhs) |
Cursor & | start () |
const Cursor & | start () const |
Cursor & | end () |
const Cursor & | end () const |
void | setBothLines (int line) |
void | setBothColumns (int column) |
void | setRange (const Cursor &start, const Cursor &end) |
bool | onSingleLine () const |
int | numberOfLines () const |
int | columnWidth () const |
bool | isEmpty () const |
bool | contains (const Range &range) const |
bool | contains (const Cursor &cursor) const |
bool | containsLine (int line) const |
bool | containsColumn (int column) const |
bool | overlaps (const Range &range) const |
bool | overlapsLine (int line) const |
bool | overlapsColumn (int column) const |
int | positionRelativeToCursor (const Cursor &cursor) const |
int | positionRelativeToLine (int line) const |
bool | boundaryAtCursor (const Cursor &cursor) const |
bool | boundaryOnLine (int line) const |
bool | boundaryOnColumn (int column) const |
Protected Member Functions | |
SmartRange (SmartCursor *start, SmartCursor *end, SmartRange *parent=0L, InsertBehaviors insertBehavior=DoNotExpand) | |
virtual void | checkFeedback () |
virtual SmartRangeNotifier * | createNotifier ()=0 |
virtual void | rangeChanged (Cursor *cursor, const Range &from) |
void | rebuildChildStructure () |
![]() | |
Range (Cursor *start, Cursor *end) | |
Additional Inherited Members | |
![]() | |
static Range | invalid () |
![]() | |
Cursor * | m_end |
Cursor * | m_start |
Detailed Description
A Range which is bound to a specific Document, and maintains its position.
A SmartRange is an extension of the basic Range class. It maintains its position in the document and provides extra functionality, including:
- convenience functions for accessing and manipulating the content of the associated document,
- adjusting behavior in response to text edits,
- forming a tree structure out of multiple SmartRanges,
- providing attribute information for the arbitrary highlighting extension,
- allowing KActions to be bound to the range (note: not currently implemented), and
- providing notification of changes to 3rd party software.
As a result of a smart range's close association with a document, and the processing that occurrs as a result, smart ranges may not be copied.
For simplicity of code, ranges always maintain their start position to be before or equal to their end position. Attempting to set either the start or end of the range beyond the respective end or start will result in both values being set to the specified position.
Hierarchical range-trees maintain specific relationships:
- When a child-range is changed, all parent-ranges are resized so the child-range fits in
- When a parent-range is changed, all child-ranges are resized so they fit in
This means that parent-ranges always completely contain all their child-ranges. However it may lead to unexpected range-changes from the perspective of your application, so keep this in mind.
The child-ranges of one smart-range are allowed to overlap each other. However overlaps should be omitted where possible, for performance-reasons, and because each range can be overlapped by max. 63 sibling-ranges while still rendering correctly.
Create a new SmartRange like this:
When finished with a SmartRange, simply delete it.
- See also
- Range, SmartRangeNotifier, SmartRangeWatcher, and SmartInterface
Definition at line 94 of file smartrange.h.
Member Enumeration Documentation
Determine how the range reacts to characters inserted immediately outside the range.
Definition at line 100 of file smartrange.h.
Constructor & Destructor Documentation
|
virtual |
Definition at line 194 of file smartrange.cpp.
|
protected |
Constructor for subclasses to utilise.
Protected to prevent direct instantiation.
- Note
- 3rd party developers: you do not (and should not) need to subclass the Smart* classes; instead, use the SmartInterface to create instances.
- Parameters
-
start the start cursor to use - ownership is taken end the end cursor to use - ownership is taken parent the parent range if this is a subrange of another range insertBehavior the behavior of this range when an insert happens immediately outside the range.
Definition at line 178 of file smartrange.cpp.
Member Function Documentation
void SmartRange::addNotifier | ( | SmartRangeNotifier * | notifier | ) |
Register a notifier to receive signals indicating change of state of this range.
NOTE: Make sure you call removeNotifier()
when deleting the notifier before the range.
- Parameters
-
notifier notifier to register. Ownership is not transferred.
Definition at line 918 of file smartrange.cpp.
void SmartRange::addWatcher | ( | SmartRangeWatcher * | watcher | ) |
Register a SmartRangeWatcher to receive calls indicating change of state of this range.
To finish receiving notifications, call removeWatcher().
