parley
#include <lesson.h>
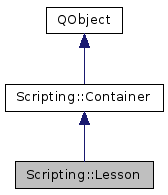
Public Slots | |
void | appendChildLesson (Lesson *child) |
void | appendEntry (Expression *entry) |
void | appendNewEntry (QStringList translations) |
QObject * | childLesson (int row) |
QObject * | childLesson (const QString &name) |
int | childLessonCount () const |
QVariantList | childLessons (bool recursive=false) |
void | clearEntries () |
QVariantList | entries (bool recursive=false) const |
QObject * | entry (int row, bool recursive=false) |
int | entryCount (bool recursive=false) |
QObject * | findChildLesson (const QString &name) |
void | insertChildLesson (int row, Lesson *child) |
void | insertEntry (int index, Expression *entry) |
QObject * | newEntry () |
QObject * | newEntry (QStringList translations) |
void | removeChildLesson (int row) |
void | removeEntry (QObject *entry) |
void | setEntries (QVariantList entries) |
![]() | |
double | averageGrade (int translation, bool recursive) |
int | expressionsOfGrade (int translation, unsigned int grade, bool recursive) |
void | resetGrades (int translation, bool recursive) |
int | row () const |
Public Member Functions | |
Lesson (KEduVocLesson *lesson) | |
Lesson (KEduVocContainer *container) | |
Lesson (const QString &name) | |
~Lesson () | |
![]() | |
Container (KEduVocContainer *container=0) | |
~Container () | |
void | appendChildContainer (Container *child) |
Container * | childContainer (int row) |
Container * | childContainer (const QString &name) |
int | childContainerCount () const |
QVariantList | childContainers () |
KEduVocContainer * | findContainer (const QString &name) |
QString | imageUrl () |
bool | inPractice () |
void | insertChildContainer (int row, Container *child) |
KEduVocContainer * | kEduVocContainer () |
QString | name () |
void | removeChildContainer (int row) |
void | removeTranslation (int translation) |
void | setImageUrl (const QString &url) |
void | setInPractice (bool inPractice) |
void | setName (const QString &name) |
template<class T , class S > | |
QVariantList | toVariantList (QList< T * > objList) const |
Additional Inherited Members | |
![]() | |
static KEduVocContainer::EnumEntriesRecursive | boolToEnum (bool recursive) |
static bool | enumToBool (KEduVocContainer::EnumEntriesRecursive recursive) |
static QList< KEduVocContainer * > | flattenContainer (KEduVocContainer *root) |
![]() | |
KEduVocContainer * | m_container |
![]() | |
QString | imageUrl |
bool | inPractice |
QString | name |
Detailed Description
KEduVocLesson wrapping class for Kross scripts.
The Lesson class gives access to lesson properties, entries and child-lessons. The lesson properties documentation can be found in Container class as well as few function's documentation.
The main way of accessing the lesson entries is with the entries() function which allows you to iterate through all the lesson entries (recursively also) and access their properties and modifiy them (see entries() function example code). For individual entry access use the entry(int) function.
To add new entries to a lesson you can use the following ways:
- newEntry() or newEntry(QStringList) with appendEntry() or insertEntry() function
- appendNewEntry() function [easiest way]
To remove an entry use the removeEntry() function
Constructor & Destructor Documentation
Scripting::Lesson::Lesson | ( | KEduVocLesson * | lesson | ) |
Definition at line 24 of file lesson.cpp.
Scripting::Lesson::Lesson | ( | KEduVocContainer * | container | ) |
Definition at line 29 of file lesson.cpp.
Scripting::Lesson::Lesson | ( | const QString & | name | ) |
Definition at line 35 of file lesson.cpp.
Scripting::Lesson::~Lesson | ( | ) |
Definition at line 42 of file lesson.cpp.
Member Function Documentation
|
inlineslot |
|
slot |
Appends an entry at the end of the lesson.
- Parameters
-
entry The entry to add the lesson
Definition at line 94 of file lesson.cpp.
|
slot |
Creates and appends a new entry with the given translations
.
- Parameters
-
translations A string list with the translations (in same order as their identifiers)
Definition at line 141 of file lesson.cpp.
|
inlineslot |
|
inlineslot |
|
inlineslot |
|
slot |
Returns a list of all the child lessons.
- Parameters
-
recursive If true, then will return the child lessons recursively
- Returns
- A List with Lesson objects (child lessons)
Definition at line 58 of file lesson.cpp.
|
slot |
Removes all the lesson entries (not recursively)
Definition at line 118 of file lesson.cpp.
|
slot |
Returns a list of all the entries of a lesson.
- Parameters
-
recursive If true, then will return recursively all the entries below this lesson
- Returns
- A list of Expression objects (entries)
Definition at line 65 of file lesson.cpp.
|
slot |
Returns the entry of the given row
.
If recursive
is true then it considers all the entries of the sublessons too.
Definition at line 84 of file lesson.cpp.
|
slot |
Returns how many entries are in this lesson (or with sublessons if recursive
is true)
Definition at line 89 of file lesson.cpp.
|
slot |
Searches through all the child lessons (recursively) and returns the first lesson the specified name
.
- Parameters
-
name Name of the lesson to look for
- Returns
- A reference to a lesson if found. 0 otherwise
Definition at line 147 of file lesson.cpp.
|
inlineslot |
|
slot |
Insert an entry on a specific index
.
- Parameters
-
index Index where the entry will be added entry The entry to add (can be created by newEntry() function)
Definition at line 99 of file lesson.cpp.
|
slot |
Creates and returns a new Expression Object.
Definition at line 126 of file lesson.cpp.
|
slot |
Creates and returns a new Expression Object.
- Parameters
-
translations A list with the translations of this entry (must be in correct order)
- Returns
- A new Expression object
Definition at line 136 of file lesson.cpp.
|
inlineslot |
|
slot |
Removes an entry from this lesson.
- Parameters
-
entry A reference to the entry to remove
- Note
- parameter has to be QObject (tried with Expression * entry but didn't work)
Definition at line 104 of file lesson.cpp.
|
slot |
Definition at line 70 of file lesson.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:42:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.