Kate
#include <katedocument.h>
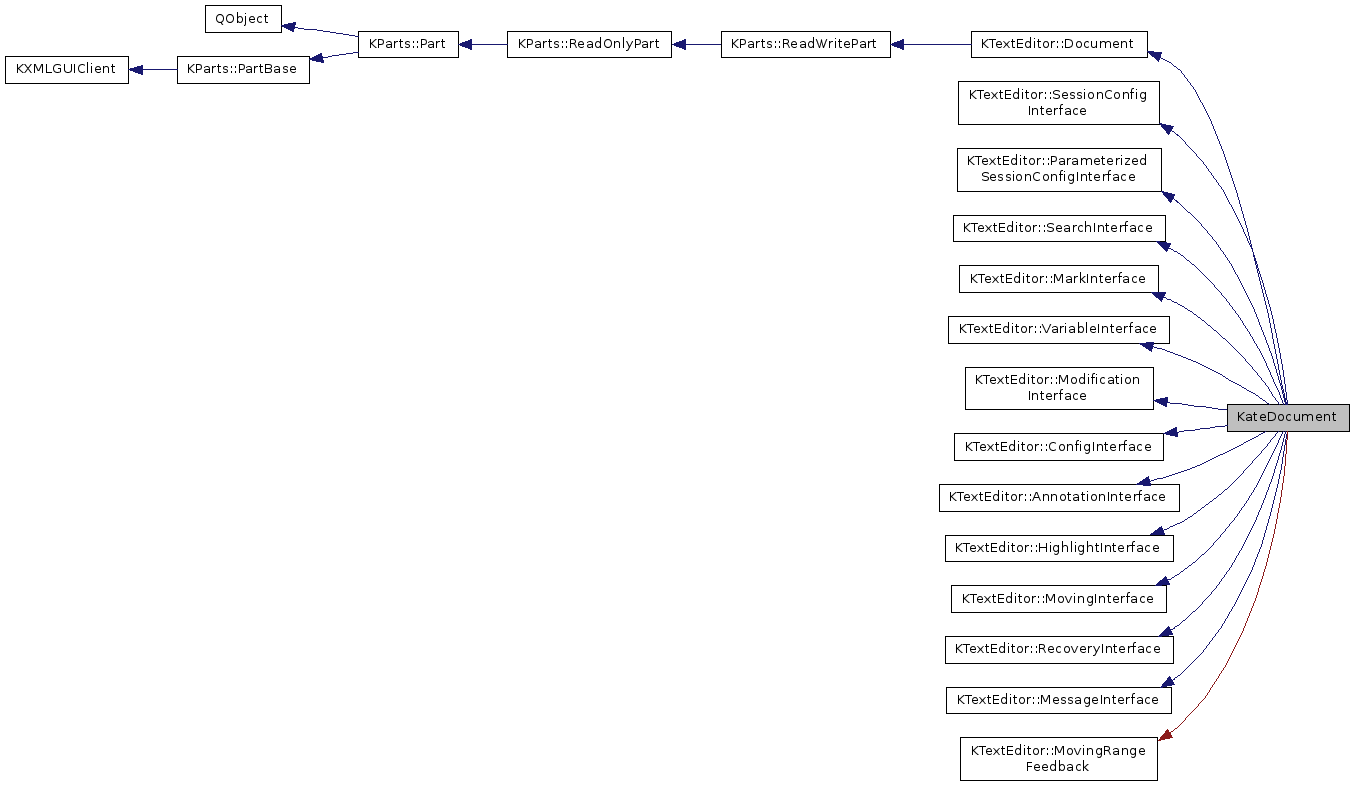
Public Types | |
enum | EncodedCharaterInsertionPolicy { EncodeAlways, EncodeWhenPresent, EncodeNever } |
typedef QList< QPair< int, int > > | OffsetList |
enum | TextTransform { Uppercase, Lowercase, Capitalize } |
![]() | |
enum | ReverseStateChange |
![]() | |
enum | SessionConfigParameter |
![]() | |
enum | MarkChangeAction |
enum | MarkTypes |
![]() | |
enum | ModifiedOnDiskReason |
![]() | |
enum | DefaultStyle |
Public Slots | |
virtual void | addMark (int line, uint markType) |
virtual bool | clear () |
void | clearDictionaryRanges () |
virtual void | clearMark (int line) |
virtual void | clearMarks () |
virtual bool | documentReload () |
virtual bool | documentSave () |
virtual bool | documentSaveAs () |
bool | handleMarkClick (int line) |
bool | handleMarkContextMenu (int line, QPoint position) |
virtual bool | insertLine (int line, const QString &s) |
virtual bool | insertLines (int line, const QStringList &s) |
virtual bool | insertText (const KTextEditor::Cursor &position, const QString &s, bool block=false) |
virtual bool | insertText (const KTextEditor::Cursor &position, const QStringList &text, bool block=false) |
void | messageDestroyed (KTextEditor::Message *message) |
void | onTheFlySpellCheckingEnabled (bool enable) |
Q_SCRIPTABLE bool | print () |
bool | printDialog () |
void | redo () |
void | refreshOnTheFlyCheck (const KTextEditor::Range &range=KTextEditor::Range::invalid()) |
virtual bool | removeLine (int line) |
virtual void | removeMark (int line, uint markType) |
virtual bool | removeText (const KTextEditor::Range &range, bool block=false) |
virtual bool | replaceText (const KTextEditor::Range &range, const QString &s, bool block=false) |
virtual bool | replaceText (const KTextEditor::Range &r, const QStringList &l, bool b) |
void | requestMarkTooltip (int line, QPoint position) |
void | revertToDefaultDictionary (const KTextEditor::Range &range) |
virtual bool | save () |
void | setDefaultDictionary (const QString &dict) |
void | setDictionary (const QString &dict, const KTextEditor::Range &range) |
virtual void | setEditableMarks (uint markMask) |
virtual void | setMark (int line, uint markType) |
virtual void | setMarkDescription (MarkInterface::MarkTypes, const QString &) |
virtual void | setMarkPixmap (MarkInterface::MarkTypes, const QPixmap &) |
void | setPageUpDownMovesCursor (bool on) |
virtual bool | setText (const QString &) |
virtual bool | setText (const QStringList &text) |
void | setWordWrap (bool on) |
void | setWordWrapAt (uint col) |
virtual void | slotModifiedOnDisk (KTextEditor::View *v=0) |
void | slotQueryClose_save (bool *handled, bool *abortClosing) |
