KDECore
#include <kar.h>
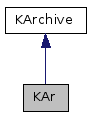
Public Member Functions | |
KAr (const QString &filename) | |
KAr (QIODevice *dev) | |
virtual | ~KAr () |
![]() | |
virtual | ~KArchive () |
bool | addLocalDirectory (const QString &path, const QString &destName) |
bool | addLocalFile (const QString &fileName, const QString &destName) |
virtual bool | close () |
QIODevice * | device () const |
const KArchiveDirectory * | directory () const |
QString | fileName () const |
virtual bool | finishWriting (qint64 size) |
bool | isOpen () const |
QIODevice::OpenMode | mode () const |
virtual bool | open (QIODevice::OpenMode mode) |
virtual bool | prepareWriting (const QString &name, const QString &user, const QString &group, qint64 size, mode_t perm=0100644, time_t atime=UnknownTime, time_t mtime=UnknownTime, time_t ctime=UnknownTime) |
virtual bool | writeData (const char *data, qint64 size) |
virtual bool | writeDir (const QString &name, const QString &user, const QString &group, mode_t perm=040755, time_t atime=UnknownTime, time_t mtime=UnknownTime, time_t ctime=UnknownTime) |
virtual bool | writeFile (const QString &name, const QString &user, const QString &group, const char *data, qint64 size, mode_t perm=0100644, time_t atime=UnknownTime, time_t mtime=UnknownTime, time_t ctime=UnknownTime) |
virtual bool | writeSymLink (const QString &name, const QString &target, const QString &user, const QString &group, mode_t perm=0120755, time_t atime=UnknownTime, time_t mtime=UnknownTime, time_t ctime=UnknownTime) |
Protected Member Functions | |
virtual bool | closeArchive () |
virtual bool | doFinishWriting (qint64 size) |
virtual bool | doPrepareWriting (const QString &name, const QString &user, const QString &group, qint64 size, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
virtual bool | doWriteDir (const QString &name, const QString &user, const QString &group, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
virtual bool | doWriteSymLink (const QString &name, const QString &target, const QString &user, const QString &group, mode_t perm, time_t atime, time_t mtime, time_t ctime) |
virtual bool | openArchive (QIODevice::OpenMode mode) |
virtual void | virtual_hook (int id, void *data) |
![]() | |
KArchive (const QString &fileName) | |
KArchive (QIODevice *dev) | |
virtual bool | createDevice (QIODevice::OpenMode mode) |
KArchiveDirectory * | findOrCreate (const QString &path) |
virtual KArchiveDirectory * | rootDir () |
void | setDevice (QIODevice *dev) |
void | setRootDir (KArchiveDirectory *rootDir) |
Additional Inherited Members | |
![]() | |
enum | { UnknownTime = static_cast<time_t>( -1 ) } |
Detailed Description
KAr is a class for reading archives in ar format.
Writing is not supported. A class for reading ar archives.
Constructor & Destructor Documentation
KAr::KAr | ( | const QString & | filename | ) |
KAr::KAr | ( | QIODevice * | dev | ) |
Creates an instance that operates on the given device.
The device can be compressed (KFilterDev) or not (QFile, etc.).
- Parameters
-
dev the device to read from
|
virtual |
Member Function Documentation
|
protectedvirtual |
Called after writing the data.
This virtual method must be implemented by subclasses.
- Parameters
-
size the size of the file
- See also
- finishWriting()
Implements KArchive.
|
protectedvirtual |
This virtual method must be implemented by subclasses.
Depending on the archive type not all metadata might be used.
- Parameters
-
name the name of the file user the user that owns the file group the group that owns the file size the size of the file perm permissions of the file. Use 0100644 if you don't have any more specific permissions to set. atime time the file was last accessed mtime modification time of the file ctime time of last status change
- See also
- prepareWriting
Implements KArchive.
|
protectedvirtual |
Write a directory to the archive.
This virtual method must be implemented by subclasses.
Depending on the archive type not all metadata might be used.
- Parameters
-
name the name of the directory user the user that owns the directory group the group that owns the directory perm permissions of the directory. Use 040755 if you don't have any other information. atime time the file was last accessed mtime modification time of the file ctime time of last status change
- See also
- writeDir
Implements KArchive.
|
protectedvirtual |
Writes a symbolic link to the archive.
This virtual method must be implemented by subclasses.
- Parameters
-
name name of symbolic link target target of symbolic link user the user that owns the directory group the group that owns the directory perm permissions of the directory atime time the file was last accessed mtime modification time of the file ctime time of last status change
- See also
- writeSymLink
Implements KArchive.
|
protectedvirtual |
|
protectedvirtual |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:47:10 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.