KDECore
#include <klocalsocket.h>
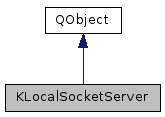
Signals | |
void | newConnection () |
Public Member Functions | |
KLocalSocketServer (QObject *parent=0) | |
virtual | ~KLocalSocketServer () |
void | close () |
QString | errorString () const |
virtual bool | hasPendingConnections () const |
bool | isListening () const |
bool | listen (const QString &path, KLocalSocket::LocalSocketType type=KLocalSocket::UnixSocket) |
QString | localPath () const |
KLocalSocket::LocalSocketType | localSocketType () const |
int | maxPendingConnections () const |
virtual KLocalSocket * | nextPendingConnection () |
QAbstractSocket::SocketError | serverError () const |
void | setMaxPendingConnections (int numConnections) |
bool | waitForNewConnection (int msec=0, bool *timedOut=0) |
Protected Member Functions | |
virtual void | incomingConnection (int handle) |
Detailed Description
KLocalSocketServer allows one to create a listening local socket and accept incoming connections.
On some platforms, local sockets are a kind of streaming socket that can be used to transmit and receive data just like Internet (TCP) streaming sockets. The difference is that they remain local to the host running them and cannot be accessed externally. They are also very fast and (in theory) consume less resources than standard TCP sockets.
KLocalSocketServer allows you to create the listening (i.e., passive) end of this local socket and accept incoming connections from users of KLocalSocket. It supports the same kind of socket types that KLocalSocket does (see KLocalSocket::LocalSocketType).
Definition at line 159 of file klocalsocket.h.
Constructor & Destructor Documentation
|
explicit |
Creates a KLocalSocketServer object with parent
as the parent object.
The object is created without binding to any address.
- Parameters
-
parent the parent object
Definition at line 120 of file klocalsocket.cpp.
|
virtual |
Destroys the KLocalSocketServer object and frees up any resource associated.
If the socket is still listening, it's closed (see close()).
The sockets that were accepted using this KLocalSocketServer object are not affected and will remain open. However, note that nextPendingConnection() returns objects that have this KLocalSocketServer as parents, so the QObject destruction will delete any objects that were not reparented.
Definition at line 125 of file klocalsocket.cpp.
Member Function Documentation
void KLocalSocketServer::close | ( | ) |
Closes the socket.
No further connections will be accepted, but connections that were already pending can still be retrieved with nextPendingConnection().
Connections that were accepted and are already open will not be affected.
Definition at line 150 of file klocalsocket.cpp.
QString KLocalSocketServer::errorString | ( | ) | const |
If an error occurred, return the error message.
Definition at line 220 of file klocalsocket.cpp.
|
virtual |
Returns true if a new socket can be received with nextPendingConnection().
Definition at line 188 of file klocalsocket.cpp.
|
protectedvirtual |
Definition at line 202 of file klocalsocket.cpp.
bool KLocalSocketServer::isListening | ( | ) | const |
Returns true if the socket is listening, false otherwise.
Definition at line 131 of file klocalsocket.cpp.
bool KLocalSocketServer::listen | ( | const QString & | path, |
KLocalSocket::LocalSocketType | type = KLocalSocket::UnixSocket |
||
) |
Binds this socket to the address path
and starts listening there.
If type
is KLocalSocket::UnixSocket, path
is treated as a Unix filesystem path and the calling user must have permission to create the named directory entry (that is, the user must have write permission to the parent directory, etc.)
If type
is KLocalSocket::AbstractUnixSocket, path
is just a name that can be anything. It'll be converted to an 8-bit identifier just as if it were a file path, but filesystem restrictions do not apply.
This function returns true if it succeeded in binding the socket to path
and placing it in listen mode. It returns false otherwise.
- Parameters
-
path the path to listen on type the local socket type
- Returns
- true on success, false otherwise
Definition at line 136 of file klocalsocket.cpp.
QString KLocalSocketServer::localPath | ( | ) | const |
Returns the address of this socket if it is listening on, or QString() if it is not listening.
Definition at line 175 of file klocalsocket.cpp.
KLocalSocket::LocalSocketType KLocalSocketServer::localSocketType | ( | ) | const |
Returns the socket type that this socket is listening on.
If it is not listening, returns QAbstractSocket::UnknownLocalSocketType.
Definition at line 170 of file klocalsocket.cpp.
int KLocalSocketServer::maxPendingConnections | ( | ) | const |
Returns the value set with setMaxPendingConnections().
Definition at line 165 of file klocalsocket.cpp.
|
signal |
The newConnection() signal is emitted whenever a new connection is ready and has been accepted.
Whenever it is emitted, calling nextPendingConnection() will return a valid object at least once.
|
virtual |
Returns a new socket if one is available or 0 if none is.
Note that the objects returned by this function will have the current KLocalSocketServer object as its parent. You may want to reparent the accepted objects if you intend them to outlive the current object.
Definition at line 193 of file klocalsocket.cpp.
QAbstractSocket::SocketError KLocalSocketServer::serverError | ( | ) | const |
If an error occurred, return the error code.
Definition at line 215 of file klocalsocket.cpp.
void KLocalSocketServer::setMaxPendingConnections | ( | int | numConnections | ) |
Sets the maximum number of connections that KLocalSocketServer will accept on your behalf and keep queued, ready to be retrieved with nextPendingConnection().
If you set numConnections
to 0, hasPendingConnections() will always return false. You can still use waitForNewConnection(), though.
- Parameters
-
numConnections the number of connections to accept and keep queued.
Definition at line 155 of file klocalsocket.cpp.
Suspends the execution of the calling thread for at most msec
milliseconds and wait for a new socket connection to be accepted (whichever comes first).
If no new socket connection is received within msec
milliseconds, consider this a time-out and set the boolean pointed by timedOut
to false (if it's not 0).
If msec
is 0, this call will not block, but will simply poll the system to check if a new connection has been received in the background.
Use msec
value of -1 to block indefinitely.
- Parameters
-
msec the time in milliseconds to block at most (-1 to block forever) timedOut points to a boolean that will be set to true if a timeout did occur
- Returns
- true if a new connection has been accepted or false if an error occurred or if the operation timed out.
Definition at line 180 of file klocalsocket.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:47:11 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.