Kate
#include <katetextrange.h>
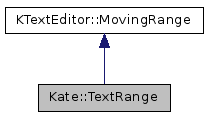
Additional Inherited Members | |
![]() | |
enum | EmptyBehavior |
enum | InsertBehavior |
![]() | |
MovingRange () | |
Detailed Description
Class representing a 'clever' text range.
It will automagically move if the text inside the buffer it belongs to is modified. By intention no subclass of KTextEditor::Range, must be converted manually. A TextRange is allowed to be empty. If you call setInvalidateIfEmpty(true), a TextRange will become automatically invalid as soon as start() == end() position holds.
Definition at line 46 of file katetextrange.h.
Constructor & Destructor Documentation
Kate::TextRange::TextRange | ( | TextBuffer & | buffer, |
const KTextEditor::Range & | range, | ||
InsertBehaviors | insertBehavior, | ||
EmptyBehavior | emptyBehavior = AllowEmpty |
||
) |
Construct a text range.
A TextRange is not allowed to be empty, as soon as start == end position, it will become automatically invalid!
- Parameters
-
buffer parent text buffer range The initial text range assumed by the new range. insertBehavior Define whether the range should expand when text is inserted adjacent to the range. emptyBehavior Define whether the range should invalidate itself on becoming empty.
Definition at line 29 of file katetextrange.cpp.
Kate::TextRange::~TextRange | ( | ) |
Destruct the text block.
reset feedback, don't want feedback during destruction
trigger update, if we have attribute notify right view here we can ignore feedback, even with feedback, we want none if the range is deleted!
Definition at line 46 of file katetextrange.cpp.
Member Function Documentation
|
inlinevirtual |
Gets the active Attribute for this range.
- Returns
- a pointer to the active attribute
Implements KTextEditor::MovingRange.
Definition at line 173 of file katetextrange.h.
|
inlinevirtual |
Is this range's attribute only visible in views, not for example prints? Default is false.
- Returns
- range visible only for views
Implements KTextEditor::MovingRange.
Definition at line 211 of file katetextrange.h.
|
virtual |
Gets the document to which this range is bound.
- Returns
- a pointer to the document
Implements KTextEditor::MovingRange.
Definition at line 326 of file katetextrange.cpp.
|
inlinevirtual |
Will this range invalidate itself if it becomes empty?
- Returns
- behavior on becoming empty
Implements KTextEditor::MovingRange.
Definition at line 89 of file katetextrange.h.
|
inlinevirtual |
Retrieve end cursor of this range, read-only.
- Returns
- end cursor
Implements KTextEditor::MovingRange.
Definition at line 131 of file katetextrange.h.
|
inline |
Nonvirtual version of end(), which is faster.
- Returns
- end cursor
Definition at line 137 of file katetextrange.h.
|
inlinevirtual |
Gets the active MovingRangeFeedback for this range.
- Returns
- a pointer to the active MovingRangeFeedback
Implements KTextEditor::MovingRange.
Definition at line 195 of file katetextrange.h.
|
inline |
- Returns
- whether a nonzero attribute is set. This is faster than checking attribute(), because the reference-counting is omitted.
Definition at line 179 of file katetextrange.h.
|
virtual |
Get current insert behaviors.
- Returns
- current insert behaviors
Implements KTextEditor::MovingRange.
Definition at line 89 of file katetextrange.cpp.
|
inline |
Convert this clever range into a dumb one.
Equal to toRange, allowing to use implicit conversion.
- Returns
- normal range
Definition at line 149 of file katetextrange.h.
|
virtual |
Sets the currently active attribute for this range.
This will trigger update of the relevant view parts.
- Parameters
-
attribute Attribute to assign to this range. If null, simply removes the previous Attribute.
remember the new attribute
notify buffer about attribute change, it will propagate the changes notify right view
Implements KTextEditor::MovingRange.
Definition at line 264 of file katetextrange.cpp.
|
virtual |
Set if this range's attribute is only visible in views, not for example prints.
- Parameters
-
onlyForViews attribute only valid for views
just set the value, no need to trigger updates, printing is not interruptable
Implements KTextEditor::MovingRange.
Definition at line 298 of file katetextrange.cpp.
|
virtual |
Set if this range will invalidate itself if it becomes empty.
- Parameters
-
emptyBehavior behavior on becoming empty
nothing to do?
remember value
invalidate range?
Implements KTextEditor::MovingRange.
Definition at line 102 of file katetextrange.cpp.
|
virtual |
Sets the currently active MovingRangeFeedback for this range.
This will trigger evaluation if feedback must be send again (for example if mouse is already inside range).
- Parameters
-
attribute MovingRangeFeedback to assign to this range. If null, simply removes the previous MovingRangeFeedback.
nothing changes, nop
remember the new feedback object
notify buffer about feedback change, it will propagate the changes notify right view
Implements KTextEditor::MovingRange.
Definition at line 278 of file katetextrange.cpp.
|
virtual |
Set insert behaviors.
- Parameters
-
insertBehaviors new insert behaviors
nothing to do?
modify cursors
notify world
Implements KTextEditor::MovingRange.
Definition at line 68 of file katetextrange.cpp.
|
virtual |
Set the range of this range.
A TextRange is not allowed to be empty, as soon as start == end position, it will become automatically invalid!
- Parameters
-
range new range for this clever range
notify buffer about attribute change, it will propagate the changes notify right view
Implements KTextEditor::MovingRange.
Definition at line 122 of file katetextrange.cpp.
|
inline |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Set the range of this range A TextRange is not allowed to be empty, as soon as start == end position, it will become automatically invalid!
- Parameters
-
start new start for this clever range end new end for this clever range
Definition at line 113 of file katetextrange.h.
|
virtual |
Sets the currently active view for this range.
This will trigger update of the relevant view parts, if the view changed. Set view before the attribute, that will avoid not needed redraws.
- Parameters
-
attribute View to assign to this range. If null, simply removes the previous view.
nothing changes, nop
remember the new attribute
notify buffer about attribute change, it will propagate the changes notify all views (can be optimized later)
Implements KTextEditor::MovingRange.
Definition at line 243 of file katetextrange.cpp.
|
virtual |
Set the current Z-depth of this range.
Ranges with smaller Z-depth than others will win during rendering. This will trigger update of the relevant view parts, if the depth changed. Set depth before the attribute, that will avoid not needed redraws. Default is 0.0.
- Parameters
-
zDepth new Z-depth of this range
nothing changes, nop
remember the new attribute
notify buffer about attribute change, it will propagate the changes
Implements KTextEditor::MovingRange.
Definition at line 306 of file katetextrange.cpp.
|
inlinevirtual |
Retrieve start cursor of this range, read-only.
- Returns
- start cursor
Implements KTextEditor::MovingRange.
Definition at line 119 of file katetextrange.h.
|
inline |
Non-virtual version of start(), which is faster.
- Returns
- start cursor
Definition at line 125 of file katetextrange.h.
|
inline |
Convert this clever range into a dumb one.
- Returns
- normal range
Definition at line 143 of file katetextrange.h.
|
inlinevirtual |
Gets the active view for this range.
Might be already invalid, internally only used for pointer comparisons.
- Returns
- a pointer to the active view
Implements KTextEditor::MovingRange.
Definition at line 156 of file katetextrange.h.
|
inlinevirtual |
Gets the current Z-depth of this range.
Ranges with smaller Z-depth than others will win during rendering. Default is 0.0.
- Returns
- current Z-depth of this range
Implements KTextEditor::MovingRange.
Definition at line 226 of file katetextrange.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:55 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.