rocs/RocsCore
#include <GraphNode.h>
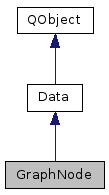
Public Slots | |
QScriptValue | adj_edges () |
QScriptValue | adj_nodes () |
QScriptValue | connected_edges (Data *n) |
QScriptValue | input_edges () |
QScriptValue | output_edges () |
QScriptValue | overlay_edges (int overlay) |
![]() | |
void | add_property (const QString &name, const QString &value) |
void | addDynamicProperty (const QString &property, const QVariant &value) |
QScriptValue | adj_data () |
QScriptValue | adj_pointers () |
QScriptValue | adj_pointers (int pointerType) |
QScriptValue | connected_pointers (Data *n) |
QScriptValue | input_pointers () |
QScriptValue | input_pointers (int pointerType) |
QScriptValue | output_pointers () |
QScriptValue | output_pointers (int pointerType) |
void | remove () |
void | remove_property (const QString &name) |
void | removeDynamicProperty (const QString &property) |
void | renameDynamicProperty (const QString &oldName, const QString &newName) |
void | self_remove () |
QScriptValue | set_type (int type) |
void | setColor (const QVariant &s) |
void | setDataType (int dataType) |
void | setPos (qreal x, qreal y) |
void | setVisible (bool visible) |
void | setWidth (double w) |
void | setX (int x) |
void | setY (int y) |
QScriptValue | type () |
void | updateDynamicProperty (const QString &property) |
void | updatePointerList () |
Signals | |
void | scriptError (const QString &message) |
![]() | |
void | colorChanged (const QColor &c) |
void | dataTypeChanged (int dataType) |
void | pointerListChanged () |
void | posChanged (const QPointF &p) |
void | propertyAdded (const QString &name) |
void | propertyChanged (const QString &name) |
void | propertyRemoved (const QString &name) |
void | removed () |
void | useColorChanged (bool b) |
void | visibilityChanged (bool visible) |
void | widthChanged (double w) |
Public Member Functions | |
GraphNode (DataStructurePtr parent, int uniqueIdentifier, int dataType) | |
virtual | ~GraphNode () |
Q_INVOKABLE QScriptValue | edges () |
Q_INVOKABLE QScriptValue | edges (int type) |
Q_INVOKABLE QScriptValue | edgesTo (Data *node) |
Q_INVOKABLE QScriptValue | inEdges () |
Q_INVOKABLE QScriptValue | inEdges (int type) |
Q_INVOKABLE QScriptValue | neighbors () |
Q_INVOKABLE QScriptValue | outEdges () |
Q_INVOKABLE QScriptValue | outEdges (int type) |
virtual void | setEngine (QScriptEngine *_engine) |
![]() | |
virtual | ~Data () |
void | addPointer (PointerPtr pointer) |
DataList | adjacentDataList () const |
QVariant | color () const |
PointerPtr | createPointer (DataPtr to) |
DataStructurePtr | dataStructure () const |
int | dataType () const |
QScriptEngine * | engine () const |
virtual DataPtr | getData () const |
QString | icon () const |
int | identifier () const |
PointerList & | inPointerList () const |
bool | isVisible () const |
PointerList & | outPointerList () const |
PointerList | pointerList () const |
PointerList | pointerList (DataPtr to) const |
QList< QString > | properties () const |
void | remove (PointerPtr e) |
QScriptValue | scriptValue () const |
qreal | width () const |
qreal | x () const |
qreal | y () const |
Static Public Member Functions | |
static DataPtr | create (DataStructurePtr parent, int uniqueIdentifier, int dataType) |
![]() | |
static DataPtr | create (DataStructurePtr dataStructure, int uniqueIdentifier, int dataType) |
Additional Inherited Members | |
![]() | |
Data (DataStructurePtr dataStructure, int uniqueIdentifer, int dataType) | |
bool | eventFilter (QObject *obj, QEvent *event) |
![]() | |
template<typename T > | |
static DataPtr | create (DataStructurePtr parent, int uniqueIdentifier, int dataType) |
![]() | |
QVariant | color |
int | id |
qreal | width |
qreal | x |
qreal | y |
Detailed Description
Definition at line 24 of file GraphNode.h.
Constructor & Destructor Documentation
GraphNode::GraphNode | ( | DataStructurePtr | parent, |
int | uniqueIdentifier, | ||
int | dataType | ||
) |
Definition at line 31 of file GraphNode.cpp.
|
virtual |
Definition at line 41 of file GraphNode.cpp.
Member Function Documentation
|
slot |
Definition at line 97 of file GraphNode.cpp.
|
slot |
Definition at line 89 of file GraphNode.cpp.
|
slot |
Definition at line 105 of file GraphNode.cpp.
|
static |
Definition at line 26 of file GraphNode.cpp.
QScriptValue GraphNode::edges | ( | ) |
Definition at line 45 of file GraphNode.cpp.
QScriptValue GraphNode::edges | ( | int | type | ) |
Definition at line 50 of file GraphNode.cpp.
QScriptValue GraphNode::edgesTo | ( | Data * | node | ) |
Definition at line 81 of file GraphNode.cpp.
QScriptValue GraphNode::inEdges | ( | ) |
Definition at line 55 of file GraphNode.cpp.
QScriptValue GraphNode::inEdges | ( | int | type | ) |
Definition at line 70 of file GraphNode.cpp.
|
slot |
Definition at line 116 of file GraphNode.cpp.
QScriptValue GraphNode::neighbors | ( | ) |
Definition at line 75 of file GraphNode.cpp.
QScriptValue GraphNode::outEdges | ( | ) |
Definition at line 60 of file GraphNode.cpp.
QScriptValue GraphNode::outEdges | ( | int | type | ) |
Definition at line 65 of file GraphNode.cpp.
|
slot |
Definition at line 124 of file GraphNode.cpp.
|
slot |
Gives array of edges at specified overlay if present.
Otherwise if overlay does not exist returns empty array. Function respects type of graph, i.e., returns either output edges or output plus input edges if graph is directed or undirected, respectively.
- Parameters
-
overlay is identifier for overlay.
Definition at line 132 of file GraphNode.cpp.
|
signal |
|
virtual |
Set script engine.
The engine must be set to access the script value.
Reimplemented from Data.
Definition at line 36 of file GraphNode.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:42:26 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.