Kate
#include <katetextbuffer.h>
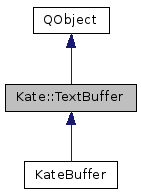
Public Types | |
enum | EndOfLineMode { eolUnknown = -1, eolUnix = 0, eolDos = 1, eolMac = 2 } |
Signals | |
void | cleared () |
void | editingFinished () |
void | editingStarted () |
void | lineUnwrapped (int line) |
void | lineWrapped (const KTextEditor::Cursor &position) |
void | loaded (const QString &filename, bool encodingErrors) |
void | saved (const QString &filename) |
void | textInserted (const KTextEditor::Cursor &position, const QString &text) |
void | textRemoved (const KTextEditor::Range &range, const QString &text) |
Detailed Description
Class representing a text buffer.
The interface is line based, internally the text will be stored in blocks of text lines.
Definition at line 48 of file katetextbuffer.h.
Member Enumeration Documentation
End of line mode.
Enumerator | |
---|---|
eolUnknown | |
eolUnix | |
eolDos | |
eolMac |
Definition at line 59 of file katetextbuffer.h.
Constructor & Destructor Documentation
Kate::TextBuffer::TextBuffer | ( | KateDocument * | parent, |
int | blockSize = 64 |
||
) |
Construct an empty text buffer.
Empty means one empty line in one block.
- Parameters
-
parent parent qobject blockSize block size in lines the buffer should try to hold, default 64 lines
Definition at line 44 of file katetextbuffer.cpp.
|
virtual |
Destruct the text buffer Virtual, we allow inheritance.
Definition at line 72 of file katetextbuffer.cpp.
Member Function Documentation
|
virtual |
Clears the buffer, reverts to initial empty state.
Empty means one empty line in one block. Virtual, can be overwritten.
Reimplemented in KateBuffer.
Definition at line 108 of file katetextbuffer.cpp.
|
signal |
void Kate::TextBuffer::debugPrint | ( | const QString & | title | ) | const |
Debug output, print whole buffer content with line numbers and line length.
- Parameters
-
title title for this output
Definition at line 503 of file katetextbuffer.cpp.
const QByteArray & Kate::TextBuffer::digest | ( | ) | const |
md5 digest of the document on disk, set either through file loading in openFile() or in KateDocument::saveFile()
- Returns
- md5 digest for this document
Definition at line 692 of file katetextbuffer.cpp.
|
inline |
Gets the document to which this buffer is bound.
- Returns
- a pointer to the document
Definition at line 408 of file katetextbuffer.h.
|
inline |
Query information from the last editing transaction: was the content of the buffer changed? This is checked by comparing the editingLastRevision() with the current revision().
- Returns
- content of buffer was changed in last transaction?
Definition at line 250 of file katetextbuffer.h.
|
inline |
Query information from the last editing transaction: was the number of lines of the buffer changed? This is checked by comparing the editingLastLines() with the current lines().
- Returns
- content of buffer was changed in last transaction?
Definition at line 257 of file katetextbuffer.h.
|
signal |
Editing transaction has finished.
|
inline |
Query the number of lines of this buffer before the ongoing editing transactions.
- Returns
- number of lines of buffer before current editing transaction altered it
Definition at line 243 of file katetextbuffer.h.
|
inline |
Query the revsion of this buffer before the ongoing editing transactions.
- Returns
- revision of buffer before current editing transaction altered it
Definition at line 237 of file katetextbuffer.h.
|
inline |
Get maximal line number changed by last editing transaction.
- Returns
- maximal line number changed by last editing transaction, or -1, if none changed
Definition at line 269 of file katetextbuffer.h.
|
inline |
Get minimal line number changed by last editing transaction.
- Returns
- maximal line number changed by last editing transaction, or -1, if none changed
Definition at line 263 of file katetextbuffer.h.
|
signal |
Editing transaction has started.
|
inline |
Query the number of editing transactions running atm.
- Returns
- number of running transactions
Definition at line 231 of file katetextbuffer.h.
|
inline |
Get encoding prober type for this buffer.