void | tagLines (int start, int end) |
void | undo () |
![]() | |
virtual bool | save () |
void | setModified () |
bool | waitSaveComplete () |
![]() |
Static Public Member Functions | |
static bool | checkOverwrite (KUrl u, QWidget *parent) |
static bool | simpleMode () |
![]() | |
static QString | findMostRecentXMLFile (const QStringList &files, QString &doc) |
![]() | |
static int | reservedMarkersCount () |
Protected Member Functions | |
void | deleteDictionaryRange (KTextEditor::MovingRange *movingRange) |
void | rangeEmpty (KTextEditor::MovingRange *movingRange) |
void | rangeInvalid (KTextEditor::MovingRange *movingRange) |
![]() | |
void | setOpeningError (bool errors) |
void | setOpeningErrorMessage (const QString &message) |
![]() | |
virtual bool | saveToUrl () |
![]() | |
ReadOnlyPart (ReadOnlyPartPrivate &dd, QObject *parent) | |
void | abortLoad () |
virtual void | guiActivateEvent (GUIActivateEvent *event) |
bool | isLocalFileTemporary () const |
QString | localFilePath () const |
void | setLocalFilePath (const QString &localFilePath) |
void | setLocalFileTemporary (bool temp) |
void | setUrl (const KUrl &url) |
![]() | |
Part (PartPrivate &dd, QObject *parent) | |
virtual void | customEvent (QEvent *event) |
QWidget * | hostContainer (const QString &containerName) |
void | loadPlugins () |
virtual void | partActivateEvent (PartActivateEvent *event) |
virtual void | partSelectEvent (PartSelectEvent *event) |
virtual void | setWidget (QWidget *widget) |
![]() | |
void | loadStandardsXmlFile () |
virtual void | setComponentData (const KComponentData &componentData) |
virtual void | setDOMDocument (const QDomDocument &document, bool merge=false) |
virtual void | setLocalXMLFile (const QString &file) |
virtual void | setXML (const QString &document, bool merge=false) |
virtual void | setXMLFile (const QString &file, bool merge=false, bool setXMLDoc=true) |
virtual void | stateChanged (const QString &newstate, ReverseStateChange reverse=StateNoReverse) |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
QString | m_defaultDictionary |
QList< QPair < KTextEditor::MovingRange *, QString > > | m_dictionaryRanges |
KateOnTheFlyChecker * | m_onTheFlyChecker |
KateUndoManager *const | m_undoManager |
Additional Inherited Members | |
![]() | |
void | slotWidgetDestroyed () |
![]() | |
KUrl | url |
Detailed Description
Definition at line 74 of file katedocument.h.
Member Typedef Documentation
typedef QList<QPair<int, int> > KateDocument::OffsetList |
Definition at line 1131 of file katedocument.h.
Member Enumeration Documentation
Enumerator | |
---|---|
EncodeAlways | |
EncodeWhenPresent | |
EncodeNever |
Definition at line 1144 of file katedocument.h.
