KDEUI
#include <kdatepicker.h>
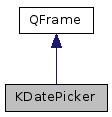
Signals | |
void | dateChanged (const QDate &date) |
void | dateEntered (const QDate &date) |
void | dateSelected (const QDate &date) |
void | tableClicked () |
Public Member Functions | |
KDatePicker (QWidget *parent=0) | |
KDatePicker (const QDate &dt, QWidget *parent=0) | |
virtual | ~KDatePicker () |
const KCalendarSystem * | calendar () const |
const QDate & | date () const |
KDateTable * | dateTable () const |
int | fontSize () const |
bool | hasCloseButton () const |
bool | setCalendar (KCalendarSystem *calendar=0) |
bool | setCalendar (const QString &calendarType) |
bool | setCalendarSystem (KLocale::CalendarSystem calendarSystem) |
void | setCloseButton (bool enable) |
bool | setDate (const QDate &date) |
void | setEnabled (bool enable) |
void | setFontSize (int) |
QSize | sizeHint () const |
Protected Slots | |
void | dateChangedSlot (const QDate &date) |
void | lineEnterPressed () |
void | monthBackwardClicked () |
void | monthForwardClicked () |
void | selectMonthClicked () |
void | selectYearClicked () |
void | tableClickedSlot () |
void | todayButtonClicked () |
void | uncheckYearSelector () |
void | weekSelected (int) |
void | yearBackwardClicked () |
void | yearForwardClicked () |
Protected Member Functions | |
virtual bool | eventFilter (QObject *o, QEvent *e) |
virtual void | resizeEvent (QResizeEvent *) |
Properties | |
bool | closeButton |
QDate | date |
int | fontSize |
Detailed Description
A date selection widget.
Provides a widget for calendar date input.
Different from the previous versions, it now emits two types of signals, either
dateSelected() or dateEntered() (see documentation for both signals).
A line edit has been added in the newer versions to allow the user to select a date directly by entering numbers like 19990101 or 990101.
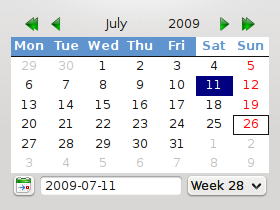
@author Tim Gilman, Mirko Boehm
Definition at line 55 of file kdatepicker.h.
Constructor & Destructor Documentation
|
explicit |
The constructor.
The current date will be displayed initially.
Definition at line 214 of file kdatepicker.cpp.
|
explicit |
The constructor.
The given date will be displayed initially.
Definition at line 219 of file kdatepicker.cpp.
|
virtual |
The destructor.
Definition at line 331 of file kdatepicker.cpp.
Member Function Documentation
const KCalendarSystem * KDatePicker::calendar | ( | ) | const |
Returns the currently selected calendar system.
- Returns
- a KCalendarSystem object
Definition at line 396 of file kdatepicker.cpp.
const QDate& KDatePicker::date | ( | ) | const |
- Returns
- the selected date.
|
signal |
This signal is emitted each time the selected date is changed.
Usually, this does not mean that the date has been entered, since the date also changes, for example, when another month is selected.
- See also
- dateSelected
|
protectedslot |
Definition at line 358 of file kdatepicker.cpp.
|
signal |
This signal is emitted when enter is pressed and a VALID date has been entered before into the line edit.
Connect to both dateEntered() and dateSelected() to receive all events where the user really enters a date.
|
signal |
This signal is emitted each time a day has been selected by clicking on the table (hitting a day in the current month).
It has the same meaning as dateSelected() in older versions of KDatePicker.
KDateTable * KDatePicker::dateTable | ( | ) | const |
- Returns
- the KDateTable widget child of this KDatePicker widget.
Definition at line 554 of file kdatepicker.cpp.
to catch move keyEvents when QLineEdit has keyFocus
Definition at line 336 of file kdatepicker.cpp.
int KDatePicker::fontSize | ( | ) | const |
Returns the font size of the widget elements.
bool KDatePicker::hasCloseButton | ( | ) | const |
- Returns
- true if a KDatePicker shows a close-button.
- See also
- setCloseButton
Definition at line 668 of file kdatepicker.cpp.
|
protectedslot |
Definition at line 559 of file kdatepicker.cpp.
|
protectedslot |
Definition at line 424 of file kdatepicker.cpp.
|
protectedslot |
Definition at line 416 of file kdatepicker.cpp.
|
protectedvirtual |
the resize event
Definition at line 353 of file kdatepicker.cpp.
|
protectedslot |
Definition at line 458 of file kdatepicker.cpp.
|
protectedslot |
Definition at line 495 of file kdatepicker.cpp.
bool KDatePicker::setCalendar | ( | KCalendarSystem * | calendar = 0 | ) |
Changes the calendar system to use.
Can use its own local locale if set.
- Parameters
-
calendar the calendar system object to use, defaults to global
- Returns
true
if the calendar system was successfully set,false
otherwise
Definition at line 401 of file kdatepicker.cpp.
Changes the calendar system to use.
Will always use global locale.
- Parameters
-
calendarType the calendar system type to use
- Returns
true
if the calendar system was successfully set,false
otherwise
Definition at line 406 of file kdatepicker.cpp.
bool KDatePicker::setCalendarSystem | ( | KLocale::CalendarSystem | calendarSystem | ) |
- Since
- 4.6
Changes the calendar system to use. Will always use global locale.
- Parameters
-
calendarSystem the calendar system to use
- Returns
true
if the calendar system was successfully set,false
otherwise
Definition at line 411 of file kdatepicker.cpp.
void KDatePicker::setCloseButton | ( | bool | enable | ) |
By calling this method with enable
= true, KDatePicker will show a little close-button in the upper button-row.
Clicking the close-button will cause the KDatePicker's topLevelWidget()'s close() method being called. This is mostly useful for toplevel datepickers without a window manager decoration.
- See also
- hasCloseButton
Definition at line 645 of file kdatepicker.cpp.
bool KDatePicker::setDate | ( | const QDate & | date | ) |
Sets the date.
- Returns
false
and does not change anything if the date given is invalid.
Definition at line 389 of file kdatepicker.cpp.
void KDatePicker::setEnabled | ( | bool | enable | ) |
Enables or disables the widget.
Definition at line 538 of file kdatepicker.cpp.
void KDatePicker::setFontSize | ( | int | s | ) |
Sets the font size of the widgets elements.
Definition at line 583 of file kdatepicker.cpp.
QSize KDatePicker::sizeHint | ( | void | ) | const |
The size hint for date pickers.
The size hint recommends the minimum size of the widget so that all elements may be placed without clipping. This sometimes looks ugly, so when using the size hint, try adding 28 to each of the reported numbers of pixels.
Definition at line 578 of file kdatepicker.cpp.
|
signal |
This signal is emitted when the day has been selected by clicking on it in the table.
|
protectedslot |
Definition at line 378 of file kdatepicker.cpp.
|
protectedslot |
Definition at line 572 of file kdatepicker.cpp.
|
protectedslot |
Definition at line 530 of file kdatepicker.cpp.
|
protectedslot |
Definition at line 448 of file kdatepicker.cpp.
|
protectedslot |
Definition at line 440 of file kdatepicker.cpp.
|
protectedslot |
Definition at line 432 of file kdatepicker.cpp.
Property Documentation
|
readwrite |
Definition at line 60 of file kdatepicker.h.
|
readwrite |
Definition at line 58 of file kdatepicker.h.
|
readwrite |
Definition at line 61 of file kdatepicker.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:17 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.