- Returns
- currently in use prober type of this buffer
Definition at line 97 of file katetextbuffer.h.
|
inline |
|
inline |
Get fallback codec for this buffer.
- Returns
- currently in use fallback codec of this buffer
Definition at line 109 of file katetextbuffer.h.
|
virtual |
Finish an editing transaction.
Only allowed to be called if editing transaction is started.
- Returns
- returns true, if this finished last running transaction Virtual, can be overwritten.
Definition at line 193 of file katetextbuffer.cpp.
|
inline |
Generate byte order mark on save?
- Returns
- should BOM be generated?
Definition at line 136 of file katetextbuffer.h.
|
inline |
TextHistory of this buffer.
- Returns
- text history for this buffer
Definition at line 304 of file katetextbuffer.h.
|
virtual |
Insert text at given cursor position.
Does nothing if text is empty, beside some consistency checks.
- Parameters
-
position position where to insert text text text to insert Virtual, can be overwritten.
Definition at line 306 of file katetextbuffer.cpp.
void Kate::TextBuffer::invalidateRanges | ( | ) |
Invalidate all ranges in this buffer.
Definition at line 100 of file katetextbuffer.cpp.
TextLine Kate::TextBuffer::line | ( | int | line | ) | const |
Retrieve a text line.
- Parameters
-
line wanted line number
- Returns
- text line
Definition at line 150 of file katetextbuffer.cpp.
|
inline |
Lines currently stored in this buffer.
This is never 0, even clear will let one empty line remain.
Definition at line 189 of file katetextbuffer.h.
|
signal |
A line got unwrapped.
- Parameters
-
line line where the unwrap occurred
|
signal |
A line got wrapped.
- Parameters
-
position position where the wrap occurred
|
virtual |
Load the given file.
This will first clear the buffer and then load the file. Even on error during loading the buffer will still be cleared. Before calling this, setTextCodec must have been used to set codec!
- Parameters
-
filename file to open encodingErrors were there problems occurred while decoding the file? tooLongLinesWrapped were too long lines found and wrapped? enforceTextCodec enforce to use only the set text codec
- Returns
- success, the file got loaded, perhaps with encoding errors Virtual, can be overwritten.
first: clear buffer in any case!
construct the file loader for the given file, with correct prober type
triple play, maximal three loading rounds 0) use the given encoding, be done, if no encoding errors happen 1) use BOM to decided if unicode or if that fails, use encoding prober, if no encoding errors happen, be done 2) use fallback encoding, be done, if no encoding errors happen 3) use again given encoding, be done in any case
kill all blocks beside first one
remove lines in first block
try to open file, with given encoding in round 0 + 3 use the given encoding from user in round 1 use 0, to trigger detection in round 2 use fallback
split lines, if too large
calculate line length
search for place to wrap
wrap place found?
wrap the line
be done after this round
construct new text line with content from file move data pointer
ensure blocks aren't too large
append line to last block
Definition at line 513 of file katetextbuffer.cpp.
Buffer loaded successfully a file.
- Parameters
-
filename file which was loaded encodingErrors were there problems occurred while decoding the file?
Check if the given range pointer is still valid.
- Returns
- range pointer still belongs to range for this buffer
Definition at line 429 of file katetextbuffer.h.
QList< TextRange * > Kate::TextBuffer::rangesForLine | ( | int | line, |
KTextEditor::View * | view, | ||
bool | rangesWithAttributeOnly | ||
) | const |
Return the ranges which affect the given line.
- Parameters
-
line line to look at view only return ranges associated with given view rangesWithAttributeOnly only return ranges which have a attribute set
- Returns
- list of ranges affecting this line
we want only ranges with attributes, but this one has none
we want ranges for no view, but this one's attribute is only valid for views
the range's attribute is not valid for this view
if line is in the range, ok
Definition at line 872 of file katetextbuffer.cpp.
|
virtual |
Remove text at given range.
Does nothing if range is empty, beside some consistency checks.
- Parameters
-
range range of text to remove, must be on one line only. Virtual, can be overwritten.
Definition at line 338 of file katetextbuffer.cpp.
|
inline |
Revision of this buffer.