Enumerator | |
---|---|
Uppercase | |
Lowercase | |
Capitalize |
Definition at line 734 of file katedocument.h.
Constructor & Destructor Documentation
|
explicit |
avoid spamming plasma and other window managers with progress dialogs we show such stuff inline in the views!
load handling this is needed to ensure we signal the user if a file ist still loading and to disallow him to edit in that time
Definition at line 111 of file katedocument.cpp.
KateDocument::~KateDocument | ( | ) |
we are about to delete cursors/ranges/...
Definition at line 229 of file katedocument.cpp.
Member Function Documentation
|
signal |
This signal is emitted before the cursors/ranges/revisions of a document are destroyed as the document is deleted.
- Parameters
-
document the document which the interface belongs too which is in the process of being deleted
|
signal |
This signal is emitted before the ranges of a document are invalidated and the revisions are deleted as the document is cleared (for example on load/reload).
While this signal is emitted, still the old document content is around before the clear.
- Parameters
-
document the document which the interface belongs too which will invalidate its data
|
signal |
KateView * KateDocument::activeKateView | ( | ) | const |
Definition at line 4677 of file katedocument.cpp.
|
inlinevirtual |
Implements KTextEditor::Document.
Definition at line 156 of file katedocument.h.
|
virtualslot |
Definition at line 1677 of file katedocument.cpp.
void KateDocument::addView | ( | KTextEditor::View * | view | ) |
Definition at line 2523 of file katedocument.cpp.
void KateDocument::align | ( | KateView * | view, |
const KTextEditor::Range & | range | ||
) |
Definition at line 2908 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::AnnotationInterface.
Definition at line 4800 of file katedocument.cpp.
|
signal |
void KateDocument::backspace | ( | KateView * | view, |
const KTextEditor::Cursor & | c | ||
) |
Definition at line 2725 of file katedocument.cpp.
void KateDocument::bomSetByUser | ( | ) |
Set that the BOM marker is forced via the tool menu.
Definition at line 1536 of file katedocument.cpp.
|
inline |
Definition at line 139 of file katedocument.h.
|
inline |
Get access to buffer of this document.
Is needed to create cursors and ranges for example.
- Returns
- document buffer
Definition at line 946 of file katedocument.h.
void KateDocument::bufferHlChanged | ( | ) |
Definition at line 1519 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::Document.
Definition at line 388 of file katedocument.cpp.
|
signal |
Definition at line 4585 of file katedocument.cpp.
|
virtualslot |
Definition at line 509 of file katedocument.cpp.
|
slot |
Definition at line 5042 of file katedocument.cpp.
|
virtualslot |
Definition at line 1661 of file katedocument.cpp.
|
virtualslot |
Definition at line 1787 of file katedocument.cpp.
void KateDocument::clearMisspellingForWord | ( | const QString & | word | ) |
Definition at line 5187 of file katedocument.cpp.
|
virtual |
reset reloading
reset reloading
delete all KTE::Messages
we are about to invalidate all cursors/ranges/.. => m_buffer->clear will do so
Reimplemented from KParts::ReadWritePart.
Definition at line 2336 of file katedocument.cpp.
void KateDocument::comment | ( | KateView * | view, |
uint | line, | ||
uint | column, | ||
int | change | ||
) |
Definition at line 3301 of file katedocument.cpp.
int KateDocument::computePositionWrtOffsets | ( | const OffsetList & | offsetList, |
int | pos | ||
) |
Definition at line 5254 of file katedocument.cpp.
|
inline |
Configuration.
Definition at line 1009 of file katedocument.h.
|
inline |
Definition at line 1010 of file katedocument.h.
|
signal |
|
virtual |
Implements KTextEditor::ConfigInterface.
Definition at line 4604 of file katedocument.cpp.
Implements KTextEditor::ConfigInterface.
Definition at line 4609 of file katedocument.cpp.
bool KateDocument::containsCharacterEncoding | ( | const KTextEditor::Range & | range | ) |
Definition at line 5228 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::Document.
Definition at line 292 of file katedocument.cpp.
QString KateDocument::decodeCharacters | ( | const KTextEditor::Range & | range, |
KateDocument::OffsetList & | decToEncOffsetList, | ||
KateDocument::OffsetList & | encToDecOffsetList | ||
) |
The first OffsetList is from decoded to encoded, and the second OffsetList from encoded to decoded.
Definition at line 5266 of file katedocument.cpp.
QString KateDocument::defaultDictionary | ( | ) | const |
Definition at line 5032 of file katedocument.cpp.
|
signal |
|
virtual |
TODO: move attributes to document, they are not view-dependant
Implements KTextEditor::HighlightInterface.
