rocs/RocsCore
#include <ListStructure.h>
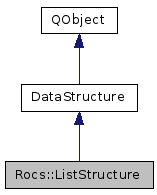
Public Member Functions | |
ListStructure (Document *parent=0) | |
virtual | ~ListStructure () |
Q_INVOKABLE QScriptValue | begin () |
DataPtr | createData (const QString &name, int dataType) |
Q_INVOKABLE QScriptValue | createNode (int type) |
Q_INVOKABLE QScriptValue | createNode () |
PointerPtr | createPointer (DataPtr from, DataPtr to, int pointerType) |
Q_INVOKABLE QScriptValue | head () |
void | importStructure (DataStructurePtr other) |
void | remove (DataPtr n) |
void | remove (PointerPtr e) |
Q_INVOKABLE void | setBegin (Data *node) |
Q_INVOKABLE void | setHead (Data *headNode) |
![]() | |
virtual void | cleanUpBeforeConvert () |
const DataList | dataList (int dataType) const |
DataList | dataListAll () const |
Document * | document () const |
QScriptEngine * | engine () const |
virtual DataStructurePtr | getDataStructure () const |
const QList< GroupPtr > | groups () const |
bool | isDataVisible (int dataType) const |
bool | isPointerVisible (int pointerType) const |
QString | name () const |
virtual QMap< QString, QString > | pluginProperties () const |
PointerList | pointerListAll () const |
const PointerList | pointers (int pointerType) const |
bool | readOnly () const |
QScriptValue | scriptValue () const |
virtual void | setEngine (QScriptEngine *engine) |
virtual void | setPluginProperty (const QString &, const QString &) |
void | setReadOnly (bool r) |
void | updateData (DataPtr data) |
void | updatePointer (PointerPtr pointer) |
Static Public Member Functions | |
static DataStructurePtr | create (Document *parent) |
static DataStructurePtr | create (DataStructurePtr other, Document *parent) |
![]() | |
static DataStructurePtr | create (Document *parent=0) |
static DataStructurePtr | create (DataStructurePtr other, Document *parent=0) |
Properties | |
QString | name |
![]() | |
QString | name |
Additional Inherited Members | |
![]() | |
void | add_property (const QString &name, const QVariant &value) |
virtual DataList | addDataList (DataList dataList) |
virtual DataList | addDataList (QList< QPair< QString, QPointF > > dataList, int dataType) |
void | addDynamicProperty (const QString &property, const QVariant &value=QVariant(0)) |
virtual GroupPtr | addGroup (const QString &name) |
virtual DataPtr | createData (const QString &name, const QPointF &point, int dataType) |
DataPtr | getData (int uniqueIdentifier) |
virtual void | remove (GroupPtr group) |
void | remove () |
void | remove_property (const QString &name) |
void | removeDynamicProperty (const QString &property) |
void | renameDynamicProperty (const QString &oldName, const QString &newName) |
void | setName (const QString &s) |
![]() | |
void | changed () |
void | dataCreated (DataPtr n) |
void | dataPositionChanged (const QPointF) |
void | nameChanged (const QString &name) |
void | pointerCreated (PointerPtr e) |
void | scriptError (const QString &message) |
![]() | |
DataStructure (Document *parent=0) | |
virtual | ~DataStructure () |
DataPtr | addData (DataPtr data) |
PointerPtr | addPointer (PointerPtr pointer) |
int | generateUniqueIdentifier () |
void | initialize () |
![]() | |
template<typename T > | |
static DataStructurePtr | create (Document *parent=0) |
template<typename T > | |
static DataStructurePtr | create (DataStructurePtr other, Document *parent=0) |
Detailed Description
Definition at line 31 of file ListStructure.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 40 of file ListStructure.cpp.
|
virtual |
Definition at line 94 of file ListStructure.cpp.
Member Function Documentation
QScriptValue Rocs::ListStructure::begin | ( | ) |
|
static |
Definition at line 28 of file ListStructure.cpp.
|
static |
Definition at line 33 of file ListStructure.cpp.
|
virtual |
Internal method to create new graph node.
Use
- See also
- Data::create(...) for creating new nodes.
- Parameters
-
name is the name of the node dataType is the type of this node, defaults to 0
- Returns
- the created node as DataPtr
Reimplemented from DataStructure.
Definition at line 113 of file ListStructure.cpp.
QScriptValue Rocs::ListStructure::createNode | ( | int | type | ) |
Creates a new data element and return it.
If the specified data type is not registered, no data element is created.
- Parameters
-
type is the data type of the created node
- Returns
- script value for the new node
Definition at line 176 of file ListStructure.cpp.
QScriptValue Rocs::ListStructure::createNode | ( | ) |
Creates a new data element and return it.
- Returns
- script value for the new node
Definition at line 171 of file ListStructure.cpp.
|
virtual |
Internal method to create new graph edge.
Use
- See also
- Pointer::create(...) for creating new edges.
- Parameters
-
from is the origin of the new edge to is the target of the new edge pointerType is the type of this edge, defaults to 0
- Returns
- the created edge as PointerPtr
Reimplemented from DataStructure.
Definition at line 103 of file ListStructure.cpp.
QScriptValue Rocs::ListStructure::head | ( | ) |
- Returns
- head node of linked list
Definition at line 135 of file ListStructure.cpp.
|
virtual |
overwrites the current DataStructure with all values (Data and Pointer) from the given datastructure object.
- Parameters
-
other the data structure that shall be imported
- Returns
- void
Reimplemented from DataStructure.
Definition at line 48 of file ListStructure.cpp.
|
virtual |
Remove data
from data structure and (if necessary) destroys the data object.
It is valid to call this method more than once for the same data object.
- Parameters
-
data the data element that shall be removed
Reimplemented from DataStructure.
Definition at line 127 of file ListStructure.cpp.
|
virtual |
Remove pointer
from data structure and (if necessary) destroys the pointer object.
It is valid to call this method more than once for the same pointer object.
- Parameters
-
pointer the pointer that shall be removed
Reimplemented from DataStructure.
Definition at line 166 of file ListStructure.cpp.
void Rocs::ListStructure::setBegin | ( | Data * | node | ) |
void Rocs::ListStructure::setHead | ( | Data * | headNode | ) |
Set head node of linked list.
- Parameters
-
node is the
Definition at line 140 of file ListStructure.cpp.
Property Documentation
|
readwrite |
Definition at line 34 of file ListStructure.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:42:26 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.