NOTE: Make sure you call removeWachter()
when deleting the notifier before the range.
- Parameters
-
watcher the instance of a class which is to receive notifications about changes to this range.
Definition at line 891 of file smartrange.cpp.
void SmartRange::associateAction | ( | KAction * | action | ) |
Associate an action with this range.
The associated action(s) will be enabled when the caret enters the range, and disabled them on exit. The action is also added to the context menu when the mouse/caret is within an associated range.
- Parameters
-
action KAction to associate with this range
Definition at line 528 of file smartrange.cpp.
Access the list of currently associated KActions.
- Returns
- the list of associated actions
Definition at line 487 of file smartrange.h.
Attribute::Ptr SmartRange::attribute | ( | ) | const |
Gets the active Attribute for this range.
- Returns
- a pointer to the active attribute
Definition at line 636 of file smartrange.cpp.
|
protectedvirtual |
This routine is called when the range changes how much feedback it may need, eg. if it adds an action.
Definition at line 942 of file smartrange.cpp.
SmartRange * SmartRange::childAfter | ( | const SmartRange * | range | ) | const |
Find the child after range
, if any.
The order is determined by the range end-cursors.
- Parameters
-
range to seach forwards from
- Returns
- the range after
range
if one exists, otherwise null.
Definition at line 259 of file smartrange.cpp.
SmartRange * SmartRange::childBefore | ( | const SmartRange * | range | ) | const |
Find the child before range
, if any.
The order is determined by the range end-cursors.
- Parameters
-
range to seach backwards from
- Returns
- the range before
range
if one exists, otherwise null.
Definition at line 249 of file smartrange.cpp.
const QList< SmartRange * > & SmartRange::childRanges | ( | ) | const |
Get the ordered list of child ranges.
To insert a child range, simply set its parent to this range using setParentRange().
- Returns
- a list of child ranges.
Definition at line 244 of file smartrange.cpp.
void SmartRange::clearAndDeleteChildRanges | ( | ) |
Clears child ranges - i.e., clears the text that is covered by the ranges, and deletes the SmartRange objects.
Definition at line 582 of file smartrange.cpp.
void SmartRange::clearAssociatedActions | ( | ) |
Clears all associations between KActions and this range.
Definition at line 550 of file smartrange.cpp.
void SmartRange::clearChildRanges | ( | ) |
Clears child ranges - i.e., removes the text that is covered by the ranges.
The ranges themselves are not deleted.
- See also
- removeText()
Definition at line 567 of file smartrange.cpp.
|
virtual |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Reimplemented from KTextEditor::Range.
Definition at line 208 of file smartrange.cpp.
|
protectedpure virtual |
Called to request creation of a new SmartRangeNotifier for this object.
SmartRange * SmartRange::deepestRangeContaining | ( | const Cursor & | pos, |
QStack< SmartRange * > * | rangesEntered = 0L , |
||
QStack< SmartRange * > * | rangesExited = 0L |
||
) | const |
Finds the deepest range in the heirachy which contains position pos
.
Allows the caller to determine which ranges were entered and exited by providing pointers to QStack<SmartRange*>.
If child-ranges overlap in the given position, the first smallest one is returned.
- Parameters
-
pos the cursor position to use in searching rangesEntered provide a QStack<SmartRange*> here to find out which ranges were entered during the traversal. The top item was the first descended. rangesExited provide a QStack<SmartRange*> here to find out which ranges were exited during the traversal. The top item was the first exited.
- Returns
- the deepest range (from and including this range) which contains
pos
, or null if no ranges contain this position.
Definition at line 452 of file smartrange.cpp.
QList< SmartRange * > SmartRange::deepestRangesContaining | ( | const Cursor & | pos | ) | const |
Definition at line 426 of file smartrange.cpp.
void SmartRange::deleteChildRanges | ( | ) |
Deletes child ranges - i.e., deletes the SmartRange objects only.
The underlying text is not affected.
Definition at line 573 of file smartrange.cpp.
void SmartRange::deletePrimaryNotifier | ( | ) |
When finished with the primaryNotifier(), call this method to save memory by having the SmartRangeNotifier deleted.
- Note
- If a notifier was first registered via addNotifier() rather than created inside primaryNotifier(), this method will delete that notifier. Text editor implementations should not use notifiers for internal purposes, instead use watchers (faster and has documentation to this effect)
Definition at line 932 of file smartrange.cpp.
|
inline |
Calculate the current depth of this range.