Definition at line 5354 of file katedocument.cpp.
int KateDocument::defStyleNum | ( | int | line, |
int | column | ||
) |
- Returns
-1
ifline
orcolumn
invalid, otherwise one of standard style attribute number
either get char attribute or attribute of context still active at end of line
Definition at line 5451 of file katedocument.cpp.
void KateDocument::del | ( | KateView * | view, |
const KTextEditor::Cursor & | c | ||
) |
Definition at line 2805 of file katedocument.cpp.
|
protected |
Definition at line 5211 of file katedocument.cpp.
QString KateDocument::dictionaryForMisspelledRange | ( | const KTextEditor::Range & | range | ) | const |
Definition at line 5177 of file katedocument.cpp.
QList< QPair< KTextEditor::MovingRange *, QString > > KateDocument::dictionaryRanges | ( | ) | const |
Definition at line 5037 of file katedocument.cpp.
|
signal |
const QByteArray & KateDocument::digest | ( | ) | const |
md5 digest of this document
- Returns
- md5 digest for this document
Definition at line 4410 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::RecoveryInterface.
Definition at line 2462 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::Document.
Definition at line 4682 of file katedocument.cpp.
|
inlinevirtual |
Implements KTextEditor::Document.
Definition at line 828 of file katedocument.h.
|
virtualslot |
Reloads the current document from disk if possible.
Definition at line 3805 of file katedocument.cpp.
|
virtualslot |
Definition at line 3899 of file katedocument.cpp.
|
virtualslot |
Definition at line 3907 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::MarkInterface.
Definition at line 1841 of file katedocument.cpp.
|
inline |
Alias for editStart()
Definition at line 213 of file katedocument.h.
void KateDocument::editEnd | ( | ) |
Insert a string at the given line.
- Parameters
-
line line number s string to insert
- Returns
- true on success
Definition at line 1223 of file katedocument.cpp.
Add a string in the given line/column.
- Parameters
-
line line number col column s string to be inserted
- Returns
- true on success
Definition at line 971 of file katedocument.cpp.
|
signal |
Emitted each time text from nextLine
was upwrapped onto line
.
|
signal |
Emmitted when text from line
was wrapped at position pos onto line nextLine
.
Mark line
as autowrapped
.
This is necessary if static word warp is enabled, because we have to know whether to insert a new line or add the wrapped words to the followin line.
- Parameters
-
line line number autowrapped autowrapped?
- Returns
- true on success
Definition at line 1056 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::Document.
Definition at line 314 of file katedocument.cpp.
bool KateDocument::editRemoveLine | ( | int | line | ) |
Remove a line.
- Parameters
-
line line number
- Returns
- true on success
Definition at line 1289 of file katedocument.cpp.
bool KateDocument::editRemoveLines | ( | int | from, |
int | to | ||
) |
Definition at line 1294 of file katedocument.cpp.
bool KateDocument::editRemoveText | ( | int | line, |
int | col, | ||
int | len | ||
) |
Remove a string in the given line/column.
- Parameters
-
line line number col column len length of text to be removed
- Returns
- true on success
Definition at line 1012 of file katedocument.cpp.
void KateDocument::editStart | ( | ) |
Enclose editor actions with editStart()
and editEnd()
to group them.
Definition at line 776 of file katedocument.cpp.
Unwrap line
.
If removeLine
is true, we force to join the lines. If removeLine
is true, length
is ignored (eg not needed).
- Parameters
-
line line number removeLine if true, force to remove the next line
- Returns
- true on success
Definition at line 1153 of file katedocument.cpp.
Wrap line
.
If newLine
is true, ignore the textline's flag KateTextLine::flagAutoWrapped and force a new line. Whether a new line was needed/added you can grab with newLineAdded
.
- Parameters
-
line line number col column newLine if true, force a new line newLineAdded return value is true, if new line was added (may be 0)
- Returns
- true on success
Definition at line 1083 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::HighlightInterface.
Definition at line 5401 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::Document.
Definition at line 3958 of file katedocument.cpp.
|
inlinevirtual |
Implements KTextEditor::Document.
Definition at line 225 of file katedocument.h.
|
inline |
Definition at line 939 of file katedocument.h.
bool KateDocument::findMatchingBracket | ( | KTextEditor::Range & | range, |
int | maxLines = -1 |
||
) |
Definition at line 3588 of file katedocument.cpp.
int KateDocument::fromVirtualColumn | ( | int | line, |
int | column | ||
) | const |
Definition at line 2579 of file katedocument.cpp.
int KateDocument::fromVirtualColumn | ( | const KTextEditor::Cursor & | cursor | ) | const |
Definition at line 2589 of file katedocument.cpp.
