KDECore
#include <k3resolverworkerbase.h>
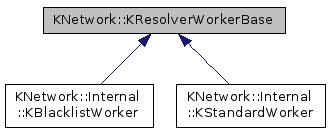
Classes | |
class | ResolverLocker |
Public Member Functions | |
KResolverWorkerBase () | |
virtual | ~KResolverWorkerBase () |
int | familyMask () const |
void | finished () |
int | flags () const |
QString | nodeName () const |
int | protocol () const |
QByteArray | protocolName () const |
QString | serviceName () const |
int | socketType () const |
Public Attributes | |
KResolverResults | results |
Protected Member Functions | |
void | acquireResolver () |
bool | checkResolver () |
bool | enqueue (KResolver *other) |
bool | enqueue (KResolverWorkerBase *worker) |
virtual bool | postprocess () |
virtual bool | preprocess ()=0 |
void | releaseResolver () |
virtual bool | run ()=0 |
void | setError (int errorcode, int syserror=0) |
Detailed Description
This class is the base functionality for a resolver worker. That is, the class that does the actual work.
In the future, this class might be exposed to allow plug-ins. So, try and make it binary compatible.
Note that hostnames are still encoded in Unicode at this point. It's up to the worker class to decide which encoding to use. In the case of DNS, an ASCII Compatible Encoding (ACE) must be used. See KResolver::domainToAscii().
Also specially note that the run method in this class is called in a thread that is not the program's main thread. So do not do anything there that you shouldn't!
- Deprecated:
- Use KSocketFactory or KLocalSocket instead
Definition at line 64 of file k3resolverworkerbase.h.
Constructor & Destructor Documentation
KResolverWorkerBase::KResolverWorkerBase | ( | ) |
Definition at line 41 of file k3resolverworkerbase.cpp.
|
virtual |
Definition at line 46 of file k3resolverworkerbase.cpp.
Member Function Documentation
|
protected |
This function has to be called from the resolver workers that require use of the DNS resolver code (i.e., res_* functions, generally in libresolv).
It indicates that the function is starting a resolution and that the resolver backend shouldn't change asynchronously.
If any pending res_init's are required, they will be performed before this function returns.
Definition at line 136 of file k3resolverworkerbase.cpp.
|
protected |
Checks the resolver subsystem status.
- Returns
- true if the resolver subsystem changed, false otherwise. If this function returns true, it might be necessary to restart the resolution altogether.
Definition at line 130 of file k3resolverworkerbase.cpp.
Enqueue the given resolver for post-processing.
Use this function to make the manager call for another resolution. This is suitable for workers that do post-processing.
The manager will make sure that any requests enqueued by this function are done before calling the postprocessing function, which you should override.
Important: do use KResolver's own enqueueing functions (i.e., KResolver::start()). Instead, use this function.
- Returns
- true on successful queuing or false if a problem ocurred
Definition at line 110 of file k3resolverworkerbase.cpp.
|
protected |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 116 of file k3resolverworkerbase.cpp.
int KResolverWorkerBase::familyMask | ( | ) | const |
gets the family mask
Definition at line 71 of file k3resolverworkerbase.cpp.
void KResolverWorkerBase::finished | ( | ) |
Call this function to indicate that processing has finished.
This is useful in the preprocessing stage, to indicate that run() doesn't have to be called.
Definition at line 100 of file k3resolverworkerbase.cpp.
int KResolverWorkerBase::flags | ( | ) | const |
gets the flags
Definition at line 64 of file k3resolverworkerbase.cpp.
QString KResolverWorkerBase::nodeName | ( | ) | const |
This is the hostname to be looked for.
Definition at line 50 of file k3resolverworkerbase.cpp.
|
protectedvirtual |
This function gets called during post processing for this class.
Important: this function gets called in the main event thread. And it MUST NOT block.
- Returns
- true on success
Reimplemented in KNetwork::Internal::KStandardWorker, and KNetwork::Internal::KBlacklistWorker.
Definition at line 105 of file k3resolverworkerbase.cpp.
|
protectedpure virtual |
This function gets called during pre processing for this class and you must override it.
Important: this function gets called in the main event thread. And it MUST NOT block.
This function can be used for an object to determine if it will be able to resolve the given data or not even before launching into a blocking operation. This function should return true if the object is capable of handling this kind of data; false otherwise. Note that the return value of 'true' means that the object's blocking answer will be considered authoritative.
This function MUST NOT queue further requests. Leave that to run().
This function is pure virtual; you must override it.
- Returns
- true on success
Implemented in KNetwork::Internal::KStandardWorker, and KNetwork::Internal::KBlacklistWorker.
int KResolverWorkerBase::protocol | ( | ) | const |
gets the protocol number
Definition at line 85 of file k3resolverworkerbase.cpp.
QByteArray KResolverWorkerBase::protocolName | ( | ) | const |
gets the protocol name, if applicable
Definition at line 92 of file k3resolverworkerbase.cpp.
|
protected |
This function is the counterpart for acquireResolver() - the worker thread indicates that it's done with the resolver.
Definition at line 142 of file k3resolverworkerbase.cpp.
|
protectedpure virtual |
This is the function that should be overridden in derived classes.
Derived classes will do their blocking job in this function and return either success or failure to work (not the lookup). That is, whether the lookup result was a domain found or not, if we got our answer, we should indicate success. The error itself must be set with setError().
Important: this function gets called in a separate thread!
- Returns
- true on success
Implemented in KNetwork::Internal::KStandardWorker, and KNetwork::Internal::KBlacklistWorker.
QString KResolverWorkerBase::serviceName | ( | ) | const |
And this is the service name.
Definition at line 57 of file k3resolverworkerbase.cpp.
|
inlineprotected |
Sets the error.
Definition at line 230 of file k3resolverworkerbase.h.
int KResolverWorkerBase::socketType | ( | ) | const |
gets the socket type
Definition at line 78 of file k3resolverworkerbase.cpp.
Member Data Documentation
KResolverResults KNetwork::KResolverWorkerBase::results |
Derived classes will put their resolved data in this list, or will leave it empty in case of error.
Status and error codes should also be stored in this object (the setError() function does that).
Definition at line 128 of file k3resolverworkerbase.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:47:12 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.