libkdeedu/keduvocdocument
#include <keduvocdocument.h>
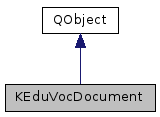
Public Types | |
enum | ErrorCode { NoError = 0, Unknown, InvalidXml, FileTypeUnknown, FileCannotWrite, FileWriterFailed, FileCannotRead, FileReaderFailed, FileDoesNotExist } |
enum | FileDialogMode { Reading, Writing } |
enum | FileType { KvdNone, Automatic, Kvtml, Wql, Pauker, Vokabeln, Xdxf, Csv, Kvtml1 } |
enum | LessonDeletion { DeleteEmptyLesson, DeleteEntriesAndLesson } |
Signals | |
void | docModified (bool mod) |
void | progressChanged (KEduVocDocument *, int curr_percent) |
Public Member Functions | |
KEduVocDocument (QObject *parent=0) | |
~KEduVocDocument () | |
int | appendIdentifier (const KEduVocIdentifier &identifier=KEduVocIdentifier()) |
QString | author () const |
QString | authorContact () const |
QString | category () const |
QString | csvDelimiter () const |
QString | documentComment () const |
QString | generator () const |
KEduVocIdentifier & | identifier (int index) |
const KEduVocIdentifier & | identifier (int index) const |
int | identifierCount () const |
int | indexOfIdentifier (const QString &name) const |
bool | isModified () const |
KEduVocLeitnerBox * | leitnerContainer () |
KEduVocLesson * | lesson () |
QString | license () const |
void | merge (KEduVocDocument *docToMerge, bool matchIdentifiers) |
int | open (const KUrl &url) |
KDE_DEPRECATED void | queryIdentifier (QString &org, QString &trans) const |
void | removeIdentifier (int index) |
int | saveAs (const KUrl &url, FileType ft, const QString &generator) |
void | setAuthor (const QString &author) |
void | setAuthorContact (const QString &authorContact) |
void | setCategory (const QString &category) |
void | setCsvDelimiter (const QString &delimiter) |
void | setDocumentComment (const QString &comment) |
void | setGenerator (const QString &generator) |
void | setIdentifier (int index, const KEduVocIdentifier &lang) |
void | setLicense (const QString &license) |
void | setModified (bool dirty=true) |
KDE_DEPRECATED void | setQueryIdentifier (const QString &org, const QString &trans) |
void | setTitle (const QString &title) |
void | setUrl (const KUrl &url) |
void | setVersion (const QString &ver) |
QString | title () const |
QByteArray | toByteArray (const QString &generator) |
KUrl | url () const |
QString | version () const |
KEduVocWordType * | wordTypeContainer () |
Static Public Member Functions | |
static FileType | detectFileType (const QString &fileName) |
static QString | errorDescription (int errorCode) |
static QString | pattern (FileDialogMode mode) |
Detailed Description
This class contains the expressions of your vocabulary as well as other information about the vocabulary.
Definition at line 44 of file keduvocdocument.h.
Member Enumeration Documentation
the return code when opening/saving
Enumerator | |
---|---|
NoError | |
Unknown | |
InvalidXml | |
FileTypeUnknown | |
FileCannotWrite | |
FileWriterFailed | |
FileCannotRead | |
FileReaderFailed | |
FileDoesNotExist |
Definition at line 63 of file keduvocdocument.h.
used as parameter for pattern
Enumerator | |
---|---|
Reading | |
Writing |
Definition at line 76 of file keduvocdocument.h.
known vocabulary file types
Enumerator | |
---|---|
KvdNone | |
Automatic | |
Kvtml | |
Wql | |
Pauker | |
Vokabeln | |
Xdxf | |
Csv | |
Kvtml1 |
Definition at line 50 of file keduvocdocument.h.
delete only empty lessons or also if they have entries
Enumerator | |
---|---|
DeleteEmptyLesson | |
DeleteEntriesAndLesson |
Definition at line 83 of file keduvocdocument.h.
Constructor & Destructor Documentation
|
explicit |
Constructor for a KDEEdu vocabulary document.
- Parameters
-
parent calling object
Definition at line 143 of file keduvocdocument.cpp.
KEduVocDocument::~KEduVocDocument | ( | ) |
Destructor.
Definition at line 150 of file keduvocdocument.cpp.