QString KateDocument::getWord | ( | const KTextEditor::Cursor & | cursor | ) |
Definition at line 3537 of file katedocument.cpp.
|
slot |
Returns true if the click on the mark should not be further processed.
Definition at line 1762 of file katedocument.cpp.
Returns true if the context-menu event should not further be processed.
Definition at line 1774 of file katedocument.cpp.
KateHighlighting * KateDocument::highlight | ( | ) | const |
Definition at line 4702 of file katedocument.cpp.
|
virtual |
Return the name of the currently used mode.
- Returns
- name of the used mode
Implements KTextEditor::Document.
Definition at line 1494 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::HighlightInterface.
Definition at line 5406 of file katedocument.cpp.
|
virtual |
Return a list of the names of all possible modes.
- Returns
- list of mode names
Implements KTextEditor::Document.
Definition at line 1499 of file katedocument.cpp.
|
virtual |
Returns the name of the section for a highlight given its index in the highlight list (as returned by highlightModes()).
You can use this function to build a tree of the highlight names, organized in sections.
- Parameters
-
name the name of the highlight for which to find the section name.
Implements KTextEditor::Document.
Definition at line 1509 of file katedocument.cpp.
void KateDocument::ignoreModifiedOnDiskOnce | ( | ) |
Definition at line 4697 of file katedocument.cpp.
void KateDocument::indent | ( | KTextEditor::Range | range, |
int | change | ||
) |
Definition at line 2898 of file katedocument.cpp.
void KateDocument::inputMethodEnd | ( | ) |
Definition at line 850 of file katedocument.cpp.
void KateDocument::inputMethodStart | ( | ) |
Definition at line 845 of file katedocument.cpp.
Definition at line 701 of file katedocument.cpp.
|
virtualslot |
Definition at line 712 of file katedocument.cpp.
void KateDocument::insertTab | ( | KateView * | view, |
const KTextEditor::Cursor & | |||
) |
Definition at line 2913 of file katedocument.cpp.
|
virtualslot |
Definition at line 530 of file katedocument.cpp.
|
virtualslot |
Definition at line 624 of file katedocument.cpp.
bool KateDocument::isComment | ( | int | line, |
int | column | ||
) |
Definition at line 5491 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::RecoveryInterface.
Definition at line 2451 of file katedocument.cpp.
bool KateDocument::isEditRunning | ( | ) | const |
Definition at line 4718 of file katedocument.cpp.
|
inline |
- Returns
- whether the document is modified on disk since last saved
Definition at line 837 of file katedocument.h.
bool KateDocument::isOnTheFlySpellCheckingEnabled | ( | ) | const |
Definition at line 5172 of file katedocument.cpp.
void KateDocument::joinLines | ( | uint | first, |
uint | last | ||
) |
Unwrap a range of lines.
Definition at line 3496 of file katedocument.cpp.
Kate::TextLine KateDocument::kateTextLine | ( | uint | i | ) |
Definition at line 4707 of file katedocument.cpp.
|
inline |
gets the last line number (lines() - 1)
Definition at line 691 of file katedocument.h.
|
virtual |
Last revision the buffer got successful saved.
- Returns
- last revision buffer got saved, -1 if none
Implements KTextEditor::MovingInterface.
Definition at line 4752 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::Document.
Definition at line 447 of file katedocument.cpp.
|
virtual |
TODO: move attributes to document, they are not view-dependant
Implements KTextEditor::HighlightInterface.
Definition at line 5372 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::Document.
Definition at line 758 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::Document.
Definition at line 753 of file katedocument.cpp.
|
virtual |
Lock a revision, this will keep it around until released again.
But all revisions will always be cleared on buffer clear() (and therefor load())
- Parameters
-
revision revision to lock
Implements KTextEditor::MovingInterface.
Definition at line 4757 of file katedocument.cpp.
void KateDocument::makeAttribs | ( | bool | needInvalidate = true | ) |
Definition at line 2502 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::MarkInterface.
Definition at line 1646 of file katedocument.cpp.
|
signal |
|
signal |
|
virtual |
Definition at line 1821 of file katedocument.cpp.
|
signal |
|
virtual |
Definition at line 1831 of file katedocument.cpp.
|
virtual |
Definition at line 1816 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::MarkInterface.
Definition at line 1747 of file katedocument.cpp.
|
signal |
|
signal |
|
slot |
Definition at line 5543 of file katedocument.cpp.
|
virtual |
- Returns
- the name of the mimetype for the document.
This method is using KMimeType::findByUrl, and if the pointer is then still the default MimeType for a nonlocal or unsaved file, uses mimeTypeForContent().
Implements KTextEditor::Document.
Definition at line 1860 of file katedocument.cpp.
