Kate
#include <katetextfolding.h>
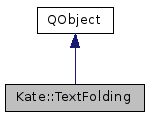
Public Types | |
enum | FoldingRangeFlag { Persistent = 0x1, Folded = 0x2 } |
Public Slots | |
void | clear () |
Signals | |
void | foldingRangesChanged () |
Public Member Functions | |
TextFolding (TextBuffer &buffer) | |
~TextFolding () | |
QString | debugDump () const |
void | debugPrint (const QString &title) const |
void | ensureLineIsVisible (int line) |
QVariantList | exportFoldingRanges () const |
KTextEditor::Range | foldingRange (qint64 id) const |
QVector< QPair< qint64, FoldingRangeFlags > > | foldingRangesStartingOnLine (int line) const |
bool | foldRange (qint64 id) |
void | importFoldingRanges (const QVariantList &folds) |
bool | isLineVisible (int line, qint64 *foldedRangeId=0) const |
int | lineToVisibleLine (int line) const |
qint64 | newFoldingRange (const KTextEditor::Range &range, FoldingRangeFlags flags=FoldingRangeFlags()) |
bool | unfoldRange (qint64 id, bool remove=false) |
int | visibleLines () const |
int | visibleLineToLine (int visibleLine) const |
Detailed Description
Class representing the folding information for a TextBuffer.
The interface allows to arbitrary fold given regions of a buffer as long as they are well nested. Multiple instances of this class can exist for the same buffer.
Definition at line 42 of file katetextfolding.h.
Member Enumeration Documentation
Folding state of a range.
Enumerator | |
---|---|
Persistent |
Range is persistent, e.g. it should not auto-delete after unfolding! |
Folded |
Range is folded away. |
Definition at line 60 of file katetextfolding.h.
Constructor & Destructor Documentation
Kate::TextFolding::TextFolding | ( | TextBuffer & | buffer | ) |
Create folding object for given buffer.
- Parameters
-
buffer text buffer we want to provide folding info for
connect needed signals from buffer
Definition at line 47 of file katetextfolding.cpp.
Kate::TextFolding::~TextFolding | ( | ) |
Cleanup.
only delete the folding ranges, the folded ranges and mapped ranges are the same objects
Definition at line 58 of file katetextfolding.cpp.
Member Function Documentation
|
slot |
Clear the complete folding.
This is automatically triggered if the buffer is cleared.
reset counter
no ranges, no work
assert all stuff is consistent and return!
cleanup
folding changed!
Definition at line 66 of file katetextfolding.cpp.
QString Kate::TextFolding::debugDump | ( | ) | const |
Dump folding state as string, for unit testing and debugging.
- Returns
- current state as text
dump toplevel ranges recursively
Definition at line 535 of file katetextfolding.cpp.
void Kate::TextFolding::debugPrint | ( | const QString & | title | ) | const |
Print state to stdout for testing.
Definition at line 543 of file katetextfolding.cpp.
void Kate::TextFolding::ensureLineIsVisible | ( | int | line | ) |
Ensure that a given line will be visible.
Potentially unfold recursively all folds hiding this line, else just returns.
- Parameters
-
line line to make visible
skip if nothing folded
while not visible, unfold
id should be valid!
unfold shall work!
Definition at line 313 of file katetextfolding.cpp.
QVariantList Kate::TextFolding::exportFoldingRanges | ( | ) | const |
Return the current known folding ranges a QVariantList to store in configs.
- Returns
- current folds as variant list
Definition at line 874 of file katetextfolding.cpp.
KTextEditor::Range Kate::TextFolding::foldingRange | ( | qint64 | id | ) | const |
Returns the folding range associated with id
.
If id
is not a valid id, the returned range matches KTextEditor::Range::invalid().
- Note
- This works for either persistend ranges or folded ranges. Note, that the highlighting does not add folds unless text is folded.
- Returns
- the folding range for
id
Definition at line 159 of file katetextfolding.cpp.
|
signal |
If the folding state of existing ranges changes or ranges are added/removed, this signal is emitted.
QVector< QPair< qint64, TextFolding::FoldingRangeFlags > > Kate::TextFolding::foldingRangesStartingOnLine | ( | int | line | ) | const |
Queries which folding ranges start at the given line and returns the id + flags for all of them.
Very fast if nothing is folded, else binary search.
- Parameters
-
line line to query starting folding ranges
- Returns
- vector of id's + flags
results vector
recursively do binary search
return found results
Definition at line 476 of file katetextfolding.cpp.
