KDECore
#include <karchive.h>
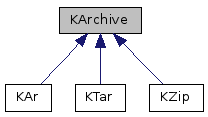
Public Types | |
enum | { UnknownTime = static_cast<time_t>( -1 ) } |
Public Member Functions | |
virtual | ~KArchive () |
bool | addLocalDirectory (const QString &path, const QString &destName) |
bool | addLocalFile (const QString &fileName, const QString &destName) |
virtual bool | close () |
QIODevice * | device () const |
const KArchiveDirectory * | directory () const |
QString | fileName () const |
virtual bool | finishWriting (qint64 size) |
bool | isOpen () const |
QIODevice::OpenMode | mode () const |
virtual bool | open (QIODevice::OpenMode mode) |
virtual bool | prepareWriting (const QString &name, const QString &user, const QString &group, qint64 size, mode_t perm=0100644, time_t atime=UnknownTime, time_t mtime=UnknownTime, time_t ctime=UnknownTime) |
virtual bool | writeData (const char *data, qint64 size) |
virtual bool | writeDir (const QString &name, const QString &user, const QString &group, mode_t perm=040755, time_t atime=UnknownTime, time_t mtime=UnknownTime, time_t ctime=UnknownTime) |
virtual bool | writeFile (const QString &name, const QString &user, const QString &group, const char *data, qint64 size, mode_t perm=0100644, time_t atime=UnknownTime, time_t mtime=UnknownTime, time_t ctime=UnknownTime) |
virtual bool | writeSymLink (const QString &name, const QString &target, const QString &user, const QString &group, mode_t perm=0120755, time_t atime=UnknownTime, time_t mtime=UnknownTime, time_t ctime=UnknownTime) |
Protected Member Functions | |
KArchive (const QString &fileName) | |
KArchive (QIODevice *dev) | |
virtual bool | closeArchive ()=0 |
virtual bool | createDevice (QIODevice::OpenMode mode) |
virtual bool | doFinishWriting (qint64 size)=0 |
virtual bool | doPrepareWriting (const QString &name, const QString &user, const QString &group, qint64 size, mode_t perm, time_t atime, time_t mtime, time_t ctime)=0 |
virtual bool | doWriteDir (const QString &name, const QString &user, const QString &group, mode_t perm, time_t atime, time_t mtime, time_t ctime)=0 |
virtual bool | doWriteSymLink (const QString &name, const QString &target, const QString &user, const QString &group, mode_t perm, time_t atime, time_t mtime, time_t ctime)=0 |
KArchiveDirectory * | findOrCreate (const QString &path) |
virtual bool | openArchive (QIODevice::OpenMode mode)=0 |
virtual KArchiveDirectory * | rootDir () |
void | setDevice (QIODevice *dev) |
void | setRootDir (KArchiveDirectory *rootDir) |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
KArchive is a base class for reading and writing archives.
generic class for reading/writing archives
Definition at line 43 of file karchive.h.
Member Enumeration Documentation
anonymous enum |
Enumerator | |
---|---|
UnknownTime |
Definition at line 141 of file karchive.h.
Constructor & Destructor Documentation
|
protected |
Base constructor (protected since this is a pure virtual class).
- Parameters
-
fileName is a local path (e.g. "/tmp/myfile.ext"), from which the archive will be read from, or into which the archive will be written, depending on the mode given to open().
Definition at line 81 of file karchive.cpp.
|
protected |
Base constructor (protected since this is a pure virtual class).
- Parameters
-
dev the I/O device where the archive reads its data Note that this can be a file, but also a data buffer, a compression filter, etc. For a file in writing mode it is better to use the other constructor though, to benefit from the use of KSaveFile when saving.
Definition at line 90 of file karchive.cpp.
|
virtual |
Definition at line 96 of file karchive.cpp.
Member Function Documentation
Writes a local directory into the archive, including all its contents, recursively.
Calls addLocalFile for each file to be added.
Since KDE 3.2 it will also add a path
that is a symbolic link to a directory. The symbolic link will be dereferenced and the content of the directory it is pointing to added recursively. However, symbolic links under path
will be stored as is.
- Parameters
-
path full path to an existing local directory, to be added to the archive. destName the resulting name (or relative path) of the file in the archive.
Definition at line 293 of file karchive.cpp.
Writes a local file into the archive.
The main difference with writeFile, is that this method minimizes memory usage, by not loading the whole file into memory in one go.
If fileName
is a symbolic link, it will be written as is, i. e. it will not be resolved before.
- Parameters
-
fileName full path to an existing local file, to be added to the archive. destName the resulting name (or relative path) of the file in the archive.
Definition at line 205 of file karchive.cpp.
|
virtual |
Closes the archive.
Inherited classes might want to reimplement closeArchive instead.
- Returns
- true if close succeeded without problems
- See also
- open
Definition at line 164 of file karchive.cpp.
|
protectedpure virtual |
|
protectedvirtual |
Can be reimplemented in order to change the creation of the device (when using the fileName constructor).
By default this method uses KSaveFile when saving, and a simple QFile on reading. This method is called by open().
Reimplemented in KTar.
Definition at line 131 of file karchive.cpp.
QIODevice * KArchive::device | ( | ) | const |
const KArchiveDirectory * KArchive::directory | ( | ) | const |
If an archive is opened for reading, then the contents of the archive can be accessed via this function.
- Returns
- the directory of the archive
Definition at line 198 of file karchive.cpp.