KMimeType::Ptr KateDocument::mimeTypeForContent | ( | ) |
- Returns
- a pointer to the KMimeType for this document, found by analyzing the actual content.
Note that this method is not part of the DocumentInfoInterface.
Definition at line 1874 of file katedocument.cpp.
|
virtual |
Return the name of the currently used mode.
- Returns
- name of the used mode
Implements KTextEditor::Document.
Definition at line 1468 of file katedocument.cpp.
|
virtual |
Return a list of the names of all possible modes.
- Returns
- list of mode names
Implements KTextEditor::Document.
Definition at line 1473 of file katedocument.cpp.
|
virtual |
Returns the name of the section for a mode given its index in the highlight list (as returned by modes()).
You can use this function to build a tree of the mode names, organized in sections.
- Parameters
-
name the name of the highlight for which to find the section name.
Implements KTextEditor::Document.
Definition at line 1514 of file katedocument.cpp.
|
signal |
Indicate this file is modified on disk.
- Parameters
-
doc the KTextEditor::Document object that represents the file on disk isModified indicates the file was modified rather than created or deleted reason the reason we are emitting the signal.
void KateDocument::newBracketMark | ( | const KTextEditor::Cursor & | start, |
KTextEditor::Range & | bm, | ||
int | maxLines = -1 |
||
) |
Definition at line 3572 of file katedocument.cpp.
void KateDocument::newLine | ( | KateView * | view | ) |
Definition at line 2659 of file katedocument.cpp.
|
virtual |
Create a new moving cursor for this document.
- Parameters
-
position position of the moving cursor to create insertBehavior insertion behavior
- Returns
- new moving cursor for the document
Implements KTextEditor::MovingInterface.
Definition at line 4737 of file katedocument.cpp.
|
virtual |
Create a new moving range for this document.
- Parameters
-
range range of the moving range to create insertBehaviors insertion behaviors emptyBehavior behavior on becoming empty
- Returns
- new moving range for the document
Definition at line 4742 of file katedocument.cpp.
|
slot |
Definition at line 5152 of file katedocument.cpp.
|
virtual |
open the file obtained by the kparts framework the framework abstracts the loading of remote files
- Returns
- success
we are about to invalidate all cursors/ranges/.. => m_buffer->openFile will do so
Reimplemented from KParts::ReadOnlyPart.
Definition at line 1931 of file katedocument.cpp.
Reimplemented from KParts::ReadOnlyPart.
Definition at line 2330 of file katedocument.cpp.
Definition at line 2559 of file katedocument.cpp.
bool KateDocument::pageUpDownMovesCursor | ( | ) | const |
Definition at line 3947 of file katedocument.cpp.
Definition at line 2828 of file katedocument.cpp.
Kate::TextLine KateDocument::plainKateTextLine | ( | uint | i | ) |
Definition at line 4713 of file katedocument.cpp.
void KateDocument::popEditState | ( | ) |
Definition at line 836 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::MessageInterface.
Definition at line 5498 of file katedocument.cpp.
|
slot |
Definition at line 1853 of file katedocument.cpp.
|
slot |
Definition at line 1848 of file katedocument.cpp.
void KateDocument::pushEditState | ( | ) |
Definition at line 831 of file katedocument.cpp.
|
virtual |
Reimplemented from KParts::ReadWritePart.
Definition at line 4807 of file katedocument.cpp.
|
protectedvirtual |
Reimplemented from KTextEditor::MovingRangeFeedback.
Definition at line 5206 of file katedocument.cpp.
|
protectedvirtual |
Reimplemented from KTextEditor::MovingRangeFeedback.
Definition at line 5201 of file katedocument.cpp.
KTextEditor::Range KateDocument::rangeOnLine | ( | KTextEditor::Range | range, |
int | line | ||
) | const |
Definition at line 319 of file katedocument.cpp.
|
inline |
Definition at line 138 of file katedocument.h.
|
virtual |
Implements KTextEditor::ParameterizedSessionConfigInterface.
Definition at line 1548 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::SessionConfigInterface.
Definition at line 1543 of file katedocument.cpp.
|
signal |
|
virtual |
Implements KTextEditor::RecoveryInterface.
Definition at line 2456 of file katedocument.cpp.
|
slot |
Definition at line 1380 of file katedocument.cpp.
uint KateDocument::redoCount | ( | ) | const |
Definition at line 1370 of file katedocument.cpp.
|
slot |
Definition at line 5194 of file katedocument.cpp.
|
inline |
set indentation mode by user this will remember that a user did set it and will avoid reset on save
Definition at line 952 of file katedocument.h.
|
virtualslot |
Definition at line 727 of file katedocument.cpp.
|
virtualslot |
Definition at line 1714 of file katedocument.cpp.
|
virtualslot |
Definition at line 633 of file katedocument.cpp.
void KateDocument::removeView | ( | KTextEditor::View * | view | ) |
removes the view from the list of views.