Fold the given range.
- Parameters
-
id id of the range to fold
- Returns
- success
try to find the range, else bail out
already folded? nothing to do
fold and be done
Definition at line 168 of file katetextfolding.cpp.
void Kate::TextFolding::importFoldingRanges | ( | const QVariantList & | folds | ) |
Import the folding ranges given as a QVariantList like read from configs.
- Parameters
-
folds list of folds to import
try to create all folding ranges
get map
construct range start/end
get flags
create folding range
Definition at line 905 of file katetextfolding.cpp.
Query if a given line is visible.
Very fast, if nothing is folded, else does binary search log(n) for n == number of folded ranges
- Parameters
-
line line to query foldedRangeId if the line is not visible and that pointer is not 0, will be filled with id of range hiding the line or -1
- Returns
- is that line visible?
skip if nothing folded
search upper bound, index to item with start line higher than our one
check if we overlap with the range in front of us
fill in folded range id, if needed
visible == !hidden
Definition at line 281 of file katetextfolding.cpp.
int Kate::TextFolding::lineToVisibleLine | ( | int | line | ) | const |
Convert a text buffer line to a visible line number.
Very fast, if nothing is folded, else walks over all folded regions O(n) for n == number of folded ranges
- Parameters
-
line line index in the text buffer
- Returns
- index in visible lines
valid input needed!
start with identity
skip if nothing folded or first line
walk over all folded ranges until we reach the line keep track of seen visible lines, for the case we want to convert a hidden line!
abort if we reach our line!
count visible lines
we might be contained in the region, then we return last visible line
subtrace folded lines
be done, assert we did no trash
Definition at line 366 of file katetextfolding.cpp.
qint64 Kate::TextFolding::newFoldingRange | ( | const KTextEditor::Range & | range, |
FoldingRangeFlags | flags = FoldingRangeFlags() |
||
) |
Create a new folding range.
- Parameters
-
range folding range flags initial flags for the new folding range
- Returns
- on success, id of new range >= 0, else -1, we return no pointer as folding ranges might be auto-deleted internally! the ids are stable for one Kate::TextFolding, e.g. you can rely in unit tests that you get 0,1,.... for successfully created ranges!
sort out invalid and empty ranges that makes no sense, they will never grow again!
create new folding region that we want to insert this will internally create moving cursors!
the construction of the text cursors might have invalidated this check and bail out if that happens bail out, too, if it can't be inserted!
cleanup and be done
set id, catch overflows, even if they shall not happen
remember the range
update our folded ranges vector!
emit that something may have changed do that only, if updateFoldedRangesForNewRange did not already do the job!
all went fine, newRange is now registered internally!
Definition at line 99 of file katetextfolding.cpp.
Unfold the given range.
In addition it can be forced to remove the region, even if it is persistent.
- Parameters
-
id id of the range to unfold remove should the range be removed from the folding after unfolding? ranges that are not persistent auto-remove themself on unfolding
- Returns
- success
try to find the range, else bail out
nothing to do? range is already unfolded and we need not to remove it!
do we need to delete the range?
first: remove the range, if forced or non-persistent!
remove from outside visible mapping!
remove from folding vectors! FIXME: OPTIMIZE
insert our nested ranges and reparent them
else just transfer elements
second: unfold the range, if needed!
emit that something may have changed do that only, if updateFoldedRangesForRemoveRange did not already do the job!
really delete the range, if needed!
clear ranges first, they got moved!
be done ;)
Definition at line 191 of file katetextfolding.cpp.
int Kate::TextFolding::visibleLines | ( | ) | const |
Query number of visible lines.
Very fast, if nothing is folded, else walks over all folded regions O(n) for n == number of folded ranges
start with all lines we have
skip if nothing folded
count all folded lines and subtract them from visible lines
be done, assert we did no trash
Definition at line 340 of file katetextfolding.cpp.
int Kate::TextFolding::visibleLineToLine | ( | int | visibleLine | ) | const |
Convert a visible line number to a line number in the text buffer.
Very fast, if nothing is folded, else walks over all folded regions O(n) for n == number of folded ranges
- Parameters
-
visibleLine visible line index
- Returns
- index in text buffer lines
valid input needed!
start with identity
skip if nothing folded or first line
last visible line seen, as line in buffer
else compute visible lines and move last seen
bail out if enough seen
check if still no enough visible!
compute visible line
Definition at line 422 of file katetextfolding.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:54 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.