Member Function Documentation
int KEduVocDocument::appendIdentifier | ( | const KEduVocIdentifier & | identifier = KEduVocIdentifier() | ) |
Appends a new identifier (usually a language)
- Parameters
-
identifier the identifier to append. If empty default names are used.
- Returns
- the identifier number
Definition at line 699 of file keduvocdocument.cpp.
QString KEduVocDocument::author | ( | ) | const |
- Returns
- the author of the file
Definition at line 756 of file keduvocdocument.cpp.
QString KEduVocDocument::authorContact | ( | ) | const |
- Returns
- the author contact information
Definition at line 767 of file keduvocdocument.cpp.
QString KEduVocDocument::category | ( | ) | const |
- Returns
- the category of the file
- Todo:
- make writer/reader use this
Definition at line 794 of file keduvocdocument.cpp.
QString KEduVocDocument::csvDelimiter | ( | ) | const |
Returns the delimiter (separator) used for csv import and export.
The default is a single tab character
- Returns
- the delimiter used
Definition at line 847 of file keduvocdocument.cpp.
|
static |
Definition at line 163 of file keduvocdocument.cpp.
|
signal |
Emitted when the document becomes modified or saved.
- Returns
- state (true=modified)
QString KEduVocDocument::documentComment | ( | ) | const |
- Returns
- the comment of the file
Definition at line 783 of file keduvocdocument.cpp.
|
static |
Definition at line 892 of file keduvocdocument.cpp.
QString KEduVocDocument::generator | ( | ) | const |
- Returns
- the generator of the file
Definition at line 831 of file keduvocdocument.cpp.
KEduVocIdentifier & KEduVocDocument::identifier | ( | int | index | ) |
Returns the identifier of translation index
.
- Parameters
-
index number of translation 0..x
- Returns
- the language identifier: en=english, de=german, ...
Definition at line 654 of file keduvocdocument.cpp.
const KEduVocIdentifier & KEduVocDocument::identifier | ( | int | index | ) | const |
Const overload of identifier(int);.
Definition at line 646 of file keduvocdocument.cpp.
int KEduVocDocument::identifierCount | ( | ) | const |
- Returns
- the number of different identifiers (usually languages)
Definition at line 694 of file keduvocdocument.cpp.
int KEduVocDocument::indexOfIdentifier | ( | const QString & | name | ) | const |
Determines the index of a given identifier.
- Parameters
-
lang identifier of language
- Returns
- index of identifier, 0 = original, 1..n = translation, -1 = not found
Definition at line 671 of file keduvocdocument.cpp.
bool KEduVocDocument::isModified | ( | ) | const |
- Returns
- the modification state of the doc
Definition at line 688 of file keduvocdocument.cpp.
KEduVocLeitnerBox * KEduVocDocument::leitnerContainer | ( | ) |
Definition at line 726 of file keduvocdocument.cpp.
KEduVocLesson * KEduVocDocument::lesson | ( | ) |
Get the lesson root object.
- Returns
- a pointer to the lesson object
Definition at line 716 of file keduvocdocument.cpp.
QString KEduVocDocument::license | ( | ) | const |
- Returns
- the license of the file
Definition at line 778 of file keduvocdocument.cpp.
void KEduVocDocument::merge | ( | KEduVocDocument * | docToMerge, |
bool | matchIdentifiers | ||
) |
Merges data from another document.
- Parameters
-
docToMerge document containing the data to be merged matchIdentifiers if true only entries having identifiers present in the current document will be mergedurl is empty (or NULL) actual name is preserved
- Todo:
- IMPLEMENT ME
Definition at line 430 of file keduvocdocument.cpp.
int KEduVocDocument::open | ( | const KUrl & | url | ) |
Open a document file.
- Parameters
-
url url to file to open
- Returns
- ErrorCode
- Todo:
- make the readers return int, pass on the error message properly
Definition at line 239 of file keduvocdocument.cpp.
|
static |
Create a string with the supported document types, that can be used as filter in KFileDialog.
It includes also an entry to match all the supported types.
- Parameters
-
mode the mode for the supported document types
- Returns
- the filter string
Definition at line 859 of file keduvocdocument.cpp.
|
signal |
void KEduVocDocument::queryIdentifier | ( | QString & | org, |
QString & | trans | ||
) | const |
Retrieves the identifiers for the current query not written in the new version!
