rocs/RocsCore
#include <RootedTreeStructure.h>
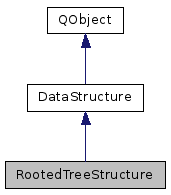
Public Slots | |
QScriptValue | add_data (const QString &name) |
QScriptValue | root_node () const |
void | set_root_node (RootedTreeNode *node) |
void | setShowAllPointers (const bool v) |
![]() | |
void | add_property (const QString &name, const QVariant &value) |
virtual DataList | addDataList (DataList dataList) |
virtual DataList | addDataList (QList< QPair< QString, QPointF > > dataList, int dataType) |
void | addDynamicProperty (const QString &property, const QVariant &value=QVariant(0)) |
virtual GroupPtr | addGroup (const QString &name) |
virtual DataPtr | createData (const QString &name, const QPointF &point, int dataType) |
DataPtr | getData (int uniqueIdentifier) |
virtual void | remove (DataPtr data) |
virtual void | remove (PointerPtr pointer) |
virtual void | remove (GroupPtr group) |
void | remove () |
void | remove_property (const QString &name) |
void | removeDynamicProperty (const QString &property) |
void | renameDynamicProperty (const QString &oldName, const QString &newName) |
void | setName (const QString &s) |
Signals | |
void | showPointersChanged (const bool) |
![]() | |
void | changed () |
void | dataCreated (DataPtr n) |
void | dataPositionChanged (const QPointF) |
void | nameChanged (const QString &name) |
void | pointerCreated (PointerPtr e) |
void | scriptError (const QString &message) |
Public Member Functions | |
RootedTreeStructure (Document *parent=0) | |
~RootedTreeStructure () | |
DataPtr | createData (const QString &name, int dataType=0) |
PointerPtr | createPointer (DataPtr from, DataPtr to, int dataType=0) |
void | importStructure (DataStructurePtr other) |
bool | isShowingAllPointers () const |
DataPtr | rootNode () const |
void | setEngine (QScriptEngine *engine) |
![]() | |
virtual void | cleanUpBeforeConvert () |
const DataList | dataList (int dataType) const |
DataList | dataListAll () const |
Document * | document () const |
QScriptEngine * | engine () const |
virtual DataStructurePtr | getDataStructure () const |
const QList< GroupPtr > | groups () const |
bool | isDataVisible (int dataType) const |
bool | isPointerVisible (int pointerType) const |
QString | name () const |
virtual QMap< QString, QString > | pluginProperties () const |
PointerList | pointerListAll () const |
const PointerList | pointers (int pointerType) const |
bool | readOnly () const |
QScriptValue | scriptValue () const |
virtual void | setPluginProperty (const QString &, const QString &) |
void | setReadOnly (bool r) |
void | updateData (DataPtr data) |
void | updatePointer (PointerPtr pointer) |
Static Public Member Functions | |
static DataStructurePtr | create (Document *parent) |
static DataStructurePtr | create (DataStructurePtr other, Document *parent) |
![]() | |
static DataStructurePtr | create (Document *parent=0) |
static DataStructurePtr | create (DataStructurePtr other, Document *parent=0) |
Properties | |
RootedTreeNode * | m_rootNode |
bool | m_showPointers |
bool | ShowAllPointers |
![]() | |
QString | name |
Additional Inherited Members | |
![]() | |
DataStructure (Document *parent=0) | |
virtual | ~DataStructure () |
DataPtr | addData (DataPtr data) |
PointerPtr | addPointer (PointerPtr pointer) |
int | generateUniqueIdentifier () |
void | initialize () |
![]() | |
template<typename T > | |
static DataStructurePtr | create (Document *parent=0) |
template<typename T > | |
static DataStructurePtr | create (DataStructurePtr other, Document *parent=0) |
Detailed Description
Definition at line 27 of file RootedTreeStructure.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 59 of file RootedTreeStructure.cpp.
RootedTreeStructure::~RootedTreeStructure | ( | ) |
Definition at line 133 of file RootedTreeStructure.cpp.
Member Function Documentation
|
slot |
Create a new data (tree node) Note that it only create but not insert it into the tree.
Definition at line 138 of file RootedTreeStructure.cpp.
|
static |
Definition at line 45 of file RootedTreeStructure.cpp.
|
static |
Definition at line 51 of file RootedTreeStructure.cpp.
|
virtual |
Reimplemented from DataStructure.
Definition at line 199 of file RootedTreeStructure.cpp.
|
virtual |
FIXME addeding multiple pointers.
Reimplemented from DataStructure.
Definition at line 147 of file RootedTreeStructure.cpp.
|
virtual |
overwrites the current DataStructure with all values (Data and Pointer) from the given datastructure object.
- Parameters
-
other the data structure that shall be imported
- Returns
- void
Reimplemented from DataStructure.
Definition at line 69 of file RootedTreeStructure.cpp.
bool RootedTreeStructure::isShowingAllPointers | ( | ) | const |
return true if all the pointers need to be draw.
Definition at line 240 of file RootedTreeStructure.cpp.
|
slot |
Definition at line 210 of file RootedTreeStructure.cpp.
DataPtr RootedTreeStructure::rootNode | ( | ) | const |
Definition at line 225 of file RootedTreeStructure.cpp.
|
slot |
Definition at line 219 of file RootedTreeStructure.cpp.
|
virtual |
Reimplemented from DataStructure.
Definition at line 233 of file RootedTreeStructure.cpp.
|
slot |
set if all the pointers should be draw or not.
All pointers is a complete draw of this data structure, including pointers to parents and children division on nodes.
Definition at line 245 of file RootedTreeStructure.cpp.
|
signal |
signal emitted on change of pointers visibility
Property Documentation
RootedTreeNode* RootedTreeStructure::m_rootNode |
Definition at line 33 of file RootedTreeStructure.h.
bool RootedTreeStructure::m_showPointers |
Definition at line 35 of file RootedTreeStructure.h.
|
readwrite |
Definition at line 31 of file RootedTreeStructure.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:42:26 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.