Called after writing the data.
This virtual method must be implemented by subclasses.
- Parameters
-
size the size of the file
- See also
- finishWriting()
|
protectedpure virtual |
This virtual method must be implemented by subclasses.
Depending on the archive type not all metadata might be used.
- Parameters
-
name the name of the file user the user that owns the file group the group that owns the file size the size of the file perm permissions of the file. Use 0100644 if you don't have any more specific permissions to set. atime time the file was last accessed mtime modification time of the file ctime time of last status change
- See also
- prepareWriting
|
protectedpure virtual |
Write a directory to the archive.
This virtual method must be implemented by subclasses.
Depending on the archive type not all metadata might be used.
- Parameters
-
name the name of the directory user the user that owns the directory group the group that owns the directory perm permissions of the directory. Use 040755 if you don't have any other information. atime time the file was last accessed mtime modification time of the file ctime time of last status change
- See also
- writeDir
|
protectedpure virtual |
Writes a symbolic link to the archive.
This virtual method must be implemented by subclasses.
- Parameters
-
name name of symbolic link target target of symbolic link user the user that owns the directory group the group that owns the directory perm permissions of the directory atime time the file was last accessed mtime modification time of the file ctime time of last status change
- See also
- writeSymLink
QString KArchive::fileName | ( | ) | const |
The name of the archive file, as passed to the constructor that takes a fileName, or an empty string if you used the QIODevice constructor.
- Returns
- the name of the file, or QString() if unknown
Definition at line 485 of file karchive.cpp.
|
protected |
Ensures that path
exists, create otherwise.
This handles e.g. tar files missing directory entries, like mico-2.3.0.tar.gz :)
- Parameters
-
path the path of the directory
- Returns
- the directory with the given
path
Definition at line 406 of file karchive.cpp.
Call finishWriting after writing the data.
- Parameters
-
size the size of the file
- See also
- prepareWriting()
Definition at line 386 of file karchive.cpp.
bool KArchive::isOpen | ( | ) | const |
Checks whether the archive is open.
- Returns
- true if the archive is opened
Definition at line 480 of file karchive.cpp.
QIODevice::OpenMode KArchive::mode | ( | ) | const |
Returns the mode in which the archive was opened.
- Returns
- the mode in which the archive was opened (QIODevice::ReadOnly or QIODevice::WriteOnly)
- See also
- open()
Definition at line 470 of file karchive.cpp.
|
virtual |
Opens the archive for reading or writing.
Inherited classes might want to reimplement openArchive instead.
- Parameters
-
mode may be QIODevice::ReadOnly or QIODevice::WriteOnly
- See also
- close
Definition at line 104 of file karchive.cpp.
|
protectedpure virtual |
|
virtual |
Here's another way of writing a file into an archive: Call prepareWriting(), then call writeData() as many times as wanted then call finishWriting( totalSize ).
For tar.gz files, you need to know the size before hand, it is needed in the header! For zip files, size isn't used.
This method also allows some file metadata to be set. However, depending on the archive type not all metadata might be regarded.
- Parameters
-
name the name of the file user the user that owns the file group the group that owns the file size the size of the file perm permissions of the file atime time the file was last accessed mtime modification time of the file ctime time of last status change
Definition at line 375 of file karchive.cpp.
|
protectedvirtual |
Retrieves or create the root directory.
The default implementation assumes that openArchive() did the parsing, so it creates a dummy rootdir if none was set (write mode, or no '/' in the archive). Reimplement this to provide parsing/listing on demand.
- Returns
- the root directory
Definition at line 391 of file karchive.cpp.
|
protected |
Can be called by derived classes in order to set the underlying device.
Note that KArchive will -not- own the device, it must be deleted by the derived class.
Definition at line 456 of file karchive.cpp.
|
protected |
Derived classes call setRootDir from openArchive, to set the root directory after parsing an existing archive.
Definition at line 464 of file karchive.cpp.
|
protectedvirtual |
Reimplemented in KZip, KTar, and KAr.
Definition at line 860 of file karchive.cpp.
Write data into the current file - to be called after calling prepareWriting.
Reimplemented in KZip.
Definition at line 345 of file karchive.cpp.
|
virtual |
If an archive is opened for writing then you can add new directories using this function.
KArchive won't write one directory twice.
This method also allows some file metadata to be set. However, depending on the archive type not all metadata might be regarded.
- Parameters
-
name the name of the directory user the user that owns the directory group the group that owns the directory perm permissions of the directory atime time the file was last accessed mtime modification time of the file ctime time of last status change
Definition at line 359 of file karchive.cpp.
|
virtual |
If an archive is opened for writing then you can add a new file using this function.
If the file name is for example "mydir/test1" then the directory "mydir" is automatically appended first if that did not happen yet.
This method also allows some file metadata to be set. However, depending on the archive type not all metadata might be regarded.
- Parameters
-
name the name of the file user the user that owns the file group the group that owns the file data the data to write ( size
bytes)size the size of the file perm permissions of the file atime time the file was last accessed mtime modification time of the file ctime time of last status change
Definition at line 319 of file karchive.cpp.
|
virtual |
Writes a symbolic link to the archive if supported.
The archive must be opened for writing.
- Parameters
-
name name of symbolic link target target of symbolic link user the user that owns the directory group the group that owns the directory perm permissions of the directory atime time the file was last accessed mtime modification time of the file ctime time of last status change
Definition at line 366 of file karchive.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:47:10 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.