- Parameters
-
org identifier for original trans identifier for translation
Definition at line 800 of file keduvocdocument.cpp.
void KEduVocDocument::removeIdentifier | ( | int | index | ) |
Removes identifier and the according translation in all entries.
- Parameters
-
index number of translation 0..x
Definition at line 679 of file keduvocdocument.cpp.
int KEduVocDocument::saveAs | ( | const KUrl & | url, |
FileType | ft, | ||
const QString & | generator | ||
) |
Saves the data under the given name.
- Parameters
-
url if url is empty (or NULL) actual name is preserved ft the filetype to be used when saving the document generator the name of the application saving the document
- Returns
- ErrorCode
- Todo:
- port me
Definition at line 361 of file keduvocdocument.cpp.
void KEduVocDocument::setAuthor | ( | const QString & | author | ) |
Set the author of the file.
- Parameters
-
author author to set
Definition at line 761 of file keduvocdocument.cpp.
void KEduVocDocument::setAuthorContact | ( | const QString & | authorContact | ) |
Set the author contact info.
- Parameters
-
contact email/contact info to set
Definition at line 772 of file keduvocdocument.cpp.
void KEduVocDocument::setCategory | ( | const QString & | category | ) |
Set the category of the file.
- Parameters
-
category category to set
Definition at line 788 of file keduvocdocument.cpp.
void KEduVocDocument::setCsvDelimiter | ( | const QString & | delimiter | ) |
Sets the delimiter (separator) used for csv import and export.
- Parameters
-
delimiter the delimiter to use
Definition at line 852 of file keduvocdocument.cpp.
void KEduVocDocument::setDocumentComment | ( | const QString & | comment | ) |
Set the comment of the file.
- Parameters
-
comment comment to set
Definition at line 819 of file keduvocdocument.cpp.
void KEduVocDocument::setGenerator | ( | const QString & | generator | ) |
Sets the generator of the file.
Definition at line 825 of file keduvocdocument.cpp.
void KEduVocDocument::setIdentifier | ( | int | index, |
const KEduVocIdentifier & | lang | ||
) |
Sets the identifier of translation.
- Parameters
-
index number of translation 0..x lang the language identifier: en=english, de=german, ...
Definition at line 662 of file keduvocdocument.cpp.
void KEduVocDocument::setLicense | ( | const QString & | license | ) |
Set the license of the file.
- Parameters
-
license license to set
Definition at line 813 of file keduvocdocument.cpp.
void KEduVocDocument::setModified | ( | bool | dirty = true | ) |
Indicates if the document is modified.
- Parameters
-
dirty new state
Definition at line 156 of file keduvocdocument.cpp.
void KEduVocDocument::setQueryIdentifier | ( | const QString & | org, |
const QString & | trans | ||
) |
Sets the identifiers for the current query not written in the new version!
- Parameters
-
org identifier for original trans identifier for translation
Definition at line 806 of file keduvocdocument.cpp.
void KEduVocDocument::setTitle | ( | const QString & | title | ) |
Set the title of the file.
- Parameters
-
title title to set
Definition at line 749 of file keduvocdocument.cpp.
void KEduVocDocument::setUrl | ( | const KUrl & | url | ) |
Sets the URL of the XML file.
Definition at line 736 of file keduvocdocument.cpp.
void KEduVocDocument::setVersion | ( | const QString & | ver | ) |
Sets version of the loaded file.
- Parameters
-
ver the new version
Definition at line 841 of file keduvocdocument.cpp.
QString KEduVocDocument::title | ( | ) | const |
- Returns
- the title of the file
Definition at line 741 of file keduvocdocument.cpp.
QByteArray KEduVocDocument::toByteArray | ( | const QString & | generator | ) |
Definition at line 423 of file keduvocdocument.cpp.
KUrl KEduVocDocument::url | ( | ) | const |
- Returns
- the URL of the XML file
Definition at line 731 of file keduvocdocument.cpp.
QString KEduVocDocument::version | ( | ) | const |
- Returns
- the version of the loaded file
Definition at line 836 of file keduvocdocument.cpp.
KEduVocWordType * KEduVocDocument::wordTypeContainer | ( | ) |
Definition at line 721 of file keduvocdocument.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:37:22 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.