The view is not deleted. That's your job. Or, easier, just delete the view in the first place. It will remove itself. TODO: this could be converted to a private slot connected to the view's destroyed() signal. It is not currently called anywhere except from the KateView destructor.
Definition at line 2540 of file katedocument.cpp.
void KateDocument::repaintViews | ( | bool | paintOnlyDirty = true | ) |
Definition at line 3559 of file katedocument.cpp.
void KateDocument::replaceCharactersByEncoding | ( | const KTextEditor::Range & | range | ) |
Definition at line 5322 of file katedocument.cpp.
|
virtualslot |
Definition at line 4687 of file katedocument.cpp.
|
inlinevirtualslot |
Definition at line 185 of file katedocument.h.
|
slot |
Definition at line 1752 of file katedocument.cpp.
|
slot |
Definition at line 5132 of file katedocument.cpp.
|
virtual |
Current revision.
- Returns
- current revision
Implements KTextEditor::MovingInterface.
Definition at line 4747 of file katedocument.cpp.
|
virtualslot |
no double save/load we need to allow DocumentPreSavingAs here as state, as save is called in saveAs!
if we are idle, we are now saving
call back implementation for real work
Definition at line 4981 of file katedocument.cpp.
abort on bad URL that is done in saveAs below, too but we must check it here already to avoid messing up as no signals will be send, then
no double save/load
we enter the pre save as phase
call base implementation for real work
Reimplemented from KParts::ReadWritePart.
Definition at line 5004 of file katedocument.cpp.
|
virtual |
save the file obtained by the kparts framework the framework abstracts the uploading of remote files
- Returns
- success
Implements KParts::ReadWritePart.
Definition at line 2076 of file katedocument.cpp.
|
virtual |
Definition at line 1387 of file katedocument.cpp.
void KateDocument::setActiveView | ( | KTextEditor::View * | view | ) |
Definition at line 2551 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::AnnotationInterface.
Definition at line 4793 of file katedocument.cpp.
Implements KTextEditor::ConfigInterface.
Definition at line 4633 of file katedocument.cpp.
|
slot |
Definition at line 5137 of file katedocument.cpp.
|
slot |
Definition at line 5057 of file katedocument.cpp.
void KateDocument::setDontChangeHlOnSave | ( | ) |
allow to mark, that we changed hl on user wish and should not reset it atm used for the user visible menu to select highlightings
Definition at line 1531 of file katedocument.cpp.
|
virtualslot |
Definition at line 1836 of file katedocument.cpp.
Implements KTextEditor::Document.
Definition at line 3953 of file katedocument.cpp.
Set the current mode of the document by giving its name.
- Parameters
-
name name of the mode to use for this document
- Returns
- true on success, otherwise false
Implements KTextEditor::Document.
Definition at line 1484 of file katedocument.cpp.
|
virtualslot |
Definition at line 1655 of file katedocument.cpp.
|
virtualslot |
Definition at line 1811 of file katedocument.cpp.
|
virtualslot |
Definition at line 1806 of file katedocument.cpp.
Set the current mode of the document by giving its name.
- Parameters
-
name name of the mode to use for this document
- Returns
- true on success, otherwise false
Implements KTextEditor::Document.
Definition at line 1462 of file katedocument.cpp.
|
virtual |
Reimplemented from KParts::ReadWritePart.
Definition at line 2483 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::ModificationInterface.
Definition at line 3786 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::ModificationInterface.
Definition at line 3800 of file katedocument.cpp.
|
slot |
Definition at line 3942 of file katedocument.cpp.
|
virtual |
Reimplemented from KParts::ReadWritePart.
Definition at line 2468 of file katedocument.cpp.
Definition at line 457 of file katedocument.cpp.
|
virtualslot |
Definition at line 483 of file katedocument.cpp.
void KateDocument::setUndoMergeAllEdits | ( | bool | merge | ) |
Definition at line 4723 of file katedocument.cpp.
Definition at line 4344 of file katedocument.cpp.
|
slot |
Definition at line 3922 of file katedocument.cpp.
|
slot |
Definition at line 3932 of file katedocument.cpp.
|
static |
Definition at line 4787 of file katedocument.cpp.
|
inline |
Definition at line 140 of file katedocument.h.
|
virtualslot |
Ask the user what to do, if the file has been modified on disk.
Reimplemented from KTextEditor::Document.
Definition at line 3712 of file katedocument.cpp.
Definition at line 4559 of file katedocument.cpp.
|
inlinevirtual |
Implements KTextEditor::Document.