Is set to 0 on construction, clear() (load will trigger clear()). Is incremented on each change to the buffer.
- Returns
- current revision
Definition at line 196 of file katetextbuffer.h.
Save the current buffer content to the given file.
Before calling this, setTextCodec and setFallbackTextCodec must have been used to set codec!
- Parameters
-
filename file to save
- Returns
- success Virtual, can be overwritten.
use KSaveFile for save write + rename
try to open or fail
construct correct filter device and try to open
try to open, if new file
construct stream + disable Unicode headers
Definition at line 714 of file katetextbuffer.cpp.
|
signal |
Buffer saved successfully a file.
- Parameters
-
filename file which was saved
void Kate::TextBuffer::setDigest | ( | const QByteArray & | md5sum | ) |
Set the md5sum of this buffer.
Make sure this checksum is up-to-date when reading digest().
- Parameters
-
md5sum md5 digest for the document on disk
Definition at line 697 of file katetextbuffer.cpp.
|
inline |
Set encoding prober type for this buffer to use for load.
- Parameters
-
proberType prober type to use for encoding
Definition at line 91 of file katetextbuffer.h.
|
inline |
Set end of line mode for this buffer, not allowed to be set to unknown.
Loading might overwrite this setting, if there is a eol found inside the file.
- Parameters
-
endOfLineMode new eol mode
Definition at line 143 of file katetextbuffer.h.
|
inline |
Set fallback codec for this buffer to use for load.
- Parameters
-
codec fallback QTextCodec to use for encoding
Definition at line 103 of file katetextbuffer.h.
|
inline |
Generate byte order mark on save.
Loading might overwrite this setting, if there is a BOM found inside the file.
- Parameters
-
generateByteOrderMark should BOM be generated?
Definition at line 130 of file katetextbuffer.h.
|
inline |
Set line length limit.
- Parameters
-
lineLengthLimit new line length limit
Definition at line 161 of file katetextbuffer.h.
|
inline |
Set whether to insert a newline character on save at the end of the file.
- Parameters
-
newlineAtEof should newline be added if non-existing
Definition at line 155 of file katetextbuffer.h.
void Kate::TextBuffer::setTextCodec | ( | QTextCodec * | codec | ) |
Set codec for this buffer to use for load/save.
Loading might overwrite this, if it encounters problems and finds a better codec. Might change BOM setting.
- Parameters
-
codec QTextCodec to use for encoding
Definition at line 702 of file katetextbuffer.cpp.
|
virtual |
Start an editing transaction, the wrapLine/unwrapLine/insertText and removeText functions are only to be allowed to be called inside a editing transaction.
Editing transactions can stack. The number startEdit and endEdit calls must match.
- Returns
- returns true, if no transaction was already running Virtual, can be overwritten.
Definition at line 171 of file katetextbuffer.cpp.
QString Kate::TextBuffer::text | ( | ) | const |
Retrieve text of complete buffer.
- Returns
- text for this buffer, lines separated by '
'
Definition at line 159 of file katetextbuffer.cpp.
|
inline |
Get codec for this buffer.
- Returns
- currently in use codec of this buffer
Definition at line 123 of file katetextbuffer.h.
|
signal |
Text got inserted.
- Parameters
-
position position where the insertion occurred text inserted text
|
signal |
Text got removed.
- Parameters
-
range range where the removal occurred text removed text
|
virtual |
Unwrap given line.
- Parameters
-
line line to unwrap Virtual, can be overwritten.
let the block handle the unwrapLine this can either lead to one line less in this block or the previous one the previous one could even end up with zero lines this call will trigger fixStartLines
Reimplemented in KateBuffer.
Definition at line 257 of file katetextbuffer.cpp.
|
virtual |
Wrap line at given cursor position.
- Parameters
-
position line/column as cursor where to wrap Virtual, can be overwritten.
let the block handle the wrapLine this can only lead to one more line in this block no other blocks will change this call will trigger fixStartLines
Reimplemented in KateBuffer.
Definition at line 218 of file katetextbuffer.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:54 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.