KMediaPlayer
#include <player.h>
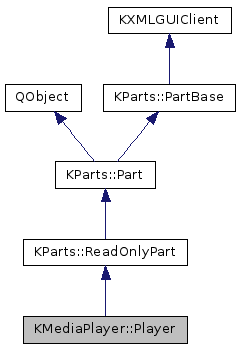
Public Types | |
enum | State { Empty, Stop, Pause, Play } |
![]() | |
enum | ReverseStateChange |
Public Slots | |
virtual void | pause (void)=0 |
virtual void | play (void)=0 |
virtual void | seek (qlonglong msec)=0 |
void | setLooping (bool) |
virtual void | stop (void)=0 |
![]() | |
virtual bool | openUrl (const KUrl &url) |
Signals | |
void | loopingChanged (bool) |
void | stateChanged (int) |
![]() | |
void | canceled (const QString &errMsg) |
void | completed () |
void | completed (bool pendingAction) |
void | started (KIO::Job *) |
void | urlChanged (const KUrl &url) |
![]() | |
void | setStatusBarText (const QString &text) |
void | setWindowCaption (const QString &caption) |
Public Member Functions | |
Player (QObject *parent) | |
Player (QWidget *parentWidget, const char *widgetName, QObject *parent) | |
virtual | ~Player (void) |
virtual bool | hasLength (void) const =0 |
bool | isLooping (void) const |
virtual bool | isSeekable (void) const =0 |
virtual qlonglong | length (void) const =0 |
virtual qlonglong | position (void) const =0 |
int | state (void) const |
virtual View * | view (void)=0 |
![]() | |
ReadOnlyPart (QObject *parent=0) | |
virtual | ~ReadOnlyPart () |
OpenUrlArguments | arguments () const |
BrowserExtension * | browserExtension () const |
bool | closeStream () |
virtual bool | closeUrl () |
bool | isProgressInfoEnabled () const |
bool | openStream (const QString &mimeType, const KUrl &url) |
void | setArguments (const OpenUrlArguments &arguments) |
void | setProgressInfoEnabled (bool show) |
void | showProgressInfo (bool show) |
KUrl | url () const |
bool | writeStream (const QByteArray &data) |
![]() | |
Part (QObject *parent=0) | |
virtual | ~Part () |
virtual void | embed (QWidget *parentWidget) |
virtual Part * | hitTest (QWidget *widget, const QPoint &globalPos) |
KIconLoader * | iconLoader () |
bool | isSelectable () const |
PartManager * | manager () const |
void | setAutoDeletePart (bool autoDeletePart) |
void | setAutoDeleteWidget (bool autoDeleteWidget) |
virtual void | setManager (PartManager *manager) |
virtual void | setSelectable (bool selectable) |
virtual QWidget * | widget () |
![]() | |
KXMLGUIClient () | |
KXMLGUIClient (KXMLGUIClient *parent) | |
virtual | ~KXMLGUIClient () |
QAction * | action (const char *name) const |
virtual QAction * | action (const QDomElement &element) const |
virtual KActionCollection * | actionCollection () const |
void | addStateActionDisabled (const QString &state, const QString &action) |
void | addStateActionEnabled (const QString &state, const QString &action) |
void | beginXMLPlug (QWidget *) |
QList< KXMLGUIClient * > | childClients () |
KXMLGUIBuilder * | clientBuilder () const |
virtual KComponentData | componentData () const |
virtual QDomDocument | domDocument () const |
void | endXMLPlug () |
KXMLGUIFactory * | factory () const |
StateChange | getActionsToChangeForState (const QString &state) |
void | insertChildClient (KXMLGUIClient *child) |
virtual QString | localXMLFile () const |
KXMLGUIClient * | parentClient () const |
void | plugActionList (const QString &name, const QList< QAction * > &actionList) |
void | prepareXMLUnplug (QWidget *) |
void | reloadXML () |
void | removeChildClient (KXMLGUIClient *child) |
void | replaceXMLFile (const QString &xmlfile, const QString &localxmlfile, bool merge=false) |
void | setClientBuilder (KXMLGUIBuilder *builder) |
void | setFactory (KXMLGUIFactory *factory) |
void | setXMLGUIBuildDocument (const QDomDocument &doc) |
void | unplugActionList (const QString &name) |
virtual QString | xmlFile () const |
QDomDocument | xmlguiBuildDocument () const |
Protected Slots | |
void | setState (int) |
![]() | |
void | slotWidgetDestroyed () |
Properties | |
bool | hasLength |
qlonglong | length |
bool | looping |
qlonglong | position |