Definition at line 224 of file katedocument.h.
|
virtual |
Implements KTextEditor::SearchInterface.
Definition at line 1432 of file katedocument.cpp.
Kate::SwapFile * KateDocument::swapFile | ( | ) |
Definition at line 5442 of file katedocument.cpp.
|
slot |
Definition at line 3553 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::Document.
Definition at line 337 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::Document.
Definition at line 332 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::Document.
Definition at line 398 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::Document.
Definition at line 738 of file katedocument.cpp.
int KateDocument::toVirtualColumn | ( | int | line, |
int | column | ||
) | const |
Definition at line 2564 of file katedocument.cpp.
int KateDocument::toVirtualColumn | ( | const KTextEditor::Cursor & | cursor | ) | const |
Definition at line 2574 of file katedocument.cpp.
void KateDocument::transform | ( | KateView * | view, |
const KTextEditor::Cursor & | c, | ||
KateDocument::TextTransform | t | ||
) |
Handling uppercase, lowercase and capitalize for the view.
If there is a selection, that is transformed, otherwise for uppercase or lowercase the character right of the cursor is transformed, for capitalize the word under the cursor is transformed.
Definition at line 3383 of file katedocument.cpp.
|
virtual |
Transform a cursor from one revision to an other.
- Parameters
-
cursor cursor to transform insertBehavior behavior of this cursor on insert of text at its position fromRevision from this revision we want to transform toRevision to this revision we want to transform, default of -1 is current revision
Implements KTextEditor::MovingInterface.
Definition at line 4772 of file katedocument.cpp.
|
virtual |
Transform a cursor from one revision to an other.
- Parameters
-
line line number of the cursor to transform column column number of the cursor to transform insertBehavior behavior of this cursor on insert of text at its position fromRevision from this revision we want to transform toRevision to this revision we want to transform, default of -1 is current revision
Implements KTextEditor::MovingInterface.
Definition at line 4767 of file katedocument.cpp.
|
virtual |
Transform a range from one revision to an other.
- Parameters
-
range range to transform insertBehaviors behavior of this range on insert of text at its position emptyBehavior behavior on becoming empty fromRevision from this revision we want to transform toRevision to this revision we want to transform, default of -1 is current revision
Implements KTextEditor::MovingInterface.
Definition at line 4780 of file katedocument.cpp.
void KateDocument::transpose | ( | const KTextEditor::Cursor & | cursor | ) |
Definition at line 2694 of file katedocument.cpp.
Type chars in a view.
Will filter out non-printable chars from the realChars array before inserting.
filter out non-printable
Definition at line 2594 of file katedocument.cpp.
|
slot |
Definition at line 1375 of file katedocument.cpp.
|
signal |
uint KateDocument::undoCount | ( | ) | const |
Definition at line 1365 of file katedocument.cpp.
|
inline |
Definition at line 338 of file katedocument.h.
|
virtual |
Release a revision.
- Parameters
-
revision revision to release
Implements KTextEditor::MovingInterface.
Definition at line 4762 of file katedocument.cpp.
void KateDocument::updateConfig | ( | ) |
Definition at line 3963 of file katedocument.cpp.
set the indentation mode, if any in the mode... and user did not set it before!
Definition at line 4504 of file katedocument.cpp.
|
inline |
User did set encoding for next reload => enforce it!
Definition at line 960 of file katedocument.h.
Implements KTextEditor::VariableInterface.
Definition at line 4339 of file katedocument.cpp.
|
signal |
|
virtual |
Implements KTextEditor::Document.
Definition at line 309 of file katedocument.cpp.
|
virtual |
- Returns
- The widget defined by this part, set by setWidget().
Reimplemented from KParts::Part.
Definition at line 273 of file katedocument.cpp.
bool KateDocument::wordWrap | ( | ) | const |
Definition at line 3927 of file katedocument.cpp.
unsigned int KateDocument::wordWrapAt | ( | ) | const |
Definition at line 3937 of file katedocument.cpp.
bool KateDocument::wrapText | ( | int | startLine, |
int | endLine | ||
) |
Remove a line.
- Parameters
-
startLine line to begin wrapping endLine line to stop wrapping
- Returns
- true on success
Definition at line 855 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::ParameterizedSessionConfigInterface.
Definition at line 1602 of file katedocument.cpp.
|
virtual |
Implements KTextEditor::SessionConfigInterface.
Definition at line 1597 of file katedocument.cpp.
Member Data Documentation
|
protected |
Definition at line 1148 of file katedocument.h.
|
protected |
Definition at line 1149 of file katedocument.h.
|
protected |
Definition at line 1147 of file katedocument.h.
|
protected |
Definition at line 344 of file katedocument.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:54 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.