cantor/src/lib
#include <expression.h>
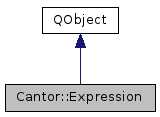
Public Types | |
enum | FinishingBehavior { DoNotDelete, DeleteOnFinish } |
enum | Status { Computing, Done, Error, Interrupted } |
Signals | |
void | gotResult () |
void | idChanged () |
void | needsAdditionalInformation (const QString &question) |
void | statusChanged (Cantor::Expression::Status status) |
Public Member Functions | |
Expression (Session *session) | |
virtual | ~Expression () |
virtual void | addInformation (const QString &information) |
void | clearResult () |
QString | command () |
QString | errorMessage () |
virtual void | evaluate ()=0 |
FinishingBehavior | finishingBehavior () |
int | id () |
virtual void | interrupt ()=0 |
bool | isInternal () |
Result * | result () |
void | saveAdditionalData (KZip *archive) |
Session * | session () |
void | setCommand (const QString &cmd) |
void | setErrorMessage (const QString &cmd) |
void | setFinishingBehavior (FinishingBehavior behavior) |
void | setId (int id) |
void | setInternal (bool internal) |
Status | status () |
QDomElement | toXml (QDomDocument &doc) |
Protected Member Functions | |
virtual QString | additionalLatexHeaders () |
void | setResult (Result *result) |
void | setStatus (Status status) |
Detailed Description
An Expression object is used, to store the information needed when running a command of a Session Evaluation of Expression is an asynchroneous process in most cases, so most of the members of this class are not useful directly after its construction.
Therefore there are signals indicating, when the Expression goes through the different stages of the Running process. An Expression is never constructed directly, but by using Session::evaluateExpression()
Definition at line 49 of file expression.h.
Member Enumeration Documentation
Enum indicating how this Expression behaves on finishing.
Enumerator | |
---|---|
DoNotDelete |
This Expression will not be deleted. This is the normal behaviour. |
DeleteOnFinish |
< The Object will delete itself when finished. This is used for fire-and-forget commands. All output/results will be dropped |
Definition at line 62 of file expression.h.
Enumerator | |
---|---|
Computing |
The Expression is still being computed. |
Done |
The Running of the Expression is finished sucessfully. |
Error |
An Error occurred when running the Expression. |
Interrupted |
The Expression was interrupted by the user while running. |
Definition at line 53 of file expression.h.
Constructor & Destructor Documentation
Expression::Expression | ( | Session * | session | ) |
Expression constructor.
Should only be called from Session::evaulateExpression
- Parameters
-
session the session, this Expression belongs to
Definition at line 75 of file expression.cpp.
|
virtual |
destructor
Definition at line 82 of file expression.cpp.
Member Function Documentation
|
virtual |
Adds some additional information/input to this expression.
this is needed, when the Expression has emitted the needsAdditionalInformation signal, and the user has answered the question. This is used for e.g. if maxima asks wether n+1 is zero or not when running the command "integrate(x^n,x)" This method is part of the InteractiveMode feature
Definition at line 232 of file expression.cpp.
|
protectedvirtual |
Definition at line 237 of file expression.cpp.
void Expression::clearResult | ( | ) |
Deletes the result of this expression.
Definition at line 142 of file expression.cpp.
QString Expression::command | ( | ) |
Returns the command, represented by this Expression.
- Returns
- the command, represented by this Expression
Definition at line 93 of file expression.cpp.
QString Expression::errorMessage | ( | ) |
returns the Error message, if an error occurred during the evaluation of the expression.
- Returns
- the error message
Definition at line 103 of file expression.cpp.
|
pure virtual |
Evaluate the Expression.
before this is called, you should set the Command first This method can be implemented asynchroneous, thus the Evaluation doesn't need to happen in the method, It can also only be scheduled for evaluating.
- See also
- setCommand()
Expression::FinishingBehavior Expression::finishingBehavior | ( | ) |
get the Expressions finishing behaviour
- Returns
- the current finishing behaviour
Definition at line 258 of file expression.cpp.
|
signal |
A Result of the Expression has arrived.
int Expression::id | ( | ) |
Returns the unique id of the Expression.
- Returns
- the unique id of the Expression
Definition at line 242 of file expression.cpp.
|
signal |
the Id of this Expression changed
|
pure virtual |
Interrupt the running of the Expression.
This should set the state to Interrupted.
bool Expression::isInternal | ( | ) |
returns whether or not this expression is internal, or comes from the user
Definition at line 268 of file expression.cpp.
|
signal |
the Expression needs more information for the evaluation
- See also
- addInformation()
- Parameters
-
question question, the user needs to answer
Result * Expression::result | ( | ) |
The result of this Expression.
It can have different types, represented by various subclasses of Result, like text, image, etc. The result will be null, until the computation is completed. When the result changes, the gotResult() signal is emitted. The Result object is owned by the Expression, and will get deleted, as soon as the Expression dies, or newer results appear.
- Returns
- the result of the Expression, 0 if it isn't yet set
Definition at line 137 of file expression.cpp.
void Expression::saveAdditionalData | ( | KZip * | archive | ) |
saves all the data, that can't be saved in xml in an extra file in the archive.
for Example images of plots
- Parameters
-
archive a Zip archive, the data should be stored in
Definition at line 225 of file expression.cpp.
Session * Expression::session | ( | ) |
Returns the Session, this Expression belongs to.
Definition at line 164 of file expression.cpp.
void Expression::setCommand | ( | const QString & | cmd | ) |
Sets the command, represented by this Expression.
- Parameters
-
cmd the command
Definition at line 88 of file expression.cpp.
void Expression::setErrorMessage | ( | const QString & | cmd | ) |
Sets the error message.
- Parameters
-
cmd the error message
- See also
- errorMessage()
Definition at line 98 of file expression.cpp.
void Expression::setFinishingBehavior | ( | Expression::FinishingBehavior | behavior | ) |
set the finishing behaviour
- Parameters
-
behavior the new Finishing Behaviour
Definition at line 253 of file expression.cpp.
void Expression::setId | ( | int | id | ) |
set the id of the Expression.
It should be unique
- Parameters
-
id the new Id
Definition at line 247 of file expression.cpp.
void Expression::setInternal | ( | bool | internal | ) |
mark this expression as an internal expression, so for example latex will not be run on it
Definition at line 263 of file expression.cpp.
|
protected |
Set the result of the Expression.
this will cause gotResult() to be emited The old result will be deleted, and the Expression takes over ownership of the result object, taking care of deleting it.
- Parameters
-
result the new result
Definition at line 108 of file expression.cpp.
|
protected |
Set the status statusChanged will be emitted.
- Parameters
-
status the new status
Definition at line 150 of file expression.cpp.
Expression::Status Expression::status | ( | ) |
Returns the status of this Expression.
- Returns
- the status of this Expression
Definition at line 159 of file expression.cpp.
|
signal |
the status of the Expression has changed.
- Parameters
-
status the new status
QDomElement Expression::toXml | ( | QDomDocument & | doc | ) |
returns an xml representation of this expression used for saving the worksheet
- Parameters
-
doc DomDocument used for storing the information
- Returns
- QDomElemt containing the representation of this Expression
Definition at line 208 of file expression.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:42:50 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.