KDECore
#include <k3socketaddress.h>
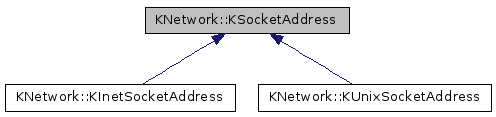
Public Member Functions | |
KSocketAddress () | |
KSocketAddress (const sockaddr *sa, quint16 len) | |
KSocketAddress (const KSocketAddress &other) | |
virtual | ~KSocketAddress () |
const sockaddr * | address () const |
sockaddr * | address () |
KInetSocketAddress & | asInet () |
KInetSocketAddress | asInet () const |
KUnixSocketAddress & | asUnix () |
KUnixSocketAddress | asUnix () const |
int | family () const |
int | ianaFamily () const |
quint16 | length () const |
virtual QString | nodeName () const |
operator const sockaddr * () const | |
KSocketAddress & | operator= (const KSocketAddress &other) |
bool | operator== (const KSocketAddress &other) const |
virtual QString | serviceName () const |
KSocketAddress & | setAddress (const sockaddr *sa, quint16 len) |
virtual KSocketAddress & | setFamily (int family) |
KSocketAddress & | setLength (quint16 len) |
virtual QString | toString () const |
Static Public Member Functions | |
static int | fromIanaFamily (int iana) |
static int | ianaFamily (int af) |
Protected Member Functions | |
KSocketAddress (KSocketAddressData *d) | |
Protected Attributes | |
KSocketAddressData * | d |
Detailed Description
A generic socket address.
This class holds one generic socket address.
- Deprecated:
- Use KSocketFactory or KLocalSocket instead
Definition at line 414 of file k3socketaddress.h.
Constructor & Destructor Documentation
KSocketAddress::KSocketAddress | ( | ) |
KSocketAddress::KSocketAddress | ( | const sockaddr * | sa, |
quint16 | len | ||
) |
Creates this object with the given data.
The raw socket address is copied into this object.
- Parameters
-
sa the socket address structure len the socket address length
Definition at line 412 of file k3socketaddress.cpp.
KSocketAddress::KSocketAddress | ( | const KSocketAddress & | other | ) |
Copy constructor.
This creates a copy of the other object.
Data is not shared.
- Parameters
-
other the object to copy from
Definition at line 418 of file k3socketaddress.cpp.
|
virtual |
|
protected |
extra constructor
Definition at line 424 of file k3socketaddress.cpp.
Member Function Documentation
const sockaddr * KSocketAddress::address | ( | ) | const |
Returns the socket address structure, to be passed down to low level functions.
Note that this function returns NULL for invalid or empty sockets, so you may use to to test for validity.
Definition at line 449 of file k3socketaddress.cpp.
sockaddr * KSocketAddress::address | ( | ) |
Returns the socket address structure, to be passed down to low level functions.
Note that this function returns NULL for invalid or empty sockets, so you may use to to test for validity.
The returned value, if not NULL, is an internal buffer which is guaranteed to be at least length() bytes long.
Definition at line 456 of file k3socketaddress.cpp.
KInetSocketAddress & KSocketAddress::asInet | ( | ) |
Returns an object reference that can be used to manipulate this socket as an Internet socket address.
Both objects share the same data.
Definition at line 628 of file k3socketaddress.cpp.
KInetSocketAddress KSocketAddress::asInet | ( | ) | const |
Returns an object is equal to this object's data, but they don't share it.
Definition at line 633 of file k3socketaddress.cpp.
KUnixSocketAddress & KSocketAddress::asUnix | ( | ) |
Returns an object reference that can be used to manipulate this socket as a Unix socket address.
Both objects share the same data.
Definition at line 638 of file k3socketaddress.cpp.
KUnixSocketAddress KSocketAddress::asUnix | ( | ) | const |
Returns an object is equal to this object's data, but they don't share it.
Definition at line 643 of file k3socketaddress.cpp.
int KSocketAddress::family | ( | ) | const |
Returns the family of this address.
- Returns
- the family of this address, AF_UNSPEC if it's undefined
Definition at line 487 of file k3socketaddress.cpp.
|
static |
Returns the address family of the given IANA family number.
- Returns
- the address family, AF_UNSPEC for unknown IANA family numbers
Definition at line 665 of file k3socketaddress.cpp.
|
inline |
Returns the IANA family number of this address.
- Returns
- the IANA family number of this address (1 for AF_INET. 2 for AF_INET6, otherwise 0)
Definition at line 541 of file k3socketaddress.h.
|
static |
Returns the IANA family number of the given address family.
Returns 0 if there is no corresponding IANA family number.
- Parameters
-
af the address family, in AF_* constants
- Returns
- the IANA family number of this address (1 for AF_INET. 2 for AF_INET6, otherwise 0)
Definition at line 648 of file k3socketaddress.cpp.
quint16 KSocketAddress::length | ( | ) | const |
Returns the length of this socket address structure.
Definition at line 473 of file k3socketaddress.cpp.
|
virtual |
Returns the node name of this socket.
In the case of Internet sockets, this is string representation of the IP address. The default implementation returns QString().
- Returns
- the node name, can be QString()
Definition at line 560 of file k3socketaddress.cpp.
|
inline |
Returns the socket address structure, to be passed down to low level functions.
Definition at line 490 of file k3socketaddress.h.
KSocketAddress & KSocketAddress::operator= | ( | const KSocketAddress & | other | ) |
Performs a shallow copy of the other object into this one.
Data will be copied.
- Parameters
-
other the object to copy from
Definition at line 440 of file k3socketaddress.cpp.
bool KSocketAddress::operator== | ( | const KSocketAddress & | other | ) | const |
Returns true if this equals the other socket.
Socket addresses are considered matching if and only if all data is the same.
- Parameters
-
other the other socket
- Returns
- true if both sockets are equal
Definition at line 503 of file k3socketaddress.cpp.
|
virtual |
Returns the service name for this socket.
In the case of Internet sockets, this is the port number. The default implementation returns QString().
- Returns
- the service name, can be QString()
Definition at line 586 of file k3socketaddress.cpp.
KSocketAddress & KSocketAddress::setAddress | ( | const sockaddr * | sa, |
quint16 | len | ||
) |
Sets the address to the given address.
The raw socket address is copied into this object.
- Parameters
-
sa the socket address structure len the socket address length
Definition at line 463 of file k3socketaddress.cpp.
|
virtual |
Sets the family of this object.
Note: setting the family will probably invalidate any address data contained in this object. Use this function with care.
- Parameters
-
family the new family to set
Definition at line 494 of file k3socketaddress.cpp.
KSocketAddress & KSocketAddress::setLength | ( | quint16 | len | ) |
Sets the length of this socket structure.
Use this function with care. It allows you to resize the internal buffer to fit needs. This function should not be used except for handling unknown socket address structures.
Also note that this function may invalidate the socket if a known family is set (Internet or Unix socket) and the new length would be too small to hold the system's sockaddr_* structure. If unsure, reset the family:
- Parameters
-
len the new length
Definition at line 480 of file k3socketaddress.cpp.
|
virtual |
Returns this socket address as a string suitable for printing.
Family, node and service are part of this address.
Definition at line 606 of file k3socketaddress.cpp.
Member Data Documentation
|
protected |
private data
Definition at line 609 of file k3socketaddress.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:47:12 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.