bool | seekable |
int | state |
![]() | |
KUrl | url |
Additional Inherited Members | |
![]() | |
static QString | findMostRecentXMLFile (const QStringList &files, QString &doc) |
![]() | |
ReadOnlyPart (ReadOnlyPartPrivate &dd, QObject *parent) | |
void | abortLoad () |
virtual void | guiActivateEvent (GUIActivateEvent *event) |
bool | isLocalFileTemporary () const |
QString | localFilePath () const |
virtual bool | openFile () |
void | setLocalFilePath (const QString &localFilePath) |
void | setLocalFileTemporary (bool temp) |
void | setUrl (const KUrl &url) |
![]() | |
Part (PartPrivate &dd, QObject *parent) | |
virtual void | customEvent (QEvent *event) |
QWidget * | hostContainer (const QString &containerName) |
void | loadPlugins () |
virtual void | partActivateEvent (PartActivateEvent *event) |
virtual void | partSelectEvent (PartSelectEvent *event) |
virtual void | setWidget (QWidget *widget) |
![]() | |
void | loadStandardsXmlFile () |
virtual void | setComponentData (const KComponentData &componentData) |
virtual void | setDOMDocument (const QDomDocument &document, bool merge=false) |
virtual void | setLocalXMLFile (const QString &file) |
virtual void | setXML (const QString &document, bool merge=false) |
virtual void | setXMLFile (const QString &file, bool merge=false, bool setXMLDoc=true) |
virtual void | stateChanged (const QString &newstate, ReverseStateChange reverse=StateNoReverse) |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
Player is the center of the KMediaPlayer interface.
It provides all of the necessary media player operations, and optionally provides the GUI to control them.
There are two servicetypes for Player: KMediaPlayer/Player and KMediaPlayer/Engine. KMediaPlayer/Player provides a widget (accessable through view as well as XML GUI KActions. KMediaPlayer/Engine omits the user interface facets, for those who wish to provide their own interface.
Member Enumeration Documentation
Constructor & Destructor Documentation
KMediaPlayer::Player::Player | ( | QObject * | parent | ) |
This constructor is what to use when no GUI is required, as in the case of a KMediaPlayer/Engine.
Definition at line 36 of file player.cpp.
This constructor is what to use when a GUI is required, as in the case of a KMediaPlayer/Player.
Definition at line 27 of file player.cpp.
|
virtual |
Definition at line 45 of file player.cpp.
Member Function Documentation
|
pure virtual |
Returns whether the current track has a length.
Some streams are endless, and do not have one.
bool KMediaPlayer::Player::isLooping | ( | void | ) | const |
Return the current looping state.
Definition at line 58 of file player.cpp.
|
pure virtual |
Returns whether the current track honors seek requests.
|
pure virtual |
Returns the length of the current track.
|
signal |
Emitted when the looping state is changed.
|
pure virtualslot |
Pause playback of the media track.
|
pure virtualslot |
Begin playing the media track.
|
pure virtual |
Returns the current playback position in the track.
|
pure virtualslot |
Move the current playback position to the specified time in milliseconds, if the track is seekable.
Some streams may not be seeked.
|
slot |
Set whether the Player should continue playing at the beginning of the track when the end of the track is reached.
Definition at line 49 of file player.cpp.
|
protectedslot |
Implementers use this to control what users see as the current state.
Definition at line 63 of file player.cpp.
int KMediaPlayer::Player::state | ( | void | ) | const |
Return the current state of the player.
Definition at line 72 of file player.cpp.
|
signal |
Emitted when the state changes.
|
pure virtualslot |
Stop playback of the media track and return to the beginning.
|
pure virtual |
Property Documentation
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:52:16 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.