- Returns
- the depth of this range, where 0 is no parent, 1 is one parent, etc.
Definition at line 310 of file smartrange.h.
void SmartRange::dissociateAction | ( | KAction * | action | ) |
Remove the association with an action from this range; it will no longer be managed.
- Parameters
-
action KAction to dissociate from this range
Definition at line 543 of file smartrange.cpp.
Document * SmartRange::document | ( | ) | const |
Retrieve the document associated with this SmartRange.
- Returns
- a pointer to the associated document
Definition at line 523 of file smartrange.cpp.
|
virtual |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Reimplemented from KTextEditor::Range.
Definition at line 220 of file smartrange.cpp.
SmartRange * SmartRange::firstRangeContaining | ( | const Cursor & | pos | ) | const |
Finds the first child range which contains position pos
.
- Parameters
-
pos the cursor position to use in searching
- Returns
- the most shallow range (from and including this range) which contains
pos
Definition at line 402 of file smartrange.cpp.
bool SmartRange::hasParent | ( | SmartRange * | parent | ) | const |
Determine whether parent is a parent of this range.
- Parameters
-
parent range to check to see if it is a parent of this range.
- Returns
- true if parent is in the parent heirachy, otherwise false.
Definition at line 875 of file smartrange.cpp.
SmartRange::InsertBehaviors SmartRange::insertBehavior | ( | ) | const |
Returns how this range reacts to characters inserted immediately outside the range.
- Returns
- the current insert behavior.
Definition at line 556 of file smartrange.cpp.
|
virtual |
Returns that this range is a SmartRange.
Reimplemented from KTextEditor::Range.
Definition at line 865 of file smartrange.cpp.
SmartRange * SmartRange::mostSpecificRange | ( | const Range & | input | ) | const |
Finds the most specific range in a heirachy for the given input range (ie.
the smallest range which wholly contains the input range)
In case of overlaps, the smallest containing range is chosen, if there are multiple of the same size, then the first one.
- Parameters
-
input the range to use in searching
- Returns
- the deepest range which contains
input
Definition at line 363 of file smartrange.cpp.
const QList< SmartRangeNotifier * > SmartRange::notifiers | ( | ) | const |
Returns a list of notifiers which are receiving signals indicating change of state of this range.
These notifiers may be receiving signals from other ranges as well.
Definition at line 913 of file smartrange.cpp.
|
inline |
Assignment operator.
Assigns the current position of the provided range, rhs
, only; does not assign watchers, notifiers, behavior etc.
- Note
- The assignment will be performed even if the provided range belongs to another Document.
- Parameters
-
rhs range to assign to this range.
- Returns
- a reference to this range, after assignment has occurred.
- See also
- setRange()
Definition at line 591 of file smartrange.h.
|
inline |
Assignment operator.
Assigns the current position of the provided range, rhs
.
- Parameters
-
rhs range to assign to this range.
- Returns
- a reference to this range, after assignment has occurred.
- See also
- setRange()
Definition at line 603 of file smartrange.h.
int SmartRange::overlapCount | ( | ) | const |
Returns the count of ranges within the parent-range that end behind this range, and that overlap this range.
Definition at line 421 of file smartrange.cpp.
|
inline |
Returns this range's parent range, if one exists.
At all times, this range will be contained within parentRange().
- Returns
- a pointer to the current parent range
Definition at line 277 of file smartrange.h.
SmartRangeNotifier * SmartRange::primaryNotifier | ( | ) |
Connect to a notifier to receive signals indicating change of state of this range.
This function creates a notifier if none is already bound to this range; if one has already been assigned this will return the first notifier.
If you have finished with notifications for a reasonable period of time you can save memory by calling deleteNotifier().
Definition at line 905 of file smartrange.cpp.
Notify this range that one or both of the cursors' position has changed directly.
- Parameters
-
cursor the cursor that changed. If null, both cursors have changed. from the previous position of this range
Reimplemented from KTextEditor::Range.
Definition at line 687 of file smartrange.cpp.
|
protected |
Is called after child-ranges have changed internally without the rangeChanged() notification, for example after translations.
It rebuilds the child-structure, so it is consistent again.
Re-order
Update overlap
Definition at line 660 of file smartrange.cpp.
void SmartRange::removeNotifier | ( | SmartRangeNotifier * | notifier | ) |
Deregister a notifier and no longer deliver signals indicating change of state of this range.
- Parameters
-
notifier notifier to deregister.
Definition at line 926 of file smartrange.cpp.
Remove text contained within this range.
The range itself will not be deleted.
- Parameters
-
block specify whether the text should be deleted from the range's visual block, rather than all the text within the range.
Definition at line 651 of file smartrange.cpp.
void SmartRange::removeWatcher | ( | SmartRangeWatcher * | watcher | ) |
Stop delivery of notifications to a SmartRangeWatcher.
- Parameters
-
watcher the watcher that no longer wants notifications.
Definition at line 899 of file smartrange.cpp.
|
virtual |
Replace text in this range with text
.
- Parameters
-
text text to use as a replacement block insert this text as a visual block of text rather than a linear sequence
- Returns
- true on success, otherwise false
Definition at line 646 of file smartrange.cpp.
void SmartRange::setAttribute | ( | Attribute::Ptr | attribute | ) |
Sets the currently active attribute for this range.
- Parameters
-
attribute Attribute to assign to this range. If null, simply removes the previous Attribute.
- Note
- SmartInterface::addHighlightToDocument must be called with the top-range before the highlighting can work.
Definition at line 620 of file smartrange.cpp.
void SmartRange::setInsertBehavior | ( | InsertBehaviors | behavior | ) |
Determine how the range should react to characters inserted immediately outside the range.
- Todo:
- does this need a custom function to enable determining of the behavior based on the text that is inserted / deleted?
- Parameters
-
behavior the insertion behavior to use for future edits
- See also
- InsertBehavior
Definition at line 561 of file smartrange.cpp.
|
virtual |
Set this range's parent range.
At all times, this range will be contained within parentRange(). So, if it is outside of the new parent to begin with, it will be expanded automatically.
When being inserted into the parent range, the parent range will be fit in between any other pre-existing child ranges, and may resize them so as not to overlap. However, once insertion has occurred, changing this range directly will only resize the others, it will not change the order of the ranges. To change the order, unset the parent range, change the range, and re-set the parent range.
- Parameters
-
r range to become the new parent of this range
Definition at line 594 of file smartrange.cpp.
|
virtual |
Set the start and end cursors to range.start() and range.end() respectively.
- Parameters
-
range range to assign to this range
This function also provides any required adjustment of parent and child ranges, and notification of the change if required.
Reimplemented from KTextEditor::Range.
Definition at line 232 of file smartrange.cpp.
|
inline |
Get the end point of this range.
This version returns a casted version of end(), as SmartRanges always use SmartCursors as the start() and end().
- Returns
- a reference to the end of this range.
Definition at line 163 of file smartrange.h.
|
inline |
Get the end point of this range.
This version returns a casted version of end(), as SmartRanges always use SmartCursors as the start() and end().
- Returns
- a const reference to the end of this range.
Definition at line 173 of file smartrange.h.
|
inline |
Get the start point of this range.
This version returns a casted version of start(), as SmartRanges always use SmartCursors as the start() and end().
- Returns
- a reference to the start of this range.
Definition at line 143 of file smartrange.h.
|
inline |
Get the start point of this range.
This version returns a casted version of start(), as SmartRanges always use SmartCursors as the start() and end().
- Returns
- a const reference to the start of this range.
Definition at line 153 of file smartrange.h.
|
virtual |
Retrieve the text which is contained within this range.
- Parameters
-
block specify whether the text should be returned from the range's visual block, rather than all the text within the range.
Definition at line 641 of file smartrange.cpp.
|
inline |
Returns the range's top parent range, or this range if there are no parents.
- Returns
- a pointer to the top parent range
Definition at line 318 of file smartrange.h.
|
virtual |
Returns this range as a SmartRange, if it is one.
Reimplemented from KTextEditor::Range.
Definition at line 870 of file smartrange.cpp.
const QList< SmartRangeWatcher * > & SmartRange::watchers | ( | ) | const |
Returns a list of registered SmartRangeWatchers.
- Note
- this function may return watchers internal to the text editor's implementation, eg. in the case of arbitrary highlighting and kate part. Removing these watchers with removeWatcher() will result in malfunction.
Definition at line 886 of file smartrange.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:42 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.